Rest parameters and spread syntax
Many JavaScript built-in functions support an arbitrary number of arguments.
For instance:
- Math.max(arg1, arg2, ..., argN) – returns the greatest of the arguments.
- Object.assign(dest, src1, ..., srcN) – copies properties from src1..N into dest .
- …and so on.
In this chapter we’ll learn how to do the same. And also, how to pass arrays to such functions as parameters.
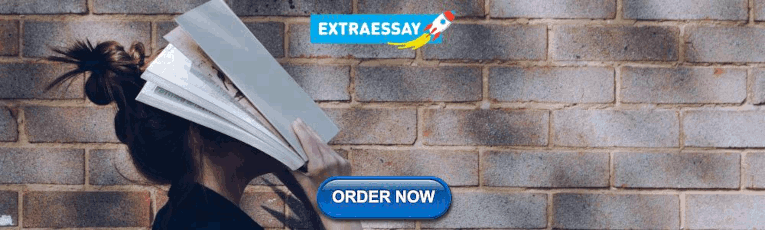
Rest parameters ...
A function can be called with any number of arguments, no matter how it is defined.
There will be no error because of “excessive” arguments. But of course in the result only the first two will be counted, so the result in the code above is 3 .
The rest of the parameters can be included in the function definition by using three dots ... followed by the name of the array that will contain them. The dots literally mean “gather the remaining parameters into an array”.
For instance, to gather all arguments into array args :
We can choose to get the first parameters as variables, and gather only the rest.
Here the first two arguments go into variables and the rest go into titles array:
The rest parameters gather all remaining arguments, so the following does not make sense and causes an error:
The ...rest must always be last.
The “arguments” variable
There is also a special array-like object named arguments that contains all arguments by their index.
In old times, rest parameters did not exist in the language, and using arguments was the only way to get all arguments of the function. And it still works, we can find it in the old code.
But the downside is that although arguments is both array-like and iterable, it’s not an array. It does not support array methods, so we can’t call arguments.map(...) for example.
Also, it always contains all arguments. We can’t capture them partially, like we did with rest parameters.
So when we need these features, then rest parameters are preferred.
If we access the arguments object from an arrow function, it takes them from the outer “normal” function.
Here’s an example:
As we remember, arrow functions don’t have their own this . Now we know they don’t have the special arguments object either.
Spread syntax
We’ve just seen how to get an array from the list of parameters.
But sometimes we need to do exactly the reverse.
For instance, there’s a built-in function Math.max that returns the greatest number from a list:
Now let’s say we have an array [3, 5, 1] . How do we call Math.max with it?
Passing it “as is” won’t work, because Math.max expects a list of numeric arguments, not a single array:
And surely we can’t manually list items in the code Math.max(arr[0], arr[1], arr[2]) , because we may be unsure how many there are. As our script executes, there could be a lot, or there could be none. And that would get ugly.
Spread syntax to the rescue! It looks similar to rest parameters, also using ... , but does quite the opposite.
When ...arr is used in the function call, it “expands” an iterable object arr into the list of arguments.
For Math.max :
We also can pass multiple iterables this way:
We can even combine the spread syntax with normal values:
Also, the spread syntax can be used to merge arrays:
In the examples above we used an array to demonstrate the spread syntax, but any iterable will do.
For instance, here we use the spread syntax to turn the string into array of characters:
The spread syntax internally uses iterators to gather elements, the same way as for..of does.
So, for a string, for..of returns characters and ...str becomes "H","e","l","l","o" . The list of characters is passed to array initializer [...str] .
For this particular task we could also use Array.from , because it converts an iterable (like a string) into an array:
The result is the same as [...str] .
But there’s a subtle difference between Array.from(obj) and [...obj] :
- Array.from operates on both array-likes and iterables.
- The spread syntax works only with iterables.
So, for the task of turning something into an array, Array.from tends to be more universal.
Copy an array/object
Remember when we talked about Object.assign() in the past ?
It is possible to do the same thing with the spread syntax.
Note that it is possible to do the same thing to make a copy of an object:
This way of copying an object is much shorter than let objCopy = Object.assign({}, obj) or for an array let arrCopy = Object.assign([], arr) so we prefer to use it whenever we can.
When we see "..." in the code, it is either rest parameters or the spread syntax.
There’s an easy way to distinguish between them:
- When ... is at the end of function parameters, it’s “rest parameters” and gathers the rest of the list of arguments into an array.
- When ... occurs in a function call or alike, it’s called a “spread syntax” and expands an array into a list.
Use patterns:
- Rest parameters are used to create functions that accept any number of arguments.
- The spread syntax is used to pass an array to functions that normally require a list of many arguments.
Together they help to travel between a list and an array of parameters with ease.
All arguments of a function call are also available in “old-style” arguments : array-like iterable object.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
JavaScript Destructuring and the Spread Operator – Explained with Example Code

JavaScript has two awesome data structures that help you write clean and efficient code. But handling them can get messy sometimes.
In this blog, I am going to show you how to handle destructuring in arrays and objects in JavaScript. We'll also learn how to use the spread operator as well.
Let's dive in.
What is Array Destructuring in JavaScript?
Let's say we have an array that contains five numbers, like this:
To get the elements from the array, we can do something like getting the number according to its indexes:
But this method is old and clunky, and there is a better way to do it – using array destructuring. It looks like this:
Both methods above will yield the same result:

Now, we have five elements in the array, and we print those. But what if we want to skip one element in between?
Here, we have skipped indexThird , and there's an empty space between indexTwo and indexFour.
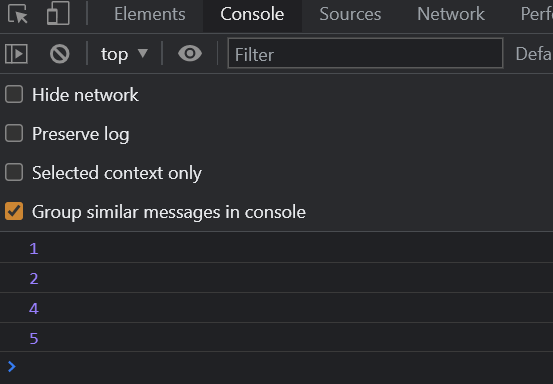
You can see that we are not getting the third element because we have set it as empty.
What is Object Destructuring in JavaScript?
This destructuring works well with objects too. Let me give you an example.
Let's say we want the name, salary, and weight from this object to be printed out in the console.
We can get them using the keys, which are name, salary, and weight.
But this code becomes difficult to understand sometimes. That's when destructuring comes in handy:
And now, we can just log name, salary, and weight instead of using that old method.
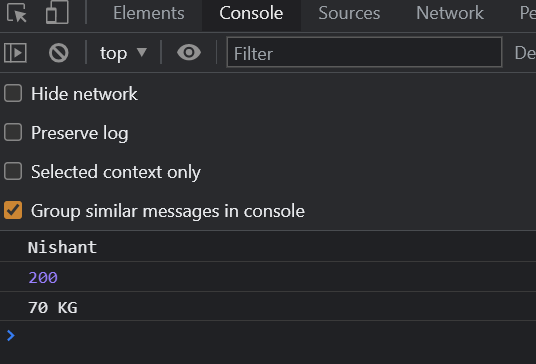
We can also use destructuring to set default values if the value is not present in the object.
Here, we have name and weight present in the object, but not the salary:

We will get an undefined value for the salary.
To correct that issue, we can set default values when we are destructuring the object.
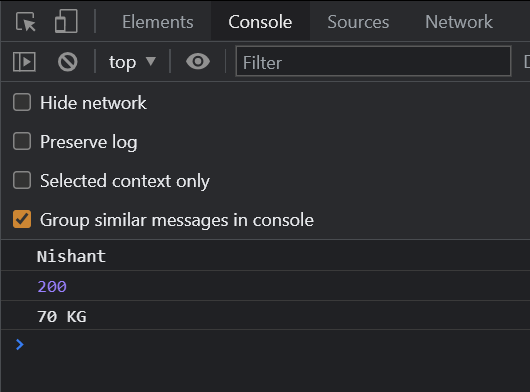
You can see that we get 200 as the Salary. This only works when we don't have that key in the object, and we want to set a default value.
Add salary in the object, and you will get 300 as the salary.
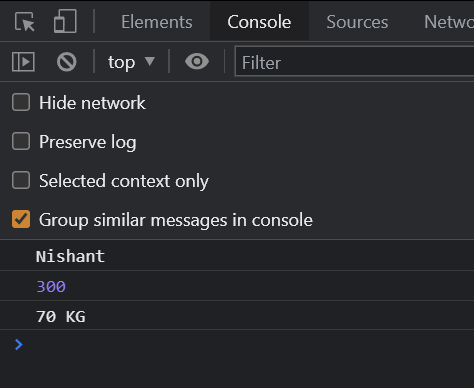
How to Use Object Destructuring with Functions
Let's say we have a function that prints all the data in the array to the console.
We are passing the object as a parameter in the function when it gets called:
Normally, we would do something like this – passing the object and logging it in the console.
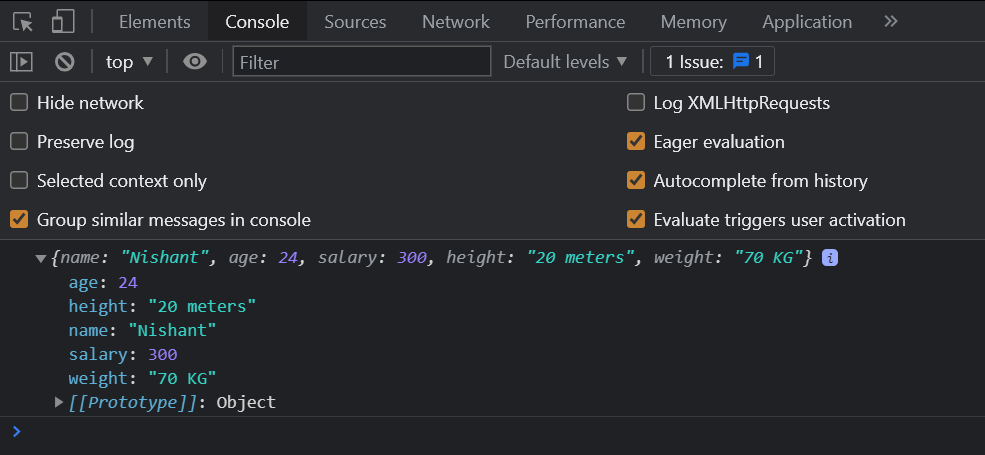
But again, we can do the same using destructuring.
Here, we are destructuring the object into name, age, salary, height and weight in the function parameters and we print everything on the same line.
You can see how destructuring makes it so much easier to understand.

Let's look at one last example.
We have a function here which accepts two numbers. It returns an array adding them and multiplying them and logs them into the console.

Let's use destructuring here instead.
We can destructure it into addition and multiplication variables like this:
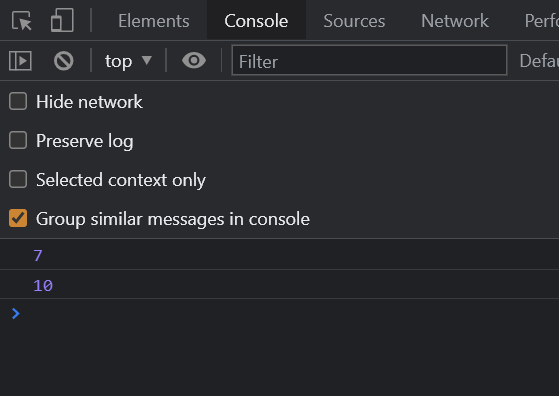
And in the output, you can see we get the addition and multiplication of both numbers.
What is the Spread Operator in JavaScript?
Spread means spreading or expanding. And the spread operator in JavaScript is denoted by three dots.
This spread operator has many different uses. Let's see them one by one.
Spread Operator Examples
Let's say we have two arrays and we want to merge them.

We are getting the combination of both arrays, which are array1 and array2.
But there is an easier way to do this:
In this case, we are using the spread operator to merge both arrays.

And you can see, we will get the same output.
Let's imagine another use case where we have to insert array1 between the elements of array2 .
For example, we want to insert array2 between the second and third element of array1 .
So, how do we do that? We can do something like this:
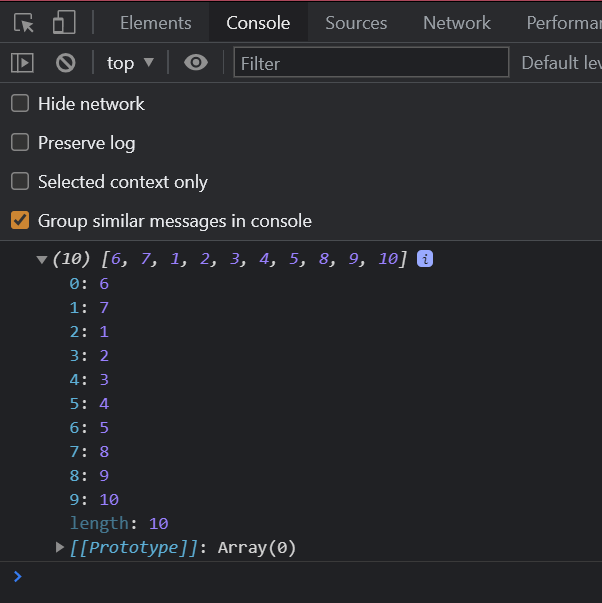
And you can see, we get the array1 elements between 7 and 8.
Now, let's merge two objects together using the spread operator.
We have two objects here. One contains firstName, age, and salary. The second one contains lastName, height, and weight.
Let's merge them together.
We have now merged both objects using the spread operator, and we've logged the value in the console.

You can see that we are getting the combination of both objects.
Lastly, we can also copy one array into another using the spread operator. Let me show you how it works:
Here, we are copying array1 into array2 using the spread operator.
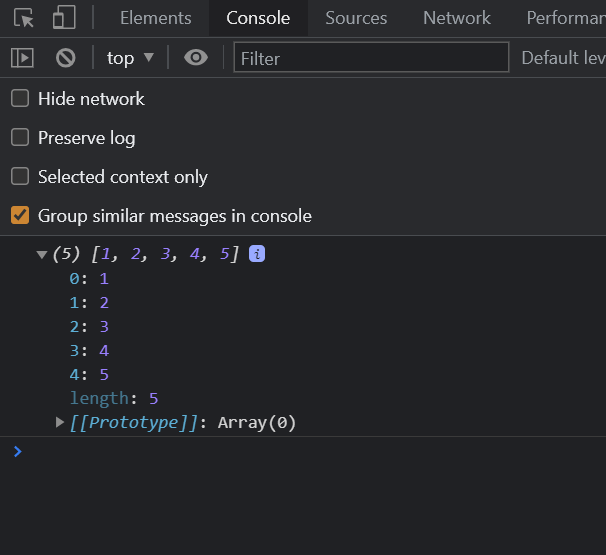
We are logging array2 in the console, and we are getting the items of array1 .
That's all, folks! In this article, we learned about array and object destructuring and the spread operator.
You can also watch my Youtube video on Array and Object Destructuring and the Spread Operator if you want to supplement your learning.
Happy Learning.
I build projects to learn how code works. And while I am not coding, I enjoy writing poetry and stories, playing the piano, and cooking delicious meals.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
- JavaScript Array reverse()
- JavaScript Math max()
- JavaScript Math min()
JavaScript Destructuring Assignment
- JavaScript Object.is()
The JavaScript spread operator ... is used to expand or spread out elements of an iterable, such as an array , string , or object .
This makes it incredibly useful for tasks like combining arrays, passing elements to functions as separate arguments, or even copying arrays.
Here's a quick example of the spread statement. You can read the rest of the tutorial for more.
Here, we used the spread operator ... inside console.log() to expand the numbers array into individual elements.
- JavaScript Spread Operator Inside Arrays
We can also use the spread operator inside arrays to expand the elements of another array. For example,
Here, ...fruits expands the fruits array inside the moreFruits2 array, which results in moreFruits2 consisting only of individual string elements and no inner arrays.
On the other hand, the moreFruits1 array consists of an inner array because we didn't expand the fruits array inside it.
Note: Since the spread operator was introduced in ES6 , some browsers may not support its use. To learn more, visit JavaScript Spread Operator support .
In JavaScript, objects are assigned by reference and not by values. For example,
Here, both variables arr1 and arr2 are referring to the same array. Hence, a change in one variable results in a change in both variables.
However, if you want to copy arrays so that they do not refer to the same array, you can use the spread operator. This way, the change in one array is not reflected in the other. For example,
- Spread Operator With Object
You can also use the spread operator with object literals. For example,
Here, the properties of obj1 and obj2 are added to obj3 using the spread operator.
However, when we add those two objects to obj4 without using the spread operator, we get obj1 and obj2 as keys for obj4 .
- JavaScript Rest Parameter
When the spread operator is used as a parameter, it is known as the rest parameter .
You can accept multiple arguments in a function call using the rest parameter. For example,
- When a single argument is passed to printArray() , the rest parameter takes only one parameter.
- When three arguments are passed, the rest parameter takes all three parameters.
Note : Using the rest parameter will pass the arguments as array elements.
- Spread Operator as Part of Function Argument
You can also use the spread operator as part of a function argument. For example,
If you pass multiple arguments using the spread operator, the function takes the required number of arguments and ignores the rest.
- JavaScript Program to Append an Object to an Array
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
JavaScript Tutorial
JavaScript typeof Operator
How to use JavaScript’s spread operator
JavaScript is a dynamic and potent language used by developers around the world to enhance web applications with complex, interactive, and rich features.
One such advanced feature in JavaScript’s arsenal is the spread operator . In this article, we’ll dive deep into what the spread operator is, its syntax, and how you can use it effectively in various programming scenarios. Armed with this knowledge, you’ll be able to simplify your code and harness the full potential of JavaScript.
What is the JavaScript Spread Operator?
The JavaScript spread operator ( ... ) is a handy syntax that allows you to ‘spread’ elements of an iterable (such as an array or string) into multiple elements.
Function Calls Made Easier ( ...args )
Simplifying array to arguments.
Imagine a scenario where you have a list of arguments as an array but your function expects individual arguments. Traditionally, you’d use the Function.prototype.apply method to achieve this. But with the spread operator, you can simply pass the array, and it would automatically be expanded to the individual arguments the function expects.
This shorthand not only looks cleaner but also saves you from additional, complicated steps.
Creation and Concatenation in Array Literals
Spreading elements into a new array.
You can use the spread operator within an array literal to incorporate all the elements of another array. This allows for more readable and maintainable code when compared to older methods of array manipulation such as push , splice , or concat .
This method is straightforward when you want to create a new array by merging other arrays without mutating the original ones.
Safe Copy with Spread
To copy an array, you might think of using assignment ( = ), but this only copies the reference, not the actual array. By using the spread operator, you create a shallow copy, thus preserving the integrity of the original array.
However, it’s essential to understand that this method only does a shallow copy. If you have nested arrays or objects, the references inside will still be shared between the original and the copied array.
Enhancing Object Literals
Merging and copying objects.
The spread operator can also be applied in object literals to combine properties of existing objects into a new object. It’s similar to Object.assign() , but the syntax is more concise and easy to read.
Here, clonedObj is a shallow copy of obj1 , and mergedObj is a new object with properties from both obj1 and obj2 . In case of a property conflict, the rightmost property takes precedence, as seen with the property foo .
Important Considerations
While the spread operator can make your code look neater and more intuitive, there are some performance and compatibility considerations you need to be conscious of:
- Performance : If you’re handling large datasets or performance-critical applications, be mindful of the spread operator’s limitations and alternative methods such as concat() or Object.assign() .
- Browser Compatibility : Most modern browsers support the spread operator, but always check the Browser Compatibility Data (BCD) tables for detailed information on cross-browser support.
- Rest vs. Spread : Don’t confuse spread with the rest syntax. The rest syntax looks similar but serves the opposite purpose—condensing multiple elements into a single one.
Let’s give a concluding example to tie everything together:
Conclusion and Practical Example
The spread operator is a flexible and powerful addition to the JavaScript language. Its ability to expand iterables into individual elements can greatly simplify your code and make it much easier to read.
Here’s a practical example to emphasize its utility:
As you can see, the spread operator transformed a two-dimensional array into a flat one with ease.
By understanding and applying the JavaScript spread operator, you can write cleaner, more expressive code. It isn’t just a tool for arrays and objects; it’s a new way of thinking that opens up a plethora of possibilities for JavaScript developers.
Take action : Review your current projects and find opportunities where replacing traditional logic with the spread operator could make your code simpler and more efficient. Happy coding!
Related posts
- Skip to main content
- Select language
- Skip to search
- Add a translation
- Print this page
- Spread operator
A better push
This is an experimental technology, part of the ECMAScript 6 (Harmony) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
For function calls:
For array literals:
For destructuring:
A better apply
Example: it is common to use Function.prototype.apply in cases where you want to use an array as arguments to a function.
With ES6 spread you can now write the above as:
Any argument in the argument list can use the spread syntax and it can be used multiple times.
A more powerful array literal
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Just like with spread for argument lists ... can be used anywhere in the array literal and it can be used multiple times.
Apply for new
Example: In ES5 it is not possible to compose new with apply (in ES5 terms apply does a [[Call]] and not a [[Construct]] ). In ES6 the spread syntax naturally supports this:
Example: push is often used to push an array to the end of an existing array. In ES5 this is often done as:
In ES6 with spread this becomes:
Specifications
Browser compatibility.
- Rest parameters
Document Tags and Contributors
- Experimental
- Expérimental
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- JavaScript basics
- JavaScript technologies overview
- Introduction to Object Oriented JavaScript
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- Standard built-in objects
- ArrayBuffer
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Statements and declarations
- Legacy generator function
- for each...in
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- constructor
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template strings
- Deprecated features
- New in JavaScript
- ECMAScript 5 support in Mozilla
- ECMAScript 6 support in Mozilla
- ECMAScript 7 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Javascript Topics
Popular articles.
- Jsx: Markup Expressions In Javascript (May 04, 2024)
- The Tostring() Method (Apr 22, 2024)
- Understanding The Reflect Api In Javascript (Apr 22, 2024)
- Proxy Invariants And Usage Guidelines (Apr 22, 2024)
- Methods For Performing Meta-Level Operations On Objects (Apr 22, 2024)
The Spread Operator for Function Calls
Table of Contents
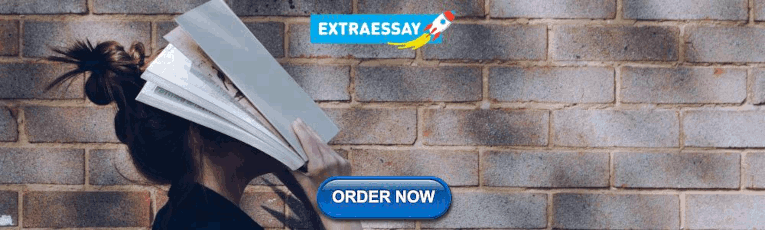
Introduction to the Spread Operator
Understanding function calls in javascript, the spread operator for function calls: syntax and usage, examples of the spread operator in function calls, benefits of using the spread operator in function calls, common mistakes and how to avoid them, comparing the spread operator with other javascript features, advanced concepts: spread operator with es6 and beyond, practical applications of the spread operator in real-world coding, summary and key takeaways.
The Spread Operator, denoted by three dots (...), is a powerful feature introduced in ES6, or ECMAScript 2015, a version of JavaScript. This operator has revolutionized the way developers work with arrays, objects, and functions in JavaScript. It allows an iterable such as an array expression or string to be expanded in places where zero or more arguments or elements are expected. In simpler terms, it 'spreads' out the elements of an iterable.
The spread operator can be used in various contexts, but in this article, we will focus on its application in function calls. Understanding the spread operator can significantly enhance your JavaScript coding skills, making your code more efficient and readable.
So, let's dive into the world of JavaScript and explore the spread operator in function calls.

What does the Spread Operator in JavaScript do?

What is the symbol for the Spread Operator in JavaScript?
In which version of JavaScript was the Spread Operator introduced?
What is the main focus of the study material in regard to the Spread Operator?
In JavaScript, functions are fundamental building blocks. They are objects that contain a sequence of statements called a function body. Functions can be invoked or called to execute these statements. The basic syntax for a function call is the function name followed by parentheses, which may contain arguments separated by commas.
In the above example, 'greet' is the function name, and 'John' is the argument passed to the function. When the function is called, it executes the code within its body.
- Arguments are values that we pass into the function when we call it. These values are then assigned to the function's parameters.
- Parameters are variables listed as part of the function definition. They act as placeholders for the values that will be input to the function.
In the 'add' function, 'a' and 'b' are parameters, and '2' and '3' are arguments. The function returns the sum of 'a' and 'b'. Understanding function calls is crucial as they form the basis of how we structure and organize code in JavaScript.
They allow us to write reusable code and make our programs more modular and efficient. In the next section, we will see how the spread operator can be used to enhance function calls.
What is the basic syntax for a function call in JavaScript?
In JavaScript, what are arguments in the context of functions?
What is the role of parameters in a JavaScript function?
What does a function call do in JavaScript?
What are arguments in JavaScript functions?
What are parameters in JavaScript functions?
What is the role of function calls in JavaScript?
What will be the output of the 'add' function if arguments '2' and '3' are passed?
The spread operator, represented by three dots (...), can be used in function calls to spread out elements of an array or object as individual arguments. This is particularly useful when you don't know how many arguments will be passed to the function, or when the arguments are already in an array or object.
In the above example, the 'sum' function expects three arguments. The 'numbers' array contains three elements. By using the spread operator, we can pass the elements of the 'numbers' array as individual arguments to the 'sum' function.
- The spread operator can be used with any iterable, not just arrays. This includes strings, sets, and even the arguments object in functions.
- The spread operator can be used multiple times in a function call, and it can be mixed with regular arguments.
In the 'greet' function, the first two arguments are regular parameters, while '...titles' is a rest parameter that collects all remaining arguments into an array. The spread operator allows us to handle an arbitrary number of arguments in a clean and efficient manner. The spread operator is a powerful tool that can make your JavaScript code more flexible and expressive.
It is an essential part of modern JavaScript and a must-know for any JavaScript developer.
What is the function of the spread operator in JavaScript?
In the 'greet' function example, what role does '...titles' play?
Can the spread operator be used multiple times in a function call?
Which of the following can the spread operator be used with?
What is the output of the 'sum' function in the given example?
What is the spread operator represented by in JavaScript?
What is the main functionality of the spread operator in function calls?
Can the spread operator be used with any iterable?
What does the '...titles' in the 'greet' function represent?
Let's explore some practical examples of using the spread operator in function calls.
1. Combining Arrays: The spread operator can be used to combine or concatenate multiple arrays.
In this example, the 'combineArrays' function takes two arrays as arguments and returns a new array that combines the elements of both arrays.
2. Finding the Maximum Value: The spread operator can be used with the 'Math.max' function to find the maximum value in an array.
Normally, 'Math.max' takes individual numbers as arguments. But with the spread operator, we can pass an array of numbers to 'Math.max'.
3. Copying Arrays: The spread operator can be used to create a shallow copy of an array.
In this example, the 'copy' array is a new array that contains all the elements of the 'original' array. Any changes to the 'original' array will not affect the 'copy' array.
These examples demonstrate the versatility and power of the spread operator in function calls. It is a tool that can simplify your code and make it more readable and efficient.
What is the output of 'console.log(combineArrays(array1, array2));' in the given material?
What does the spread operator do in the 'combineArrays' function?
What happens when the spread operator is used with the 'Math.max' function?
What is the purpose of the spread operator in the 'copy' array operation?
How can the spread operator be used with 'Math.max' function?
What is the outcome of the 'copy' array in the third example?
What will be the output of 'console.log(Math.max(...numbers))' where numbers = [1, 2, 3, 4, 5]?
What happens when the spread operator is used to create a copy of an array?
The spread operator brings several benefits to JavaScript programming, especially when used in function calls.
1. Simplicity and Readability: The spread operator simplifies the syntax for passing an array of arguments to a function. Instead of using the 'apply' method or a loop, you can use the spread operator directly. This makes the code more readable and easier to understand.
2. Flexibility: The spread operator can be used with any iterable, not just arrays. This includes strings, sets, and even the arguments object in functions. It can also be used multiple times in a function call, and it can be mixed with regular arguments.
3. Immutability: The spread operator creates a new array or object, leaving the original array or object untouched. This aligns with the principle of immutability, a key concept in functional programming and modern JavaScript frameworks like React.
4. Convenience: The spread operator can be used to perform common tasks more conveniently, such as combining arrays, finding the maximum or minimum value in an array, or copying an array. The spread operator is a powerful tool that can make your JavaScript code more flexible, readable, and efficient.
What benefits does the spread operator bring to JavaScript programming when used in function calls?
The spread operator can be used with which type of data?
When used, does the spread operator modify the original array or object?
What does the spread operator align with in functional programming?
What is one benefit of using the spread operator in JavaScript function calls?
Which of the following is NOT a benefit of using the spread operator in JavaScript?
What does the spread operator do in terms of immutability?
While the spread operator is a powerful tool, it's important to be aware of some common mistakes and pitfalls to avoid when using it in function calls.
1. Using the Spread Operator with Non-Iterables: The spread operator works with iterables, such as arrays, strings, and sets. If you try to use it with a non-iterable, like a number or a boolean, you will get an error.
To avoid this, always make sure the value you are spreading is an iterable.
2. Misunderstanding Shallow Copy: The spread operator creates a shallow copy of an array or object. This means that if the array or object contains other arrays or objects, those are copied by reference, not by value. Modifying the nested arrays or objects will affect the original array or object.
To avoid this, use a method that creates a deep copy if you need to copy nested arrays or objects.
3. Overusing the Spread Operator: While the spread operator is convenient, it can lead to performance issues if overused, especially with large arrays or objects. It's important to use it judiciously and consider other options if performance is a concern.
Remember, the spread operator is a tool, and like any tool, it's most effective when used appropriately. Understanding its strengths and limitations will help you use it effectively in your JavaScript code.
What happens when you try to use the spread operator with a non-iterable?
What kind of copy does the spread operator create of an array or object?
What can overusing the spread operator lead to?
What should you do if you need to copy nested arrays or objects?
What happens when you use the spread operator with a non-iterable?
What type of copy does the spread operator create?
What is the consequence of overusing the spread operator?
What will be the output of the following code snippet? let original = [{ a: 1 }, { b: 2 }]; let copy = [...original]; copy[0].a = 2; console.log(original[0].a);
How can we avoid the issue of shallow copy created by the spread operator?
The spread operator, while a powerful feature, is not the only way to handle multiple arguments in JavaScript. Let's compare it with some other JavaScript features.
1. Arguments Object: Before the spread operator was introduced, JavaScript provided the 'arguments' object, an array-like object that contains all arguments passed to a function. However, 'arguments' is not a real array and lacks array methods like 'map', 'reduce', and 'filter'. The spread operator, on the other hand, works with real arrays and is generally more flexible and powerful.
2. Apply Method: The 'apply' method can be used to call a function with an array of arguments. However, the syntax is more complex than using the spread operator, and 'apply' cannot be used with new operator to create new instances.
3. Rest Parameters: Rest parameters, denoted by '...', collect all remaining arguments into an array. While similar to the spread operator, rest parameters are used in function definitions, while the spread operator is used in function calls.
In conclusion, while there are other ways to handle multiple arguments in JavaScript, the spread operator offers a more modern, flexible, and powerful solution. It is a valuable addition to any JavaScript developer's toolkit.
What is the main difference between the 'arguments' object and the spread operator in JavaScript?
What is a limitation of the 'apply' method compared to the spread operator in JavaScript?
How do rest parameters differ from the spread operator in JavaScript?
Which feature allows you to handle multiple arguments in a more modern, flexible and powerful manner?
What is the major difference between the 'arguments' object and the spread operator in JavaScript?
What is the disadvantage of using the 'apply' method over the spread operator in JavaScript?
What is the difference between rest parameters and the spread operator in JavaScript?
What is the main advantage of using the spread operator in JavaScript?
The spread operator, introduced in ES6, has been further enhanced in subsequent versions of JavaScript. Let's explore some of these advanced concepts.
1. Spread Operator with Objects: ES9, or ECMAScript 2018, introduced the ability to use the spread operator with objects. This allows you to create a new object by combining the properties of existing objects.
2. Spread Operator with Destructuring: The spread operator can be used with destructuring assignment to extract and assign properties from an object to new variables.
3. Spread Operator with Array Literals: The spread operator can be used within array literals to create a new array that includes the elements of existing arrays.
These advanced concepts demonstrate the versatility and power of the spread operator in modern JavaScript. By mastering these concepts, you can write more efficient and expressive code.
What does the spread operator do when used with objects in ES9?
How can the spread operator be used with destructuring in JavaScript?
What is the output of the following code? let { a, ...rest } = { a: 1, b: 2, c: 3 }; console.log(a); console.log(rest);
What is the result of the following code? let array1 = [1, 2, 3]; let array2 = [4, 5, 6]; let combined = [...array1, ...array2]; console.log(combined);
Which version of ECMAScript introduced the ability to use the spread operator with objects?
What will be the output of the following code? let { a, ...rest } = { a: 1, b: 2, c: 3 }; console.log(a);
What does the spread operator do when used within array literals?
What will be the output of the following code? let array1 = [1, 2, 3]; let array2 = [4, 5, 6]; let combined = [...array1, ...array2]; console.log(combined);
The spread operator is not just a theoretical concept, but a practical tool that can be used in real-world coding. Here are some practical applications:
1. Merging Objects: In JavaScript frameworks like React, the spread operator is often used to merge state objects.
2. Passing Props in React: In React, the spread operator can be used to pass props to a component.
3. Copying Arrays in Redux: In Redux, the spread operator can be used to create a copy of an array when updating the state.
4. Flattening Arrays: The spread operator can be used to flatten nested arrays.
These examples demonstrate how the spread operator can be used in real-world coding. By understanding and applying these concepts, you can write more efficient and expressive code.
What is the practical use of the spread operator in React?
How can the spread operator be used in Redux?
What is the result of the code 'let newState = { ...state, age: 31 };' if 'let state = { name: 'John', age: 30 };'?
What is the purpose of the spread operator in the React component '<MyComponent {...props} />'?
What is one practical use of the spread operator in JavaScript?
How can the spread operator be used in React?
What is the result of using the spread operator on the array [1, 2, 3] and adding 4 like [...array, 4]?
How can the spread operator be used with nested arrays?
We've covered a lot of ground in this article, exploring the spread operator in JavaScript and its application in function calls. Here are the key takeaways:
1. The spread operator, introduced in ES6, allows an iterable to be expanded in places where zero or more arguments or elements are expected. It is represented by three dots (...).
2. The spread operator can be used in function calls to pass the elements of an array or object as individual arguments. This is particularly useful when the number of arguments is unknown or when the arguments are already in an array or object.
3. The spread operator can simplify your code, making it more readable and efficient. It can be used to perform common tasks like combining arrays, finding the maximum value in an array, or copying an array.
4. However, it's important to avoid common mistakes when using the spread operator, such as using it with non-iterables, misunderstanding shallow copy, or overusing it.
5. The spread operator has been further enhanced in subsequent versions of JavaScript, with features like spread with objects, spread with destructuring, and spread with array literals.
6. In real-world coding, the spread operator can be used in various scenarios, such as merging objects, passing props in React, copying arrays in Redux, or flattening arrays.
In conclusion, the spread operator is a powerful tool in JavaScript that can enhance your coding skills and make your code more flexible, readable, and efficient. It is an essential part of modern JavaScript and a must-know for any JavaScript developer.
What symbol represents the spread operator in JavaScript?
What is the primary use of the spread operator in JavaScript?
What does the spread operator not work with?
Which of the following is not a common task that the spread operator can be used for?
What is the common mistake made when using the spread operator?
What does the spread operator allow in JavaScript?
How is the spread operator represented in JavaScript?
What can be a common mistake when using the spread operator in JavaScript?
In which scenarios can the spread operator be used in real-world coding?
How TO - The Spread Operator (...)
Learn how to use the three dots operator (...) a.k.a the spread operator in JavaScript.
The Spread Operator
The JavaScript spread operator ( ... ) expands an iterable (like an array) into more elements.
This allows us to quickly copy all or parts of an existing array into another array:
Assign the first and second items from numbers to variables and put the rest in an array:
The spread operator is often used to extract only what's needed from an array:
We can use the spread operator with objects too:
Notice the properties that did not match were combined, but the property that did match, color , was overwritten by the last object that was passed, updateMyVehicle . The resulting color is now yellow.
See also : JavaScript ES6 Tutorial .

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
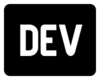
DEV Community

Posted on Sep 25, 2019 • Updated on Sep 27, 2019
Understanding the JavaScript Spread Operator - From Beginner to Expert Part 2
Introduction.
The spread operator, …, was first introduced in ES6. It quickly became one of the most popular features. So much so that despite the fact it only worked on arrays, a proposal was made to extend its functionalities to objects. This feature was finally introduced in ES9.
The goal of this tutorial, which is divided into two parts, is to show you why the spread operator should be used, how it works, and to deep dive into its uses, from the most basic to the most advanced. If you haven't read the first part of this tutorial, I encourage you to do so! Here is the link:
Understanding the JavaScript Spread Operator - From Beginner to Expert
Here is a short summary of the contents of this tutorial:
- Why the spread operator should be used
- Cloning arrays/objects
- Converting array-like structures to array
- The spread operator as an argument
- Adding elements to arrays/objects
- Merging arrays/objects
- Destructuring nested elements
Adding conditional properties
Short circuiting, the rest parameter (…), default destructuring values.
- Default properties
Cloning arrays/objects with nested elements
In the first part of this article, we learnt about reference data types, accidental variable mutation, and how we could solve this problem by cloning arrays/objects immutably , with the spread operator.
However, there's a slight problem with this approach, when it comes to nested reference data types: The spread operator only performs a shallow clone . What does this mean? If we attempt to clone an object that contains an array, for example, the array inside the cloned object will contain a reference to the memory address where the original array is stored… This means that, while our object is immutable, the array inside it isn't . Here's an example to illustrate this:
As you can see, our squirtleClone has been cloned immutably . When we change the name property of the original pokemon object to 'Charmander', our squirtleClone isn't affected, its name property isn't mutated .
However, when we add a new ability to the abilities property of the original pokemon object… Our squirtleClone 's abilities are affected by the change . Because the abilities property is a reference data type , it isn't cloned immutably. Welcome to the reality of JavaScript :)
One of the solutions to this problem would be to use the spread operator to clone the nested properties, as shown in the following example:
For obvious reasons, this isn't an ideal approach to solving our problem. We would need to use the spread operator for every single reference type property, which is why this approach is only valid for small objects. So, which is the optimal solution? Deep cloning .
Since there is plenty to say about deep cloning, I won't be going into too much detail. I'd just like to say that the correct of deep cloning is either using an external library (for example, Lodash ), or writing ourselves a function that does it.
Sometimes we need to add properties to an object, but we don't know whether or not those properties exist. This doesn't pose much of a problem, we can always check if the property exists with an if statement:
There is, however, a much simpler way of achieving the same result, by using short circuiting conditionals with the && operator . A brief explanation:
When we evaluate an expression with &&, if the first operand is false , JavaScript will short-circuit and ignore the second operand .
Let's take a look at the following code:
If starterPokemon.length > 0 is false (the array is empty), the statement will short circuit, and our choosePokemon function will never be executed . This is why the previous code is equivalent to using the traditional if statement.
Going back to our original problem, we can take advantage of the logical AND operator to add conditional properties to an object. Here's how:
What's going on here? Allow me to explain:
As we already know, by using the && operator, the second part of the statement will only be executed if the first operand is true . Therefore, only if the abilities variable is true (if the variable exists), will the second half of the statement be executed. What does this second half do? It creates an object containing the abilities variable , which is then destructured with the spread operator placed in front of the statement , thus adding the existent abilities variable into our fullPokemon object immutably .
Before we can introduce our final advanced spreading use, adding default properties to objects, we must first dive into two new concepts: default destructuring values , and the rest parameter . Once we are familiar with these techniques, we will be able to combine them to add default properties to objects .
If we try to destructure an array element or object property that doesn't exist, we will get an undefined variable. How can we avoid undefined values? By using defaults . How does this work?
We can assign default values to the variables we destructure, inside the actual destructuring statement. Here's an example:
As you can see, by adding the default value 'Water' to the type variable in the destructuring statement, we avoid an undefined variable in the case of the pokemon object not having the type property.
You may be surprised to hear that the spread operator is overloaded . This means that it has more than one function. It's second function is to act as the rest parameter .
From the MDN docs : A function's last parameter can be prefixed with ... which will cause all remaining (user supplied) arguments to be placed within a "standard" javascript array. Only the last parameter can be a "rest parameter".
Simply put, the rest operator takes all remaining elements (this is the reason it's named rest, as in the rest of the elements :p ) and places them into an array. Here's an example:
As you can see, we can pass as many abilities as we want to the printPokemon function. Every single value we introduce after the type parameter (the rest of the parameters) will be collected into an array , which we then turn into a string with the join function, and print out.
Note: Remember that the rest parameter must be the last parameter , or an error will occur.
The rest parameter can also be used when destructuring , which is the part that interests us. It allows us to obtain the remaining properties in an object , and store them in an array. Here's an example of the rest parameter used in a destructuring assignment:
As shown above, we can use the rest operator to destructure the remaining properties in the pokemon object. Like in the previous example, our pokemon object can have as many properties as we want defined after the id property, they will all be collected by the rest parameter.
Now that we know how the rest parameter works, and how to apply it in destructuring assignments, let's return to dealing with default properties .
Adding default properties
Sometimes, we have a large amount of similar objects, that aren't quite exactly the same. Some of them lack properties that the other objects do have. However, we need all of our objects to have the same properties , simply for the sake of order and coherence. How can we achieve this?
By setting default properties . These are properties with a default value that will be added to our object, if it doesn't already have that property. By using the rest parameter combined with default values and the spread operator , we can add default properties to an object. It may sound a bit daunting, but it's actually quite simple. Here's an example of how to do it:
What's going on in the previous code fragment? Let's break it down:
As you can see, when we destructure the abilities property, we are adding a default value ( [] ). As we already know, the default value will only be assigned to the abilities variable if it doesn't exist in the pokemon object . In the same line, we are gathering the remaining properties (name and type) of the pokemon object into a variable named rest, by making use of the awesome rest parameter .
On line 7, we are spreading the rest variable (which, as you can see, is an object containing the name and type properties) inside an object literal, to generate a new object. We are also adding the abilities variable, which in this case, is an empty array , since that is what we specified as its default value on the previous line.
In the case of our original pokemon object already having an abilities property, the previous code would not have modified it , and it would maintain its original value .
So, this is how we add default properties to an object. Let's place the previous code into a function, and apply it to a large collection of objects:
As you can see, all the pokemon in the array now have an abilities property. In the case of charmander and bulbasur , they have an empty array, since that is the default value we assigned. However, the squirtle object maintains its original array of abilities .
There are, of course, other ways of adding default properties to an object, mainly by using if statements. However, I wanted to show an interesting new way of doing it, by using a combination of default values, the rest parameter, and the spread operator. You can then choose which approach suits you best :)
This is the second and final part of the Understanding the JavaScript Spread Operator - From Beginner to Expert tutorial. Here's a link to the first part .
In this second part of the tutorial we have learnt some more advanced uses of the spread operator, which include destructuring nested elements, adding conditional properties and adding default properties. We have also learnt three interesting JS concepts: short-circuiting, default destructuring values and the rest parameter.
I sincerely hope you've found this piece useful, thank you for reading :) If you can think of any more uses of the spread operator or would like to comment something, don't hesitate to reach out, here's a link to my Twitter page .
Top comments (10)
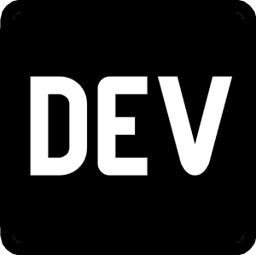
Templates let you quickly answer FAQs or store snippets for re-use.

- Email [email protected]
- Location Chile
- Education Coding Bootcamps on Desafío Latam, Escalab and Coderhouse, and self-education
- Work Senior Frontend Developer at AAXIS
- Joined Sep 25, 2019
Thanks a lot for these tutorials! I recently learned about the spread and rest operators on freecodecamp but I didn't fully understand them, your tutoriales helped me a lot! :)

- Joined Feb 25, 2019
I'm so glad you found them helpful, it's the reason I write them for! :D

- Location Málaga
- Education PhD. Computer Science
- Joined Feb 22, 2019

- Location Medellín, Colombia
- Education Systems Engineer
- Work Senior Software Developer
- Joined Mar 5, 2018
Oh Nya this series was incredible. Thanks a lot.
You have a little mistake, nested-object-clone.js was used two times.
I highly recommended change this gist to dev's liquid tags.

- Joined May 20, 2018
Nice tutorial!
Thank you :)

- Location Croatia
- Education University of Economics
- Joined May 15, 2019
Both of your articles about spread operator really cleared it up for me, thank you for writing this!
Thanks to you for reading!

- Joined Jun 3, 2018
Thanks again for such a nice tutorial.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
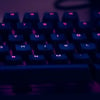
The String Type
Paul Ngugi - May 6

Structuring Javascript Code
Thapelo Letsapa - May 6
Common Mathematical Functions
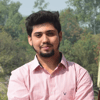
MULTITHREADING IN JAVA
gauharnawab - May 6
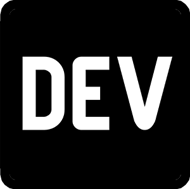
We're a place where coders share, stay up-to-date and grow their careers.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript Spread Operator
- JavaScript Operators
- JavaScript typeof Operator
- JavaScript String Operators
- JavaScript Unary Operators
- JavaScript in Operator
- JavaScript Operators Reference
- Spread vs Rest operator in JavaScript ES6
- ES6 Spread Operator
- JavaScript Comma Operator
- JavaScript Logical Operators
- JavaScript Bitwise Operators
- JavaScript Remainder Assignment(%=) Operator
- What is typeof Operator in JavaScript ?
- OR(||) Logical Operator in JavaScript
- OR(|) Bitwise Operator in JavaScript
- Remainder Assignment(%=) Operator in Javascript
- XOR(^) Bitwise Operator in JavaScript
- JavaScript Arithmetic Operators
- Dart - Spread Operator (...)
The Spread operator allows an iterable to expand in places where 0+ arguments are expected. It is mostly used in the variable array where there is more than 1 value is expected. It allows us the privilege to obtain a list of parameters from an array.
The syntax of the Spread operator is the same as the Rest parameter but it works opposite of it.
Note : To run the code in this article make use of the console provided by the browser.
Example 1: In this example, we are using the spread operator.
Note : Though we can achieve the same result as the concat method, it is not recommended to use the spread in this particular case, as for a large data set it will work slower when compared to the native concat() method .
Example 2: In this example, we can see that when we tried to insert an element inside the array, the original array is also altered which we didn’t intend and is not recommended. We can make use of the spread operator in this case,
Example 3: In this example we are doing same as above code but with spread operator, so that original array does not get altered.
By using the spread operator we made sure that the original array is not affected whenever we alter the new array.
Expand: Whenever we want to expand an array into another we do something like this:
Example 4: In this example, we want to expand an array into another
Even though we get the content on one array inside the other one, actually it is an array inside another array which is definitely what we didn’t want. If we want the content to be inside a single array we can make use of the spread operator.
Example 5: In this example, we want the content to be inside a single array we can make use of the spread operator.
Math: The Math object in javascript has different properties that we can make use of to do what we want like finding the minimum from a list of numbers, finding the maximum, etc. Consider the case that we want to find the minimum from a list of numbers, we will write something like this:
Example 6: In this example, we want to find the minimum from a list of numbers
Now consider that we have an array instead of a list, this above Math object method won’t work and will return NaN, like:
Example 7: In this example, Math object method won’t work and will return NaN
When …arr is used in the function call, it “expands” an iterable object arr into the list of arguments In order to avoid this NaN output, we make use of a spread operator, like:
Example 8: In this example, we make use of a spread operator In order to avoid this NaN
Example of spread operator with objects: ES6 has added spread property to object literals in javascript. The spread operator ( … ) with objects is used to create copies of existing objects with new or updated values or to make a copy of an object with more properties. Let’s take an example of how to use the spread operator on an object,
Example 9: In this example, we are showing how to use the spread operator on an object
Here we are spreading the user1 object. All key-value pairs of the user1 object are copied into the clonedUser object. Let’s look at another example of merging two objects using the spread operator,
Example 10: In this example, we are merging two objects using the spread operator
The mergedUsers is a copy of user1 and user2 . Actually, every enumerable property on the objects will be copied to the mergedUsers object. The spread operator is just a shorthand for the Object.assign() method but, there are some differences between the two.
We have a complete list of Javascript Operators, to check those please go through the Javascript Operators Complete Reference article.
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples .
Please Login to comment...
Similar reads.
- javascript-operators
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Currently Reading :
Currently reading:
How does spread operator work
Javascript spread operator.

Harsh Pandey
Software Developer
Published on Wed Apr 17 2024
The JavaScript spread operator ( ... ) works by expanding elements of an iterable (like an array) into individual elements. This operator is highly effective when applied to array literals or function calls where multiple parameters are expected.
Consider the usage of the spread operator in concatenating arrays. For instance, combining two arrays arr1 and arr2 can be succinctly done as follows:
The output of this operation would be:
Additionally, the spread operator is indispensable in copying arrays. To create a shallow copy of arr1 :
In function calls, the spread operator allows an array to be expanded into individual arguments. For example:
Calling the function with the spread operator:
Thus, the spread operator simplifies the process of expanding elements, combining arrays, and passing multiple parameters to functions, enhancing readability and efficiency in JavaScript coding.
Why the spread operator should be used
The spread operator should be used for its efficiency in expanding elements of an iterable (such as an array) into individual elements where zero or more arguments (for function calls) or elements (for array literals) are expected. It simplifies the process of combining arrays or objects, as seen in the following example:
This operator not only works with arrays but also with objects, which is ideal for creating copies of existing objects with new or updated values:
Using the spread operator leads to cleaner code, reduces the possibility of errors, and enhances readability by avoiding more verbose methods like concat () for arrays or Object. as sign () for objects. Additionally, it handles sparse arrays correctly, filling empty slots in arrays with undefined values, ensuring consistent outputs and predictable behavior.
Cloning Arrays/Objects
The JavaScript spread operator ( ... ) allows for the efficient cloning of arrays and objects. This operator expands the elements of an array or the properties of an object, making it ideal for creating a shallow copy quickly.
For cloning an array, you can simply use the spread operator within a new array declaration to copy all elements of the original array:
Similarly, to clone an object, the spread operator can be used to copy the properties into a new object:
Using the spread operator in this way ensures that the new array or object has the same contents as the original but is a separate instance. This method is particularly useful for avoiding unintended side-effects that can occur when objects or arrays are copied by reference rather than by value.
Converting Array-like structures to Array
Converting array-like structures to arrays is straightforward using the JavaScript Spread Operator. The spread operator, denoted by ... , efficiently transforms array-like objects (e.g., NodeList, arguments object) into true arrays. This conversion allows for the full use of array methods, such as map () , filter () , and reduce () that might not be available on the array-like object.
For example, consider a function that receives an unknown number of arguments and needs to process these arguments as an array:
Calling sumAll ( 1 , 2 , 3 ) would execute the following steps:
- Convert arguments to a true array using the spread operator.
- Use the reduce () method to calculate the sum.
This method ensures that functions can operate on parameters as if they were dealing with standard arrays, thereby expanding their utility and flexibility.
The spread operator as an argument
The spread operator as an argument allows elements of an array to be expanded in places where multiple elements can be passed.
When used in function calls, the spread operator ( ... ) efficiently passes the contents of an array as separate arguments to the function. Consider a function designed to find the maximum element in a set of numbers. Traditionally, you would call this function using individual arguments. With the spread operator, you can pass an array directly, and the operator will expand it into individual numbers.
For example:
The spread operator simplifies the syntax and improves readability when working with arrays in function arguments, making code cleaner and more intuitive. This is especially useful in cases involving array manipulations like concatenation, copying, and combining elements into new arrays.
Adding elements to Arrays/Objects
The JavaScript Spread Operator ( ... ) simplifies the process of adding elements to arrays or objects by unpacking and copying their entries. In arrays, it enables the seamless integration of new elements or the merging of multiple arrays. For instance:
Similarly, the Spread Operator can add properties to objects or combine objects by spreading properties from one object to another:
This operator is especially powerful for its concise syntax and the ability to shallow-copy the properties or elements, thereby preserving the immutability of the data structures involved. The Spread Operator effectively spreads the elements of an array or the properties of an object into a new array or object, making it an indispensable tool for modern JavaScript development.
Merging Arrays/Objects
The JavaScript spread operator ( ... ) efficiently merges arrays and objects by unpacking their elements or properties. When merging arrays, the spread operator can concatenate multiple arrays into one single array. Here's how you can merge two arrays:
Similarly, the spread operator can combine multiple objects into a single object by spreading each object's properties. If the same property exists in multiple objects, the property value from the last object will overwrite the earlier ones. Here is an example of merging two objects:
In both cases, the spread operator simplifies the process of combining data, maintaining a readable and concise code structure.
In conclusion, the JavaScript spread operator ( ... ) serves as a versatile and efficient tool for expanding elements of an iterable, such as arrays, in places where multiple elements or arguments are expected. It simplifies the process of concatenating arrays, copying arrays without reference issues, and applying elements to functions as arguments. The spread operator not only enhances the readability of the code by reducing complexity but also improves code maintenance and safety by avoiding the direct manipulation of the original data structures. Its ability to unpack and repack elements dynamically makes it indispensable for modern JavaScript development.

About the author
Software Developer adept in crafting efficient code and solving complex problems. Passionate about technology and continuous learning.
Browse Flexiple's talent pool
Explore our network of top tech talent. Find the perfect match for your project.
- Programmers
- React Native
- Ruby on Rails

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Spread operator in function calls JavaScript
The spread syntax allows us to spread an array or any iterable to be expanded across zero or more arguments in a function call.
Following is the code for spread operator in function calls in JavaScript −
Live Demo

On clicking the ‘CLICK HERE’ button −

Related Articles
- Spread operator for arrays in JavaScript
- Usage of rest parameter and spread operator in JavaScript?
- How to clone an array using spread operator in JavaScript?
- How to clone an object using spread operator in JavaScript?
- How to find maximum value in an array using spread operator in JavaScript?
- How to use spread operator to join two or more arrays in JavaScript?
- Using JSON.stringify() to display spread operator result?
- Rest and Spread operators in JavaScript
- How to pass JavaScript Variables with AJAX calls?
- Instanceof operator in JavaScript
- Explain function of % operator in Python.
- How to use Spread Syntax with arguments in JavaScript functions?
- The new operator in JavaScript
- What is function of ^ operator in Python
- What is increment (++) operator in JavaScript?
Kickstart Your Career
Get certified by completing the course
To Continue Learning Please Login
- How it works
- Homework answers
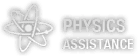
Answer to Question #170002 in HTML/JavaScript Web Application for manikanta
Unite Family
Given three objects
father, mother, and child, write a JS program to concatenate all the objects into the family object using the spread operator. Input
- The first line of input contains an object father
- The second line of input contains an object mother
- The third line of input contains an object child
- The output should be a single line string with the appropriate statement as shown in sample outputs
Constraints
- Keys of the objects should be given in quotes
Sample Input
{'surname' : 'Jones', 'city': 'Los Angeles'}
{'dish': 'puddings'}
{'pet': 'Peter'}
Sample Output
Mr and Mrs Jones went to a picnic in Los Angeles with a boy and a pet Peter. Mrs Jones made a special dish "puddings"
i want code in between write your code here
"use strict";
process.stdin.resume();
process.stdin.setEncoding("utf-8");
let inputString = "";
let currentLine = 0;
process.stdin.on("data", (inputStdin) => {
inputString += inputStdin;
process.stdin.on("end", (_) => {
inputString = inputString.trim().split("\n").map((str) => str.trim());
function readLine() {
return inputString[currentLine++];
/* Please do not modify anything above this line */
function main() {
const father = JSON.parse(readLine().replace(/'/g, '"'));
const mother = JSON.parse(readLine().replace(/'/g, '"'));
const child = JSON.parse(readLine().replace(/'/g, '"'));
/* Write your code here */
/* Please do not modify anything below this line */
console.log(`Mr and Mrs ${family.surname} went to a picnic in ${family.city} with a boy and a pet ${family.pet}. Mrs ${family.surname} made a special dish "${family.dish}"`);
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Update Pickup PointGiven a previous pickup point in the prefilled code, and updated pickup point are
- 2. Hotel BillA Hotel is offering discounts to its customers.Given charge per day dayCharge based on cat
- 3. "use strict";process.stdin.resume();process.stdin.setEncoding("utf-8");let input
- 4. String Starts or Ends with given StringGiven an arraystringsArray of strings, and startString, endSt
- 5. String SlicingGiven two strings inputString and subString as inputs, write a JS program to slice the
- 6. Speed Typing TestIn this assignment, let's build a Speed Typing Test by applying the concepts w
- 7. Book SearchIn this assignment, let's build a Book Search page by applying the concepts we learn
- Programming
- Engineering
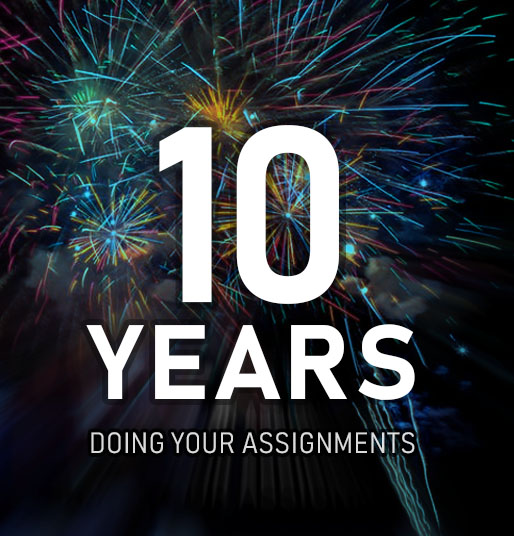
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
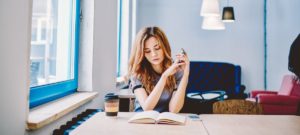
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
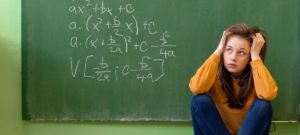
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Spread Operator in JavaScript
ZakiMohammed/javascript-spread-operator
Folders and files, repository files navigation, spread love with javascript.
A feature to resolve the array and object's cloning, inserting, and merging problems.
Link: https://codeomelet.com/posts/spread-love-with-javascript
- JavaScript 100.0%
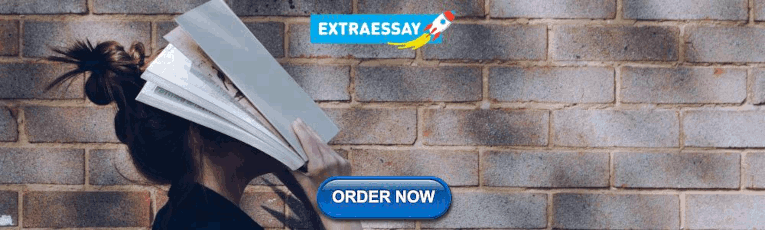
IMAGES
VIDEO
COMMENTS
The spread operator, …, was first introduced in ES6. It quickly became one of the most popular features. ... This is the second and final part of the Understanding the JavaScript Spread Operator — From Beginner to Expert tutorial. ... method creates a new array with the results of calling a provided function on every element in the calling ...
The spread (...) syntax allows an iterable, such as an array or string, to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. In an object literal, the spread syntax enumerates the properties of an object and adds the key-value pairs to the object being created. Spread syntax looks exactly like rest syntax.
The spread syntax is used to pass an array to functions that normally require a list of many arguments. Together they help to travel between a list and an array of parameters with ease. All arguments of a function call are also available in "old-style" arguments: array-like iterable object.
Let's look at one last example. function sample(a, b) {. return [a + b, a * b] } let example = sample(2, 5); console.log(example) Function to Add and Multiply two numbers. We have a function here which accepts two numbers. It returns an array adding them and multiplying them and logs them into the console.
The default assignment for object destructuring creates new variables with the same name as the object property. If you do not want the new variable to have the same name as the property name, you also have the option of renaming the new variable by using a colon (:) to decide a new name, as seen with noteId in the following:// Assign a custom name to a destructured value const {id: noteId ...
The spread operator '…' was first introduced in ES6. It quickly became one of the most popular features. So much so, that despite the fact that it only worked on Arrays, a proposal was made to extend its functionalities to Objects. This feature was finally introduced in ES9. The goal of this tutorial, which is divided into two parts, is to ...
The JavaScript spread operator ... is used to expand or spread out elements of an iterable, such as an array, string, or object. This makes it incredibly useful for tasks like combining arrays, passing elements to functions as separate arguments, or even copying arrays.
This operator can be used to carry out many routine tasks easily. The spread operator can be used to do the following: Copying an array. Concatenating or combining arrays. Using Math functions. Using an array as arguments. Adding an item to a list. Combining objects. Let's look at an example of each of these use cases.
the spread syntax as a function parameter / argument seems designed to 'spread' an array of values as separate arguments: Based on the research I've done, the spread syntax can be used to pass arrays to functions that will not accept arrays as arguments as standard, e.g. Array.prototype.push (), without having to use apply: var x = [];
JavaScript is a dynamic and potent language used by developers around the world to enhance web applications with complex, interactive, and rich features.. One such advanced feature in JavaScript's arsenal is the spread operator.In this article, we'll dive deep into what the spread operator is, its syntax, and how you can use it effectively in various programming scenarios.
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected. ... Example: In ES5 it is not possible to compose new with apply (in ES5 terms apply does a [[Call]] and not a [[Construct]]). In ES6 the spread syntax naturally supports this ...
Benefits of Using the Spread Operator in Function Calls. The spread operator brings several benefits to JavaScript programming, especially when used in function calls. 1. **Simplicity and Readability:** The spread operator simplifies the syntax for passing an array of arguments to a function.
The JavaScript spread operator (...) expands an iterable (like an array) into more elements. This allows us to quickly copy all or parts of an existing array into another array: Example. Assign the first and second items from numbers to variables and put the rest in an array:
This is the second and final part of the Understanding the JavaScript Spread Operator - From Beginner to Expert tutorial. Here's a link to the first part. In this second part of the tutorial we have learnt some more advanced uses of the spread operator, which include destructuring nested elements, adding conditional properties and adding ...
The Spread operator allows an iterable to expand in places where 0+ arguments are expected. It is mostly used in the variable array where there is more than 1 value is expected. It allows us the privilege to obtain a list of parameters from an array. The syntax of the Spread operator is the same as the Rest parameter but it works opposite of it ...
The JavaScript spread operator (...) allows an iterable such as an array or string to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. It is highly effective for concatenating arrays, copying arrays without referencing the original, and spreading elements of an iterable into ...
Spread operator in function calls JavaScript. Javascript Web Development Object Oriented Programming. The spread syntax allows us to spread an array or any iterable to be expanded across zero or more arguments in a function call. Following is the code for spread operator in function calls in JavaScript −.
Question #170002. Unite Family. Given three objects. father, mother, and child, write a JS program to concatenate all the objects into the family object using the spread operator. Input. The first line of input contains an object father. The second line of input contains an object mother. The third line of input contains an object child.
Thus, it's syntax to allow a variable number of arguments to a function, not an operator. Destructuring Assignment. Spread syntax can also be used during array destructuring assignment and is actually referred to as a rest element in the language specification (because when using in destructuring, it gets the rest of the destructured iterable ...
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior.