
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- This Chapter
- 1. Introduction' data-toggle="tooltip" >
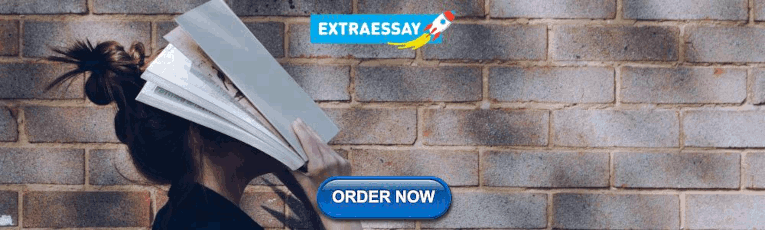
Problem Solving with Algorithms and Data Structures using C++ ¶
By Brad Miller and David Ranum, Luther College, and Jan Pearce, Berea College
- 1.1. Objectives
- 1.2. Getting Started
- 1.3. What Is Computer Science?
- 1.4. What Is Programming?
- 1.5. Why Study Data Structures and Abstract Data Types?
- 1.6. Why Study Algorithms?
- 1.7. Reviewing Basic C++
- 1.8. Getting Started with Data
- 1.9.1. Numeric Data
- 1.9.2. Boolean Data
- 1.9.3. Character Data
- 1.9.4. Pointers
- 1.10.1. Arrays
- 1.10.2. Vectors
- 1.10.3. Strings
- 1.10.4. Hash Tables
- 1.10.5. Unordered Sets
- 1.11.1. Parameter Passing: by Value versus by Reference
- 1.11.2. Arrays as Parameters in Functions
- 1.11.3. Function Overloading
- 1.12.1. A Fraction Class
- 1.12.2. Abstraction and Encapsulation
- 1.12.3. Polymorphism
- 1.12.4. Self Check
- 1.13.1. Logic Gates and Circuits
- 1.13.2. Building Circuits
- 1.14.1. Introduction to Turtles
- 1.14.2. Turtle & TurtleScreen
- 1.14.3. Geometry, Shapes, and Stamps
- 1.14.4. Advanced Features
- 1.15. Summary
- 1.16. Discussion Questions
- 1.17. Programming Exercises
- 1.18. Glossary
- 1.19. Matching
- 2.1. Objectives
- 2.2.1. Some Needed Math Notation
- 2.2.2. Applying the Math Notation
- 2.3. Big-O Notation
- 2.4.1. Solution 1: Checking Off
- 2.4.2. Solution 2: Sort and Compare
- 2.4.3. Solution 3: Brute Force
- 2.4.4. Solution 4: Count and Compare
- 2.5. Performance of C++ Data Collections
- 2.6. Analysis of Array and Vector Operators
- 2.7. Analysis of String Operators
- 2.8. Analysis of Hash Tables
- 2.9. Summary
- 2.10. Self Check
- 2.11. Discussion Questions
- 2.12. Programming Exercises
- 2.13. Glossary
- 2.14. Matching
- 3.1. Objectives
- 3.2. What Are Linear Structures?
- 3.3. What is a Stack?
- 3.4. The Stack Abstract Data Type
- 3.5. Using a Stack in C++
- 3.6. Simple Balanced Parentheses
- 3.7. Balanced Symbols - A General Case
- 3.8. Converting Decimal Numbers to Binary Numbers
- 3.9.1. Conversion of Infix Expressions to Prefix and Postfix
- 3.9.2. General Infix-to-Postfix Conversion
- 3.9.3. Postfix Evaluation
- 3.10. What Is a Queue?
- 3.11. The Queue Abstract Data Type
- 3.12. Using a Queue in C++
- 3.13. Simulation: Hot Potato
- 3.14.1. Main Simulation Steps
- 3.14.2. C++ Implementation
- 3.14.3. Discussion
- 3.15. What Is a Deque?
- 3.16. The Deque Abstract Data Type
- 3.17. Using a Deque in C++
- 3.18. Palindrome-Checker
- 3.19. Summary
- 3.20. Discussion Questions
- 3.21. Programming Exercises
- 3.22. Glossary
- 3.23. Matching
- 4.1. Objectives
- 4.2. What Are Linked Structures?
- 4.3. Implementing an Unordered Linked List
- 4.4. The Node Class
- 4.5. The Unordered Linked List Class
- 4.6.1. Analysis of Linked Lists
- 4.7.1. Forward lists
- 4.7.2. Lists
- 4.8. Summary
- 4.9. Discussion Questions
- 4.10. Programming Exercises
- 4.11. Glossary
- 4.12. Matching
- 5.1. Objectives
- 5.2. What Is Recursion?
- 5.3. Calculating the Sum of a Vector of Numbers
- 5.4. The Three Laws of Recursion
- 5.5. Converting an Integer to a String in Any Base
- 5.6. Stack Frames: Implementing Recursion
- 5.7. Introduction: Visualizing Recursion
- 5.8. Sierpinski Triangle
- 5.9. Complex Recursive Problems
- 5.10. Tower of Hanoi
- 5.11. Exploring a Maze
- 5.12. Dynamic Programming
- 5.13. Summary
- 5.14. Self-check
- 5.15. Discussion Questions
- 5.16. Programming Exercises
- 5.17. Glossary
- 5.18. Matching
- 6.1. Objectives
- 6.2. Searching
- 6.3.1. Analysis of Sequential Search
- 6.4.1. Analysis of Binary Search
- 6.5.1. Hash Functions
- 6.5.2. Collision Resolution
- 6.5.3. Implementing the Map Abstract Data Type
- 6.5.4. Analysis of Hashing
- 6.6. Self Check
- 6.7. Summary
- 6.8. Discussion Questions
- 6.9. Programming Exercises
- 6.10. Glossary
- 6.11. Matching
- 7.1. Objectives
- 7.2. Sorting
- 7.3. The Bubble Sort
- 7.4. The Selection Sort
- 7.5. The Insertion Sort
- 7.6. The Shell Sort
- 7.7. The Merge Sort
- 7.8. The Quick Sort
- 7.9. Self Check
- 7.10. Summary
- 7.11. Discussion Questions
- 7.12. Programming Exercises
- 7.13. Glossary
- 7.14. Matching
- 8.1. Objectives
- 8.2. Examples of Trees
- 8.3. Vocabulary and Definitions
- 8.4. Nodes and References
- 8.5. Parse Tree
- 8.6. Tree Traversals
- 8.7. Priority Queues with Binary Heaps
- 8.8. Priority Queues with Binary Heaps Example
- 8.9. Binary Heap Operations
- 8.10.1. The Structure Property
- 8.10.2. The Heap Order Property
- 8.10.3. Heap Operations
- 8.11. Binary Search Trees
- 8.12. Search Tree Operations
- 8.13. Search Tree Implementation
- 8.14. Search Tree Analysis
- 8.15. Balanced Binary Search Trees
- 8.16. AVL Tree Performance
- 8.17. AVL Tree Implementation
- 8.18. Summary of Map ADT Implementations
- 8.19. Summary
- 8.20. Discussion Questions
- 8.21. Programming Exercises
- 8.22. Glossary
- 8.23. Matching
- 9.1. Objectives
- 9.2. Vocabulary and Definitions
- 9.3. The Graph Abstract Data Type
- 9.4. An Adjacency Matrix
- 9.5. An Adjacency List
- 9.6. Implementation
- 9.7. The Word Ladder Problem
- 9.8. Building the Word Ladder Graph
- 9.9. Implementing Breadth First Search
- 9.10. Breadth First Search Analysis
- 9.11. The Knight’s Tour Problem
- 9.12. Building the Knight’s Tour Graph
- 9.13. Implementing Knight’s Tour
- 9.14. Knight’s Tour Analysis
- 9.15. General Depth First Search
- 9.16. Depth First Search Analysis
- 9.17. Topological Sorting
- 9.18. Strongly Connected Components
- 9.19. Shortest Path Problems
- 9.20. Dijkstra’s Algorithm
- 9.21. Analysis of Dijkstra’s Algorithm
- 9.22. Prim’s Spanning Tree Algorithm
- 9.23. Summary
- 9.24. Discussion Questions
- 9.25. Programming Exercises
- 9.26. Glossary
- 9.27. Matching
Acknowledgements ¶
We are very grateful to Franklin Beedle Publishers for allowing us to make the original Python version of this interactive textbook freely available. The original online version was dedicated to the memory of our first editor, Jim Leisy, who wanted us to “change the world.”
Indices and tables ¶
Module Index
Search Page

Learn Coding USA
Data structures in c: an example-based guide.
Last Updated on October 15, 2023
Introduction
Data structures play a crucial role in programming, enabling efficient organization and manipulation of data.
This blog section explores the importance and relevance of data structures, providing an overview of the post contents.
Importance and Relevance of Data Structures in Programming
Data structures are fundamental tools for managing data effectively and optimizing program performance.
They allow us to store and access data efficiently, enabling speedy operations and reducing time complexity.
Data structures also facilitate the implementation of algorithms, making it easier to solve complex problems efficiently.
Moreover, data structures enhance the modularity and maintainability of code, making it easier to understand and debug.
Overview of the Blog Post Contents
This blog post will delve into various data structures using C programming language as examples.
We will explore the concepts and implementations of popular data structures like arrays, linked lists, stacks, queues, trees, and graphs.
Each data structure will be explained in detail, including their working principles, advantages, and potential use cases.
Furthermore, we will provide code snippets and practical examples for better understanding and hands-on practice.
By the end of this post, readers will have a solid understanding of different data structures, their applications, and how to effectively use them in C programming.
Therefore, data structures serve as the backbone of programming, enabling efficient data organization and manipulation.
This blog post will provide a comprehensive overview of various data structures using C as a reference point and will equip readers with the necessary knowledge to employ them effectively.
Overview of Data Structures
Data structures are essential components in programming that allow efficient organization and manipulation of data.
They are designed to store, manage, and retrieve data in a structured and organized manner.
Different types of data structures (arrays, linked lists, stacks, queues, etc.)
There are various types of data structures, including arrays, linked lists, stacks, queues, trees, and graphs.
Each type has its unique characteristics, advantages, and use cases.
Arrays are a collection of elements of the same type, stored in contiguous memory locations.
Linked lists consist of nodes, each containing data and a reference to the next node in the sequence.
Stacks follow the LIFO (Last In, First Out) principle, where the item added last is the first one to be removed.
Queues use the FIFO (First In, First Out) principle, where the element added first is the first one to be removed.
Trees are hierarchical data structures with a root node and child nodes, forming a tree-like structure.
Graphs are networks formed by nodes (vertices) connected by edges, allowing for complex relationships between data.
The purpose of data structures is to provide efficient and organized ways to store and access data.
They optimize memory utilization, improve data retrieval and manipulation speed, and enhance code readability and maintainability.
By selecting the appropriate data structure, developers can optimize the performance of their programs.
For example, arrays are suitable when storing a fixed number of items with quick access to any element.
Linked lists are useful for dynamic data that can grow or shrink, as they allow efficient insertion and deletion operations.
Stacks are often used for implementing function calls and managing program execution flow.
Queues are suitable for managing tasks in a first-come, first-served manner, like job scheduling.
Trees excel in hierarchical data representation, such as file systems, organization hierarchies, and decision-making processes.
Graphs are valuable for modeling relationships between entities like social networks, recommendations, and internet connections.
Using data structures in programming brings several benefits
- Improved Efficiency: Data structures enable efficient storage and retrieval of data, leading to better algorithmic performance.
- Flexibility: Different data structures provide various ways to organize and manipulate data, catering to diverse programming needs.
- Code Reusability: Data structures are reusable components that can be applied to various programming problems.
- Scalability: Data structures allow programs to handle increasing amounts of data efficiently without major design changes.
- Simplified Maintenance: Well-designed data structures enhance code readability, understandability, and maintainability.
- Problem Solving: Familiarity with different data structures equips developers with a versatile toolkit to solve complex programming problems.
Most importantly, data structures form a crucial aspect of programming, providing efficient ways to store and manipulate data.
By understanding the purpose and characteristics of different data structures, developers can optimize their code and build efficient and scalable software.
Read: The Unsung Heroes: Pioneers of the Early Coding Wars Era
Getting Started with Data Structures in C
This secton provides a step-by-step guide to getting started with data structures in the C programming language.
We will cover the basics of C programming, setting up the development environment, and introduce the necessary tools for writing C code.
Brief Introduction to C Programming Language
C is a powerful high-level programming language that is widely used for system software development.
It provides low-level access to memory, making it suitable for implementing efficient data structures.
Before diving into data structures, it is essential to have a basic understanding of C syntax, variables, control flow, and functions.
Familiarize yourself with concepts such as loops, conditionals, and pointers, as they form the foundation of C programming.
Gathering C Programming Environment (Compiler, IDE)
To write and compile C code, you need a C compiler and an integrated development environment (IDE).
There are several options available, including:
- GNU GCC Compiler: This open-source compiler is widely used and available for various operating systems.
- IDEs: Choose from IDEs like Code::Blocks, Eclipse, or Microsoft Visual Studio, which provide a complete development environment with features like code completion and debugging tools.
Depending on your preferences and operating system, choose the compiler and IDE that suits you best.
Install and set them up on your machine to start writing C code.
Setting Up the Development Environment
Once you have selected a compiler and IDE, follow these steps to set up your development environment:
- Install the compiler: Download the compiler for your operating system and follow the installation instructions.
- Install the IDE: Download and install your preferred IDE, ensuring compatibility with the chosen compiler.
- Configure the IDE: Set the compiler path in the IDE’s settings to enable seamless compilation of C code.
- Create a new project: Begin by creating a new project in your IDE and select the C language option.
- Write your first C program: Start with a simple “Hello, World!” program to verify that your environment is set up correctly.
- Compile and run: Use the IDE’s build and run commands to compile and execute your program.
Congratulations! You now have a fully functional C development environment ready to explore data structures.
In essence, this section provided an overview of the essentials needed to begin understanding data structures in the C programming language.
We discussed the importance of familiarizing yourself with C syntax, selecting a compiler and IDE, and setting up the development environment.
With a solid foundation in place, you are now ready to dive deeper into the world of data structures in C.
Read: Mastering Algorithms: Key to Winning the Coding Wars
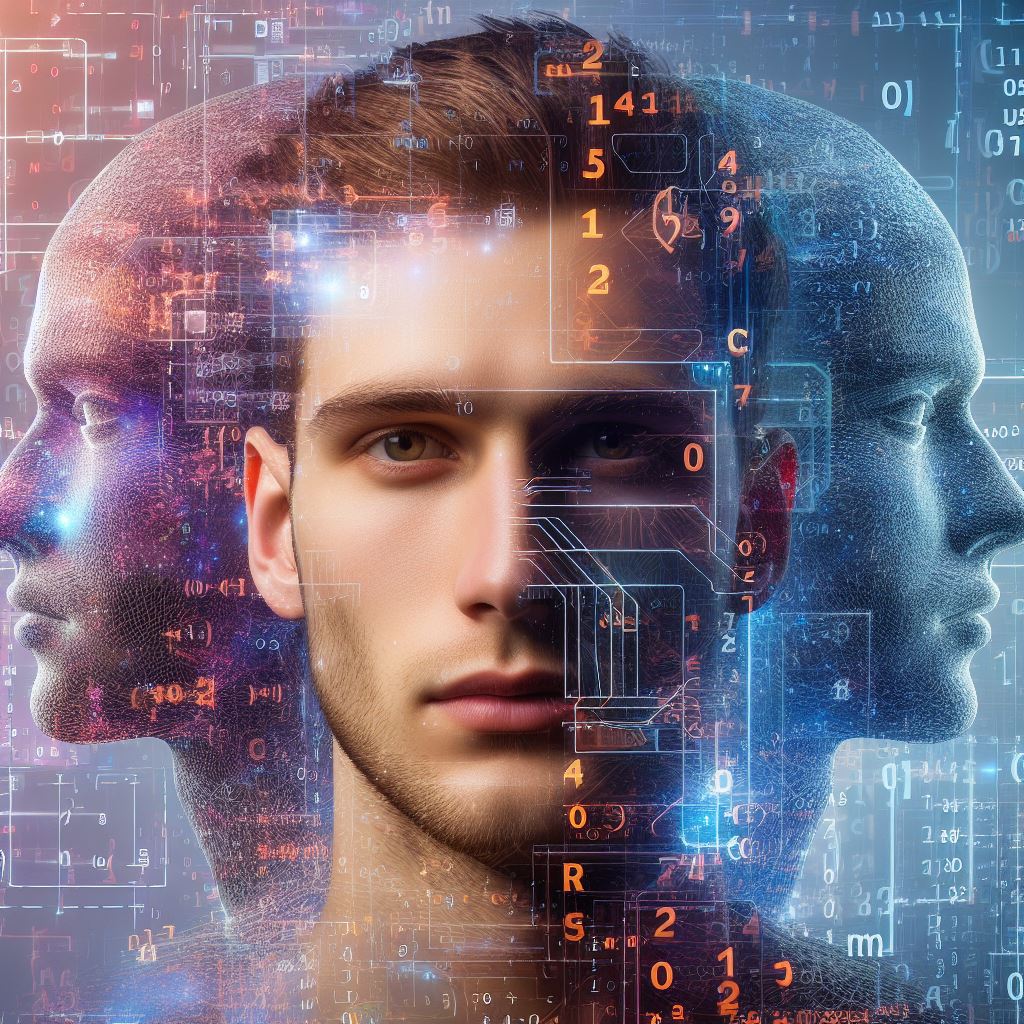
Arrays in C
In C programming, widely use arrays for their efficiency and versatility, declaring and initializing one-dimensional arrays with specific syntax.
Accessing and modifying array elements is done using their corresponding indices.
To access an element, we use the following syntax:
For example, to access the third element in the “numbers” array, we use:
Similarly, we can modify the value of an element using the assignment operator:
Arrays in C are mutable, meaning their elements can be modified after declaration and initialization.
Basic operations on arrays (accessing elements, modifying values)
Basic operations on arrays involve accessing elements and modifying their values.
These operations are extremely useful in various programming scenarios.
Arrays store data of any type, including integers or characters, with the ability to store strings using an array of characters.
Arrays make it easier to perform repetitive tasks such as sorting and searching.
By leveraging the power of loops, we can iterate through array elements and perform operations efficiently.
Examples of array applications
Here are a few examples of array applications:
- Finding the maximum or minimum value in an array: We can iterate through the array, comparing each element with a variable storing the maximum or minimum value so far.
- Calculating the sum or average of array elements: We can use a loop to iterate through the array, adding each element to a variable that accumulates the total.
- Reversing the order of array elements: By swapping elements from the ends of the array towards the center, we can reverse the order of array elements.
- Counting the occurrence of a specific value in an array: We can traverse the array and increment a counter variable whenever we encounter the target value.
In fact, arrays are an essential tool in C programming.
They provide a convenient and efficient way to store and manipulate data.
Mastering arrays will greatly enhance your ability to solve complex programming problems.
Read: Choosing the Right Coding Language at an Academy
Linked Lists in C
In this section, we will delve into the fascinating world of linked lists in C.
Linked lists are a fundamental data structure that offer a plethora of advantages over other data structures such as arrays.
We will explore these advantages and understand why linked lists are widely used in C programming.
A linked list is a collection of nodes, where each node contains both data and a reference to the next node in the sequence.
This “link” between nodes is what gives linked lists their name and differentiates them from arrays.
The absence of a fixed size makes linked lists more flexible and dynamic.
Creating and manipulating linked lists in C is relatively straightforward.
First, we need to define a struct that represents a node.
This struct will have two members: the data and a pointer to the next node.
With this struct, we can create as many nodes as needed, connecting them using their next pointers.
Traversing a linked list involves visiting each node in sequential order.
To do this, we start from the head node and follow the next pointers until we reach the end of the list.
This allows us to read or modify the data in each node.
Traversal is a common operation in linked lists and is essential for various algorithms that work with this data structure.
Searching for a specific element in a linked list is another crucial operation.
Similar to traversal, we start from the head node and compare the data in each node with the target element.
If a match is found, we can perform the desired action.
If the end of the list is reached without finding a match, we can conclude that the element is not present.
Insertion and deletion operations in linked lists
Insertion and deletion operations are fundamental for dynamically manipulating linked lists.
To insert a new node, we need to modify the next pointers of the existing nodes.
This ensures that the new node is correctly linked into the list while preserving the order.
Deletion involves updating the next pointers to bypass the node we want to remove from the list.
Linked lists offer several advantages over arrays.
One major advantage is their dynamic nature, as linked lists can grow or shrink as needed.
Additionally, linked lists can be easily modified without the need for large-scale data copying.
This makes linked lists suitable for scenarios where the size of the data is unknown or changes frequently.
In short, linked lists are a powerful data structure in C programming .
They provide flexibility, dynamic resizing, and efficient insertions and deletions.
Understanding linked lists and their advantages is crucial for any programmer aspiring to build robust and efficient applications.
By mastering the creation, manipulation, traversal, searching, insertion, and deletion operations, you can harness the full potential of linked lists in your C programs.
Read: The Real Cost: Is a Coding Academy Worth the Price?
Stacks and Queues in C
Introduction to stacks and queues.
Computer science utilizes stacks and queues as fundamental data structures for storing and managing data efficiently.
Implementation of Stacks and Queues using Arrays and Linked Lists
Implementing stacks and queues in C involves using either arrays or linked lists.
Stack Implementation
Implementing a stack in C involves following the “Last In First Out” (LIFO) principle, removing the last-inserted element first.
Using Arrays
Using arrays for stack implementation involves tracking the top element and updating it as elements are pushed or popped.
Linked Lists
Implementing stacks with linked lists involves adding elements at the beginning and removing them from the beginning as needed.
Queue Implementation
For queue implementation, apply the “First In First Out” (FIFO) principle, removing the first-inserted element first.
Arrays can be used to implement queues by using two pointers: one for the front and one for the rear of the queue.
Linked lists can also be used to implement queues by adding new elements at the rear and removing elements from the front.
Push, Pop, Enqueue, and Dequeue Operations
Stacks and queues support different operations to manipulate the data they contain.
Push Operation
In stacks, the push operation adds an element at the top of the stack.
Pop Operation
In stacks, the pop operation removes the top element from the stack.
Enqueue Operation
In queues, the enqueue operation adds an element at the rear of the queue.
Dequeue Operation
In queues, the dequeue operation removes the element from the front of the queue.
Applications and Use Cases of Stacks and Queues
Stacks and queues have various applications in real-world scenarios and computer science.
Stack Applications
Stacks are used in function calls, expression evaluation, undo/redo mechanisms, and backtracking algorithms.
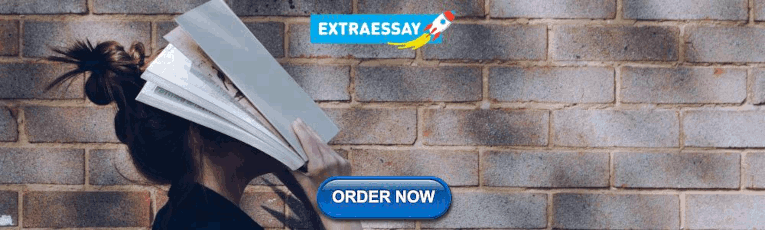
Queue Applications
Queues are used in scheduling algorithms, breadth-first search, printing and file processing, and message queues.
Basically, understanding stacks and queues is essential for building efficient and optimized algorithms in C programming.
Whether using arrays or linked lists, implementing these data structures allows for effective data management and problem-solving in various applications.
Trees are hierarchical data structures widely used to model relationships between elements.
They resemble actual trees, with a single root node and branches extending downwards.
Each node can have zero or more children nodes, forming a parent-child relationship.
Binary trees, a specific type of trees, have at most two children nodes per parent.
They can be classified as complete, full, or perfect based on the strictness of this property.
Complete binary trees have all levels completely filled, except perhaps the last, which is filled from left to right.
Full binary trees have every node either having two children or being a leaf node.
Perfect binary trees are both complete and full.
Traversing and searching binary trees in C
Traversing binary trees involves visiting every node in a specific order.
Pre-order traversal, visit the root first, then explore the left and right sub-trees recursively.
DIn in-order traversal, visit the root between the left and right sub-trees.
In post-order traversal, visit the root after the left and right sub-trees.
You can search binary trees using depth-first search (DFS) or breadth-first search (BFS) algorithms.
DFS starts at the root and explores as far as possible along each branch before backtracking.
BFS explores all the vertices of a tree in breadth-first manner, that is, it visits all the nodes at the same level before going deeper.
Insertion and deletion operations on binary trees
Insertion and deletion operations on binary trees are important for maintaining the structure.
To insert a new node into a binary tree, we need to find the appropriate position based on the node’s value.
If the position is empty, we can insert the node there.
If not, we compare the value of the node to be inserted with the current node and move left or right accordingly until we find an empty position.
To delete a node from a binary tree, we need to handle three cases: the node has no children, the node has only one child, or the node has two children.
In the first case, we can simply remove the node and update its parent’s reference to null.
In the second case, we replace the node with its child.
The third case, we find the node’s in-order successor or predecessor (the node with the next largest or smallest value) and replace the node with it.
We then delete the successor or predecessor from its original position.
Trees are powerful data structures that provide efficient ways to organize and manipulate hierarchical data.
Binary trees, in particular, are widely used and offer various traversal, searching, insertion, and deletion operations.
Understanding these concepts is essential for writing efficient C programs that deal with structured data.
The covered data structures in C include arrays, linked lists, stacks, queues, trees, and graphs.
Understanding and implementing these data structures is essential for efficient and organized programming.
By mastering data structures in C, programmers can optimize their code and solve complex problems more effectively.
It is highly encouraged to continue exploring and practicing data structures in C programming.
By doing so, programmers can enhance their problem-solving skills and become more versatile in their coding abilities.
Overall, data structures play a fundamental role in programming, and mastering them is crucial for success in the field.
- The Science Behind Music and Coding Productivity
- Elevate Coding Sessions with Binaural Beats: A Guide
You May Also Like
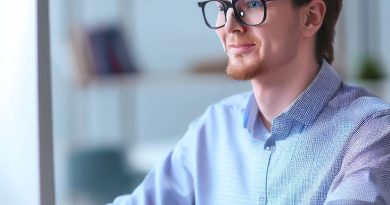
Remote Coding Jobs: Are They Worth the Salary Cut?
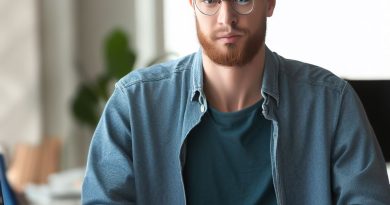
Kotlin for Android: Google’s Preferred Language
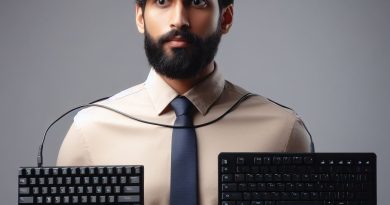
A Comparison: Wired vs Wireless Keyboards for Coding
Leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Data Science
- Courses Get 90% Refund!
Master C Programming with Data Structures
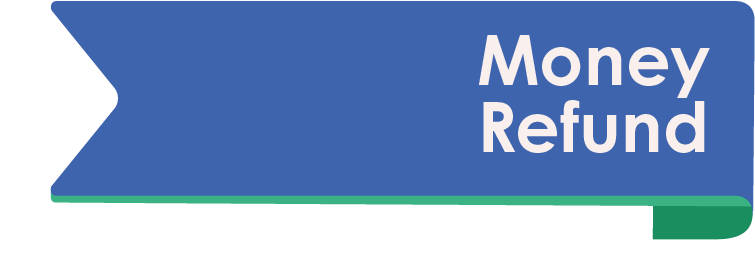
Course Description
Learn C Programming Language from the best mentor of all time! This C programming course will help you master all important concepts from basic to advanced level. Master the easy to learn C Language and take your skills to the next level. Start Today!

Three 90 Challenge is Back and It's Bigger & Better than Ever!! Complete 90% Course within 90 Days & Get 90% Refund!
Course Overview
- A Beginner to Advanced C Programming course with Data Structures
- Developed by Founder and CEO Mr. Sandeep Jain.
- Includes 15+ hours of Basic C Concepts
- And 20+ hours of Advanced C Concepts.
- Practice with 150+ coding problems and 200+ MCQs .
- Access curated notes for quick revisions.
- Participate in self-assessment contests .
- Get 24/7 doubt assistance
- Focus on data types, control structures, functions, and arrays.
- Learn pointers, structures, and file handling.
- Explore data structures like linked lists , stacks, queues, trees , etc
- Prepare for placements with coding problems.
Three 90 Challenge
We were listening, and we heard you loud and clear. The Three 90 challenge is back and this is your chance to get 90% of your fee back in just 90 days!
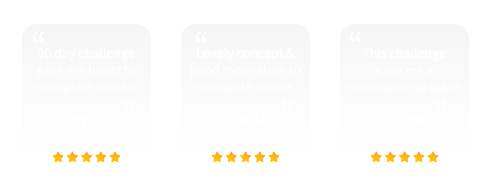
What Sets Us Apart
24 X 7 Doubt Support
Recognised Certification
Expert Mentors
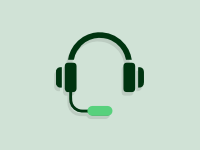
A dedicated service provided with this course for free to help you overcome any doubt, at any time, and anywhere. So unleash your coding potential with confidence, as our Doubt Support service stands by your side!
Benefits of this service:
- Access to Expert TAs - Prompt Response - Tailored Guidance - 1:1 Video & On-Call Support & Much More
Now code with confidence, triumph over doubts, and level up your skills!
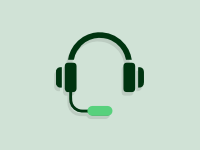
Course Content
Know about the background introduction, C introduction, How do C Programs Run, Comments in C, etc
Learn about the variables in C & Naming Rules, Data Types in C, Range of Data Types, Const in C, Type Conversion C and much more
Get your minds on to learn Inputs & Outputs in C, Buffering, Escape Sequence, IO Manipulation, Floating Point Default Print Format, etc
Build your knowledge on Operators like, Arithmetic, Comparison, Logical, Assingnment, Bitwise, Arithmetic Progression, Geometric Progression, etc
Frequently Asked Questions
Will i get 90% of my course fee back, is there any number to contact for query, do we have doubt support in this program, how can i register for the course, what type of certificate will be offered in this program, when can i make the payment for the course, can i make the payment through paypal, is there any demo lecture video of this course, what features does doubt support have, how long will the course content be available for.
Data Structures
Arrays - ds easy problem solving (basic) max score: 10 success rate: 93.21%, 2d array - ds easy problem solving (basic) max score: 15 success rate: 93.15%, dynamic array easy problem solving (basic) max score: 15 success rate: 86.82%, left rotation easy problem solving (basic) max score: 20 success rate: 91.29%, sparse arrays medium problem solving (basic) max score: 25 success rate: 97.29%, array manipulation hard problem solving (intermediate) max score: 60 success rate: 61.29%, print the elements of a linked list easy problem solving (basic) max score: 5 success rate: 97.16%, insert a node at the tail of a linked list easy problem solving (intermediate) max score: 5 success rate: 95.26%, insert a node at the head of a linked list easy problem solving (basic) max score: 5 success rate: 98.32%, insert a node at a specific position in a linked list easy problem solving (intermediate) max score: 5 success rate: 96.97%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Hemant-Jain-Author/Problem-Solving-in-Data-Structures-and-Algorithms-using-C
Folders and files, repository files navigation, problem-solving-in-data-structures-and-algorithms-using-c.
This is the code repository of book "Problem Solving in Data Structures & Algorithms Using C".
About The Book
- This textbook provides in depth coverage of various Data Structures and Algorithms.
- Concepts are discussed in easy to understand manner.
- Large number of diagrams are provided to grasp concepts easily.
- Time and Space complexities of various algorithms are discussed.
- Helpful for interviews preparation and competitive coding.
- Large number of interview questions are solved.
- C solutions are provided with input and output.
- Guide you through how to solve new problems in programming interview of various software companies.
Table of Contents
- Chapter 0: How to use this book.
- Chapter 1: Algorithms Analysis
- Chapter 2: Approach to solve algorithm design problems
- Chapter 3: Abstract Data Type
- Chapter 4: Searching
- Chapter 5: Sorting
- Chapter 6: Linked List
- Chapter 7: Stack
- Chapter 8: Queue
- Chapter 9: Tree
- Chapter 10: Priority Queue
- Chapter 11: Hash-Table
- Chapter 12: Graphs
- Chapter 13: String Algorithms
- Chapter 14: Algorithm Design Techniques
- Chapter 15: Brute Force Algorithm
- Chapter 16: Greedy Algorithm
- Chapter 17: Divide & Conquer
- Chapter 18: Dynamic Programming
- Chapter 19: Backtracking
- Chapter 20: Complexity Theory
- Python 1.7%
Academia.edu no longer supports Internet Explorer.
To browse Academia.edu and the wider internet faster and more securely, please take a few seconds to upgrade your browser .
Enter the email address you signed up with and we'll email you a reset link.
- We're Hiring!
- Help Center
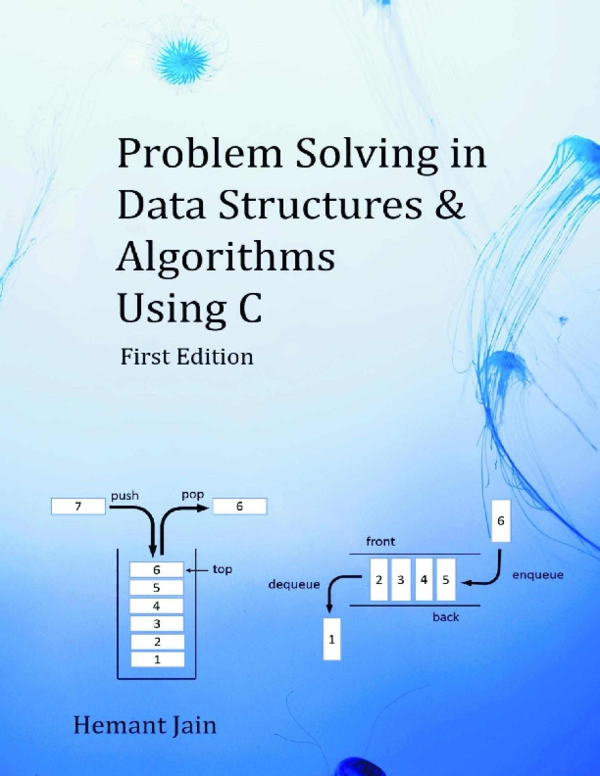
Problem Solving in Data Structures & Algorithms Using C First Edition

Related Papers
A problem that is constantly cropping up in designing even the simplest algorithm or a program is dealing with +-1 bug when we calculate positions within an array, very noticeably while splitting it in half. This bug is often found in buffer overflow type of bugs. While designing one complicated algorithm, we needed various ways of splitting an array, and we found lack of general guidance for this apparently minor problem. We present an exercise that tracks the cause of the problem and leads to the solution. This problem looks trivial because it seems obvious or insignificant, however treating it without outmost precision can lead to subtle bugs, unbalanced solution, not transparent expressions for various languages. Basically, the exercise is about dealing with <= < as well as n/2, n/2-1, (n+1)/2, n-1 and similar expressions when they are rounded down to the nearest integer and used to define a range.

Zhishan Guo
AliKarim Sayed
Ranjeet Singh
Afido Was Here
RELATED PAPERS
Muhamad Nuri Huda
Journal of Diabetes Science and Technology
Rasmus Elsborg
Études internationales
Carlos Eduardo Lavarda
Acción Pedagógica
Luz Marina Pinedo Montoña
Journal of neural engineering
prakhar agarwal
Mario Santrum , Moses Tokan
Ethnography and Education
joanna Empain
Lecture Notes in Computer Science
Y. Aloimonos
Boletin Cientifico Centro De Museos Museo De Historia Natural
Leonardo Perez
Colloids and Surfaces A: Physicochemical and Engineering Aspects
Nina Mirchin
ICASSP 2019 - 2019 IEEE International Conference on Acoustics, Speech and Signal Processing (ICASSP)
Mauricio Lisboa Perez
International Journal for Research in Applied Science & Engineering Technology (IJRASET)
IJRASET Publication
Paolo Casella
Cristina Sánchez-Cruzado
Religion, State and Society
Bayram Balci
Journal of pathology informatics
Stephen J McKenna
sapto irawan
Jurnal Pendidikan dan Pembelajaran Khatulistiwa
Agung Hartoyo
Biomaterials
Maxwell Koobatian
Lecture notes in civil engineering
Journal Widya Medika Junior
Felicia Hartono
Future Science OA
Kavita Beri
See More Documents Like This
- We're Hiring!
- Help Center
- Find new research papers in:
- Health Sciences
- Earth Sciences
- Cognitive Science
- Mathematics
- Computer Science
- Academia ©2024
Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
Data Structures and Algorithms Problems
- TopClassic, TopLiked ↗ Easy
- TopLiked ↗ Medium
- TopLiked ↗ Easy
- TopClassic, TopLiked ↗ Medium
- ↗ Medium
- ↗ Hard
- ↗ Easy
- TopAlgo ↗ Easy
- TopClassic ↗ Medium
- TopAlgo, TopClassic, TopAlgo ↗ Easy
- TopLiked ↗ Hard
- TopClassic, TopLiked ↗ Hard
- TopClassic ↗ Hard
- TopClassic ↗ Easy
- TopAlgo ↗ Medium
- TopClassic Hard
- ↗ Beginner
- TopAlgo ↗ Hard
- TopLiked Medium
- TopClassic, TopLiked, TopDP ↗ Medium
- TopLiked, TopDP ↗ Hard
- TopClassic, TopLiked, TopDP ↗ Hard
- TopDP ↗ Medium
- TopAlgo Medium
- TopClassic Medium
- TopAlgo Hard
Rate this post
Average rating 4.88 /5. Vote count: 5909
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Uh-oh, it looks like your Internet Explorer is out of date. For a better shopping experience, please upgrade now.
Javascript is not enabled in your browser. Enabling JavaScript in your browser will allow you to experience all the features of our site. Learn how to enable JavaScript on your browser
Data Structures and Problem Solving Using C++ / Edition 2 available in Paperback

Data Structures and Problem Solving Using C++ / Edition 2
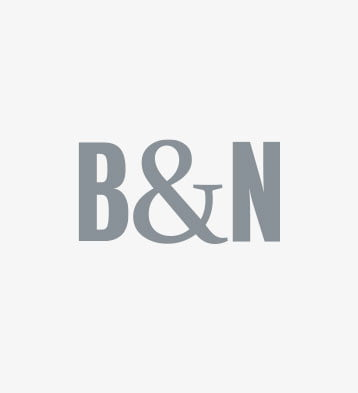
- SHIP THIS ITEM — This Item is Not Available
Available within 2 business hours
This Item is Not Available
Temporarily out of stock online.
Please check back later for updated availability.
- Condition: Used-Good Details

Data Structures and Problem Solving Using C++ provides a practical introduction to data structures and algorithms from the viewpoint of abstract thinking and problem solving, as well as the use of C++. It is a complete revision of Weissi successful CS2 book Algorithms, Data Structures, and Problem Solving with C++.
The most unique aspect of this text is the clear separation of the interface and implementation. C++ allows the programmer to write the interface and implementation separately, to place them in separate files and compile separately, and to hide the implementation details. This book goes a step further: the interface and implementation are discussed in separate parts of the book. Part I (Objects and C++), Part II (Algorithms and Building Blocks), and Part III (Applications) lay the groundwork by discussing basic concepts and tools and providing some practical examples, but implementation of data structures is not shown until Part IV (Implementations). This separation of interface and implementation promotes abstract thinking. Class interfaces are written and used before the implementation is known, forcing the reader to think about the functionality and potential efficiency of the various data structures (e.g., hash tables are written well before the hash table is implemented).
Throughout the book, Weiss has included the latest features of the C++ programming language, including a more prevalent use of the Standard Template Library (STL).
- Promotes abstract thinking by separating the interface and implementation of the data structures into different parts of the book
- All code is completely rewritten and tested for compatibility with a wide range of current compilers
- Revised material makes use of the STL whenever appropriate
- Rewritten material on inheritance simplifies the initial presentation and provides the C++ details that are important for advanced uses
- Includes a new chapter on Patterns
- Provides new material on templates, vectors, and push_back
- Illustrates both the generic interfaces and STL interfaces of data structures
- Generic data structures such as linked lists classes, search tree and hash table classes, priority_queue, and disjoint sets class are rewritten to be much simpler and cleaner
- A simplified STL implementation is illustrated at the end of the chapters in Part IV, including vector, list, stack, queue, set, map, and priority_queue
Product Details
About the author, table of contents.
Mark Allen Weiss is a Professor in the School of Computer Science at Florida International University. He received his Ph.D. in Computer Science from Princeton University where he studied under Robert Sedgewick. Dr.Weiss has received FIU's Excellence in Research Award, as well as the Teaching Incentive Program Award, which was established by the Florida Legislature to recognize teaching excellence. Mark Allen Weiss is on the Advanced Placement Computer Science Development Committee. He is the successful author of Algorithms, Data Structures, and Problem Solving with C++ and the series Data Structures and Algorithm Analysis in Pascal, Ada, C, and C++, with Addison-Wesley.
I. OBJECTS AND C++.
II. ALGORITHMS AND BUILDING BLOCKS.
III. APPLICATIONS.
IV. IMPLEMENTATIONS.
V. ADVANCED DATA STRUCTURES.
Related Subjects
Customer reviews.
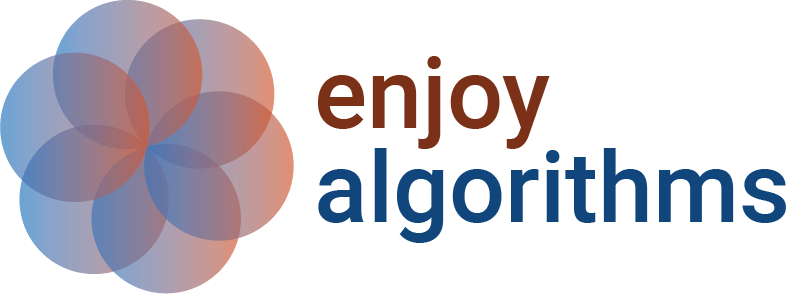
EnjoyMathematics
Steps of Problem Solving in Data Structures and Algorithms
Every solution starts with a strategy, and an algorithm is a strategy for solving a coding problem. So, we must learn to design an efficient algorithm and translate this 'algorithm' into the correct code to get the job done.
But there are many coding problems available in data structures and algorithms, and most of the time, these problems are new to us. So as programmers, we need to develop ourselves as confident problem-solvers who are not intimidated by the difficulty of the given problem.
Our long-term goal should be simple: Learn to design correct and efficient code within a given time. As we practice more and more, we will gain experience in problem-solving, and our work will become easier. Here are some essential skills that we should practice for every DSA problem:
- Developing an approach to understanding the problem
- Thinking of a correct basic solution
- Designing step-by-step pseudocode solutions
- Analyzing the efficiency of a solution
- Optimizing the solution further
- Transforming pseudocode into correct code
Now, the critical question would be: Is there a well-defined, guided strategy to approach and solve a coding problem? If yes, then what are the critical steps? Let's think and explore!
Step 1: Understanding the problem
Solving a problem requires a clear understanding of the problem. Unfortunately, sometimes we read only the first few lines and assume the rest of the problem or ignore this step because we have seen something similar in the past. We should view these as unfair practices and develop a clear approach to understanding problems.
During problem-solving, every small detail can help us design an efficient solution. Sometimes, a small change in the question can alter the solution approach. Taking extra time to understand the problem will give us more confidence later on. The fact is, we never want to realize halfway through that we misunderstood the problem.
It doesn't matter if we have encountered a question before or not; we should read the question several times. So, take a paper and write down everything while going through the problem. Exploring some examples will also help us clarify how many cases our algorithm can handle and the possible input-output patterns. We should also explore scenarios for large input, edge cases, and invalid input.
Sometimes, it is common for problem descriptions to suffer from these types of deficiencies:
- The problem description may rely on undefined assumptions
- The problem description may be ambiguous or incomplete
- The problem description may have various contradictions.
These deficiencies may be due to the abstract explanation of the problem description in our natural languages. So, it is our responsibility to identify such deficiencies and work with the interviewer or problem provider to clarify them. We should start by seeking answers to the following questions:
- What are the inputs and outputs?
- What type of data is available?
- What is the size or scale of the input?
- How is the data stored? What is the data structure?
- Are there any special conditions or orders in the data?
- What rules exist for working with the data?
Step 2: Thinking of a correct basic solution
The best approach would be to think of a correct solution that comes immediately to our mind. It does not matter even if it is an inefficient approach. Having a correct and inefficient answer is much better than an incorrect solution or significant delay in finding the solution. This could help us in so many ways:
- Help us to build good confidence or motivation at the start.
- Provide an excellent point to start a conversation with the interviewer.
- Sometimes, it provides a hint to improve efficiency by reducing some loops, removing some intermediate steps, or performing some operations efficiently.
Here are some examples of brute force patterns: three nested loops, two nested loops, solution using extra memory, solution using sorting, double traversal in the binary tree, considering all sub-arrays or substrings, exhaustive search, etc.
After thinking and communicating the brute force idea, the interviewer may ask for its time and space complexity. We need to work on paper, analyze each critical operation, and write it in the form of Big-O notation. Clear conceptual idea of time and space complexity analysis is essential at this stage.
Step 3: Designing efficient solution with pseudocode
This is a stage to use the best experience of DSA problem-solving and apply various problem-solving strategies . One practical truth is: moving from a basic algorithm to the most efficient algorithm is a little difficult in a single step. Each time, we need to optimize the previous algorithm and stop when there is no further optimization possible. Revisiting the problem description and looking for some additional information can help a lot in further optimization. For example:
- If the input array is sorted or nearly sorted, we can apply optimized algorithms such as a single loop, two-pointer approach, or binary search.
- If we need to find a subarray of size k, we can use the sliding window technique, which involves maintaining a window of size k over the array and sliding it over the elements to find the desired subarray.
- When searching is a critical operation, we can use optimized search algorithms or data structures like binary search, BST, or hash table.
- For optimization problems, we can consider divide and conquer, dynamic programming, or greedy algorithm approaches.
- If we need to find a solution with a given constraint, we can use backtracking.
- When working with string data, direct address tables, hash tables, or trie data structures can be useful.
- To frequently access and process max or min elements, we can use a priority queue or heap data structure.
- For dictionary operations such as insert, search, and delete, we can use hash tables or BST.
- If we need to perform both dictionary and priority queue operations, a BST may be useful.
- For range query operations such as range max, range min, or range sum, we can use data structures like segment trees or Fenwick trees.
- To process binary tree data level by level, BFS or level-order traversal can be used.
The idea would be simple: we should learn the use case of efficient problem-solving patterns on various data structures. Continuously thinking, analyzing, and looking for a better solution is the core idea.
Here are some best examples of problems where several levels of optimisations are feasible. Practicing such types of coding questions helps a lot in building confidence.
Find equilibrium index of an array
- Using nested loops: Time = O(n²), Memory = O(1)
- Using prefix sum array: Time = O(n), Memory = O(n)
- Using single scan: Time = O(n), Memory = O(1)
Trapping rain water
- Using Dynamic Programming: Time = O(n), Memory = O(n)
- Using Stack: Time = O(n), Memory = O(n)
- Using two pointers: Time = O(n), Memory = O(1)
Check for pair with a given sum
- Using sorting and binary search: Time = O(nlogn), Memory = O(1)
- Using sorting and Two Pointers: Time = O(nlogn), Memory = O(1)
- Using a Hash Table: Time = O(n), Memory = O(n)
Find the majority element in an array
- Using two nested loops: Time = O(n²), Memory = O(1)
- Using Sorting: Time = O(nlogn), Memory = O(1)
- Using the divide and conquer: Time = O(nlogn), Memory = O(logn)
- Using Bit Manipulation: Time = O(n), Memory = O(1)
- Using Randomisation: Time = O(nlogn), Memory = O(1) Note: If value of n is very large.
- Boyer-Moore Voting Algorithm: Time = O(n), Memory = O(1)
Maximum Subarray Sum
- Using three nested loops: Time = O(n^3), Memory = O(1)
- Using two nested loops: Time = O(n^2), Memory = O(1)
- Using divide and conquer: Time = O(nlogn), Memory = O(logn)
- Using dynamic programming: Time = O(n), Memory = O(n)
- Kadane algorithm: Time = O(n), Memory = O(1)
Before you jump into the end-to-end code implementation, it’s good practice to write pseudocode on paper. It would be helpful in defining code structure and critical operations. Some programmers skip this step, but writing the final code becomes easier when we have well-designed pseudocode.
Step 4: Transforming pseudocode into a clean, correct, and optimized code
Finally, we need to replace each line of pseudocode with actual code in our favorite programming languages like C++, Java, Python, C#, JavaScript, etc. Never forget to test actual code with sample test data and check if the actual output is equal to the expected output. When writing code in your interviews, discuss sample data or test cases with the interviewer.
Simplifying and optimizing the code may require a few iterations of observation. We need to ask these questions once we are done writing the code:
- Does this code run for every possible input, including the edge cases?
- Can we optimize the code further? Can we remove some variables or loop or some extra space?
- Are we repeating some steps a lot? Can we define it separately using another function?
- Is the code readable or written with a good coding style?
Enjoy learning, Enjoy coding, Enjoy algorithms!
Share Your Insights
Don’t fill this out if you’re human:
More from EnjoyAlgorithms
Self-paced courses and blogs, coding interview, machine learning, system design, oop concepts, our newsletter.
Subscribe to get well designed content on data structure and algorithms, machine learning, system design, object orientd programming and math.
©2023 Code Algorithms Pvt. Ltd.
All rights reserved.
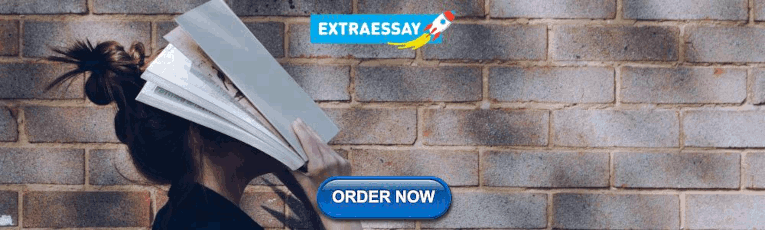
IMAGES
VIDEO
COMMENTS
An interactive version of Problem Solving with Algorithms and Data Structures using C++. ... Problem Solving with Algorithms and Data Structures using C++ by Bradley N. Miller, David L. Ranum, and Janice L. Pearce is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License.
This blog post will delve into various data structures using C programming language as examples. We will explore the concepts and implementations of popular data structures like arrays, linked lists, stacks, queues, trees, and graphs. ... Problem Solving: Familiarity with different data structures equips developers with a versatile toolkit to ...
Course Overview. Developed by Founder and CEO Mr. Sandeep Jain. And 20+ hours of Advanced C Concepts. Practice with 150+ coding problems and 200+ MCQs. Access curated notes for quick revisions. Participate in self-assessment contests. Focus on data types, control structures, functions, and arrays. Learn pointers, structures, and file handling ...
Data Structures. Data Structures. Arrays - DS. Easy Problem Solving (Basic) Max Score: 10 Success Rate: 93.21%. Solve Challenge. 2D Array - DS. ... Hard Problem Solving (Intermediate) Max Score: 60 Success Rate: 61.28%. Solve Challenge. Print the Elements of a Linked List.
Data Structures and Problem Solving Using C++ (2nd Edition) by Mark Allen Weiss. The book review and TOC. Algorithms and Data Structures: with implementations in Java and C++: Data structures Algorithms C++ Books Forum Feedback. Support us to write more tutorials to create new visualizers to keep sharing
Problem Solving in Data Structures & Algorithms Using C $29.00 In Stock "Problem Solving in Data Structures & Algorithms" is a series of books about the usage of Data Structures and Algorithms in computer programming.
Data Structures and Problem Solving Using C++ provides a practical introduction to data structures and algorithms from the viewpoint of abstract thinking and problem solving, as well as the use of C++. It is a complete revision of Weiss' successful CS2 book Algorithms, Data Structures, and Problem Solving with C++. The most unique aspect of this text is the clear separation of the interface ...
Data Structures and Problem Solving Using Java (Fourth Edition) Data Structures and Problem Solving Using C++ (Second Edition) Published by Addison-Wesley, 2010; ISBN: -321-54140-5; CS-2 Text ; Errata (last update: 8/29/16) Source code. Requires a Java 5 or higher compiler. Source code from third edition. Source code from second edition.
Problem Solving with Algorithms and Data Structures, Release 3.0 Figure 1.1: Procedural Abstraction must know the details of how operating systems work, how network protocols are configured, and how to code various scripts that control function. They must be able to control the low-level details that a user simply assumes.
"Problem Solving in Data Structures & Algorithms" is a series of books about the usage of Data Structures and Algorithms in computer programming. The book is easy to follow and is written for interview preparation point of view. In these books, the examples are solved in various languages like Go, C, C++, Java, C#, Python, VB, JavaScript and PHP.
This 53 hours of course covers each topic in greater details, every topic is covered on Whiteboard which will improve your Problem Solving and Analytical Skills. Every Data Structure is discussed, analysed and implemented with a Practical line-by-line coding. Source code for all Programs is available for you to download.
This is the code repository of book "Problem Solving in Data Structures & Algorithms Using C". About The Book. This textbook provides in depth coverage of various Data Structures and Algorithms. Concepts are discussed in easy to understand manner. Large number of diagrams are provided to grasp concepts easily.
This book is about the usage of data structures and algorithms in computer programming. Designing an efficient algorithm to solve a computer science problem is a skill of Computer programmer. This is the skill which tech companies like Google, Amazon, Microsoft, Adobe and many others are looking for in an interview.
2018. "Problem Solving in Data Structures & Algorithms" is a series of books about the usage of Data Structures and Algorithms in computer programming. The book is easy to follow and is written for interview preparation point of view. In these books, the examples are solved in various languages like Go, C, C++, Java, C#, Python, VB, JavaScript ...
Build Essential Data Structures And Algorithms Skills. The Data Structures and Algorithms courses we offer are designed to help prepare you for a career in software engineering, system design, and computational problem-solving, equipping you with the foundational knowledge to efficiently organize, manipulate, and analyze data.
A problem that is constantly cropping up in designing even the simplest algorithm or a program is dealing with +-1 bug when we calculate positions within an array, very noticeably while splitting it in half.
Practice Course 173 Problems. Learn to break down a problem. Find the algorithm to solve. Addition, subtraction, division, multiplication. If - else Conditions. Modulo operator / Ceil & Floor functions. Finding the correct formula. Exponentiation. Arrays and Loops.
Data Structures and Algorithms Problems. 1. Find a pair with the given sum in an array ↗ Easy. 2. Check if a subarray with 0 sum exists or not ↗ Medium. 3. Print all subarrays with 0 sum ↗ Medium. 4. Sort binary array in linear time ↗ Easy.
in problem solving. L1, L2 CO 2 Understand the working of stack and queue data structures and apply recursion to solve problems like tower of Hanoi. L2, L3 CO 3 Implement appropriate sorting/searching technique for a given problem and discuss the computational efficiency. L2, L3 CO 4 Apply non-linear data structure graph to solve real world
Data Structures and Problem Solving Using C++ provides a practical introduction to data structures and algorithms from the viewpoint of abstract thinking and problem solving, as well as the use of C++. It is a complete revision of Weissi successful CS2 book Algorithms, Data Structures, and Problem Solving with C++. ...
Higher Intellect | Content Delivery Network
"Problem Solving in Data Structures & Algorithms" is a series of books about the usage of Data Structures and Algorithms in computer programming. The book is easy to follow and is written for interview preparation point of view. In various books, the examples are solved in various languages like C, C++, Java, C#, Python, VB, JavaScript and PHP.
Step 3: Designing efficient solution with pseudocode. This is a stage to use the best experience of DSA problem-solving and apply various problem-solving strategies. One practical truth is: moving from a basic algorithm to the most efficient algorithm is a little difficult in a single step. Each time, we need to optimize the previous algorithm ...