You are using an outdated browser. Upgrade your browser today or install Google Chrome Frame to better experience this site.
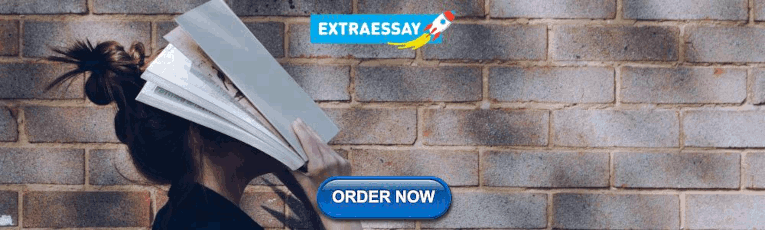
Learn X in Y minutes
Where x=prolog.
Get the code: learnprolog.pl
Prolog is a logic programming language first specified in 1972, and refined into multiple modern implementations.
Ready For More?
Got a suggestion? A correction, perhaps? Open an Issue on the GitHub Repo, or make a pull request yourself!
Originally contributed by hyphz, and updated by 4 contributors .
CSE 130 - Programming Assignment #2
(330 points + 70 points extra credit), must be turned in no later than 11:59:59 pm on 5/27 (see submission instructions below ).
(click your browser's refresh button to ensure that you have the most recent version)
Integrity of Scholarship
University rules on integrity of scholarship will be strictly enforced. By completing this assignment, you implicitly agree to abide by the UCSD Policy on Integrity of Scholarship described beginning on page 62 of the Academic Regulations section ( PDF ) of the 2002-2003 General Catalog, in particular, "all academic work will be done by the student to whom it is assigned, without unauthorized aid of any kind."
You are expected to do your own work on this assignment; there are no group projects in this course. You may (and are encouraged to) engage in general discussions with your classmates regarding the assignment, but specific details of a solution, including the solution itself, must always be your own work. Incidents which violate the University's rules on integrity of scholarship will be taken seriously.
In addition to receiving a zero (0) on the assignment, students may also face other penalties, up to and including, expulsion from the University. Should you have any doubt about the moral and/or ethical implications of an activity associated with the completion of this assignment, please see the instructors.
Code Documentation and General Requirements
Code for all programming assignments should be well documented . A working program with no comments will receive only partial credit . Documentation entails writing a description of each predicate as well as comments throughout the code to explain the program logic. Comments in Prolog begin with a percent sign (%) and are terminated by a newline/carriage return. It is understood that some of the exercises in this programming assignment require extremely little code and will not require extensive comments. Nevertheless, comments describing recursions and helper predicates are required.
While few programming assignments pretend to mimic the "real" world, they may, nevertheless, contain some of the ambiguity that exists outside the classroom. If, for example, an assignment is amenable to differing interpretations, such that more than one algorithm may implement a correct solution to the assignment, it is incumbent upon the programmer to document not only the functionality of the algorithm (and more broadly his/her interpretation of the program requirements), but to articulate clearly the reasoning behind a particular choice of solution.
Assignment Overview
The overall objective of this assignment is for you to gain some hands-on experience with problem solving using Prolog, using simple facts and rules, recursion, and database handling capabilities of the language.
The instructions for submission of your assignment may be found at the bottom of this page of this document. It is a good idea to start this assignment early; Prolog programming, while not inherently difficult, often seem somewhat foreign at first, particularly when it comes to recursion and list manipulation.
So as not to make the code overly long, it is not required that you deal with user errors: you can assume that the user always types valid commands (e.g., if a predicate is supposed to take an atom as argument, you do not have to check whether the argument is instead a list and throw an error). Note that the Prolog interpreter catches a good number of user errors anyway.
Problem #1 - Uncles and Half-Sisters (40 points)
parent(john,ann). parent(jim,john). parent(jim,keith). parent(mary,ann). parent(mary,sylvia). parent(brian,sylvia). male(keith). male(jim). female(sylvia). female(ann). male(brian).
- Question #1: Uncle (20 points)
Write a Prolog predicate uncle(X,Y) that is true if X is Y 's uncle. Note that we are not considering uncles "by marriage", meaning that for X to be Y 's uncle the two must be related by blood. For instance (user input is in red ):
?- uncle(keith,ann). Yes ?- uncle(ann,mary). No ?- uncle(keith,X). X = ann ; No ?- uncle(john,ann). No ?- uncle(X,Y). X = keith Y = ann ; No
- Question #2: Half-Sister (20 points)
Write a Prolog predicate halfsister(X,Y) that is true if X is Y 's half-sister. For instance (user input is in red ):
?- halfsister(ann,sylvia). Yes ?- halfsister(X,sylvia). X=ann ; No ?- halfsister(X,Y). X=ann X=sylvia ; X=sylvia X=ann ; No
Problem #2 - Lists (160 points)
- Question #1: Sublist (20 points)
Write a Prolog predicate sublist(X,Y) that is true if list X is a sublist of list Y . A sublist is defined as the original list, in the same order , but in which some elements may have been removed. For instance (user input is in red ):
?- sublist([a,b],[a,e,b,d,s,e]). Yes ?- sublist([a,b],[a,e,e,f]). No ?- sublist([a,b],[b,a]). No ?- sublist([],[a,e,e,f]). Yes ?- sublist([a],[]). No ?- sublist(X,[a,b,c]). X = [] ; X = [a] ; X = [a, b] ; X = [a, b, c] ; X = [a, c] ; X = [b] ; X = [b, c] ; X = [c] ; No
- Question #2: Detecting Duplicates (30 points)
Write a Prolog predicate has_duplicates(X) that is true if list X contains duplicated elements (that is at least 2 copies of an element). For instance (user input is in red ):
?- has_duplicates([a,e,b,d,s,e]). Yes ?- has_duplicates([a,b,d,s,e]). No ?- has_duplicates([]). No
- Question #3: Detecting Triplicates (40 points)
Write a Prolog predicate has_triplicate(X) that is true if list X contains triplicated elements (that is at least 3 copies of an element). For instance (user input is in red ):
?- has_triplicates([a,e,e,d,s,e]). Yes ?- has_triplicates([a,a,b,e,b,s,s,e]). No ?- has_triplicates([]). No
- Question #4: Remove the third element (10 points)
Write a Prolog predicate remove_third(X,Y) that is true if list Y is just list X with its third element removed. If X has no third element, then the predicate should fail. For instance (user input is in red ):
?- remove_third([a,e,e,d,s,e],L). L = [a,e,d,s,e] ; No ?- remove_third([a,b],L). No
- Question #5: Remove the nth element (30 points)
Write a Prolog predicate remove_nth(N,X,Y) that is true if list Y is just list X with its Nth element removed. If X does not have an Nth element then the predicate should fail. You can assume that N is strictly greater than 0. For instance (user input is in red ):
?- remove_nth(4,[a,e,e,d,s,e],L). L = [a,e,e,s,e] ; No ?- remove_nth(6,[a,b],L). No
- Question #6: Remove every other element (30 points)
Write a Prolog predicate remove_every_other(X,Y) that is true if list Y is just list X with every other element removed (the two lists should have the same first element). For instance (user input is in red ):
?- remove_every_other([a,e,e,d,s,e],L). L = [a,e,s] ; No ?- remove_every_other([a,e,e,d,s],L). L = [a,e,s] ; No ?- remove_every_other([],L). L = [] ; No
Problem #3 - A Prolog Database (130 points)
store(best_smoothies, [alan,john,mary], [ smoothie(berry, [orange, blueberry, strawberry], 2), smoothie(tropical, [orange, banana, mango, guava], 3), smoothie(blue, [banana, blueberry], 3) ]). store(all_smoothies, [keith,mary], [ smoothie(pinacolada, [orange, pineapple, coconut], 2), smoothie(green, [orange, banana, kiwi], 5), smoothie(purple, [orange, blueberry, strawberry], 2), smoothie(smooth, [orange, banana, mango],1) ]). store(smoothies_galore, [heath,john,michelle], [ smoothie(combo1, [strawberry, orange, banana], 2), smoothie(combo2, [banana, orange], 5), smoothie(combo3, [orange, peach, banana], 2), smoothie(combo4, [guava, mango, papaya, orange],1), smoothie(combo5, [grapefruit, banana, pear],1) ]).
The first store has three employees and sells three different smoothies, the second store has two employees and sells four different smoothies, and the third store has three employees and sells five different smoothies. You can assume that there are no duplicates ( pineapple is not listed twice in any ingredient list, mary is not listed twice in any employee list, the same smoothie specification is not listed twice in any store menu, etc.). Given a database of smoothie store facts, the questions below have you write predicates that implement queries to the database.
- Question #1: Sells More than Four Smoothies (10 points)
?- more_than_four(best_smoothies). No ?- more_than_four(X). X = all_smoothies ; X = smoothies_galore ; No
- Question #2: exists (20 points)
?- exists(combo1). Yes ?- exists(slimy). No ?- exists(X). X = berry ; X = tropical <enter> Yes
- Question #3: Employee to Smoothie Ratio (20 points)
?- ratio(all_smoothies,R). R = 0.5 ; No ?- ratio(Store,R). Store = best_smoothies R = 1 ; Store = all_smoothies R = 0.5 ; Store = smoothies_galore R = 0.6 ; No
- Question #4: Average Smoothie Price (25 points)
?- average(best_smoothies,A). A = 2.66667 ; No
- Question #5: Smoothies in Store (30 points)
?- smoothies_in_store(all_smoothies,L). L = [pinacolada, green, purple, smooth] ; No ?- smoothies_in_store(Store,L). Store = best_smoothies L = [berry, tropical, blue] ; Store = all_smoothies L = [pinacolada, green, purple, smooth] ; Store = smoothies_galore L = [combo1, combo2, combo3, combo4, combo5] ; No
- Question #6: Fruit in All Smoothies (25 points)
?- fruit_in_all_smoothies(Store,orange). Store = all_smoothies ; No
Problem #4 - A More Complex query (70 points) [EXTRA CREDIT]
?- find_smoothies(L,[banana,orange],[blueberry,mango]). L = [all_smoothies, green, smoothies_galore, combo1, smoothies_galore, combo2, smoothies_galore, combo3] ; No
The smoothie "green" sold in the "all_smoothies" store, and the smoothies "combo1" and "combo2" sold in the "smoothies_galore" store, are the only smoothies with both banana and orange and without any blueberries or mango, hence the result to the above query.
?- find_smoothies(L,[orange,banana],[mango,blueberry]). L = [all_smoothies, green, smoothies_galore, combo1, smoothies_galore, combo2, smoothies_galore, combo3] ; No
?- find_smoothies(L,[mango],[]). L = [best_smoothies, tropical, all_smoothies, smooth, smoothies_galore, combo4] ; No
?- find_smoothies(L,[],[banana]). L = [best_smoothies, berry, all_smoothies, pinacolada, all_smoothies, purple, smoothies_galore, combo4] ; No
Submission Guidelines/Instructions
Your functions/programs must compile and/or run on a UNIX ACS machine, as this is where the verification of your solutions will occur. While you may develop your code on any system, ensure that your code runs as expected on an ACS machine prior to submission. Code which does not compile and/or produce the desired results on the testing machine will receive little or no credit. You should test your code in the directories from which the tar file (see below) will be created, as this will approximate the environment used for grading the assignment.
Creating the tar file for submission
Your answers to this assignment will be stored in separate files under a directory called <username>_cse130_pa2/ . For instance, I would create a directory caller casanova_cse130_pa2 . The directory hierarchy should appear as follows: <username>_cse130_pa2/ problem1.prolog problem2.prolog problem3.prolog problem4.prolog For example, as indicated above, in the <username>_cse130_pa2 directory, you should have a file named problem1.prolog , which will contain the documented Prolog source code to implement the functions specified in Problem #1 of this assignment, a file named problem2.prolog , which will contain the documented Prolog source code to implement the functions specified in Problem #2, and so on. Assuming the directory hierarchy above, populated with the problem solutions as described, you should create a tar file called <username>_cse130_pa2.tar by running the tar command in the directory where the <username>_cse130_pa2 directory resides. If you don't know anything about tar , I strongly suggest you learn about it as it is very useful (the man page is rather lengthy, but you can find a more gentle introduction there ). For example, in the directory containing the above directory, I would run the command tar -cvf casanova_cse130_pa2.tar casanova_cse130_pa2
which would create the desired tar file (in this case, named casanova_cse130_pa2.tar ) from the directory named casanova_cse130_pa2 .
Submitting the program via the turnin program
Once you've created the tar file containing all of your answers to the assignment questions, you will use the turnin program to submit this file for grading. Using the turnin program is straightforward. Assuming I am in the directory where I've created the casanova_cse130_pa2.tar file, I would simply run the turnin command as follows: turnin -c cs130s -p pa2 casanova_cse130_pa2.tar
The turnin program will provide you with a confirmation of the submission process; make sure that the size of the file indicated by turnin matches the size of your tar file. See the ACS web page on turnin for more information on the operation of the program.
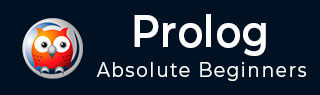
- Prolog Tutorial
- Prolog - Home
- Prolog - Introduction
- Prolog - Environment Setup
- Prolog - Hello World
- Prolog - Basics
- Prolog - Relations
- Prolog - Data Objects
Prolog - Operators
- Loop & Decision Making
- Conjunctions & Disjunctions
- Prolog - Lists
- Recursion and Structures
- Prolog - Backtracking
- Prolog - Different and Not
- Prolog - Inputs and Outputs
- Prolog - Built-In Predicates
- Tree Data Structure (Case Study)
- Prolog - Examples
- Prolog - Basic Programs
- Prolog - Examples of Cuts
- Towers of Hanoi Problem
- Prolog - Linked Lists
- Monkey and Banana Problem
- Prolog Useful Resources
- Prolog - Quick Guide
- Prolog - Useful Resources
- Prolog - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
In the following sections, we will see what are the different types of operators in Prolog. Types of the comparison operators and Arithmetic operators.
We will also see how these are different from any other high level language operators, how they are syntactically different, and how they are different in their work. Also we will see some practical demonstration to understand the usage of different operators.
Comparison Operators
Comparison operators are used to compare two equations or states. Following are different comparison operators −
You can see that the ‘=<’ operator, ‘=:=’ operator and ‘=\=’ operators are syntactically different from other languages. Let us see some practical demonstration to this.
Here we can see 1+2=:=2+1 is returning true, but 1+2=2+1 is returning false. This is because, in the first case it is checking whether the value of 1 + 2 is same as 2 + 1 or not, and the other one is checking whether two patterns ‘1+2’ and ‘2+1’ are same or not. As they are not same, it returns no (false). In the case of 1+A=B+2, A and B are two variables, and they are automatically assigned to some values that will match the pattern.
Arithmetic Operators in Prolog
Arithmetic operators are used to perform arithmetic operations. There are few different types of arithmetic operators as follows −
Let us see one practical code to understand the usage of these operators.
Note − The nl is used to create new line.
To Continue Learning Please Login
- Mastering Prolog: A Comprehensive Guide from Fundamentals to Advanced Techniques
From Theory to Practice: Excelling in Prolog University Assignments
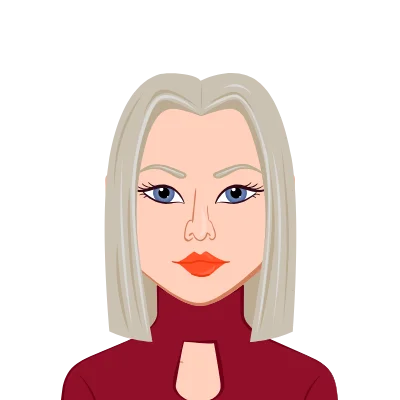
Prolog, a declarative programming language tailored for symbolic reasoning and manipulation, stands as a cornerstone in various university computer science curricula. While the mastery of Prolog's theoretical underpinnings is indispensable, achieving excellence in assignments necessitates a seamless transition from theory to hands-on application. In this comprehensive guide, we delve into essential strategies for navigating Prolog university assignments, placing a strong emphasis on fostering practical experience and insights. By exploring key concepts such as terms, atoms, variables, predicates, unification, and backtracking, this guide aims to equip students with a solid foundation in Prolog's fundamentals. Additionally, we will tackle real-world applications through case studies, shedding light on Prolog's versatility in artificial intelligence, natural language processing, and expert systems. To further aid students, practical tips on debugging techniques, time management, and the benefits of collaborative learning are discussed. This guide seeks to empower students to excel in Prolog assignment by providing a holistic approach that combines theoretical understanding with hands-on practice, fostering a deeper appreciation for the language's practical applications in the field.
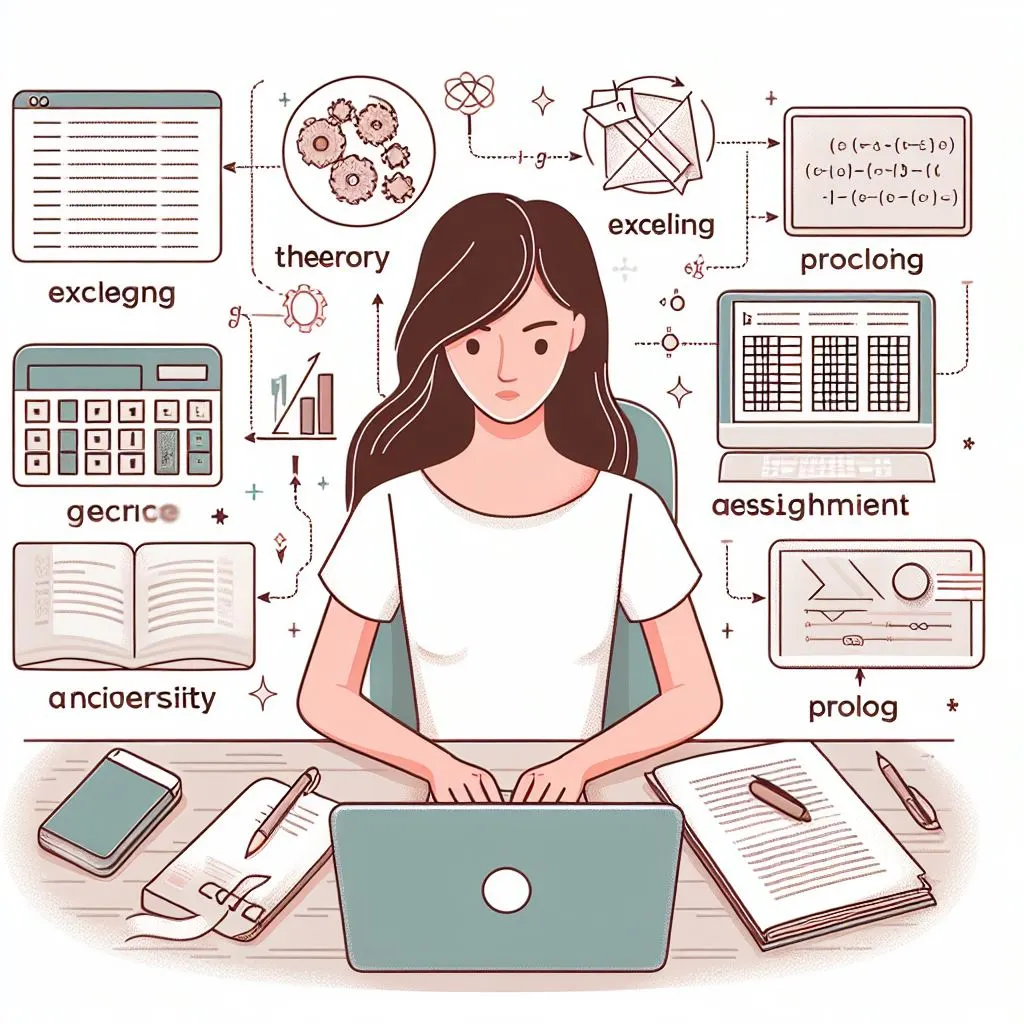
Understanding the Foundations of Prolog
Embarking on the journey of mastering Prolog necessitates a comprehensive understanding of its foundational principles. In this section, we delve into the core concepts that constitute the bedrock of Prolog programming. From unraveling the intricacies of terms, atoms, variables, to deciphering the significance of predicates, this exploration aims to provide students with a solid footing in Prolog's fundamental syntax and structure. As we unravel the nuances of unification and backtracking, learners will gain insights into Prolog's unique execution model, a crucial aspect in crafting efficient and effective solutions. By establishing a robust grasp of these foundational elements, students will be better equipped to tackle more complex aspects of Prolog, paving the way for a seamless transition from theory to practical application in their university assignments. This section serves as a gateway to the intricate world of Prolog programming, laying the groundwork for a holistic and insightful learning experience.
Prolog Fundamentals
Embark on your Prolog journey by immersing yourself in the fundamental concepts that lay the groundwork for advanced understanding. Delve into the intricacies of terms, atoms, variables, and predicates, unraveling the language's building blocks. Expand on the basic syntax and structure of Prolog programs, ensuring readers not only comprehend individual components but also grasp their interplay within the broader context of Prolog. By fostering a comprehensive understanding of these fundamentals, students are equipped with the essential knowledge needed to confidently approach more intricate aspects of Prolog programming, laying a robust foundation for their academic and practical endeavors.
Unification and Backtracking
Navigate the inner workings of Prolog's execution model through a profound exploration of unification and backtracking. Dissect these core concepts to unveil their pivotal role in problem-solving within the Prolog paradigm. Illustrate their significance with practical examples, guiding students through scenarios where Prolog dynamically searches for solutions and intelligently backtracks when faced with obstacles. By offering a nuanced perspective on these critical mechanisms, students gain not only theoretical insight but also practical prowess, enabling them to maneuver through complex problem spaces with confidence and finesse. This thorough exploration prepares students for the challenges presented in Prolog assignments, empowering them to approach problem-solving with a depth of understanding and strategic acumen.
Applying Prolog to Real-world Problems
Navigating the realm of Prolog extends beyond theoretical constructs, with its true power manifesting when applied to real-world challenges. In this segment, we explore the practical applications of Prolog through case studies, illuminating how the language can be leveraged in artificial intelligence, natural language processing, and expert systems. By showcasing Prolog's versatility in addressing tangible issues, students gain valuable insights into its relevance and potential impact across various domains. This section bridges the gap between academic learning and real-world problem-solving, encouraging learners to envision the broader applications of Prolog in industry scenarios. As we delve into the intricacies of employing Prolog in practical contexts, students are equipped with the knowledge and inspiration needed to approach their university assignments with a pragmatic mindset, ensuring they not only master the language in theory but also grasp its significance in addressing contemporary, real-world problems.
Case Studies in Prolog
Elevate your understanding of Prolog's practical applications by immersing yourself in a diverse array of case studies. Delve into real-world scenarios where Prolog emerges as a powerful problem-solving tool, particularly in artificial intelligence, natural language processing, and expert systems. Through detailed illustrations, showcase the versatility of Prolog across various domains, demonstrating its prowess in addressing complex challenges. By drawing inspiration from these case studies, students gain not only theoretical insights but also a profound appreciation for Prolog's real-world impact. This exploration serves as a bridge between classroom learning and practical application, encouraging students to approach their assignments with a heightened sense of purpose and a keen awareness of Prolog's relevance in solving contemporary, industry-level problems.
Industry Relevance
Uncover the profound relevance of Prolog in the dynamic landscape of the industry. Delve into how leading companies leverage Prolog for rule-based systems, constraint logic programming, and knowledge representation. Provide in-depth insights into the strategic advantages that Prolog affords in addressing complex challenges faced by organizations. Emphasize the symbiotic relationship between academic learning and industry needs, illustrating why a proficiency in Prolog can be a valuable asset in shaping future career prospects. By understanding the practical applications of Prolog within an industrial context, students not only enhance their academic performance but also position themselves strategically for a seamless transition into the professional realm, equipped with skills that align with contemporary industry demands.
Practical Tips for Excelling in Prolog Assignments
Mastering Prolog assignments goes beyond theoretical understanding, requiring a skillful blend of practical expertise and strategic approaches. This section delves into practical tips designed to enhance students' proficiency in Prolog programming. From effective debugging techniques that unravel the intricacies of code to time management strategies tailored for assignment completion, this segment offers valuable insights. Additionally, it advocates for collaborative learning, fostering an environment where students can share knowledge, troubleshoot challenges, and collectively elevate their Prolog programming skills. By providing actionable advice on navigating common pitfalls and optimizing time allocation, this section aims to empower students in their Prolog assignments, instilling a sense of confidence and efficiency as they bridge the gap between theoretical knowledge and real-world application.
Debugging Techniques
Navigate the intricate landscape of debugging Prolog code with a comprehensive exploration of advanced techniques. Beyond recognizing common challenges, delve into nuanced strategies for effective debugging, leveraging trace facilities and deciphering intricate error messages. Equip students with a deep understanding of how to troubleshoot not only typical issues but also complex scenarios, fostering a resilient problem-solving mindset. By providing insights into the intricacies of debugging, this section enhances the learning experience, empowering students to unravel the complexities of Prolog assignments with confidence and precision.
Time Management
Recognize the paramount role of time management in the realm of Prolog assignments and delve into advanced strategies for optimizing efficiency. Acknowledge the unique time constraints associated with university assignments, offering nuanced guidance on effective time allocation. Encourage students to adopt proactive time management practices, emphasizing the importance of breaking down assignments into manageable tasks and establishing a strategic workflow. By instilling a disciplined approach to time management, students not only enhance their productivity but also cultivate a skill set that extends beyond Prolog assignments, preparing them for the broader challenges of academic and professional life.
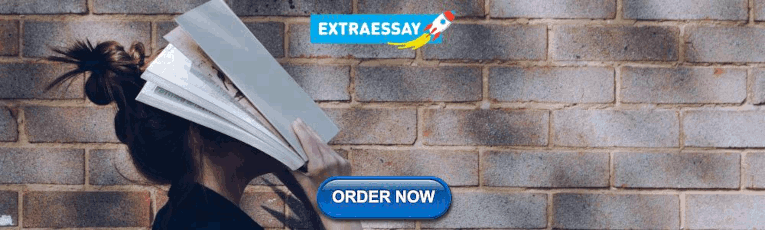
Collaborative Learning
Foster a culture of collaborative learning that transcends traditional boundaries. Encourage students to actively engage in dynamic group discussions and participate in peer reviews, emphasizing the synergistic benefits of shared insights, collaborative code snippets, and collective problem-solving approaches. Highlight the transformative power of a supportive learning community, where diverse perspectives converge to enhance understanding and elevate individual and collective proficiency in Prolog. By promoting collaborative learning, this section not only enriches the academic experience but also cultivates a collaborative mindset that proves invaluable in the multifaceted landscape of computer science and programming.
Advanced Prolog Concepts
Delving into the intricacies of Prolog programming unveils a realm of advanced concepts that propel students beyond the basics. In this section, we explore the sophisticated facets of Prolog, introducing learners to Definite Clause Grammars (DCGs) and the transformative power of meta-programming. These advanced concepts extend the capabilities of Prolog, allowing students to tackle complex challenges with heightened efficiency. By providing a comprehensive understanding of DCGs and meta-programming, this segment empowers students to elevate their Prolog proficiency, opening doors to more intricate problem-solving scenarios. As students venture into this advanced terrain, they gain a deeper appreciation for the expressive capabilities of Prolog and the strategic advantages offered by these advanced features. This section serves as a gateway for students to transcend conventional boundaries, encouraging them to harness the full potential of Prolog in their academic pursuits and future programming endeavors.
DCGs (Definite Clause Grammars)
Embark on an in-depth exploration of Definite Clause Grammars (DCGs) in Prolog, unraveling the intricacies of this advanced feature. Delve into the ways DCGs simplify language parsing and representational structures, providing students with a profound understanding of how these mechanisms streamline complex tasks. Elevate the learning experience by supplementing explanations with a rich array of examples and exercises, ensuring students not only grasp the theoretical foundations but also gain practical proficiency in employing DCGs. By immersing themselves in the world of DCGs, students can harness the power of this advanced Prolog feature, enhancing their ability to craft elegant and efficient solutions to complex language-based problems in both academic and real-world scenarios.
Meta-programming in Prolog
Embark on a transformative journey into the realm of meta-programming in Prolog, unveiling its potential to manipulate programs as data. Explore the intricate dynamics of how meta-programming augments Prolog's power and flexibility, offering students a unique perspective on program manipulation. Delve into practical examples, guiding students through the intricacies of leveraging meta-programming in their assignments. By embracing meta-programming, students not only expand their Prolog toolkit but also cultivate a mindset that embraces innovation and adaptability in the face of complex programming challenges. This section serves as a gateway for students to explore the dynamic and cutting-edge aspects of Prolog, empowering them to push the boundaries of traditional programming paradigms.
In conclusion, this guide underscores the crucial takeaways for students aiming to excel in Prolog university assignments. Emphasizing the need for a balanced approach, it advocates combining theoretical comprehension with practical application. By melding conceptual knowledge with hands-on practice, students can navigate Prolog assignments with greater confidence and efficacy. The guide encourages learners to view Prolog not just as an academic exercise but as a powerful tool with real-world applications. By embracing both theory and practice, students can unlock the full potential of Prolog, gaining a profound appreciation for its versatility in solving complex problems across diverse domains. As they embark on their Prolog journey, this balanced approach ensures that students not only meet the academic requirements of their assignments but also develop a skill set that extends beyond the classroom, preparing them for the challenges and opportunities that arise in the dynamic landscape of computer science and programming.
Post a comment...
Mastering prolog: a comprehensive guide from fundamentals to advanced techniques submit your homework, attached files.
- Data Analysis
- Data Visualization
- Machine Learning
- Deep Learning
- Computer Vision
- AI ML DS Interview Series
- AI ML DS Projects series
- Data Engineering
- Web Scrapping
Prolog | An Introduction
- AI Prolog Installation in Turbo C++
- Mathematics | Introduction to Proofs
- Introduction To Grammar in Theory of Computation
- Interactive Zero Knowledge Proof
- Logical Deduction Questions and Answers (2023)
- Boolean Parenthesization Problem | DP-37
- Resolution Theorem Proving
- Representation of Boolean Functions
- How to Install SWI Prolog on Windows?
- Turing machine for subtraction | Set 1
- Propositional Logic Reduction
- Infosys | Logical Reasoning 1 | Question 12
- Infosys | Logical Reasoning 1 | Question 11
- Infosys | Logical Reasoning 4 | Question 14
- Infosys | Logical Reasoning 1 | Question 5
- Circuit (Combinational and Sequential) Implementation using Prolog
- Logical | Reasoning 1 | Question 15
- Propositional Logic based Agent
- AMCAT | Logical Reasoning 2 | Question 5
- Differences between Procedural and Object Oriented Programming
- Structures in C++
- Introduction of Object Oriented Programming
- Decorators with parameters in Python
- Shallow Copy and Deep Copy in C++
- Difference between Shallow and Deep copy of a class
- C# | Data Types
- Features of C Programming Language
- Passing a Function as a Parameter in C++
- Clear the Console and the Environment in R Studio
Introduction :
Prolog is a logic programming language. It has important role in artificial intelligence. Unlike many other programming languages, Prolog is intended primarily as a declarative programming language. In prolog, logic is expressed as relations (called as Facts and Rules). Core heart of prolog lies at the logic being applied. Formulation or Computation is carried out by running a query over these relations.
Installation in Linux :
Open a terminal (Ctrl+Alt+T) and type:
Syntax and Basic Fields :
In prolog, We declare some facts. These facts constitute the Knowledge Base of the system. We can query against the Knowledge Base. We get output as affirmative if our query is already in the knowledge Base or it is implied by Knowledge Base, otherwise we get output as negative. So, Knowledge Base can be considered similar to database, against which we can query. Prolog facts are expressed in definite pattern. Facts contain entities and their relation. Entities are written within the parenthesis separated by comma (, ). Their relation is expressed at the start and outside the parenthesis. Every fact/rule ends with a dot (.). So, a typical prolog fact goes as follows :
Key Features : 1. Unification : The basic idea is, can the given terms be made to represent the same structure. 2. Backtracking : When a task fails, prolog traces backwards and tries to satisfy previous task. 3. Recursion : Recursion is the basis for any search in program.
Running queries : A typical prolog query can be asked as :
Advantages : 1. Easy to build database. Doesn’t need a lot of programming effort. 2. Pattern matching is easy. Search is recursion based. 3. It has built in list handling. Makes it easier to play with any algorithm involving lists.
Disadvantages : 1. LISP (another logic programming language) dominates over prolog with respect to I/O features. 2. Sometimes input and output is not easy.
Applications :
Prolog is highly used in artificial intelligence(AI). Prolog is also used for pattern matching over natural language parse trees.
Reference 1: https://en.wikipedia.org/wiki/Prolog
Reference 2: http://www.swi-prolog.org/
Please Login to comment...
Similar reads.
- Programming Language

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Individual Work
Week 1. code+readme: 80%, writeup 20%, week 2: a choice, cryptarithmetic. code+readme 80%, writeup 20%, week 2: family matters. code+readme 80%, writeup 20%.
However, you must be careful that you do not write "circular" definitions, for example: parent(X,Y) :- child(Y,X). child(A,B) :- parent(B,A).
Weeks 3-4: Parsing into FOPC
Artificial Intelligence Programming in Prolog (AIPP)
- Lecture slides are posted before each lecture. Print them off from here if you wish to annotate them during lectures.
- Course notes are available for download ( here ). The course notes contain an introduction to Prolog as well as all practical exercises and revision exercises.
- Assignment 1 (An automatic puzzle solver) is available now (04/10/04). Download the specification and template file here .
- Practical code is available here along with updated instructions.
- ****** NO LECTURE Monday 25th October due to mid-semester break **********
- ****** Thursday 11th November lecture cancelled due to my absence *********
- Assignment 2 (Six Degrees of Kevin Bacon) now available here
- Final lecture is Monday 29/11/04
- Practical sessions continue in week 11 (29/11-3/12). Dedicated to catch-up and 2nd assignment.
Introduction
- Natural Language Processing and Generation,
- Knowledge Representation and Reasoning,
- Database Search,
- Problem Solving,
- Cognitive Modelling,
- Logic and Theorem Proving,
- as well as providing computational skills that can be utilised in their MSc project.
Module Description
Assignments, assignment 1 (10%): an automatic puzzle solver.
- Assignment 1 available now (04/10/04):
- Assignment specification (.pdf) (.ps)
- Prolog template: puzzle_template.pl
- due in week 7: Monday 1/11 4pm
Assignment 2 (20%): Six degrees of Kevin Bacon.
- Assignment 2 now available (08/11/04):
- Assignment specification (.pdf)
- Prolog Movie Database (PMDB) . You should download all of these files to your home directory but only use them as instructed in the assignment specification (* warning they are large files!)
- actors_1deg.pl
- actors_2deg.pl
- actors_Ndeg.pl
- due in week 11: Friday 10th December at 4pm
- Practical 4 (week 5) sticks.pl
- Alteration to practical instructions: The second part of this weeks practical, the Sticks problem, is now optional. Last week's practical exercises should be completed before the Sticks problem is attempted. If you do choose to attempt the Sticks problem then you will find better instructions than those in the course notes included in the sticks.pl file.
- Practical 5 (week 6) mandc.pl
- Practical 6 (week 7) grammar1.pl
- Finish the Missionaries and Cannibals practical from last week before moving on to this DCG practical.
- Practical 8 (week 9) prac8.pl
- Finish up to part 5 of last week's I/O practical before starting this.
- All predicates for this practical should be added to this file.
- The coursenotes refer to a file eliza.pl, this is contained within this file.
- Practical 9 (week 10)
- *Ignore the practical description in the coursenotes. You should work from the specification below.
- Practical specification: prac9.pdf
- Monkey and Bananas code: simstrips.pl
Examination
Requirements and exemptions.
- AI MSc students are required to know Java and Prolog by the end of their MSc.
- Cognitive Science and Natural Language MSc students have to learn either Prolog or Java.
- Speech and Language Processing MSc have to learn either Prolog or Java.
- Clocksin, W.F. and Mellish, C.S., Programming in Prolog: Using the ISO Standard (5th edition) , 2003.
- A good basic introductory text. This is the most up to date text book and conforms to the new ISO standard Prolog. This is the form of Prolog that will be taught in AIPP. If you choose to use a text book other than Clocksin and Mellish refer to the course notes for correct syntax. Doesn't contain many of the AI components of the course.
- Bratko, I., Prolog Programming for Artificial Intelligence (3rd edition) , 2001.
- An introductory book that leans heavily towards AI applications. It's structure resembles that of AIPP more than Clocksin and Mellish. Occasionally uses a poor programming style and incorrect syntax. Refer to course notes for correct syntax. A major source of example AI programs.
- Sterling, L. and Shapiro, E., The Art of Prolog (Second edition) , 1994.
- Possibly the best general Prolog book around, but definitely not an introduction, especially if you don't have much programming experience.
Useful Links
- Sicstus Prolog manual
- Learn Prolog Now! by Patrick Blackburn, Johan Bos, and Kristina Striegnitz
- Sicstus homepage
- The Internet Movie Database (IMDB)

More results...
- View all quizzes
- GCSE Concepts & Quizzes
- A Level Concepts & Quizzes
- Little Man Computer (LMC)
- Computer Networks
- Database Concepts
- Cryptography
- Python Challenges – Beginner
- Python Challenges – Intermediate
- Python Challenges – Advanced
- HTML, CSS & JavaScript
- BBC micro:bit
- OCR J277/01 – 1.1 System Architecture
- OCR J277/01 – 1.2 Memory and Storage
- OCR J277/01 – 1.3 Computer networks
- OCR J277/01 – 1.4 Network security
- OCR J277/01 – 1.5 – Systems software
- OCR J277/01 – 1.6 – Ethical, legal, cultural and environmental impacts of digital technology
- OCR J277/02 – 2.1 – Algorithms
- OCR J277/02 – 2.2 – Programming fundamentals
- OCR J277/02 – 2.3 – Producing robust programs
- OCR J277/02 – 2.4 – Boolean logic
- OCR J277/02 – 2.5 – Programming languages and Integrated Development Environments
- OCR H446/01 – 1.1 The characteristics of contemporary processors, input, output and storage devices
- OCR H446/01 – 1.2 Software and software development
- OCR H446/01 – 1.3 Exchanging data
- OCR H446/01 – 1.4 Data types, data structures and algorithms
- OCR H446/01 – 1.5 Legal, moral, cultural and ethical issues
- OCR H446/02 – 2.1 Elements of computational thinking
- OCR H446/01 – 2.2 Problem solving and programming
- OCR H446/02 – 2.3 Algorithms
- 101 Extra Python Challenges
- 101 Python Challenges
- 101 Computing Challenges
- Become a member!
- Your Account
- Solved Challenges
- Membership FAQ
Prolog – Family Tree

There are only three basic constructs in Prolog: facts , rules , and queries .
A collection of facts and rules is called a knowledge base (or a database) and Prolog programming is all about writing knowledge bases. That is, Prolog programs simply are knowledge bases, collections of facts and rules which describe some collection of relationships that we find interesting.
So how do we use a Prolog program? By posing queries . That is, by asking questions about the information stored in the knowledge base. The computer will automatically find the answer (either True or False) to our queries.
Source: http://www.learnprolognow.org/ Knowledge Base (Facts & Rules) Check the following knowledge base used to store the information that appears on a family tree:
Note that \+ means NOT Queries We can now query this database. For each of the queries listed below, what do you think the computer will return?
True or False Queries: The following queries would return either True or False.
Other Queries: The following queries would return a solution which could be either:
- A unique value
- A list of values
- False if no solution can be found
Your Task: Use a range of queries, similar to the one above to interrogate the knowledge base and complete the family tree provided below.
To run your queries you will need to use the online Prolog environment: https://swish.swi-prolog.org/p/prolog-family-tree.pl

Did you like this challenge?
Click on a star to rate it!
Average rating 4.7 / 5. Vote count: 24
No votes so far! Be the first to rate this post.
As you found this challenge interesting...
Follow us on social media!
Other challenges you may enjoy...

Recent Posts
- Ice Cream Price Calculator
- Revision Progress Tracker Algorithm
- Search Engine Indexing… In your own words…
- Average Lap Time Calculator
- Return On Investment Calculator
- Storage Units Conversions – Quiz
- Flappy Bird Animation using Pygame
- Adding a Timer using Python
- The MafiaBoy dDoS attack
- Snake Game Using Python
- View more recent posts...
- View all our challenges...
- Take a Quiz...

Our Latest Book

Related Posts
- Solving a Murder Mystery using Prolog
- Prolog – Sorting Hat Challenge
- Prolog – Food Web Challenge
- Solving a Zebra Puzzle using Prolog
- Computing Concepts
- Python Challenges
- Privacy Policy
Programming Assignment 4: Wumpus world in Prolog
Table of contents, problem domain, project infrastructure, running the code.
You will be considering the Wumpus world introduced in Russell and Norvig, Chapter 7. For this programming assignment you’ll use Prolog’s ability to do inference in order to write an agent that will make safe moves within its world.
You will need to download and install your own version of SWI-Prolog. It is freely available from http://www.swi-prolog.org/ for major operating systems. Major Linux distributions have copies available within their package system.
The code for this project consists of several Prolog files, some of which you will need to read and understand in order to complete the assignment. You can download all the code and supporting files as a zip archive .
If you read the output, you’ll see the default agent just moves forward. It does this in the world shown in Russell and Norvig’s Figure 7.2, which causes the agent’s dead rather soon. (You use ˆD to exit the programming environment.)
You will need to edit my_agent.pl produce a more rational movement. To do this you will need to define an intelligent run_agent(Percept,Action) . The percepts are provided as a list of five elements [Stench, Breeze, Glitter, Bump, Scream] , where you receive a “yes” or a “no” in the respective position. There is also an init_agent function which you can use for initialization.
The simulator knows the total state of the world, while your agent will know only what it senses through its perceptions. Initially the agent knows only the perceptions in position (1,1) and in order to acquire other information must be moved in other cells of the grid. Your agent must maintain its own state, containing all the information that gradually becomes available. The state will have to be update with the new perceptions every time an action is executed, and this information must be represented so as to permit reasoning (via interference) as to what operations are safe.
Submit your assignment via Canvas using these submission instructions .
You may discuss this openly with your friends and classmates, but are expected to write your own code and compile your submission independently. If in doubt about whether a resource you used should be included in the list of resources, err on the side of caution and include it.
Academic Integrity: "An Aggie does not lie, cheat, or steal, or tolerate those who do." For additional information please visit: http://student-rules.tamu.edu/aggiecode
Getting Help: You are not alone! If you find yourself stuck on something, contact the TA for help. Office hours are there for your support; please use them. If you can't make our office hours, let us know and we will schedule more. We want these projects to be rewarding and instructional, not frustrating and demoralizing. But, we don't know when or how to help unless you ask.
- Sources/building
- Docker images

- Command line
- Prolog syntax
- HTML generation
- Publications
- Rev 7 Extensions
- Getting started
- Development tools
- RDF namespaces
- GUI options
- Linux packages
- Report a bug
- Submit a patch
- Submit an add-on
- External links
- Contributing
- Code of Conduct
- Contributors
- SWI-Prolog items
- View changes
- transportation/4
- assignment/2

Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
EzzEl-DeenMohamed/ProLog_Assignment1_AI
Folders and files, repository files navigation, prolog_assignment1_ai, contributors 2.
- Prolog 31.3%
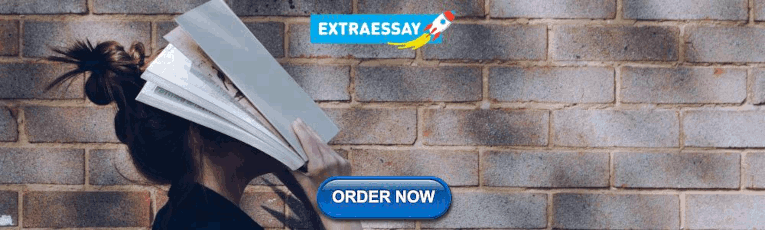
IMAGES
VIDEO
COMMENTS
Prolog is a logic programming language first specified in 1972, and refined into multiple modern implementations. ... Unification is % essentially a combination of assignment and equality! It works as % follows: % If both sides are bound (ie, defined), check equality. % If one side is free (ie, undefined), assign to match the other side. % If ...
Prolog does not have assignment. True, you can give a variable a value. But you cannot change that value later; X = X + 1 is meaningless in mathematics and it's meaningless in Prolog too. In general, you will be working recursively, so you will simply create a new variable when something like this needs to occur. It will make more sense as you ...
The overall objective of this assignment is for you to gain some hands-on experience with problem solving using Prolog, using simple facts and rules, recursion, and database handling capabilities of the language. The instructions for submission of your assignment may be found at the bottom of this page of this document.
X is less than or equal to Y. X =:= Y. the X and Y values are equal. X =\= Y. the X and Y values are not equal. You can see that the '=<' operator, '=:=' operator and '=\=' operators are syntactically different from other languages. Let us see some practical demonstration to this.
Mastering Prolog assignments goes beyond theoretical understanding, requiring a skillful blend of practical expertise and strategic approaches. This section delves into practical tips designed to enhance students' proficiency in Prolog programming. From effective debugging techniques that unravel the intricacies of code to time management ...
Prolog is a logic programming language. It has important role in artificial intelligence. Unlike many other programming languages, Prolog is intended primarily as a declarative programming language. In prolog, logic is expressed as relations (called as Facts and Rules). Core heart of prolog lies at the logic being applied.
Prolog is the fact that variables may be included in queries. A variable always begins with a capital letter. When a variable is seen, Prolog tries to find a value (binding) for the variable that will make the queried relation true. For example, fatherOf(X,harry). asks Prolog to find an value for X such that X's father is harry. When we ...
Prolog answers a query as "Yes" or "No" according to whether it can find a satisfying assignment. If it finds an assignment, it prints the first one before printing "Yes." You can press Enter to accept it, in which case you're done, or ";" to reject it, causing Prolog to backtrack and look for another. [ press ";" ]
Prolog Projects Individual Work Group work to get acquainted with Prolog is OK, and asking and answering general questions about how things work is OK. ... Also your writeup as always should explain exactly what problem you're solving, what goes beyond the assignment, what experiences you had that you think we should know about, etc. Week 1 ...
Dedicated to catch-up and 2nd assignment. Introduction Prolog(PROgrammation et LOGique) is a logic programming language widely utilised in Artificial Intelligence. It is a high-level programming language which enables the user to build programs by stating what they want the program to do rather than how it should do it. Due to Prolog's ...
Prolog is a language built around the Logical Paradigm: a declarative approach to problem-solving. There are only three basic constructs in Prolog: facts, rules, and queries. A collection of facts and rules is called a knowledge base (or a database) and Prolog programming is all about writing knowledge bases. That is, Prolog programs simply are knowledge bases, collections of facts and rules ...
Problem Domain. You will be considering the Wumpus world introduced in Russell and Norvig, Chapter 7. For this programming assignment you'll use Prolog's ability to do inference in order to write an agent that will make safe moves within its world.
Dec 29, 2023. --. Prolog, short for "Programming in Logic," stands out as a unique programming language with a profound impact on the field of Artificial Intelligence (AI). This article aims ...
This assignment is based on extending and/or modifying the contents of file treesearch.pl, which corresponds to the Prolog implementation of the basic tree search algorithm on slide 5 of uninformed search topic. Download this Prolog file from Moodle and store it in your working directory for this assignment.
The aim of the assignment is to give experience with typical Prolog programming techniques. Question 1.1: List Processing Write a predicate sumsq_even(Numbers, Sum) that sums the squares of only the even numbers in a list of integers.
An assignment with minimal cost is computed and unified with Assignment as a list of lists, representing an adjacency matrix. Tags are associated to your profile if you are logged in Tags:
ProLog_Assignment1_AI. About. No description, website, or topics provided. Resources. Readme Activity. Stars. 0 stars Watchers. 1 watching Forks. 0 forks Report repository Releases No releases published. Packages 0. No packages published . Contributors 2 . Languages. Perl 68.7%; Prolog 31.3%; Footer
The Moscow International Business Center (MIBC), also known as Moscow-City, is a commercial development in Moscow, the capital of Russia.The project occupies an area of 60 hectares, and is located just east of the Third Ring Road at the western edge of the Presnensky District in the Central Administrative Okrug.Construction of the MIBC takes place on the Presnenskaya Embankment of the Moskva ...
Prolog, renowned for its strength in logic programming and symbolic reasoning, plays a pivotal role in AI development. Its declarative nature simplifies computation logic, making it an ideal choice for knowledge representation in AI systems. ... preventing the assignment of incompatible values to variables. C++: C++ plays a vital role in AI ...
The City Hall and City Duma ( Russian: Здание Правительства Москвы) would have been the new home for the Moscow government and assembly ( duma ). It would have consisted of four 308.4 meter (1011.8 feet), 70 story towers. Currently, the government of the city are using hundreds of smaller buildings throughout the city.
On 22 March 2024, a terrorist attack which was carried out by the Islamic State (IS) occurred at the Crocus City Hall music venue in Krasnogorsk, Moscow Oblast, Russia.. The attack began at around 20:00 MSK (), shortly before the Russian band Picnic was scheduled to play a sold-out show at the venue. Four gunmen carried out a mass shooting, as well as slashing attacks on the people gathered at ...
The building in 1911. Shanyavsky Moscow City People's University ( Russian: Московский городской народный университет имени А. Л. Шанявского) was a university in Moscow that was founded in 1908 with funds from the gold mining philanthropist Alfons Shanyavsky. The university was nationalized ...