- Mailing List
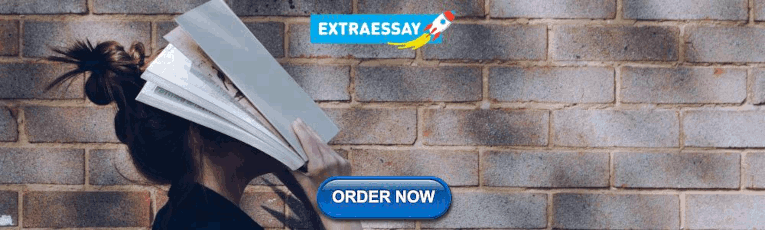
Practical Business Python
Taking care of business, one python script at a time
Creating Powerpoint Presentations with Python
Posted by Chris Moffitt in articles
Introduction
Love it or loathe it, PowerPoint is widely used in most business settings. This article will not debate the merits of PowerPoint but will show you how to use python to remove some of the drudgery of PowerPoint by automating the creation of PowerPoint slides using python.
Fortunately for us, there is an excellent python library for creating and updating PowerPoint files: python-pptx . The API is very well documented so it is pretty easy to use. The only tricky part is understanding the PowerPoint document structure including the various master layouts and elements. Once you understand the basics, it is relatively simple to automate the creation of your own PowerPoint slides. This article will walk through an example of reading in and analyzing some Excel data with pandas, creating tables and building a graph that can be embedded in a PowerPoint file.
PowerPoint File Basics
Python-pptx can create blank PowerPoint files but most people are going to prefer working with a predefined template that you can customize with your own content. Python-pptx’s API supports this process quite simply as long as you know a few things about your template.
Before diving into some code samples, there are two key components you need to understand: Slide Layouts and Placeholders . In the images below you can see an example of two different layouts as well as the template’s placeholders where you can populate your content.
In the image below, you can see that we are using Layout 0 and there is one placeholder on the slide at index 1.
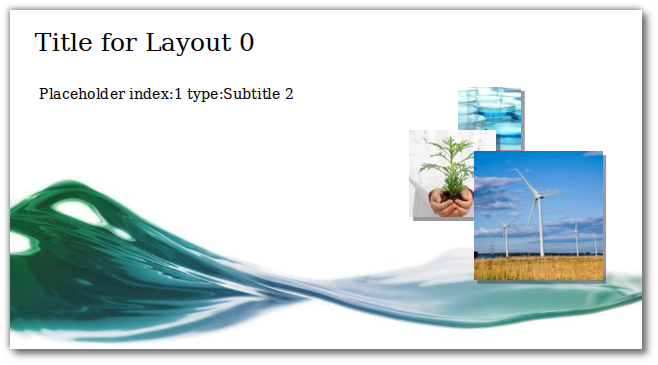
In this image, we use Layout 1 for a completely different look.
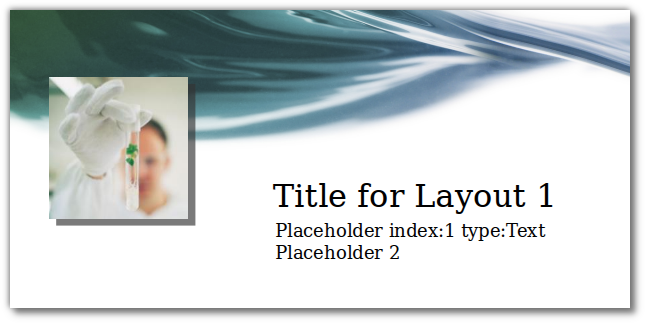
In order to make your life easier with your own templates, I created a simple standalone script that takes a template and marks it up with the various elements.
I won’t explain all the code line by line but you can see analyze_ppt.py on github. Here is the function that does the bulk of the work:
The basic flow of this function is to loop through and create an example of every layout included in the source PowerPoint file. Then on each slide, it will populate the title (if it exists). Finally, it will iterate through all of the placeholders included in the template and show the index of the placeholder as well as the type.
If you want to try it yourself:
Refer to the input and output files to see what you get.
Creating your own PowerPoint
For the dataset and analysis, I will be replicating the analysis in Generating Excel Reports from a Pandas Pivot Table . The article explains the pandas data manipulation in more detail so it will be helpful to make sure you are comfortable with it before going too much deeper into the code.
Let’s get things started with the inputs and basic shell of the program:
After we create our command line args, we read the source Excel file into a pandas DataFrame. Next, we use that DataFrame as an input to create the Pivot_table summary of the data:
Consult the Generating Excel Reports from a Pandas Pivot Table if this does not make sense to you.
The next piece of the analysis is creating a simple bar chart of sales performance by account:
Here is a scaled down version of the image:
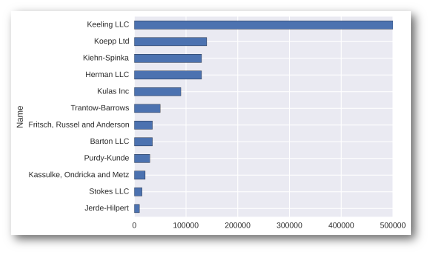
We have a chart and a pivot table completed. Now we are going to embed that information into a new PowerPoint file based on a given PowerPoint template file.
Before I go any farther, there are a couple of things to note. You need to know what layout you would like to use as well as where you want to populate your content. In looking at the output of analyze_ppt.py we know that the title slide is layout 0 and that it has a title attribute and a subtitle at placeholder 1.
Here is the start of the function that we use to create our output PowerPoint:
This code creates a new presentation based on our input file, adds a single slide and populates the title and subtitle on the slide. It looks like this:
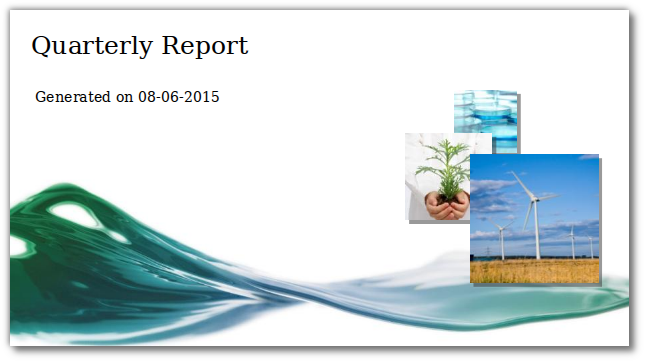
Pretty cool huh?
The next step is to embed our picture into a slide.
From our previous analysis, we know that the graph slide we want to use is layout index 8, so we create a new slide, add a title then add a picture into placeholder 1. The final step adds a subtitle at placeholder 2.
Here is our masterpiece:
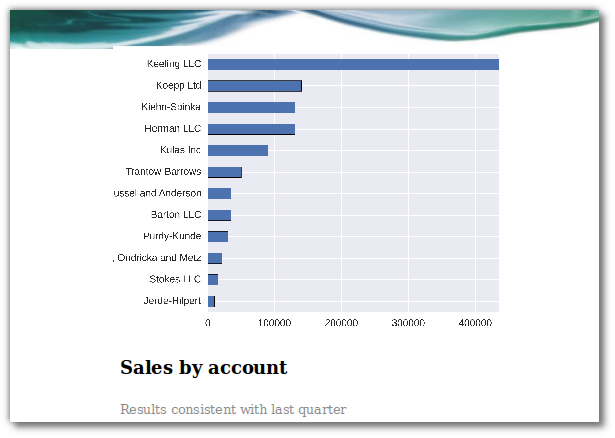
For the final portion of the presentation, we will create a table for each manager with their sales performance.
Here is an image of what we’re going to achieve:
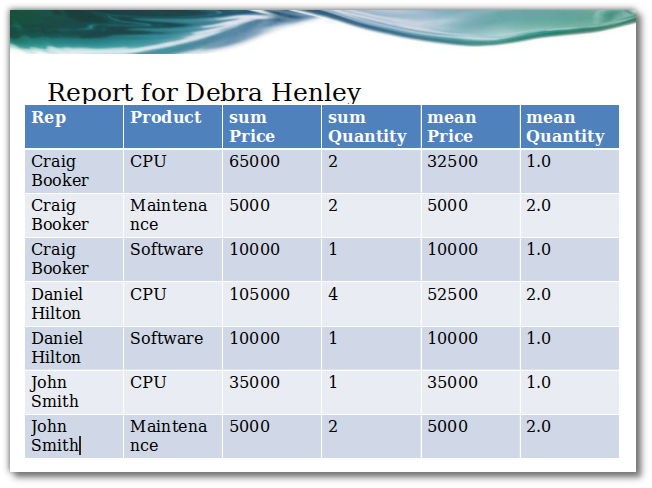
Creating tables in PowerPoint is a good news / bad news story. The good news is that there is an API to create one. The bad news is that you can’t easily convert a pandas DataFrame to a table using the built in API . However, we are very fortunate that someone has already done all the hard work for us and created PandasToPowerPoint .
This excellent piece of code takes a DataFrame and converts it to a PowerPoint compatible table. I have taken the liberty of including a portion of it in my script. The original has more functionality that I am not using so I encourage you to check out the repo and use it in your own code.
The code takes each manager out of the pivot table and builds a simple DataFrame that contains the summary data. Then uses the df_to_table to convert the DataFrame into a PowerPoint compatible table.
If you want to run this on your own, the full code would look something like this:
All of the relevant files are available in the github repository .
One of the things I really enjoy about using python to solve real world business problems is that I am frequently pleasantly surprised at the rich ecosystem of very well thought out python tools already available to help with my problems. In this specific case, PowerPoint is rarely a joy to use but it is a necessity in many environments.
After reading this article, you should know that there is some hope for you next time you are asked to create a bunch of reports in PowerPoint. Keep this article in mind and see if you can find a way to automate away some of the tedium!
- ← Best Practices for Managing Your Code Library
- Adding a Simple GUI to Your Pandas Script →
Subscribe to the mailing list
Submit a topic.
- Suggest a topic for a post
- Pandas Pivot Table Explained
- Common Excel Tasks Demonstrated in Pandas
- Overview of Python Visualization Tools
- Guide to Encoding Categorical Values in Python
- Overview of Pandas Data Types
Article Roadmap
We are a participant in the Amazon Services LLC Associates Program, an affiliate advertising program designed to provide a means for us to earn fees by linking to Amazon.com and affiliated sites.
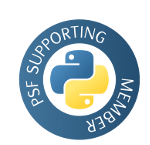
5 Best Ways to Create PowerPoint Files Using Python
💡 Problem Formulation: Automating the creation of PowerPoint presentations is a common task for those who need to generate reports or summaries regularly. For instance, a user may wish to create a presentation summarizing sales data from a CSV file or visualize a project’s progress in a structured format. The desired output is a fully formatted PowerPoint file (.pptx) with various elements like titles, texts, images, and charts, as specified by the input data or customization requirements.
Method 1: Using python-pptx
The python-pptx library provides a comprehensive set of features for creating PowerPoint files (.pptx) in Python. It allows for adding slides, text, images, charts, and more, with a high level of customization. Manipulate slides at a granular level by accessing placeholders, creating bulleted lists, and setting properties like font size or color programmatically.
Here’s an example:
The code snippet above creates a PowerPoint file named python-pptx-presentation.pptx with one slide that includes a title and a subtitle.
In this overview, we create a presentation object, add a new slide with a predefined layout, set text for the title and subtitle placeholders, and then save the presentation. This method gives users the ability to create detailed, professional presentations through code.
Method 2: Using Pandas with python-pptx
This method combines the data manipulation power of Pandas with the presentation capabilities of python-pptx to create PowerPoint files from DataFrame contents. It’s particularly useful for automating the inclusion of tabular data or creating charts based on the DataFrame’s data.
The output is a PowerPoint file named pandas-python-pptx.pptx containing a bar chart representing the quantity of fruits.
This snippet demonstrates using a Pandas DataFrame to generate chart data, which is then used to create a chart in a PowerPoint slide. It showcases the synergy between Pandas for data handling and python-pptx for presentation creation.
Method 3: Using ReportLab with python-pptx
Those seeking to include complex graphics or generate custom visuals can harness the graphic-drawing capabilities of ReportLab with python-pptx. This method leverages ReportLab to create an image, which can then be inserted into a PowerPoint slide.
The output would be a PowerPoint file named reportlab-pptx.pptx containing a slide with a custom bar chart image.
The code above creates a bar chart using ReportLab, saves the chart as an image, and then inserts the image into a PowerPoint slide. This approach is ideal if you need to include bespoke graphics that are not directly supported by python-pptx itself.
Method 4: Using Matplotlib with python-pptx
For those familiar with Matplotlib, this method involves creating a visual plot or chart with Matplotlib, saving it as an image, and then embedding the image into a PowerPoint slide using python-pptx.
The outcome is a PowerPoint file matplotlib-pptx.pptx , with a plot on a slide created by Matplotlib.
In this case, we graph a quadratic function using Matplotlib, save it as an image, and then add that image to a slide in our PowerPoint presentation. This method offers a blend of Matplotlib’s sophisticated plotting tools with the simplicity of python-pptx.
Bonus One-Liner Method 5: Using Officegen
The Officegen package allows for rapid PowerPoint creation with simpler syntax, although with less flexibility compared to python-pptx. It provides functions to add slides, titles, and bullet points.
The outcome is a PowerPoint file officegen-presentation.pptx with a single slide containing a large title.
This snippet uses Officegen to initiate a new presentation, adds a text title to a slide, and saves the presentation. While not as detailed as python-pptx, Officegen is quick for simple presentations.
Summary/Discussion
- Method 1: python-pptx. Full-featured control over presentations. Can be verbose for simple tasks.
- Method 2: Pandas with python-pptx. Ideal for data-driven presentations. Setup can be complex if unfamiliar with data libraries.
- Method 3: ReportLab with python-pptx. Powerful combo for custom graphics. Requires separate handling of graphics and presentation stages.
- Method 4: Matplotlib with python-pptx. Best for users comfortable with Matplotlib. Less direct than using python-pptx alone.
- Bonus Method 5: Officegen. Quick and easy for simple presentations. Limited customization options.
Emily Rosemary Collins is a tech enthusiast with a strong background in computer science, always staying up-to-date with the latest trends and innovations. Apart from her love for technology, Emily enjoys exploring the great outdoors, participating in local community events, and dedicating her free time to painting and photography. Her interests and passion for personal growth make her an engaging conversationalist and a reliable source of knowledge in the ever-evolving world of technology.
Create interactive slides with Python in 8 Jupyter Notebook cells
Creating presentations in Jupyter Notebook is a great alternative to manually updating slides in other presentation creation software. If your data changes, you just re-execute the cell and slide chart is updated.
Jupyter Notebook is using Reveal.js (opens in a new tab) for creating slides from cells. The standard approach is to write slides code and Markdown in the Jupyter Notebook. When notebook is ready, it can be exported to standalone HTML file with presentation.
What if, you would like to update slides during the slide show? What is more, it would be fantastic to have interactive widgets in the presentation. You can do this in Mercury framework.
In this tutorial, we will create an interactive presentation in Jupyter Notebook and serve it with Mercury.
Create presentation in notebook
Please enable Slideshow toolbar in Jupyter Notebook. It can be done by clicking View -> Cell Toolbar -> Slideshow . It is presented in the screenshot below:
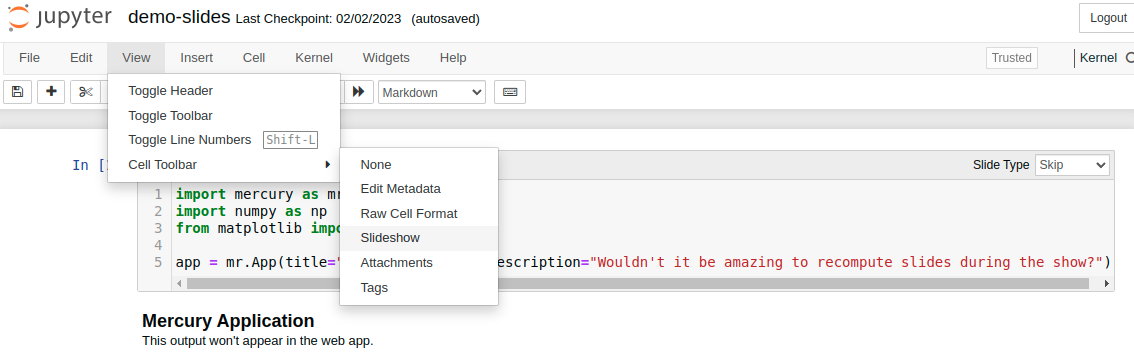
We will need following packages to create presentation in Python notebook:
Please make sure that they are installed in your environment.
1. Import packages and App setup
The first step is to import packages and setup Mercury App :
We setup title and description for App object.
Please note that we set Slide Type to Skip . This cell will not appear in the presentation.
2. Add title
The second cell is a Markdown with title:
The Slide Type is set to Slide . It is our first slide!
3. Add slide with Markdown
Add new Markdown cell with the following cell.
Please set Slide Type to Slide . It will be a second slide. I'm using ## as slide title ( # will produce too large title in my opinion).
4. Add Mercury Widget
Please add code cell with Text widget. We will use it, to ask users about their name.
We set Slide Type as Skip , so this cell will not appear in the presentation.
5. Display name
Let's use the name.value in the slide. Please add a code cell. We will display a Markdown text with Python variables by using Markdown function from Mercury package.
Please set the Slide Type to Slide .
You can display Markdown with Python variables by calling mr.Markdown() or mr.Md() functions. Both do the same.
The first five cells of the notebook:
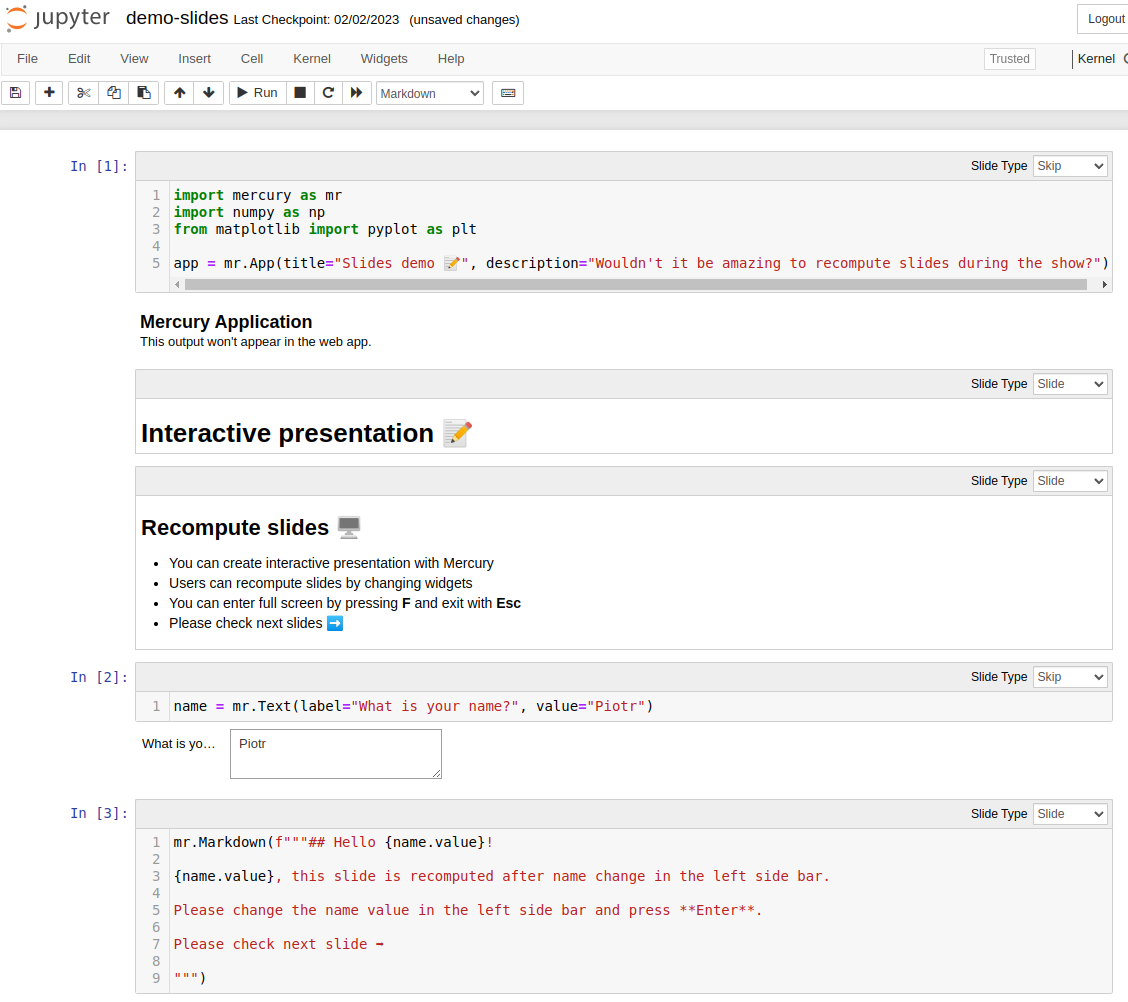
You can enter your name in the widget during the notebook development. There will be no change in other cells. If you want to update the cell with new widget value, please execute it manually.
6. More widgets
We can add more widgets to the presentation. They will be used to control chart in the next slide.
We have used Slider and Select widgets. They are displayed in the notebook. This cell will not be displayed in the presentation, so set Slide Type to Skip .
7. Scatter plot
We will add a new code cell. It will have Slide Type set to Slide .
We used widgets values by accessing them with samples.value and color.value .
Screenshot of the notebook with scatter plot:
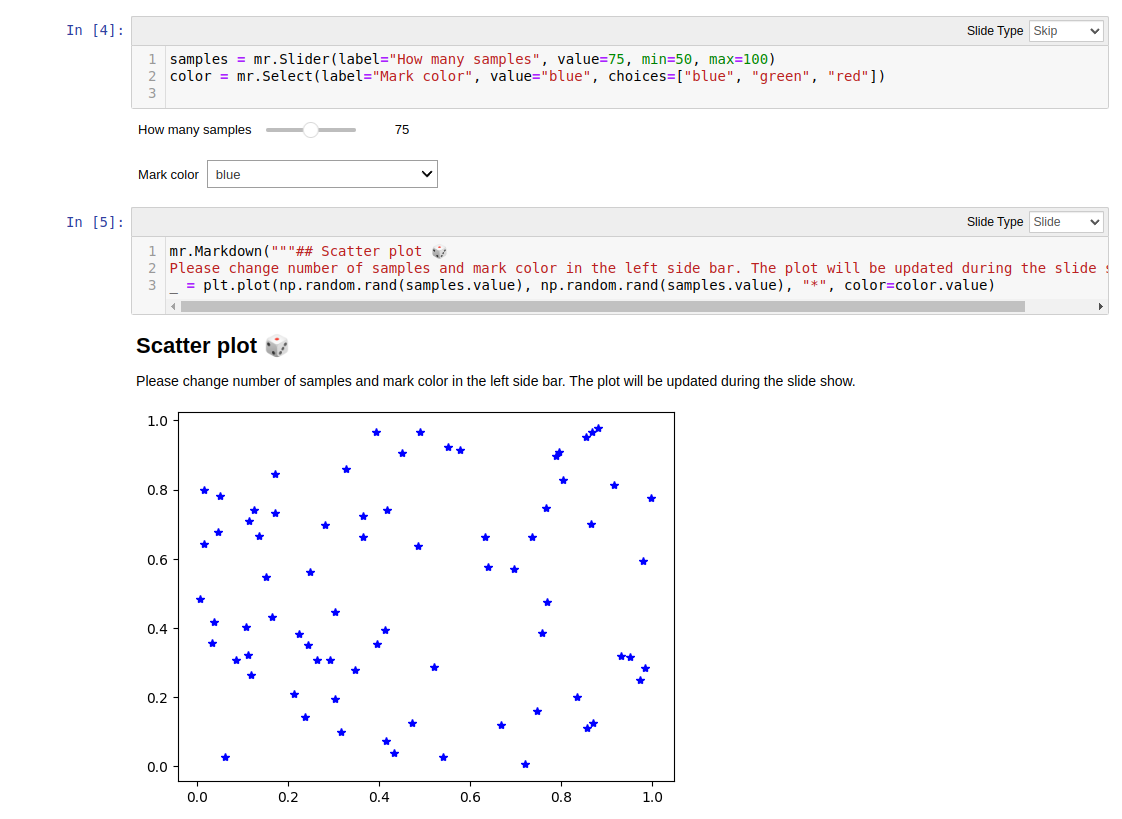
8. Final slide
Please add a last Markdown cell. Its Slide Type will be set to Slide :
Please notice that link is added with HTML syntax. There is a target="_blank" used to open link in a new tab.
Run presentation in Mercury
Please run Mercury local server in the same directory as notebook:
The above command will open a web browser at http://127.0.0.1:8000 . Please click on a card with presentation.
You can navigate between slides with arrows in the bottom right corner. You can enter the full screen mode by pressing F on the keyboard. Please use Esc to exit full screen mode.
You can change widgets values in the sidebar and presentation slides will be automatically recomputed:
You can export your slides as PDF or HTML by clicking Download button in the sidebar.
python-pptx-interface 0.0.12
pip install python-pptx-interface Copy PIP instructions
Released: Aug 15, 2020
Easy interface to create pptx-files using python-pptx
Verified details
Maintainers.
Unverified details
Project links, github statistics.
- Open issues:
View statistics for this project via Libraries.io , or by using our public dataset on Google BigQuery
License: MIT
Author: Nathanael Jöhrmann
Project description
python-pptx is a great module to create pptx-files. But it can be challenging to master the complex syntax. This module tries to present an easier interface for python-pptx to create PowerPoint files. It also adds some still missing features like moving slides, create links to other slides or remove unused place-holders. If matplotlib is installed, it can be used, to write formulas with latex syntax. And if PowerPoint is available, a presentation could be exported as pdf or png(s).
class PPTXCreator : Create pptx-File from template, incluing methods to add text, tables, figures etc. class PPTXPosition : Allows positioning as fraction of slide height/width. Style sheets class PPTXFontStyle : Helps to set/change/copy fonts. class PPTXParagraphStyle : Format paragraphs (alignment, indent …). class PPTXTableStyle : Used to layout tables. class PPTXFillStyle Working with templates class AbstractTemplate : Base class for all custom templates (enforce necessary attributes) class TemplateExample : Example class to show how to work with custom templates utils.py : A collection of useful functions, eg. to generate PDF or PNG from *.pptx (needs PowerPoint installed) Examples : Collection of examples demonstrating how to use python-pptx-interface. Example : demonstrates usage of some key-features of python-pptx-interface with explanations General example 01 : demonstrates usage of some key-features of python-pptx-interface Font style example 01 Table style example 01
class PPTXCreator
This class provides an easy interface to create a PowerPoint presentation via python-pptx. It has methods to add slides and shapes (tables, textboxes, matplotlib figures) setting format by using layouts and stylesheets. It also has methods to move slides around, remove empty placeholders or create hyperlinks.
Methods defined:
Add a content slide with hyperlinks to all other slides and puts it to position slide_index.
Add the given latex-like math-formula as an image to the presentation using matplotlib.
Add a motplotlib figure to slide and position it via position. Optional parameter zoom sets image scaling in PowerPoint. Only used if width not in kwargs (default = 1.0).
Add a new slide to presentation. If no layout is given, default_layout is used.
Add a table shape with given table_data at position using table_style. (table_data: outer iter -> rows, inner iter cols; auto_merge: not implemented jet)
Add a text box with given text using given position and font. Uses self.default_position if no position is given.
Add a new slide to presentation. If no layout is given, title_layout is used.
Move the given slide to position new_index.
Save presentation under the given filename.
Save the presentation as pdf under the given filenmae. Needs PowerPoint installed.
Saves the presentation as PNG’s in the given folder. Needs PowerPoint installed.
Static methods defined:
Make the given run a hyperlink to to_slide.
Removes empty placeholders (e.g. due to layout) from slide. Further testing needed.
Properties defined:
class PPTXPosition
To position shapes in a slide, many methods of PPTXCreator except a PPTXPosition parameter. It allows to give a position relative to slide width and high (as a fraction). Additionally you can specify the position in inches starting from the relative position. Some stylesheets e.g. PPTXTableStyle can also have an optional PPTXPosition attribute. In that case writing the style to a shape will also set its position.
Returns a kwargs dict containing “left” and “top”.
Returns an args tuple containing “left” and “top”.
Stylesheets
While python-pptx-interface can load a template file with placeholders, the intended use case is more focused on creating and positioning shapes like tables, pictures, textboxes etc. directly in python. Therefore all unused placeholders are removed by default, when creating a new slide. As it can be quite tedious to do all the necessary formatting directly using python-pptx, this package provides some style sheet like classes, to define a certain format and than “write” it to the created shapes. In general python-pptx-interface styles only change parameters, that have been set. E.g. when creating a PPTXFontStyle instance and setting the font size, using this style will only change the font size, but not color, bold … attributes. Beside setting an attribute or not changing an attribute there is a third case - using the default value as it is defined e.g. in the master slide. For that case, the value _USE_DEFAULT can be used.
To be consistent, python-pptx-interface will not change anything if an attribute is set to None. This can differ from the pyrhon-pptx behaviour in some cases, where None means “use default”.
class PPTXFontStyle
font-style example
Read attributes from a pptx.text.text.Font object.
Convenience method to set several font attributes together.
Write attributes to a pptx.text.text.Font object.
Write attributes to given paragraph.
Write attributes to given run.
Write attributes to all paragraphs in given pptx.shapes.autoshape.Shape. Raises TypeError if given shape has no text_frame or table.
Write attributes to all paragraphs in given text_frame.
class PPTXParagraphStyle
paragraph-style example ,
Read attributes from a _Paragraph object.
Convenience method to set several paragraph attributes together.
class PPTXTableStyle
table-style example ,
Read attributes from a Table object, ignoring font and cell style.
Convenience method to set several table attributes together.
Set table width as fraction of slide width.
“””Write attributes to table in given pptx.shapes.autoshape.Shape.””” Raises TypeError if given shape has no text_frame or table.
“””Write attributes to table object.”””
class PPTXFillStyle
Convenience method to set several fill attributes together.
Write attributes to a FillFormat object.
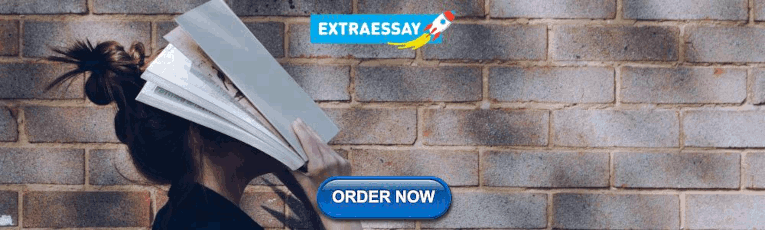
Working with templates
Class abstracttemplate, class templateexample.
This module comes with a general example , that you could run like
This will create an example.pptx, using some of the key-features of python-pptx-interface. Lets have a closer look:
First we need to import some stuff. PPTXCreator is the class used to create a *.pptx file. PPTXPosition allows as to position shapes in more intuitive units of slide width/height. font_title is a function returning a PPTXFontStyle instance. We will use it to change the formatting of the title shape. TemplateExample is a class providing access to the example-template.pptx included in python-pptx-interface and also setting some texts on the master slides like author, date and website. You could use it as reference on how to use your own template files by subclassing AbstractTemplate (you need at least to specify a path to your template and define a default_layout and a title_layout).
MSO_LANGUAGE_ID is used to set the language of text and MSO_TEXT_UNDERLINE_TYPE is used to format underlining.
Importing matplotlib is optional - it is used to demonstrate, how to get a matplotlib figure into your presentation.
Now we create our presentation with PPTXCreator using the TemplateExample . We also set the default font language and name of all PPTXFontStyle instances. This is not necessary, as ENGLISH_UK and Roboto are the defaults anyway. But in principle you could change these settings here, to fit your needs. If you create your own template class, you might also set these default parameters there. Finally we add a title slide and change the font style of the title using title_font().
Next, we add three slides, and create a content slide with hyperlinks to all other slides. By default, it is put to the second position (you could specify the position using the optional slide_index parameter).
Lets add some more stuff to the title slide.
PPTXCreator.add_text_box() places a new text shape on a slide with the given text. Optionally it accepts a PPTXPosition and a PPTXFont. With PPTXPosition(0.02, 0.24) we position the figure 0.02 slide widths from left and 0.24 slide heights from top.
We can use my_font to format individual paragraphs in a text_frame with PPTXFontStyle.write_paragraph() . Via PPTXFontStyle.set() easily customize the font before using it.
we can also easily add a table. First we define all the data we want to put in the table. Here we use a list of lists. But add_table is more flexible and can work with anything, that is an Iterable of Iterable. The outer iterable defines, how many rows the table will have. The longest inner iterable is used to get the number of columns.
If matplotlib is installed, we use it to create a demo figure, and add it to the title_slide. With PPTXPosition(0.3, 0.4) we position the figure 0.3 slide widths from left and 0.4 slide heights from top. PPTXPosition has two more optional parameters, to further position with inches values (starting from the relative position).
Finally, we save the example as example.pptx.
If you are on windows an have PowerPoint installed, you could use some additional features.
General example 01
general example 01
Font style example 01
font style example 01
Table style example 01
table style example 01
Requirements
Optional requirements, contribution.
Help with this project is welcome. You could report bugs or ask for improvements by creating a new issue.
If you want to contribute code, here are some additional notes:
Project details
Release history release notifications | rss feed.
Aug 15, 2020
Feb 15, 2020
Feb 11, 2020
Feb 2, 2020
Jan 29, 2020
Jan 28, 2020
Jan 19, 2020
Jan 17, 2020
Jan 15, 2020
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages .
Source Distributions
Built distribution.
Uploaded Aug 15, 2020 Python 3
Hashes for python_pptx_interface-0.0.12-py3-none-any.whl
- português (Brasil)
Supported by
Automating PowerPoint With Python-pptx
In daily work, we always need to create or modify PPT. But you can also use Python to create or modify PPT files. This article will tell you how to use the Python-pptx module to generate ppt automatically or with a PPT template and how to modify the existing PPT with examples.
Table of Contents
1. The Python Module python-pptx.
- The python-pptx is a library for Python to process ppt files. It focuses on reading and writing, cannot be exported, and has no rendering function.
- Before you can use the python-pptx module, you need to run the command pip3 install -i https://pypi.doubanio.com/simple/python-pptx in a terminal to install it. $ pip3 install -i https://pypi.doubanio.com/simple/ python-pptx Looking in indexes: https://pypi.doubanio.com/simple/ Collecting python-pptx Downloading https://pypi.doubanio.com/packages/eb/c3/bd8f2316a790291ef5aa5225c740fa60e2cf754376e90cb1a44fde056830/python-pptx-0.6.21.tar.gz (10.1 MB) |████████████████████████████████| 10.1 MB 2.1 MB/s Preparing metadata (setup.py) ... done Requirement already satisfied: lxml>=3.1.0 in /Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/site-packages (from python-pptx) (4.6.2) Requirement already satisfied: Pillow>=3.3.2 in /Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/site-packages (from python-pptx) (9.0.1) Requirement already satisfied: XlsxWriter>=0.5.7 in /Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/site-packages (from python-pptx) (1.3.7) Using legacy 'setup.py install' for python-pptx, since package 'wheel' is not installed. Installing collected packages: python-pptx Running setup.py install for python-pptx ... done Successfully installed python-pptx-0.6.21
- Run the command pip show python-pptx in the terminal to confirm the installation. $ pip show python-pptx Name: python-pptx Version: 0.6.21 Summary: Generate and manipulate Open XML PowerPoint (.pptx) files Home-page: http://github.com/scanny/python-pptx Author: Steve Canny Author-email: [email protected] License: The MIT License (MIT) Location: /Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/site-packages Requires: lxml, Pillow, XlsxWriter Required-by:
2. How To Use python-pptx Module To Create A PPT With Simple Text.
- The below example source code will create a PPT using the python-pptx module.
- It will also set the PPT title and subtitle text, and then save it to a pptx file. # Import the Presentation class from the pptx module. from pptx import Presentation # Create an instance of the Presentation class. prs = Presentation() # Create the title slide. title_slide_layout = prs.slide_layouts[0] # Add the title slide to the PPT slides array. slide = prs.slides.add_slide(title_slide_layout) # Get the PPT title object. title = slide.shapes.title # Get the PPT subtitle object. subtitle = slide.placeholders[1] # Set the PPT title. title.text = "Hello python-pptx Module!" # Set the PPT title slide sub title. subtitle.text = "pip install python-pptx" # Save the PPT to a .pptx file. prs.save("test.pptx")
3. How To Use python-pptx Module To Add Chart To A PPT File.
- The below source code can create a chart and add it to the output PPT file. # Import the Presentation class from the pptx module. from pptx import Presentation # Import the ChartData class form the pptx.chart.data package. from pptx.chart.data import ChartData # Import the chart type constants variable. from pptx.enum.chart import XL_CHART_TYPE # Import the units type. from pptx.util import Inches # Create the Presentation object to build the PPT file. prs = Presentation() # Add a slide to the PPT file. slide = prs.slides.add_slide(prs.slide_layouts[5]) # Create the ChartData object to save the chart data. chart_data = ChartData() # Save the categories data in an array, the categories data will be displayed in the horizontal x axis . chart_data.categories = ['Java', 'Python', 'JavaScript'] # Save the series data in a tuple, the series data will be displayed in the vertial y axis. chart_data.add_series('Series 1', (19.2, 21.4, 16.7)) # Define the x, y axis unit. x, y, cx, cy = Inches(2), Inches(2), Inches(6), Inches(4.5) # Add the column chart to the PPT slide. slide.shapes.add_chart( XL_CHART_TYPE.COLUMN_CLUSTERED, x, y, cx, cy, chart_data ) # Save the PPT file. prs.save('chart-01.pptx')
4. How To Use PPT Template To Generate PPT File With Python-pptx Module.
- Prepare a ppt template file ( you can download one from the internet or create a template PPT file by yourself).
- Load the PPT template file and use the specified slide style in your python source code using the python-pptx module.
- Add data to the PPT template file and generate a new PPT file.
- Below is the example source code. # Import the pptx module Presentation class. from pptx import Presentation # Import the pptx x axis unit. from pptx.util import Inches from pptx.util import Cm #Inches # Import the ChartData class. from pptx.chart.data import ChartData # Import the Chart type XL_CHART_TYPE. from pptx.enum.chart import XL_CHART_TYPE from pptx.enum.chart import XL_LEGEND_POSITION if __name__ == '__main__': # Create the Presentation object based on the template PPT file. prs = Presentation('template.pptx') # Add the first slide, title only slide. title_only_slide_layout = prs.slide_layouts[5] slide = prs.slides.add_slide(title_only_slide_layout) shapes = slide.shapes # Set the slide title text shapes.title.text = 'Report' # Define the chart table data in an array. name_objects = ["object1", "object2", "object3"] name_AIs = ["AI1", "AI2", "AI3"] val_AI1 = (19.2, 21.4, 16.7) val_AI2 = (22.3, 28.6, 15.2) val_AI3 = (20.4, 26.3, 14.2) val_AIs = [val_AI1, val_AI2, val_AI3] # Define the chart table style. rows = 4 cols = 4 top = Cm(12.5) left = Cm(3.5) #Inches(2.0) width = Cm(24) # Inches(6.0) height = Cm(6) # Inches(0.8) # Add the chart table to the slide. table = shapes.add_table(rows, cols, left, top, width, height).table # Set the table column width. table.columns[0].width = Cm(6)# Inches(2.0) table.columns[1].width = Cm(6) table.columns[2].width = Cm(6) table.columns[3].width = Cm(6) # Set the table text row. table.cell(0, 1).text = name_objects[0] table.cell(0, 2).text = name_objects[1] table.cell(0, 3).text = name_objects[2] # Fill data to the table. table.cell(1, 0).text = name_AIs[0] table.cell(1, 1).text = str(val_AI1[0]) table.cell(1, 2).text = str(val_AI1[1]) table.cell(1, 3).text = str(val_AI1[2]) table.cell(2, 0).text = name_AIs[1] table.cell(2, 1).text = str(val_AI2[0]) table.cell(2, 2).text = str(val_AI2[1]) table.cell(2, 3).text = str(val_AI2[2]) table.cell(3, 0).text = name_AIs[2] table.cell(3, 1).text = str(val_AI3[0]) table.cell(3, 2).text = str(val_AI3[1]) table.cell(3, 3).text = str(val_AI3[2]) # Define the ChartData object. chart_data = ChartData() chart_data.categories = name_objects chart_data.add_series(name_AIs[0], val_AI1) chart_data.add_series(name_AIs[1], val_AI2) chart_data.add_series(name_AIs[2], val_AI3) # Add the chart to the PPT file. x, y, cx, cy = Cm(3.5), Cm(4.2), Cm(24), Cm(8) graphic_frame = slide.shapes.add_chart( XL_CHART_TYPE.COLUMN_CLUSTERED, x, y, cx, cy, chart_data ) chart = graphic_frame.chart chart.has_legend = True chart.legend.position = XL_LEGEND_POSITION.TOP chart.legend.include_in_layout = False value_axis = chart.value_axis value_axis.maximum_scale = 100.0 value_axis.has_title = True value_axis.axis_title.has_text_frame = True value_axis.axis_title.text_frame.text = "False positive" value_axis.axis_title.text_frame.auto_size # Save a new PPT file based on the template. prs.save('test_template.pptx')
Leave a Comment Cancel Reply
Your email address will not be published. Required fields are marked *
This site uses Akismet to reduce spam. Learn how your comment data is processed .
You are using an outdated browser. Please upgrade your browser to improve your experience.
Presentations Tool in Python
How to create and publish a spectacle-presentation with the Python API.
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials .
Plotly Presentations ¶
To use Plotly's Presentations API you will write your presentation code in a string of markdown and then pass that through the Presentations API function pres.Presentation() . This creates a JSON version of your presentation. To upload the presentation online pass it through py.presentation_ops.upload() .
In your string, use --- on a single line to seperate two slides. To put a title in your slide, put a line that starts with any number of # s. Only your first title will be appear in your slide. A title looks like:
# slide title
Anything that comes after the title will be put as text in your slide. Check out the example below to see this in action.
Current Limitations ¶
Boldface , italics and hypertext are not supported features of the Presentation API.
Display in Jupyter ¶
The function below generates HTML code to display the presentation in an iframe directly in Jupyter.
Simple Example ¶
https://plotly.com/~AdamKulidjian/3700/simple-pres/
Click on the presentation above and use left/right arrow keys to flip through the slides.
Insert Plotly Chart ¶
If you want to insert a Plotly chart into your presentation, all you need to do is write a line in your presentation that takes the form:
Plotly(url)
where url is a Plotly url. For example:
Plotly(https://plotly.com/~AdamKulidjian/3564)
The Plotly url lines should be written on a separate line after your title line. You can put as many images in your slide as you want, as the API will arrange them on the slide automatically, but it is highly encouraged that you use 4 OR FEWER IMAGES PER SLIDE . This will produce the cleanest look.
Useful Tip : For Plotly charts it is HIGHLY ADVISED that you use a chart that has layout['autosize'] set to True . If it is False the image may be cropped or only partially visible when it appears in the presentation slide.
https://plotly.com/~AdamKulidjian/3710/pres-with-plotly-chart/
Insert Web Images ¶
To insert an image from the web, insert the a Image(url) where url is the image url.
https://plotly.com/~AdamKulidjian/3702/pres-with-images/
Image Stretch ¶
If you want to ensure that your image maintains its original width:height ratio, include the parameter imgStretch=False in your pres.Presentation() function call.
https://plotly.com/~AdamKulidjian/3703/pres-with-no-imgstretch/
Transitions ¶
You can specify how your want your slides to transition to one another. Just like in the Plotly Presentation Application, there are 4 types of transitions: slide , zoom , fade and spin .
To apply any combination of these transition to a slide, just insert transitions at the top of the slide as follows:
transition: slide, zoom
Make sure that this line comes before any heading that you define in the slide, i.e. like this:
Reference ¶
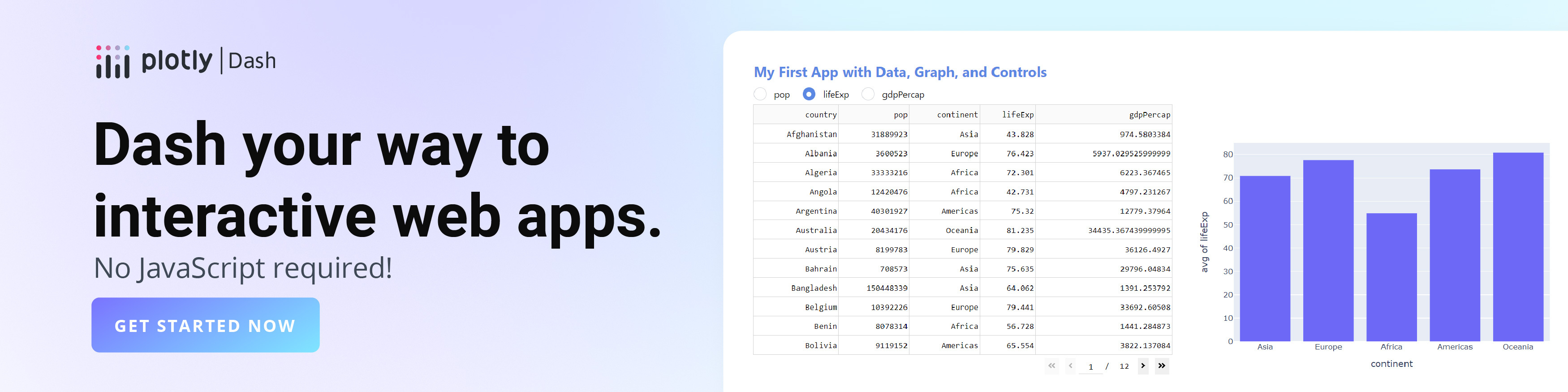
- Google Workspace
- Español – América Latina
- Português – Brasil
- Tiếng Việt
- Google Slides
Python quickstart
Quickstarts explain how to set up and run an app that calls a Google Workspace API.
Google Workspace quickstarts use the API client libraries to handle some details of the authentication and authorization flow. We recommend that you use the client libraries for your own apps. This quickstart uses a simplified authentication approach that is appropriate for a testing environment. For a production environment, we recommend learning about authentication and authorization before choosing the access credentials that are appropriate for your app.
Create a Python command-line application that makes requests to the Google Slides API.
- Set up your environment.
- Install the client library.
- Set up the sample.
- Run the sample.
Prerequisites
To run this quickstart, you need the following prerequisites:
- Python 3.10.7 or greater
- The pip package management tool
- A Google Cloud project .
- A Google Account.
Set up your environment
To complete this quickstart, set up your environment.
Enable the API
In the Google Cloud console, enable the Google Slides API.
Configure the OAuth consent screen
If you're using a new Google Cloud project to complete this quickstart, configure the OAuth consent screen and add yourself as a test user. If you've already completed this step for your Cloud project, skip to the next section.
Go to OAuth consent screen
- For User type select Internal , then click Create .
- Complete the app registration form, then click Save and Continue .
For now, you can skip adding scopes and click Save and Continue . In the future, when you create an app for use outside of your Google Workspace organization, you must change the User type to External , and then, add the authorization scopes that your app requires.
- Review your app registration summary. To make changes, click Edit . If the app registration looks OK, click Back to Dashboard .
Authorize credentials for a desktop application
Go to Credentials
- Click Create Credentials > OAuth client ID .
- Click Application type > Desktop app .
- In the Name field, type a name for the credential. This name is only shown in the Google Cloud console.
- Click Create . The OAuth client created screen appears, showing your new Client ID and Client secret.
- Click OK . The newly created credential appears under OAuth 2.0 Client IDs.
- Save the downloaded JSON file as credentials.json , and move the file to your working directory.
Install the Google client library
Install the Google client library for Python:
Configure the sample
- In your working directory, create a file named quickstart.py .
Include the following code in quickstart.py :
Run the sample
In your working directory, build and run the sample:
- If you're not already signed in to your Google Account, sign in when prompted. If you're signed in to multiple accounts, select one account to use for authorization.
- Click Accept .
Your Python application runs and calls the Google Slides API.
Authorization information is stored in the file system, so the next time you run the sample code, you aren't prompted for authorization.
- Troubleshoot authentication and authorization issues
- Slides API reference documentation
- Google APIs Client for Python documentation
- Google Slides API PyDoc documentation
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2024-04-03 UTC.
Python操作PDF全总结|pdfplumber&PyPDF2
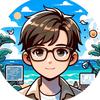
持续分享Python入门、案例、工具教程。
Python在自动化办公方面有很多实用的第三方库,可以很方便的处理word、excel、ppt、pdf文件,今天我们就学习一下Python处理PDF文档的知识,Python处理pdf有很多第三方库,这里先给大家介绍最常用的两个库 「pdfplumber」 、 「pypdf2」 。
「pdfplumber:」
pdfplumber库按页处理 pdf ,获取页面文字,提取表格等操作。
学习文档: https:// github.com/jsvine/pdfpl umber
PyPDF2 是一个纯 Python PDF 库,可以读取文档信息(标题,作者等)、写入、分割、合并PDF文档,它还可以对pdf文档进行添加水印、加密解密等。
官方文档: https:// pythonhosted.org/PyPDF2
pip install pypdf2
pip install pdfplumber
「提取单页pdf文字」
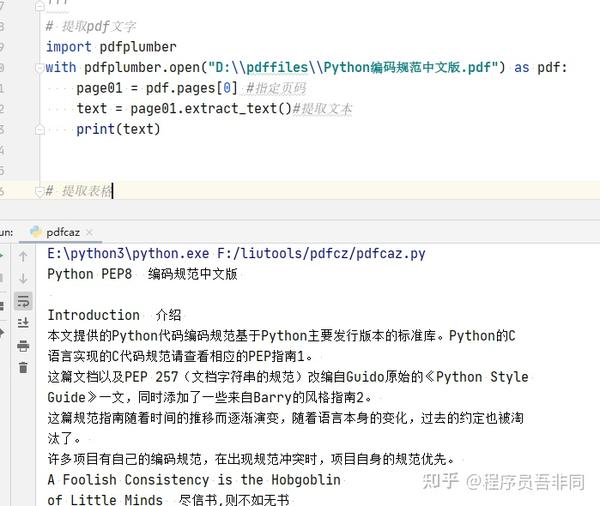
「提取所有页pdf文字」
「提取所有pdf文字并写入文本中」

「提取表格,保存为excel文件」

PyPDF2 中有两个最常用的类: PdfFileReader 和 PdfFileWriter ,分别用于读取 PDF 和写入 PDF。其中 PdfFileReader 传入参数可以是一个打开的文件对象,也可以是表示文件路径的字符串。而 PdfFileWriter 则必须传入一个以写方式打开的文件对象。
「PdfFileReader 对象的属性和方法」
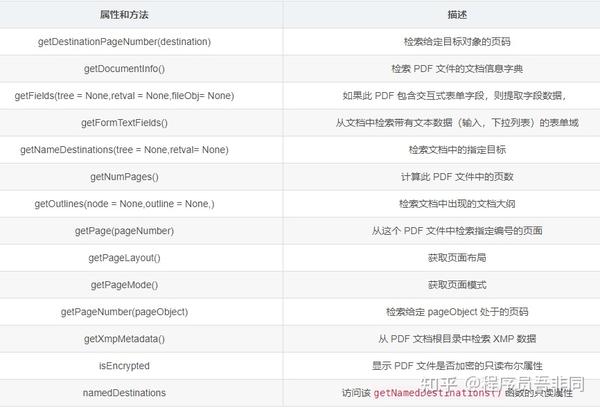
「PdfFileWriter 对象的属性和方法」
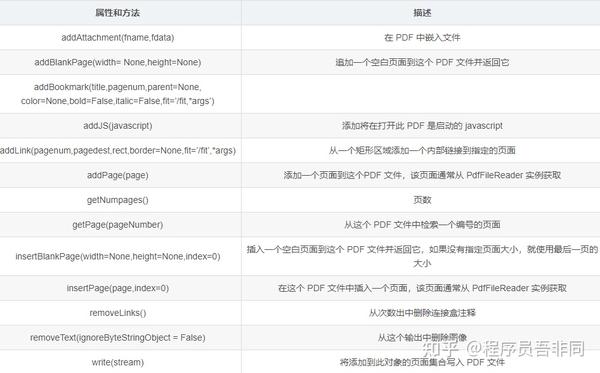
将上述分割的pdf合并成一个文件
打开文件,提示输入密码
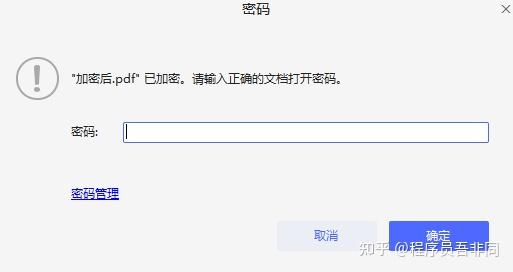
首先准备一个水印文档,可以用空白word添加图片或者文字转成pdf文件。
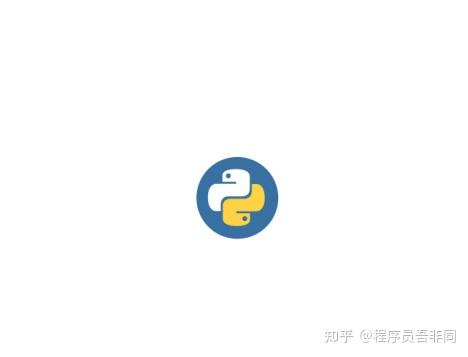
如果觉得有用,欢迎点赞、转发、收藏! 人生不止,学习不止,一路同行!
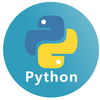
python从入门到进阶
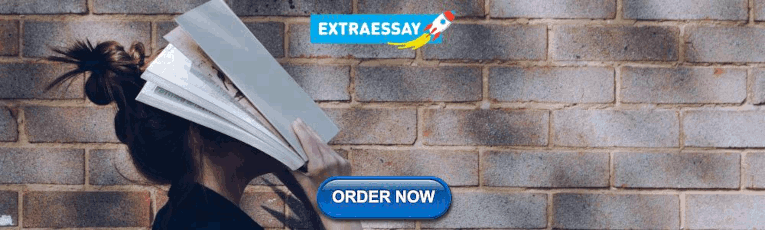
IMAGES
VIDEO
COMMENTS
II. Process Data and Design Slides with Python. You can find the source code with dummy data here: Github. Let us explore all the steps to generate your final report. Steps to create your operational report on PowerPoint — (Image by Author) 1. Data Extraction. Connect to your WMS and extract shipment records.
Here is the start of the function that we use to create our output PowerPoint: def create_ppt(input, output, report_data, chart): """ Take the input powerpoint file and use it as the template for the output file. """ prs = Presentation(input) # Use the output from analyze_ppt to understand which layouts and placeholders # to use # Create a ...
The outcome is a PowerPoint file officegen-presentation.pptx with a single slide containing a large title. This snippet uses Officegen to initiate a new presentation, adds a text title to a slide, and saves the presentation. While not as detailed as python-pptx, Officegen is quick for simple presentations. Summary/Discussion. Method 1: python-pptx.
For this example, I created a simple bar chart in Python using a public Airbnb dataset for Asheville, NC. The python-pptx can control almost every element in PowerPoint that is available when working with PowerPoint directly. It is possible to start with a PowerPoint template, an existing presentation, or create the entire presentation.
from pptx import Presentation prs = Presentation (path_to_presentation) # text_runs will be populated with a list of strings, # one for each text run in presentation text_runs = [] for slide in prs. slides: for shape in slide. shapes: if not shape. has_text_frame: continue for paragraph in shape. text_frame. paragraphs: for run in paragraph ...
Build Web Apps from Jupyter Notebook. Create interactive slides with Python in 8 Jupyter Notebook cells. Creating presentations in Jupyter Notebook is a great alternative to manually updating slides in other presentation creation software.
Project description. python-pptx is a Python library for creating, reading, and updating PowerPoint (.pptx) files. A typical use would be generating a PowerPoint presentation from dynamic content such as a database query, analytics output, or a JSON payload, perhaps in response to an HTTP request and downloading the generated PPTX file in ...
In this tutorial I will be showing you how to create POWERPOINT PRESENTATIONS using only Python. This tutorial provides a step-by-step walk-through made to h...
To use a template in Python, you'll need to load an existing presentation as a template. You can do this using the following code: template = Presentation('template.pptx') presentation = Presentation(template) This code loads the presentation template.pptx and creates a new presentation based on that template.
The Basic Structure of python-pptx. After installing the package, using python-pptx is the same as any other library. At the top of the file, import the dependencies you will need: Besides the ...
This allows content from one deck to be pasted into another and be connected with the right new slide layout: In python-pptx, these are prs.slide_layouts[0] through prs.slide_layouts[8] . However, there's no rule they have to appear in this order, it's just a convention followed by the themes provided with PowerPoint.
python-pptx¶. Release v0.6.22 (Installation)python-pptx is a Python library for creating, reading, and updating PowerPoint (.pptx) files.. A typical use would be generating a PowerPoint presentation from dynamic content such as a database query, analytics output, or a JSON payload, perhaps in response to an HTTP request and downloading the generated PPTX file in response.
Spire.Presentation for Python is a comprehensive PowerPoint compatible API designed for developers to efficiently create, modify, read, and convert PowerPoint files within Python programs. It offers a broad spectrum of functions to manipulate PowerPoint documents without any external dependencies. Spire.Presentation for Python supports a wide ...
This class provides an easy interface to create a PowerPoint presentation via python-pptx. It has methods to add slides and shapes (tables, textboxes, matplotlib figures) setting format by using layouts and stylesheets. It also has methods to move slides around, remove empty placeholders or create hyperlinks. Methods defined:
How To Use PPT Template To Generate PPT File With Python-pptx Module. 1. The Python Module python-pptx. The python-pptx is a library for Python to process ppt files. It focuses on reading and writing, cannot be exported, and has no rendering function. Before you can use the python-pptx module, you need to run the command pip3 install -i https ...
Using python-pptx. To help us automate the PowerPoint presentation report, we would use the Python package called python-pptx. It is a Python package developed to create and update PowerPoint files. To start using the package, we need to install it first with the following code. pip install python-pptx.
To upload the presentation online pass it through py.presentation_ops.upload(). In your string, use --- on a single line to seperate two slides. To put a title in your slide, put a line that starts with any number of # s. Only your first title will be appear in your slide. A title looks like: Anything that comes after the title will be put as ...
I found another use-case for the code shared by @d_bergeron, where I wanted to copy a slide from another presentation into the one I generated with python-pptx:. As an argument, I pass in the Presentation() object I created using python-pptx (prs = Presentation()).. from pptx import Presentation import copy def copy_slide_from_external_prs(prs): # copy from external presentation all objects ...
Presentations. ¶. A presentation is opened using the Presentation() function, provided directly by the pptx package: from pptx import Presentation. This function returns a Presentation object which is the root of a graph containing the components that constitute a presentation, e.g. slides, shapes, etc. All existing presentation components are ...
Python is a general purpose programming language that can be used for both programming and scripting. It was created in the 1990s by Guido van Rossum who named it after the Monty Python comedy troupe. People use Python for a variety of tasks due to its readability, object-oriented capabilities, extensive libraries, and ability to integrate with ...
Create a Python command-line application that makes requests to the Google Slides API. Objectives. Set up your environment. Install the client library. Set up the sample. Run the sample. Prerequisites. To run this quickstart, you need the following prerequisites: Python 3.10.7 or greater; The pip package management tool; A Google Cloud project ...
Python Programming (58 Slides) 93495 Views. Unlock a Vast Repository of Python Programming PPT Slides, Meticulously Curated by Our Expert Tutors and Institutes. Download Free and Enhance Your Learning!
These 10 GitHub repositories introduce you to the world of Python programming, covering basics to advanced topics, including interactive, project-based, and exercise-based learning. By exploring these repositories, you can build a strong foundation in Python, develop problem-solving skills, and work on practical projects that will help you gain ...
Python在自动化办公方面有很多实用的第三方库,可以很方便的处理word、excel、ppt、pdf文件,今天我们就学习一下Python处理PDF文档的知识,Python处理pdf有很多第三方库,这里先给大家介绍最常用的两个库 「pdfplumber」 、 「pypdf2」 。. 「pdfplumber:」. pdfplumber库按页 ...