- Java Operators
Last updated: January 8, 2024
DbSchema is a super-flexible database designer, which can take you from designing the DB with your team all the way to safely deploying the schema .
The way it does all of that is by using a design model , a database-independent image of the schema, which can be shared in a team using GIT and compared or deployed on to any database.
And, of course, it can be heavily visual, allowing you to interact with the database using diagrams, visually compose queries, explore the data, generate random data, import data or build HTML5 database reports.
>> Take a look at DBSchema
Now that the new version of REST With Spring - “REST With Spring Boot” is finally out, the current price will be available until this Friday , after which it will permanently increase by 50$
>> GET ACCESS NOW
Azure Spring Apps is a fully managed service from Microsoft (built in collaboration with VMware), focused on building and deploying Spring Boot applications on Azure Cloud without worrying about Kubernetes.
The Enterprise plan comes with some interesting features, such as commercial Spring runtime support, a 99.95% SLA and some deep discounts (up to 47%) when you are ready for production.
>> Learn more and deploy your first Spring Boot app to Azure.
And, you can participate in a very quick (1 minute) paid user research from the Java on Azure product team.
Slow MySQL query performance is all too common. Of course it is. A good way to go is, naturally, a dedicated profiler that actually understands the ins and outs of MySQL.
The Jet Profiler was built for MySQL only , so it can do things like real-time query performance, focus on most used tables or most frequent queries, quickly identify performance issues and basically help you optimize your queries.
Critically, it has very minimal impact on your server's performance, with most of the profiling work done separately - so it needs no server changes, agents or separate services.
Basically, you install the desktop application, connect to your MySQL server , hit the record button, and you'll have results within minutes:
>> Try out the Profiler
A quick guide to materially improve your tests with Junit 5:
Do JSON right with Jackson
Download the E-book
Get the most out of the Apache HTTP Client
Get Started with Apache Maven:
Get started with Spring and Spring Boot, through the reference Learn Spring course:
>> LEARN SPRING
The AI Assistant to boost Boost your productivity writing unit tests - Machinet AI .
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code. And, the AI Chat crafts code and fixes errors with ease, like a helpful sidekick.
Simplify Your Coding Journey with Machinet AI :
>> Install Machinet AI in your IntelliJ
Get non-trivial analysis (and trivial, too!) suggested right inside your IDE or Git platform so you can code smart, create more value, and stay confident when you push.
Get CodiumAI for free and become part of a community of over 280,000 developers who are already experiencing improved and quicker coding.
Write code that works the way you meant it to:
>> CodiumAI. Meaningful Code Tests for Busy Devs
Yes, Spring Security can be complex, from the more advanced functionality within the Core to the deep OAuth support in the framework.
I built the security material as two full courses - Core and OAuth , to get practical with these more complex scenarios. We explore when and how to use each feature and code through it on the backing project .
You can explore the course here:
>> Learn Spring Security
Get started with Spring Boot and with core Spring, through the Learn Spring course:
>> CHECK OUT THE COURSE
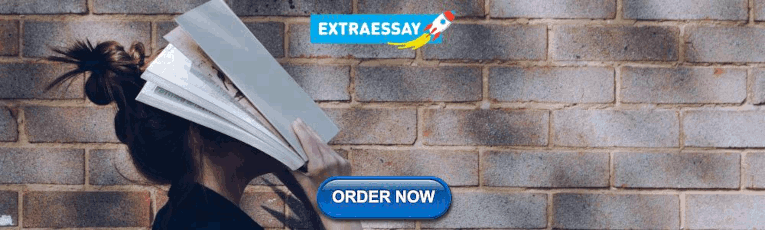
1. Overview
Operators are a fundamental building block of any programming language. We use operators to perform operations on values and variables.
Java provides many groups of operators. They are categorized by their functionalities.
In this tutorial, we’ll walk through all Java operators to understand their functionalities and how to use them.
2. Arithmetic Operators
We use arithmetic operators to perform simple mathematical operations. We should note that arithmetic operators only work with primitive number types and their boxed types , such as int and Integer .
Next, let’s see what operators we have in the arithmetic operator group.
2.1. The Addition Operator
The addition operator (+) allows us to add two values or concatenate two strings:
2.2. The Subtraction Operator
Usually, we use the subtraction operator (-) to subtract one value from another:
2.3. The Multiplication Operator
The multiplication operator (*) is used to multiply two values or variables:
2.4. The Division Operator
The division operator (/) allows us to divide the left-hand value by the right-hand one:
When we use the division operator on two integer values ( byte , short , int , and long ), we should note that the result is the quotient value. The remainder is not included .
As the example above shows, if we calculate 15 / 2 , the quotient is 7, and the remainder is 1 . Therefore, we have 15 / 2 = 7 .
2.5. The Modulo Operator
We can get the quotient using the division operator. However, if we just want to get the remainder of a division calculation, we can use the modulo operator (%):
3. Unary Operators
As the name implies, unary operators only require one single operand . For example, we usually use unary operators to increment, decrement, or negate a variable or value.
Now, let’s see the details of unary operators in Java.
3.1. The Unary Plus Operator
The unary plus operator (+) indicates a positive value. If the number is positive, we can omit the ‘+’ operator:
3.2. The Unary Minus Operator
Opposite to the unary plus operator, the unary minus operator (-) negates a value or an expression:
3.3. The Logical Complement Operator
The logical complement operator (!) is also known as the “NOT” operator . We can use it to invert the value of a boolean variable or value:
3.4. The Increment Operator
The increment operator (++) allows us to increase the value of a variable by 1:
3.5. The Decrement Opeartor
The decrement operator (–) does the opposite of the increment operator. It decreases the value of a variable by 1:
We should keep in mind that the increment and decrement operators can only be used on a variable . For example, “ int a = 5; a++; ” is fine. However, the expression “ 5++ ” won’t be compiled.
4. Relational Operators
Relational operators can be called “comparison operators” as well. Basically, we use these operators to compare two values or variables.
4.1. The “Equal To” Operator
We use the “equal to” operator (==) to compare the values on both sides. If they’re equal, the operation returns true :
The “equal to” operator is pretty straightforward. On the other hand, the Object class has provided the equals() method. As the Object class is the superclass of all Java classes, all Java objects can use the equals() method to compare each other.
When we want to compare two objects – for instance, when we compare Long objects or compare String s – we should choose between the comparison method from the equals() method and that of the “equal to” operator wisely .
4.2. The “Not Equal To” Operator
The “not equal to” operator (!=) does the opposite of the ‘==’ operator. If the values on both sides are not equal, the operation returns true :
4.3. The “Greater Than” Operator
When we compare two values with the “greater than” operator (>), it returns true if the value on the left-hand side is greater than the value on the right-hand side:
4.4. The “Greater Than or Equal To” Operator
The “greater than or equal to” operator (>=) compares the values on both sides and returns true if the left-hand side operand is greater than or equal to the right-hand side operand:
4.5. The “Less Than” Operator
The “less than” operator (<) compares two values on both sides and returns true if the value on the left-hand side is less than the value on the right-hand side:
4.6. The “Less Than or Equal To” Operator
Similarly, the “less than or equal to” operator (<=) compares the values on both sides and returns true if the left-hand side operand is less than or equal to the right-hand side:
5. Logical Operators
We have two logical operators in Java: the logical AND and OR operators. Basically, their function is pretty similar to the AND gate and the OR gate in digital electronics.
Usually, we use a logical operator with two operands, which are variables or expressions that can be evaluated as boolean .
Next, let’s take a closer look at them.
5.1. The Logical AND Operator
The logical AND operator ( && ) returns true only if both operands are true :
5.2. The Logical OR Operator
Unlike the ‘ && ‘ operator, the logical OR operator ( || ) returns true if at least one operand is true :
We should note that the logical OR operator has the short-circuiting effect : It returns true as soon as one of the operands is evaluated as true, without evaluating the remaining operands.
6. Ternary Operator
A ternary operator is a short form of the if-then-else statement. It has the name ternary as it has three operands. First, let’s have a look at the standard if-then-else statement syntax:
We can convert the above if-then-else statement into a compact version using the ternary operator:
Let’s look at its syntax:
Next, let’s understand how the ternary operator works through a simple example:
7. Bitwise and Bit Shift Operators
As the article “ Java bitwise operators ” covers the details of bitwise and bit shift operators, we’ll briefly summarize these operators in this tutorial.
7.1. The Bitwise AND Operator
The bitwise AND operator (&) returns the bit-by-bit AND of input values:
7.2. The Bitwise OR Operator
The bitwise OR operator (|) returns the bit-by-bit OR of input values:
7.3. The Bitwise XOR Operator
The bitwise XOR (exclusive OR) operator (^) returns the bit-by-bit XOR of input values:
7.4. The Bitwise Complement Operator
The bitwise complement operator (~) is a unary operator. It returns the value’s complement representation, which inverts all bits from the input value:
7.5. The Left Shift Operator
Shift operators shift the bits to the left or right by the given number of times.
The left shift operator (<<) shifts the bits to the left by the number of times defined by the right-hand side operand. After the left shift, the empty space in the right is filled with 0.
Next, let’s left shift the number 12 twice:
n << x has the same effect of multiplying the number n with x power of two.
7.6. The Signed Right Shift Operator
The signed right shift operator (>>) shifts the bits to the right by the number of times defined by the right-hand side operand and fills 0 on voids left as a result.
We should note that the leftmost position after the shifting depends on the sign extension .
Next, let’s do “signed right shift” twice on the numbers 12 and -12 to see the difference:
As the second example above shows, if the number is negative, the leftmost position after each shift will be set by the sign extension.
n >> x has the same effect of dividing the number n by x power of two.
7.7. The Unsigned Right Shift Operator
The unsigned right shift operator (>>>) works in a similar way as the ‘>>’ operator. The only difference is that after a shift, the leftmost bit is set to 0 .
Next, let’s unsigned right shift twice on the numbers 12 and -12 to see the difference:
As we can see in the second example above, the >>> operator fills voids on the left with 0 irrespective of whether the number is positive or negative .
8. The “ instanceof ” Operator
Sometimes, when we have an object, we would like to test if it’s an instance of a given type . The “ instanceof ” operator can help us to do it:
9. Assignment Operators
We use assignment operators to assign values to variables. Next, let’s see which assignment operators we can use in Java.
9.1. The Simple Assignment Operator
The simple assignment operator (=) is a straightforward but important operator in Java. Actually, we’ve used it many times in previous examples. It assigns the value on its right to the operand on its left:
9.2. Compound Assignments
We’ve learned arithmetic operators. We can combine the arithmetic operators with the simple assignment operator to create compound assignments.
For example, we can write “ a = a + 5 ” in a compound way: “ a += 5 “.
Finally, let’s walk through all supported compound assignments in Java through examples:
10. Conclusion
Java provides many groups of operators for different functionalities. In this article, we’ve passed through the operators in Java.
Slow MySQL query performance is all too common. Of course it is.
The Jet Profiler was built entirely for MySQL , so it's fine-tuned for it and does advanced everything with relaly minimal impact and no server changes.
Explore the secure, reliable, and high-performance Test Execution Cloud built for scale. Right in your IDE:
Basically, write code that works the way you meant it to.
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code.
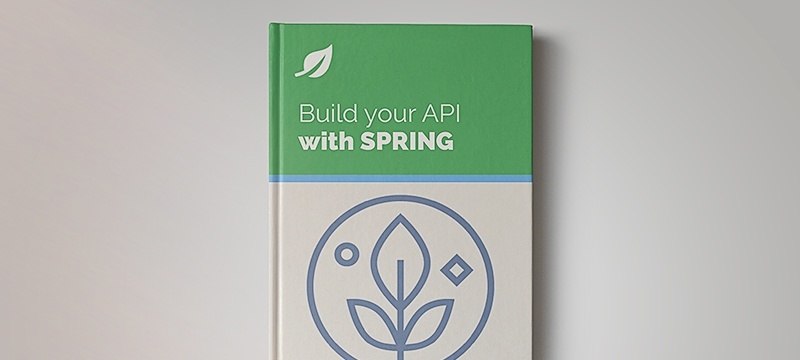
- Enterprise Java
- Web-based Java
- Data & Java
- Project Management
- Visual Basic
- Ruby / Rails
- Java Mobile
- Architecture & Design
- Open Source
- Web Services

Developer.com content and product recommendations are editorially independent. We may make money when you click on links to our partners. Learn More .

Java provides many types of operators to perform a variety of calculations and functions, such as logical , arithmetic , relational , and others. With so many operators to choose from, it helps to group them based on the type of functionality they provide. This programming tutorial will focus on Java’s numerous a ssignment operators.
Before we begin, however, you may want to bookmark our other tutorials on Java operators, which include:
- Arithmetic Operators
- Comparison Operators
- Conditional Operators
- Logical Operators
- Bitwise and Shift Operators
Assignment Operators in Java
As the name conveys, assignment operators are used to assign values to a variable using the following syntax:
The left side operand of the assignment operator must be a variable, whereas the right side operand of the assignment operator may be a literal value or another variable. Moreover, the value or variable on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. Assignment operators have a right to left associativity in that the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side variable must be declared before assignment.
You can learn more about variables in our programming tutorial: Working with Java Variables .
Types of Assignment Operators in Java
Java assignment operators are classified into two types: simple and compound .
The Simple assignment operator is the equals ( = ) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left.
Compound operators are comprised of both an arithmetic, bitwise, or shift operator in addition to the equals ( = ) sign.
Equals Operator (=) Java Example
First, let’s learn to use the one-and-only simple assignment operator – the Equals ( = ) operator – with the help of a Java program. It includes two assignments: a literal value to num1 and the num1 variable to num2 , after which both are printed to the console to show that the values have been assigned to the numbers:
The += Operator Java Example
A compound of the + and = operators, the += adds the current value of the variable on the left to the value on the right before assigning the result to the operand on the left. Here is some sample code to demonstrate how to use the += operator in Java:
The -= Operator Java Example
Made up of the – and = operators, the -= first subtracts the variable’s value on the right from the current value of the variable on the left before assigning the result to the operand on the left. We can see it at work below in the following code example showing how to decrement in Java using the -= operator:
The *= Operator Java Example
This Java operator is comprised of the * and = operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left. Here’s a program that shows the *= operator in action:
The /= Operator Java Example
A combination of the / and = operators, the /= Operator divides the current value of the variable on the left by the value on the right and then assigns the quotient to the operand on the left. Here is some example code showing how to use the /= operator in Java:
%= Operator Java Example
The %= operator includes both the % and = operators. As seen in the program below, it divides the current value of the variable on the left by the value on the right and then assigns the remainder to the operand on the left:
Compound Bitwise and Shift Operators in Java
The Bitwise and Shift Operators that we just recently covered can also be utilized in compound form as seen in the list below:
- &= – Compound bitwise Assignment operator.
- ^= – Compound bitwise ^ assignment operator.
- >>= – Compound right shift assignment operator.
- >>>= – Compound right shift filled 0 assignment operator.
- <<= – Compound left shift assignment operator.
The following program demonstrates the working of all the Compound Bitwise and Shift Operators :
Final Thoughts on Java Assignment Operators
This programming tutorial presented an overview of Java’s simple and compound assignment Operators. An essential building block to any programming language, developers would be unable to store any data in their programs without them. Though not quite as indispensable as the equals operator, compound operators are great time savers, allowing you to perform arithmetic and bitwise operations and assignment in a single line of code.
Read more Java programming tutorials and guides to software development .
Get the Free Newsletter!
Subscribe to Developer Insider for top news, trends & analysis
Latest Posts
What is the role of a project manager in software development, how to use optional in java, overview of the jad methodology, microsoft project tips and tricks, how to become a project manager in 2023, related stories, understanding types of thread synchronization errors in java, understanding memory consistency in java threads.
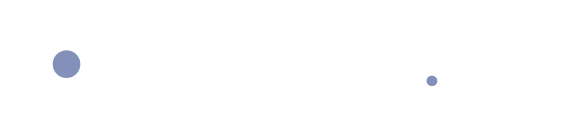
Interesting Questions about Java Operators
1. precedence and associativity:.
There is often confusion when it comes to hybrid equations which are equations having multiple operators. The problem is which part to solve first. There is a golden rule to follow in these situations. If the operators have different precedence, solve the higher precedence first. If they have the same precedence, solve according to associativity, that is, either from right to left or from left to right. The explanation of the below program is well written in comments within the program itself.
2. Be a Compiler:
The compiler in our systems uses a lex tool to match the greatest match when generating tokens. This creates a bit of a problem if overlooked. For example, consider the statement a=b+++c ; too many of the readers might seem to create a compiler error. But this statement is absolutely correct as the token created by lex is a, =, b, ++, +, c. Therefore, this statement has a similar effect of first assigning b+c to a and then incrementing b. Similarly, a=b+++++c; would generate an error as the tokens generated are a, =, b, ++, ++, +, c. which is actually an error as there is no operand after the second unary operand.
3. Using + over ():
When using the + operator inside system.out.println() make sure to do addition using parenthesis. If we write something before doing addition, then string addition takes place, that is, associativity of addition is left to right, and hence integers are added to a string first producing a string, and string objects concatenate when using +. Therefore it can create unwanted results.
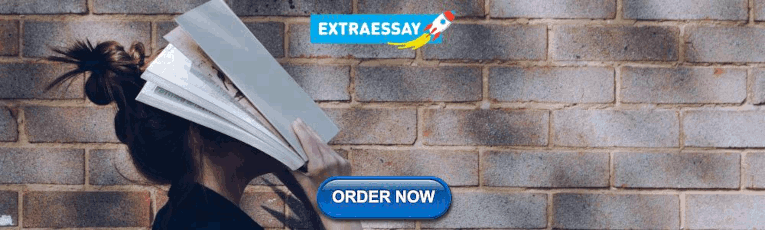
Advantages of Operators in Java
The advantages of using operators in Java are mentioned below:
- Expressiveness : Operators in Java provide a concise and readable way to perform complex calculations and logical operations.
- Time-Saving: Operators in Java save time by reducing the amount of code required to perform certain tasks.
- Improved Performance : Using operators can improve performance because they are often implemented at the hardware level, making them faster than equivalent Java code.
Disadvantages of Operators in Java
The disadvantages of Operators in Java are mentioned below:
- Operator Precedence: Operators in Java have a defined precedence, which can lead to unexpected results if not used properly.
- Type Coercion : Java performs implicit type conversions when using operators, which can lead to unexpected results or errors if not used properly.
FAQs in Java Operators
1. what is operators in java with example.
Operators are the special symbols that are used for performing certain operations. For example, ‘+’ is used for addition where 5+4 will return the value 9.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
JavaScript disabled. A lot of the features of the site won't work. Find out how to turn on JavaScript HERE .

- Fundamentals
- Objects & Classes
- OO Concepts
- API Contents
- Input & Output
- Collections
- Concurrency
- Swing & RMI
- Certification
Assignment Operators J8 Home « Assignment Operators
- << Relational & Logical Operators
- Bitwise Logical Operators >>
Symbols used for mathematical and logical manipulation that are recognized by the compiler are commonly known as operators in Java. In the third of five lessons on operators we look at the assignment operators available in Java.
Assignment Operators Overview Top
The single equal sign = is used for assignment in Java and we have been using this throughout the lessons so far. This operator is fairly self explanatory and takes the form variable = expression; . A point to note here is that the type of variable must be compatible with the type of expression .
Shorthand Assignment Operators
The shorthand assignment operators allow us to write compact code that is implemented more efficiently.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
+= | Addition | 10 | ||
-= | Subtraction | 0 | ||
/= | Division | 3 | When used with an type, any remainder will be truncated. | |
*= | Multiplication | 25 | ||
%= | Modulus | 1 | Holds the remainder value of a division. | |
&= | AND | | Will check both operands for values and assign or to the first operand dependant upon the outcome of the expression. | |
|= | OR | | Will check both operands for values and assign or to the first operand dependant upon the outcome of the expression. | |
^= | XOR | | Will check both operands for different values and assign or to the first operand dependant upon the outcome of the expression. |
Automatic Type Conversion, Assignment Rules Top
The following table shows which types can be assigned to which other types, of course we can assign to the same type so these boxes are greyed out.
When using the table use a row for the left assignment and a column for the right assignment. So in the highlighted permutations byte = int won't convert and int = byte will convert.
Type | ||||||||
---|---|---|---|---|---|---|---|---|
NO | NO | NO | NO | NO | NO | NO | ||
NO | NO | NO | NO | NO | NO | NO | ||
NO | NO | NO | NO | NO | NO | NO | ||
NO | NO | YES | NO | NO | NO | NO | ||
NO | YES | YES | YES | NO | NO | NO | ||
NO | YES | YES | YES | YES | NO | NO | ||
NO | YES | YES | YES | YES | YES | NO | ||
NO | YES | YES | YES | YES | YES | YES |
Casting Incompatible Types Top
The above table isn't the end of the story though as Java allows us to cast incompatible types. A cast instructs the compiler to convert one type to another enforcing an explicit type conversion.
A cast takes the form target = (target-type) expression .
There are a couple of things to consider when casting incompatible types:
- With narrowing conversions such as an int to a short there may be a loss of precision if the range of the int exceeds the range of a short as the high order bits will be removed.
- When casting a floating-point type to an integer type the fractional component is lost through truncation.
- The target-type can be the same type as the target or a narrowing conversion type.
- The boolean type is not only incompatible but also inconvertible with other types.
Lets look at some code to see how casting works and the affect it has on values:
Running the Casting class produces the following output:
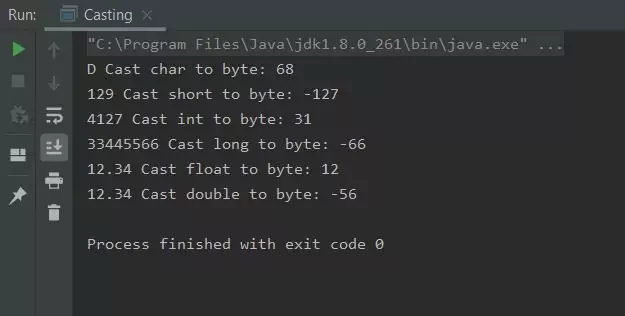
The first thing to note is we got a clean compile because of the casts, all the type conversions would fail otherwise. You might be suprised by some of the results shown in the screenshot above, for instance some of the values have become negative. Because we are truncating everything to a byte we are losing not only any fractional components and bits outside the range of a byte , but in some cases the signed bit as well. Casting can be very useful but just be aware of the implications to values when you enforce explicit type conversion.
Related Quiz
Fundamentals Quiz 8 - Assignment Operators Quiz
Lesson 9 Complete
In this lesson we looked at the assignment operators used in Java.
What's Next?
In the next lesson we look at the bitwise logical operators used in Java.
Getting Started
Code structure & syntax, java variables, primitives - boolean & char data types, primitives - numeric data types, method scope, arithmetic operators, relational & logical operators, assignment operators, assignment operators overview, automatic type conversion, casting incompatible types, bitwise logical operators, bitwise shift operators, if construct, switch construct, for construct, while construct.
Java 8 Tutorials
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
Java Operator Precedence
Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
- Java Math IEEEremainder()
Operators are symbols that perform operations on variables and values. For example, + is an operator used for addition, while * is also an operator used for multiplication.
Operators in Java can be classified into 5 types:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Bitwise Operators
1. Java Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations on variables and data. For example,
Here, the + operator is used to add two variables a and b . Similarly, there are various other arithmetic operators in Java.
Operator | Operation |
Addition | |
Subtraction | |
Multiplication | |
Division | |
Modulo Operation (Remainder after division) |
Example 1: Arithmetic Operators
In the above example, we have used + , - , and * operators to compute addition, subtraction, and multiplication operations.
/ Division Operator
Note the operation, a / b in our program. The / operator is the division operator.
If we use the division operator with two integers, then the resulting quotient will also be an integer. And, if one of the operands is a floating-point number, we will get the result will also be in floating-point.
% Modulo Operator
The modulo operator % computes the remainder. When a = 7 is divided by b = 4 , the remainder is 3 .
Note : The % operator is mainly used with integers.
2. Java Assignment Operators
Assignment operators are used in Java to assign values to variables. For example,
Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age .
Let's see some more assignment operators available in Java.
Operator | Example | Equivalent to |
---|---|---|
Example 2: Assignment Operators
3. java relational operators.
Relational operators are used to check the relationship between two operands. For example,
Here, < operator is the relational operator. It checks if a is less than b or not.
It returns either true or false .
Operator | Description | Example |
---|---|---|
Is Equal To | returns | |
Not Equal To | returns | |
Greater Than | returns | |
Less Than | returns | |
Greater Than or Equal To | returns | |
Less Than or Equal To | returns |
Example 3: Relational Operators
Note : Relational operators are used in decision making and loops.
4. Java Logical Operators
Logical operators are used to check whether an expression is true or false . They are used in decision making.
Operator | Example | Meaning |
---|---|---|
(Logical AND) | expression1 expression2 | only if both and are |
(Logical OR) | expression1 expression2 | if either or is |
(Logical NOT) | expression | if is and vice versa |
Example 4: Logical Operators
Working of Program
- (5 > 3) && (8 > 5) returns true because both (5 > 3) and (8 > 5) are true .
- (5 > 3) && (8 < 5) returns false because the expression (8 < 5) is false .
- (5 < 3) || (8 > 5) returns true because the expression (8 > 5) is true .
- (5 > 3) || (8 < 5) returns true because the expression (5 > 3) is true .
- (5 < 3) || (8 < 5) returns false because both (5 < 3) and (8 < 5) are false .
- !(5 == 3) returns true because 5 == 3 is false .
- !(5 > 3) returns false because 5 > 3 is true .
5. Java Unary Operators
Unary operators are used with only one operand. For example, ++ is a unary operator that increases the value of a variable by 1 . That is, ++5 will return 6 .
Different types of unary operators are:
Operator | Meaning |
---|---|
: not necessary to use since numbers are positive without using it | |
: inverts the sign of an expression | |
: increments value by 1 | |
: decrements value by 1 | |
: inverts the value of a boolean |
- Increment and Decrement Operators
Java also provides increment and decrement operators: ++ and -- respectively. ++ increases the value of the operand by 1 , while -- decrease it by 1 . For example,
Here, the value of num gets increased to 6 from its initial value of 5 .
Example 5: Increment and Decrement Operators
In the above program, we have used the ++ and -- operator as prefixes (++a, --b) . We can also use these operators as postfix (a++, b++) .
There is a slight difference when these operators are used as prefix versus when they are used as a postfix.
To learn more about these operators, visit increment and decrement operators .
6. Java Bitwise Operators
Bitwise operators in Java are used to perform operations on individual bits. For example,
Here, ~ is a bitwise operator. It inverts the value of each bit ( 0 to 1 and 1 to 0 ).
The various bitwise operators present in Java are:
Operator | Description |
---|---|
Bitwise Complement | |
Left Shift | |
Right Shift | |
Unsigned Right Shift | |
Bitwise AND | |
Bitwise exclusive OR |
These operators are not generally used in Java. To learn more, visit Java Bitwise and Bit Shift Operators .
Other operators
Besides these operators, there are other additional operators in Java.
The instanceof operator checks whether an object is an instanceof a particular class. For example,
Here, str is an instance of the String class. Hence, the instanceof operator returns true . To learn more, visit Java instanceof .
The ternary operator (conditional operator) is shorthand for the if-then-else statement. For example,
Here's how it works.
- If the Expression is true , expression1 is assigned to the variable .
- If the Expression is false , expression2 is assigned to the variable .
Let's see an example of a ternary operator.
In the above example, we have used the ternary operator to check if the year is a leap year or not. To learn more, visit the Java ternary operator .
Now that you know about Java operators, it's time to know about the order in which operators are evaluated. To learn more, visit Java Operator Precedence .
Table of Contents
- Introduction
- Java Arithmetic Operators
- Java Assignment Operators
- Java Relational Operators
- Java Logical Operators
- Java Unary Operators
- Java Bitwise Operators
Sorry about that.
Related Tutorials
Java Tutorial
Java Tutorial
Java methods, java classes, java file handling, java how to's, java reference, java examples, java booleans.
Very often, in programming, you will need a data type that can only have one of two values, like:
- TRUE / FALSE
For this, Java has a boolean data type, which can store true or false values.
Boolean Values
A boolean type is declared with the boolean keyword and can only take the values true or false :
Try it Yourself »
However, it is more common to return boolean values from boolean expressions, for conditional testing (see below).
Boolean Expression
A Boolean expression returns a boolean value: true or false .
This is useful to build logic, and find answers.
For example, you can use a comparison operator , such as the greater than ( > ) operator, to find out if an expression (or a variable) is true or false:
Or even easier:
In the examples below, we use the equal to ( == ) operator to evaluate an expression:
Real Life Example
Let's think of a "real life example" where we need to find out if a person is old enough to vote.
In the example below, we use the >= comparison operator to find out if the age ( 25 ) is greater than OR equal to the voting age limit, which is set to 18 :
Cool, right? An even better approach (since we are on a roll now), would be to wrap the code above in an if...else statement, so we can perform different actions depending on the result:
Output "Old enough to vote!" if myAge is greater than or equal to 18 . Otherwise output "Not old enough to vote.":
Booleans are the basis for all Java comparisons and conditions.
You will learn more about conditions ( if...else ) in the next chapter.
Test Yourself With Exercises
Fill in the missing parts to print the values true and false :
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Summary of Operators
The following quick reference summarizes the operators supported by the Java programming language.
Simple Assignment Operator
Arithmetic operators, unary operators, equality and relational operators, conditional operators, type comparison operator, bitwise and bit shift operators.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Java operator
last modified January 27, 2024
Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of the operators.
Certain operators may be used in different contexts. For example the + operator. It can be used in different cases. It adds numbers, concatenates strings, or indicates the sign of a number. We say that the operator is overloaded .
Java sign operators
There are two sign operators: + and - . They are used to indicate or change the sign of a value.
The + and - signs indicate the sign of a value. The plus sign can be used to signal that we have a positive number. It can be omitted and it is mostly done so.
Java assignment operator
The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one.
Here we assign a number to the x variable.
This code line results in syntax error. We cannot assign a value to a literal.
Java concatenating strings
In Java the + operator is also used to concatenate strings.
An alternative method for concatenating strings is the concat method.
Java increment and decrement operators
Incrementing or decrementing a value by one is a common task in programming. Java has two convenient operators for this: ++ and -- .
In the above example, we demonstrate the usage of both operators.
And here is the output of the example.
Java arithmetic operators
Symbol | Name |
---|---|
Addition | |
Subtraction | |
Multiplication | |
Division | |
Remainder |
The following example shows arithmetic operations.
Java Boolean operators
Symbol | Name |
---|---|
logical and | |
logical or | |
negation |
Relational operators always result in a boolean value. These two lines print false and true.
The true and false keywords represent boolean literals in Java.
The code example shows the logical and (&&) operator. It evaluates to true only if both operands are true.
The example shows the negation operator in action.
The One method returns false. The short circuit && does not evaluate the second method. It is not necessary. Once an operand is false, the result of the logical conclusion is always false. Only "Inside one" is only printed to the console.
Java relational operators
Relational operators are used to compare values. These operators always result in a boolean value.
Symbol | Meaning |
---|---|
less than | |
less than or equal to | |
greater than | |
greater than or equal to | |
equal to | |
not equal to |
Relational operators are also called comparison operators.
Java bitwise operators
Decimal numbers are natural to humans. Binary numbers are native to computers. Binary, octal, decimal, or hexadecimal symbols are only notations of a number. Bitwise operators work with bits of a binary number. Bitwise operators are seldom used in higher level languages like Java.
Symbol | Meaning |
---|---|
bitwise negation | |
bitwise exclusive or | |
bitwise and | |
bitwise or |
The bitwise negation operator changes each 1 to 0 and 0 to 1.
The operator reverts all bits of a number 7. One of the bits also determines whether the number is negative or not. If we negate all the bits one more time, we get number 7 again.
The first number is a binary notation of 6, the second is 3 and the result is 2.
The result is 00101 or decimal 5.
Java compound assignment operators
We use the += and *= compound operators.
The a variable is initiated to one. Value 1 is added to the variable using the non-shorthand notation.
Java instanceof operator
This line checks if the variable d points to the class that is an instance of the Base class. Since the Derived class inherits from the Base class, it is also an instance of the Base class too. The line prints true.
Java lambda operator
Java 8 introduced the lambda operator ( -> ).
In the example, we define an array of strings. The array is sorted using the Arrays.sort method and a lambda expression.
In the example, we create a greeting service with the help of a lambda expression.
Java double colon operator
In the code example, we create a reference to a static method with the double colon operator.
We perform the functional operation with the accept method.
Java operator precedence
The operator precedence tells us which operators are evaluated first. The precedence level is necessary to avoid ambiguity in expressions.
To change the order of evaluation, we can use parentheses. Expressions inside parentheses are always evaluated first. The result of the above expression is 40.
Java operators precedence list
Operator | Meaning | Associativity |
---|---|---|
array access, method invoke, object member access | Left-to-right | |
increment, decrement, unary plus and minus | Right-to-left | |
negation, bitwise NOT, type cast, object creation | Right-to-left | |
multiplication, division, modulo | Left-to-right | |
addition, subtraction | Left-to-right | |
string concatenation | Left-to-right | |
shift | Left-to-right | |
relational | Left-to-right | |
type comparison | Left-to-right | |
equality | Left-to-right | |
bitwise AND | Left-to-right | |
bitwise XOR | Left-to-right | |
bitwise OR | Left-to-right | |
logical AND | Left-to-right | |
logical OR | Left-to-right | |
ternary | Right-to-left | |
simple assignment | Right-to-left | |
compound assignment | Right-to-left | |
compound assignment | Right-to-left |
Java associativity rule
What is the outcome of this expression, 9 or 1? The multiplication, deletion, and the modulo operator are left to right associated. So the expression is evaluated this way: (9 / 3) * 3 and the result is 9.
The compound assignment operators are right to left associated. We might expect the result to be 1. But the actual result is 0. Because of the associativity. The expression on the right is evaluated first and then the compound assignment operator is applied.
Java ternary operator
Calculating prime numbers.
In the above example, we deal with several operators. A prime number (or a prime) is a natural number that has exactly two distinct natural number divisors: 1 and itself. We pick up a number and divide it by numbers from 1 to the selected number. Actually, we do not have to try all smaller numbers; we can divide by numbers up to the square root of the chosen number. The formula will work. We use the remainder division operator.
We will calculate primes from these numbers.
We are OK if we only try numbers smaller than the square root of a number in question.
In this article we covered Java expressions. We mentioned various types of operators and described precedence and associativity rules in expressions.
Java - Compound Boolean Logical Assignment Operators
What are compound boolean logical assignment operators.
There are three compound Boolean logical assignment operators.
The operand1 must be a boolean variable and op may be &, |, or ^.
Java does not have any operators like &&= and ||=.
Compound Boolean Logical Assignment Operators are used in the form
The above form is equivalent to writing
The following table lists the compound logical assignment operators and their equivalents.
Expression | is equivalent to |
---|---|
operand1 &= operand2 | operand1 = operand1 & operand2 |
operand1 |= operand2 | operand1 = operand1 | operand2 |
operand1 ^= operand2 | operand1 = operand1 ^ operand2 |
For &= operator, if both operands evaluate to true, &= returns true. Otherwise, it returns false.
For != operator, if either operand evaluates to true, != returns true. Otherwise, it returns false.
For ^= operator, if both operands evaluate to different values, that is, one of the operands is true but not both, ^= returns true. Otherwise, it returns false.
Free Java Guide
- Programming Tutorials
- History of Java
- Free JAVA Tutorials
- SCJP Notes 1
- SCJP Notes 2
- SCJP Notes 3
- SCJP Notes 4
- SCJP Notes 5
- SCJP Notes 6
- SCJP Notes 7
- SCJP Notes 8
- JDBC Program Tutorial
- Java Source Code
- Java Applet
- Java Servlet
- JavaServer Pages
- Criticism of Java
- SQL Tutorial
- PLSQL Tutorial
- PLSQL Examples
- HTML Tutorial
- What is XML?
- XML Tutorial
- Job Interview Questions
- Java Interview
- SQL Interview
- XML Interview
- HTML Interview
- Partner websites
- Bird Watching
- Haryana Online
- Asia Newscast
- North India Online
These tutorials will introduce you to Java programming Language. You'll compile and run your own Java application, using Sun's JDK. It's very easy to learn java programming skills, and in these parts, you'll learn how to write, compile, and run Java applications. Before you can develop corejava applications, you'll need to download the Java Development Kit ( JDK ).
- Java Arithmetic Operators
- Java Assignment Operators
- Java Increment and Decrement Operators
- Java Relational Operators
Java Boolean Operators
- Java Conditional Operators
The Boolean logical operators are : | , & , ^ , ! , || , && , == , != . Java supplies a primitive data type called Boolean, instances of which can take the value true or false only, and have the default value false. The major use of Boolean facilities is to implement the expressions which control if decisions and while loops. These operators act on Boolean operands according to this table
Example
Previous 1 2 3 4 5 Next
Page 2 of 5
Copyright © 2006-2013 Free Java Guide & Tutorials . All Rights Reserved.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Comma operator (,)
The comma ( , ) operator evaluates each of its operands (from left to right) and returns the value of the last operand. This is commonly used to provide multiple updaters to a for loop's afterthought.
One or more expressions, the last of which is returned as the value of the compound expression.
Description
You can use the comma operator when you want to include multiple expressions in a location that requires a single expression. The most common usage of this operator is to supply multiple updaters in a for loop.
Because all expressions except the last are evaluated and then discarded, these expressions must have side effects to be useful. Common expressions that have side effects are assignments, function calls, and ++ and -- operators. Others may also have side effects if they invoke getters or trigger type coercions .
The comma operator has the lowest precedence of all operators. If you want to incorporate a comma-joined expression into a bigger expression, you must parenthesize it.
The comma operator is completely different from commas used as syntactic separators in other locations, which include:
- Elements in array initializers ( [1, 2, 3] )
- Properties in object initializers ( { a: 1, b: 2 } )
- Parameters in function declarations /expressions ( function f(a, b) { … } )
- Arguments in function calls ( f(1, 2) )
- Binding lists in let , const , or var declarations ( const a = 1, b = 2; )
- Import lists in import declarations ( import { a, b } from "c"; )
- Export lists in export declarations ( export { a, b }; )
In fact, although some of these places accept almost all expressions, they don't accept comma-joined expressions because that would be ambiguous with the syntactic comma separators. In this case, you must parenthesize the comma-joined expression. For example, the following is a const declaration that declares two variables, where the comma is not the comma operator:
It is different from the following, where b = 2 is an assignment expression , not a declaration. The value of a is 2 , the return value of the assignment, while the value of 1 is discarded:
Comma operators cannot appear as trailing commas .
Using the comma operator in a for loop
If a is a 2-dimensional array with 10 elements on each side, the following code uses the comma operator to increment i and decrement j at once, thus printing the values of the diagonal elements in the array:
Using the comma operator to join assignments
Because commas have the lowest precedence — even lower than assignment — commas can be used to join multiple assignment expressions. In the following example, a is set to the value of b = 3 (which is 3). Then, the c = 4 expression evaluates and its result becomes the return value of the entire comma expression.
Processing and then returning
Another example that one could make with the comma operator is processing before returning. As stated, only the last element will be returned but all others are going to be evaluated as well. So, one could do:
This is especially useful for one-line arrow functions . The following example uses a single map() to get both the sum of an array and the squares of its elements, which would otherwise require two iterations, one with reduce() and one with map() :
Discarding reference binding
The comma operator always returns the last expression as a value instead of a reference . This causes some contextual information such as the this binding to be lost. For example, a property access returns a reference to the function, which also remembers the object that it's accessed on, so that calling the property works properly. If the method is returned from a comma expression, then the function is called as if it's a new function value, and this is undefined .
You can enter indirect eval with this technique, because direct eval requires the function call to happen on the reference to the eval() function.
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Boolean assign with OR operator
Suppose that i have the following code:
the final value of j will be 2
z in the 1st assign, will have true or false , which is true
z in the 2nd assign, will have false or true , which is true
why the final value is 2? what is the difference between having true || false and false||true ?
I am not asking about "short-circuit" operators,
I just need more explanation for the assign operator, and how the first j didn't changed the value of j and the 2nd did.

- 4 Possible duplicate of Java logical operator short-circuiting – user6073886 Commented Nov 21, 2017 at 12:45
- 4 j++ is not evaluated since x is true. – Eran Commented Nov 21, 2017 at 12:45
the || operator validates from left to right.
In your case, the first condition true || false , once || finds true , it does not have to check for the other condition as the result is going to be true regardless. Thats the reason here x||((j++)==0) once x = true if figured, the next statement (j++==0) is skipped.
the second condition false || true , once || finds false , it have to check for next condition.
Ref > https://docs.oracle.com/javase/tutorial/java/nutsandbolts/op2.html
The && and || operators perform Conditional-AND and Conditional-OR operations on two boolean expressions. These operators exhibit "short-circuiting" behavior, which means that the second operand is evaluated only if needed.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged java or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- The return of Staging Ground to Stack Overflow
- Policy: Generative AI (e.g., ChatGPT) is banned
Hot Network Questions
- How can I create a quantum fluctuation simulation?
- Solve the differential equation that define exp(x)
- Visiting every digit
- I did not contest a wrong but inconsequential accusation of plagiarism – can this come back to haunt me?
- The connection between determinants and eigenvalues
- What is the proper way to terminate unused electrical wires?
- What does "the dogs of prescriptivism" mean?
- Longest checkmates in (almost) fully covered boards?
- My 5-year-old is stealing food and lying
- Delimiter sizes with unicode-math
- Reaching max stay on multi entry Schengen visa
- How to repair a "pull" shut-off valve for the toilet?
- Advice for job application to a university position as a non-student
- Looking for a caveman discovers fire short story that is a pun on "nuclear" power
- NaN is not equal to NaN
- Why we can not recharge alkaline batteries to their former voltage values
- Is teaching how to solve recurrence relations using generating functions too much for a first year discrete maths course?
- Can a contract require you to accept new T&C?
- Can I race if I cannot or no longer ride a sit up cycle?
- What would cause desoldering braid to simply not work?
- Finding reflexive, transitive closure
- What is a Very Frighteningly Dangerous Word™?
- how to format a text file in bash with dots from right
- The method of substitution in the problem of finding the integral
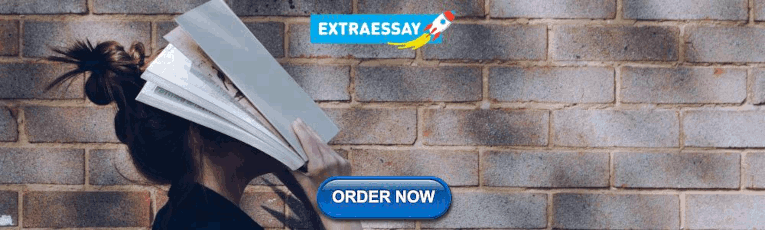
COMMENTS
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
Java Comparison Operators. Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions. The return value of a comparison is either true or false. These values are known as Boolean values, and you will learn more about them in the Booleans and If ...
The |= is a compound assignment operator ( JLS 15.26.2) for the boolean logical operator | ( JLS 15.22.2 ); not to be confused with the conditional-or || ( JLS 15.24 ). There are also &= and ^= corresponding to the compound assignment version of the boolean logical & and ^ respectively. In other words, for boolean b1, b2, these two are ...
Learning the operators of the Java programming language is a good place to start. Operators are special symbols that perform specific operations on one, two, or three operands, and then return a result. As we explore the operators of the Java programming language, it may be helpful for you to know ahead of time which operators have the highest ...
The Arithmetic Operators. The Java programming language provides operators that perform addition, subtraction, multiplication, and division. ... You can also combine the arithmetic operators with the simple assignment operator to create compound assignments. For ... negating an expression, or inverting the value of a boolean. Operator ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Basically, we use these operators to compare two values or variables. 4.1. The "Equal To" Operator. We use the "equal to" operator (==) to compare the values on both sides. If they're equal, the operation returns true: int number1 = 5 ; int number2 = 5 ; boolean theyAreEqual = number1 == number2; Copy.
Java assignment operators are classified into two types: simple and compound. The Simple assignment operator is the equals ( =) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left. Compound operators are comprised of both an arithmetic, bitwise, or shift operator ...
Logical operators are commonly used in conditional statements ( if, else, while, do-while, etc.) and boolean expressions to control the flow of the program based on certain conditions. They allow for the creation of complex conditions by combining simpler conditions. Previous - Operators Assignment Operators.
3. Assignment Operator '=' Assignment operator is used to assign a value to any variable. It has right-to-left associativity, i.e. value given on the right-hand side of the operator is assigned to the variable on the left, and therefore right-hand side value must be declared before using it or should be a constant.
Assignment Operators Overview Top. The single equal sign = is used for assignment in Java and we have been using this throughout the lessons so far. This operator is fairly self explanatory and takes the form variable = expression; . A point to note here is that the type of variable must be compatible with the type of expression.
2. Java Assignment Operators. Assignment operators are used in Java to assign values to variables. For example, int age; age = 5; Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age. Let's see some more assignment operators available in Java.
Boolean Expression. A Boolean expression returns a boolean value: true or false. This is useful to build logic, and find answers. For example, you can use a comparison operator, such as the greater than (>) operator, to find out if an expression (or a variable) is true or false:
The following quick reference summarizes the operators supported by the Java programming language. Simple Assignment Operator = Simple assignment operator Arithmetic Operators ... decrements a value by 1 ! Logical complement operator; inverts the value of a boolean Equality and Relational Operators ...
Java also supports a number of Boolean, string, and assignment operators. Boolean operators are used to perform logical comparisons, and always result in one of two values: true or false. Following are the most commonly used Boolean operators :
The operators are used to process data. An operand is one of the inputs (arguments) of an operator. Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of ...
There are three compound Boolean logical assignment operators. The operand1 must be a boolean variable and op may be &, |, or ^. Java does not have any operators like &&= and ||=. Compound Boolean Logical Assignment Operators are used in the form. The above form is equivalent to writing. The following table lists the compound logical assignment ...
The Boolean logical operators are : | , & , ^ , ! , || , && , == , != . Java supplies a primitive data type called Boolean, instances of which can take the value true or false only, and have the default value false. The major use of Boolean facilities is to implement the expressions which control if decisions and while loops. & the AND operator.
Boolean value assignment in Java. 28. Java boolean |= operator. 43. How Does The Bitwise & (AND) Work In Java? 2. Need help understanding this line. 3. Bitwise and (&) operator. 0. Unable to understand Bitwise & operator in java. Hot Network Questions Travelling to Iceland via Germany with Germany-issued Schengen visa
Common expressions that have side effects are assignments, function calls, and ++ and --operators. Others may also have side effects if they invoke getters or trigger type coercions. The comma operator has the lowest precedence of all operators. If you want to incorporate a comma-joined expression into a bigger expression, you must parenthesize it.
Boolean value assignment in Java. Ask Question Asked 11 years, 4 months ago. Modified 11 years, 4 months ago. Viewed 29k times 2 Apologies for the total noob question, but can anyone explain what's happening to the value of match after the for-each loop has finished in the following method? Attempts to compile ...
the || operator validates from left to right. In your case, the first condition true || false, once || finds true, it does not have to check for the other condition as the result is going to be true regardless. Thats the reason here x||((j++)==0) once x = true if figured, the next statement (j++==0) is skipped.. the second condition false || true, once || finds false, it have to check for next ...