
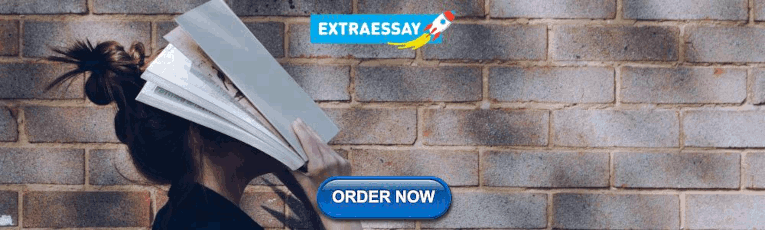
1.4 — Variable assignment and initialization
In the previous lesson ( 1.3 -- Introduction to objects and variables ), we covered how to define a variable that we can use to store values. In this lesson, we’ll explore how to actually put values into variables and use those values.
As a reminder, here’s a short snippet that first allocates a single integer variable named x , then allocates two more integer variables named y and z :
Variable assignment
After a variable has been defined, you can give it a value (in a separate statement) using the = operator . This process is called assignment , and the = operator is called the assignment operator .
By default, assignment copies the value on the right-hand side of the = operator to the variable on the left-hand side of the operator. This is called copy assignment .
Here’s an example where we use assignment twice:
This prints:
When we assign value 7 to variable width , the value 5 that was there previously is overwritten. Normal variables can only hold one value at a time.
One of the most common mistakes that new programmers make is to confuse the assignment operator ( = ) with the equality operator ( == ). Assignment ( = ) is used to assign a value to a variable. Equality ( == ) is used to test whether two operands are equal in value.
Initialization
One downside of assignment is that it requires at least two statements: one to define the variable, and another to assign the value.
These two steps can be combined. When an object is defined, you can optionally give it an initial value. The process of specifying an initial value for an object is called initialization , and the syntax used to initialize an object is called an initializer .
In the above initialization of variable width , { 5 } is the initializer, and 5 is the initial value.
Different forms of initialization
Initialization in C++ is surprisingly complex, so we’ll present a simplified view here.
There are 6 basic ways to initialize variables in C++:
You may see the above forms written with different spacing (e.g. int d{7}; ). Whether you use extra spaces for readability or not is a matter of personal preference.
Default initialization
When no initializer is provided (such as for variable a above), this is called default initialization . In most cases, default initialization performs no initialization, and leaves a variable with an indeterminate value.
We’ll discuss this case further in lesson ( 1.6 -- Uninitialized variables and undefined behavior ).
Copy initialization
When an initial value is provided after an equals sign, this is called copy initialization . This form of initialization was inherited from C.
Much like copy assignment, this copies the value on the right-hand side of the equals into the variable being created on the left-hand side. In the above snippet, variable width will be initialized with value 5 .
Copy initialization had fallen out of favor in modern C++ due to being less efficient than other forms of initialization for some complex types. However, C++17 remedied the bulk of these issues, and copy initialization is now finding new advocates. You will also find it used in older code (especially code ported from C), or by developers who simply think it looks more natural and is easier to read.
For advanced readers
Copy initialization is also used whenever values are implicitly copied or converted, such as when passing arguments to a function by value, returning from a function by value, or catching exceptions by value.
Direct initialization
When an initial value is provided inside parenthesis, this is called direct initialization .
Direct initialization was initially introduced to allow for more efficient initialization of complex objects (those with class types, which we’ll cover in a future chapter). Just like copy initialization, direct initialization had fallen out of favor in modern C++, largely due to being superseded by list initialization. However, we now know that list initialization has a few quirks of its own, and so direct initialization is once again finding use in certain cases.
Direct initialization is also used when values are explicitly cast to another type.
One of the reasons direct initialization had fallen out of favor is because it makes it hard to differentiate variables from functions. For example:
List initialization
The modern way to initialize objects in C++ is to use a form of initialization that makes use of curly braces. This is called list initialization (or uniform initialization or brace initialization ).
List initialization comes in three forms:
As an aside…
Prior to the introduction of list initialization, some types of initialization required using copy initialization, and other types of initialization required using direct initialization. List initialization was introduced to provide a more consistent initialization syntax (which is why it is sometimes called “uniform initialization”) that works in most cases.
Additionally, list initialization provides a way to initialize objects with a list of values (which is why it is called “list initialization”). We show an example of this in lesson 16.2 -- Introduction to std::vector and list constructors .
List initialization has an added benefit: “narrowing conversions” in list initialization are ill-formed. This means that if you try to brace initialize a variable using a value that the variable can not safely hold, the compiler is required to produce a diagnostic (usually an error). For example:
In the above snippet, we’re trying to assign a number (4.5) that has a fractional part (the .5 part) to an integer variable (which can only hold numbers without fractional parts).
Copy and direct initialization would simply drop the fractional part, resulting in the initialization of value 4 into variable width . Your compiler may optionally warn you about this, since losing data is rarely desired. However, with list initialization, your compiler is required to generate a diagnostic in such cases.
Conversions that can be done without potential data loss are allowed.
To summarize, list initialization is generally preferred over the other initialization forms because it works in most cases (and is therefore most consistent), it disallows narrowing conversions, and it supports initialization with lists of values (something we’ll cover in a future lesson). While you are learning, we recommend sticking with list initialization (or value initialization).
Best practice
Prefer direct list initialization (or value initialization) for initializing your variables.
Author’s note
Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) also recommend using list initialization to initialize your variables.
In modern C++, there are some cases where list initialization does not work as expected. We cover one such case in lesson 16.2 -- Introduction to std::vector and list constructors .
Because of such quirks, some experienced developers now advocate for using a mix of copy, direct, and list initialization, depending on the circumstance. Once you are familiar enough with the language to understand the nuances of each initialization type and the reasoning behind such recommendations, you can evaluate on your own whether you find these arguments persuasive.
Value initialization and zero initialization
When a variable is initialized using empty braces, value initialization takes place. In most cases, value initialization will initialize the variable to zero (or empty, if that’s more appropriate for a given type). In such cases where zeroing occurs, this is called zero initialization .
Q: When should I initialize with { 0 } vs {}?
Use an explicit initialization value if you’re actually using that value.
Use value initialization if the value is temporary and will be replaced.
Initialize your variables
Initialize your variables upon creation. You may eventually find cases where you want to ignore this advice for a specific reason (e.g. a performance critical section of code that uses a lot of variables), and that’s okay, as long as the choice is made deliberately.
Related content
For more discussion on this topic, Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) make this recommendation themselves here .
We explore what happens if you try to use a variable that doesn’t have a well-defined value in lesson 1.6 -- Uninitialized variables and undefined behavior .
Initialize your variables upon creation.
Initializing multiple variables
In the last section, we noted that it is possible to define multiple variables of the same type in a single statement by separating the names with a comma:
We also noted that best practice is to avoid this syntax altogether. However, since you may encounter other code that uses this style, it’s still useful to talk a little bit more about it, if for no other reason than to reinforce some of the reasons you should be avoiding it.
You can initialize multiple variables defined on the same line:
Unfortunately, there’s a common pitfall here that can occur when the programmer mistakenly tries to initialize both variables by using one initialization statement:
In the top statement, variable “a” will be left uninitialized, and the compiler may or may not complain. If it doesn’t, this is a great way to have your program intermittently crash or produce sporadic results. We’ll talk more about what happens if you use uninitialized variables shortly.
The best way to remember that this is wrong is to consider the case of direct initialization or brace initialization:
Because the parenthesis or braces are typically placed right next to the variable name, this makes it seem a little more clear that the value 5 is only being used to initialize variable b and d , not a or c .
Unused initialized variables warnings
Modern compilers will typically generate warnings if a variable is initialized but not used (since this is rarely desirable). And if “treat warnings as errors” is enabled, these warnings will be promoted to errors and cause the compilation to fail.
Consider the following innocent looking program:
When compiling this with the g++ compiler, the following error is generated:
and the program fails to compile.
There are a few easy ways to fix this.
- If the variable really is unused, then the easiest option is to remove the defintion of x (or comment it out). After all, if it’s not used, then removing it won’t affect anything.
- Another option is to simply use the variable somewhere:
But this requires some effort to write code that uses it, and has the downside of potentially changing your program’s behavior.
The [[maybe_unused]] attribute C++17
In some cases, neither of the above options are desirable. Consider the case where we have a set of math/physics values that we use in many different programs:
If we use these values a lot, we probably have these saved somewhere and copy/paste/import them all together.
However, in any program where we don’t use all of these values, the compiler will likely complain about each variable that isn’t actually used. While we could go through and remove/comment out the unused ones for each program, this takes time and energy. And later if we need one that we’ve previously removed, we’ll have to go back and re-add it.
To address such cases, C++17 introduced the [[maybe_unused]] attribute, which allows us to tell the compiler that we’re okay with a variable being unused. The compiler will not generate unused variable warnings for such variables.
The following program should generate no warnings/errors:
Additionally, the compiler will likely optimize these variables out of the program, so they have no performance impact.
The [[maybe_unused]] attribute should only be applied selectively to variables that have a specific and legitimate reason for being unused.
In future lessons, we’ll often define variables we don’t use again, in order to demonstrate certain concepts. Making use of [[maybe_unused]] allows us to do so without compilation warnings/errors.
Question #1
What is the difference between initialization and assignment?
Show Solution
Initialization gives a variable an initial value at the point when it is created. Assignment gives a variable a value at some point after the variable is created.
Question #2
What form of initialization should you prefer when you want to initialize a variable with a specific value?
Direct list initialization (aka. direct brace initialization).
Question #3
What are default initialization and value initialization? What is the behavior of each? Which should you prefer?
Default initialization is when a variable initialization has no initializer (e.g. int x; ). In most cases, the variable is left with an indeterminate value.
Value initialization is when a variable initialization has an empty brace (e.g. int x{}; ). In most cases this will perform zero-initialization.
You should prefer value initialization to default initialization.
Python Variables – The Complete Beginner's Guide

Variables are an essential part of Python. They allow us to easily store, manipulate, and reference data throughout our projects.
This article will give you all the understanding of Python variables you need to use them effectively in your projects.
If you want the most convenient way to review all the topics covered here, I've put together a helpful cheatsheet for you right here:
Download the Python variables cheatsheet (it takes 5 seconds).
What is a Variable in Python?
So what are variables and why do we need them?
Variables are essential for holding onto and referencing values throughout our application. By storing a value into a variable, you can reuse it as many times and in whatever way you like throughout your project.
You can think of variables as boxes with labels, where the label represents the variable name and the content of the box is the value that the variable holds.
In Python, variables are created the moment you give or assign a value to them.
How Do I Assign a Value to a Variable?
Assigning a value to a variable in Python is an easy process.
You simply use the equal sign = as an assignment operator, followed by the value you want to assign to the variable. Here's an example:
In this example, we've created two variables: country and year_founded. We've assigned the string value "United States" to the country variable and integer value 1776 to the year_founded variable.
There are two things to note in this example:
- Variables in Python are case-sensitive . In other words, watch your casing when creating variables, because Year_Founded will be a different variable than year_founded even though they include the same letters
- Variable names that use multiple words in Python should be separated with an underscore _ . For example, a variable named "site name" should be written as "site_name" . This convention is called snake case (very fitting for the "Python" language).
How Should I Name My Python Variables?
There are some rules to follow when naming Python variables.
Some of these are hard rules that must be followed, otherwise your program will not work, while others are known as conventions . This means, they are more like suggestions.
Variable naming rules
- Variable names must start with a letter or an underscore _ character.
- Variable names can only contain letters, numbers, and underscores.
- Variable names cannot contain spaces or special characters.
Variable naming conventions
- Variable names should be descriptive and not too short or too long.
- Use lowercase letters and underscores to separate words in variable names (known as "snake_case").
What Data Types Can Python Variables Hold?
One of the best features of Python is its flexibility when it comes to handling various data types.
Python variables can hold various data types, including integers, floats, strings, booleans, tuples and lists:
Integers are whole numbers, both positive and negative.
Floats are real numbers or numbers with a decimal point.
Strings are sequences of characters, namely words or sentences.
Booleans are True or False values.
Lists are ordered, mutable collections of values.
Tuples are ordered, immutable collections of values.
There are more data types in Python, but these are the most common ones you will encounter while working with Python variables.
Python is Dynamically Typed
Python is what is known as a dynamically-typed language. This means that the type of a variable can change during the execution of a program.
Another feature of dynamic typing is that it is not necessary to manually declare the type of each variable, unlike other programming languages such as Java.
You can use the type() function to determine the type of a variable. For instance:
What Operations Can Be Performed?
Variables can be used in various operations, which allows us to transform them mathematically (if they are numbers), change their string values through operations like concatenation, and compare values using equality operators.
Mathematic Operations
It's possible to perform basic mathematic operations with variables, such as addition, subtraction, multiplication, and division:
It's also possible to find the remainder of a division operation by using the modulus % operator as well as create exponents using the ** syntax:
String operators
Strings can be added to one another or concatenated using the + operator.
Equality comparisons
Values can also be compared in Python using the < , > , == , and != operators.
These operators, respectively, compare whether values are less than, greater than, equal to, or not equal to each other.
Finally, note that when performing operations with variables, you need to ensure that the types of the variables are compatible with each other.
For example, you cannot directly add a string and an integer. You would need to convert one of the variables to a compatible type using a function like str() or int() .
Variable Scope
The scope of a variable refers to the parts of a program where the variable can be accessed and modified. In Python, there are two main types of variable scope:
Global scope : Variables defined outside of any function or class have a global scope. They can be accessed and modified throughout the program, including within functions and classes.
Local scope : Variables defined within a function or class have a local scope. They can only be accessed and modified within that function or class.
In this example, attempting to access local_var outside of the function_with_local_var function results in a NameError , as the variable is not defined in the global scope.
Don't be afraid to experiment with different types of variables, operations, and scopes to truly grasp their importance and functionality. The more you work with Python variables, the more confident you'll become in applying these concepts.
Finally, if you want to fully learn all of these concepts, I've put together for you a super helpful cheatsheet that summarizes everything we've covered here.
Just click the link below to grab it for free. Enjoy!
Download the Python variables cheatsheet
Become a Professional React Developer
React is hard. You shouldn't have to figure it out yourself.
I've put everything I know about React into a single course, to help you reach your goals in record time:
Introducing: The React Bootcamp
It’s the one course I wish I had when I started learning React.
Click below to try the React Bootcamp for yourself:

Full stack developer sharing everything I know.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
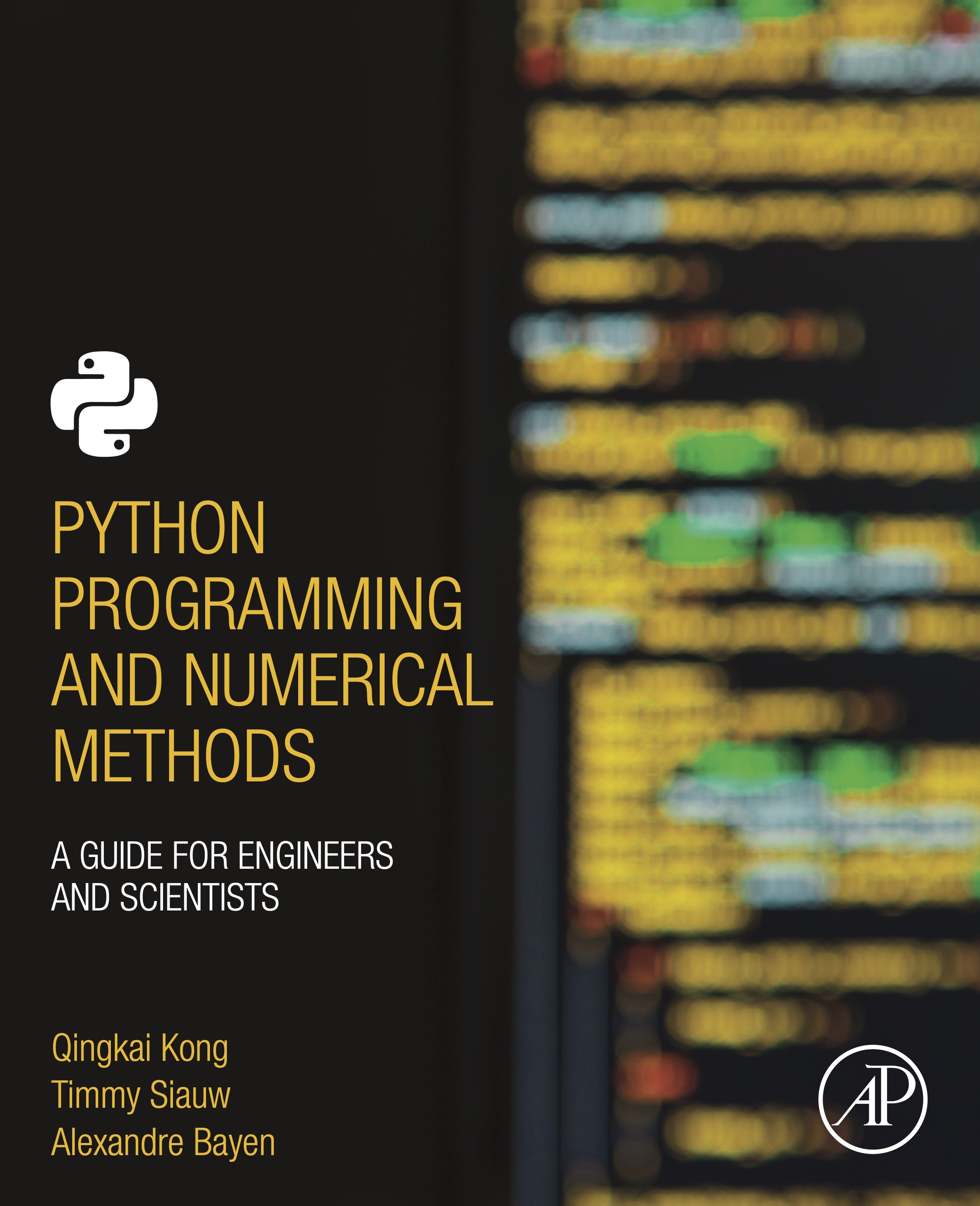
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
Computer programming - JavaScript and the web
Course: computer programming - javascript and the web > unit 1.
- Intro to Variables
- Using variables
- Challenge: Bucktooth Bunny
- More on Variables
- Challenge: Funky Frog
Review: Variables

- Variable names can begin with letters, or the symbols $ or _. They can only contain letters, numbers, $ and _. They cannot begin with a number. "myVariable", "leaf_1", and "$money3" are all examples of valid variable names.
- Variable names are case sensitive, which means that "xPos" is different from "xpos", so make sure you are consistent.
- Variable names can't be the same as existing variable names, and there are a lot in our ProcessingJS programming environment. If you ever see an error pop up like "Read only!", try changing your variable name.
- Variable names should be clear and meaningful; for example, instead of "ts", use "toothSize".
- Variable names should use camel case for multiple words, like "toothSize" instead of "toothsize" or "tooth_size".
Want to join the conversation?
- Upvote Button navigates to signup page
- Downvote Button navigates to signup page
- Flag Button navigates to signup page

A Guide to Variable Assignment and Mutation in JavaScript
Share this article
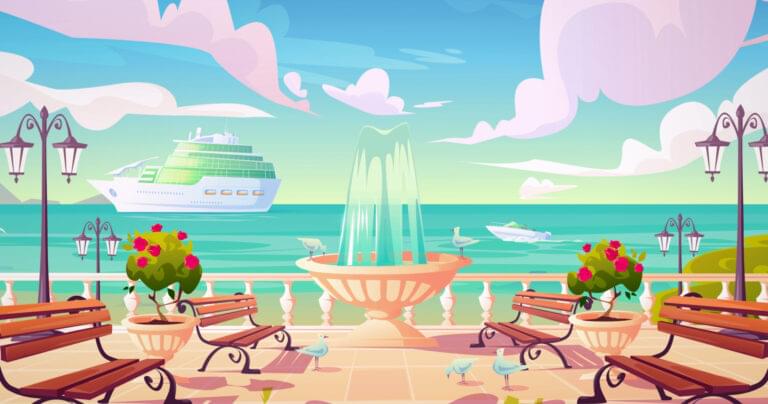
Variable Assignment
Variable reassignment, variable assignment by reference, copying by reference, the spread operator to the rescue, are mutations bad, frequently asked questions (faqs) about javascript variable assignment and mutation.
Mutations are something you hear about fairly often in the world of JavaScript, but what exactly are they, and are they as evil as they’re made out to be?
In this article, we’re going to cover the concepts of variable assignment and mutation and see why — together — they can be a real pain for developers. We’ll look at how to manage them to avoid problems, how to use as few as possible, and how to keep your code predictable.
If you’d like to explore this topic in greater detail, or get up to speed with modern JavaScript, check out the first chapter of my new book Learn to Code with JavaScript for free.
Let’s start by going back to the very basics of value types …
Every value in JavaScript is either a primitive value or an object. There are seven different primitive data types:
- numbers, such as 3 , 0 , -4 , 0.625
- strings, such as 'Hello' , "World" , `Hi` , ''
- Booleans, true and false
- symbols — a unique token that’s guaranteed never to clash with another symbol
- BigInt — for dealing with large integer values
Anything that isn’t a primitive value is an object , including arrays, dates, regular expressions and, of course, object literals. Functions are a special type of object. They are definitely objects, since they have properties and methods, but they’re also able to be called.
Variable assignment is one of the first things you learn in coding. For example, this is how we would assign the number 3 to the variable bears :
A common metaphor for variables is one of boxes with labels that have values placed inside them. The example above would be portrayed as a box containing the label “bears” with the value of 3 placed inside.
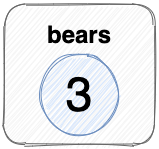
An alternative way of thinking about what happens is as a reference, that maps the label bears to the value of 3 :
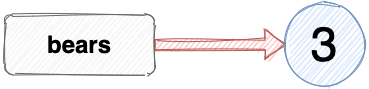
If I assign the number 3 to another variable, it’s referencing the same value as bears:
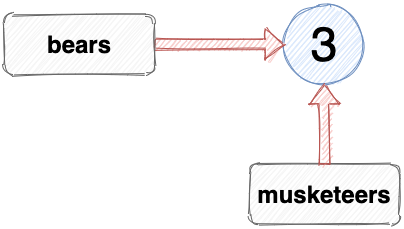
The variables bears and musketeers both reference the same primitive value of 3. We can verify this using the strict equality operator, === :
The equality operator returns true if both variables are referencing the same value.
Some gotchas when working with objects
The previous examples showed primitive values being assigned to variables. The same process is used when assigning objects:
This assignment means that the variable ghostbusters references an object:
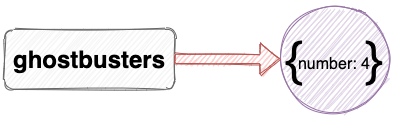
A big difference when assigning objects to variables, however, is that if you assign another object literal to another variable, it will reference a completely different object — even if both object literals look exactly the same! For example, the assignment below looks like the variable tmnt (Teenage Mutant Ninja Turtles) references the same object as the variable ghostbusters :
Even though the variables ghostbusters and tmnt look like they reference the same object, they actually both reference a completely different object, as we can see if we check with the strict equality operator:
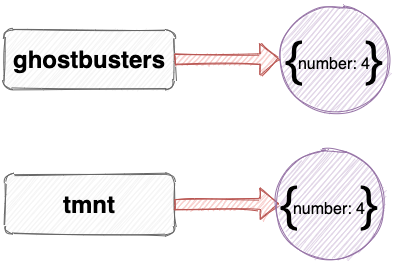
When the const keyword was introduced in ES6, many people mistakenly believed that constants had been introduced to JavaScript, but this wasn’t the case. The name of this keyword is a little misleading.
Any variable declared with const can’t be reassigned to another value. This goes for primitive values and objects. For example, the variable bears was declared using const in the previous section, so it can’t have another value assigned to it. If we try to assign the number 2 to the variable bears , we get an error:
The reference to the number 3 is fixed and the bears variable can’t be reassigned another value.
The same applies to objects. If we try to assign a different object to the variable ghostbusters , we get the same error:
Variable reassignment using let
When the keyword let is used to declare a variable, it can be reassigned to reference a different value later on in our code. For example, we declared the variable musketeers using let , so we can change the value that musketeers references. If D’Artagnan joined the Musketeers, their number would increase to 4:
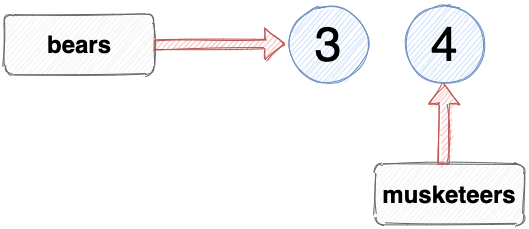
This can be done because let was used to declare the variable. We can alter the value that musketeers references as many times as we like.
The variable tmnt was also declared using let , so it can also be reassigned to reference another object (or a different type entirely if we like):
Note that the variable tmnt now references a completely different object ; we haven’t just changed the number property to 5.
In summary , if you declare a variable using const , its value can’t be reassigned and will always reference the same primitive value or object that it was originally assigned to. If you declare a variable using let , its value can be reassigned as many times as required later in the program.
Using const as often as possible is generally considered good practice, as it means that the value of variables remains constant and the code is more consistent and predictable, making it less prone to errors and bugs.
In native JavaScript, you can only assign values to variables. You can’t assign variables to reference another variable, even though it looks like you can. For example, the number of Stooges is the same as the number of Musketeers, so we can assign the variable stooges to reference the same value as the variable musketeers using the following:
This looks like the variable stooges is referencing the variable musketeers , as shown in the diagram below:
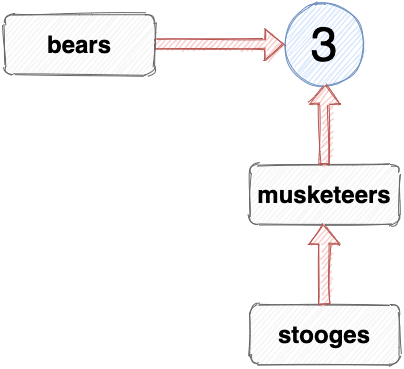
However, this is impossible in native JavaScript: a variable can only reference an actual value; it can’t reference another variable . What actually happens when you make an assignment like this is that the variable on the left of the assignment will reference the value the variable on the right references, so the variable stooges will reference the same value as the musketeers variable, which is the number 3. Once this assignment has been made, the stooges variable isn’t connected to the musketeers variable at all.
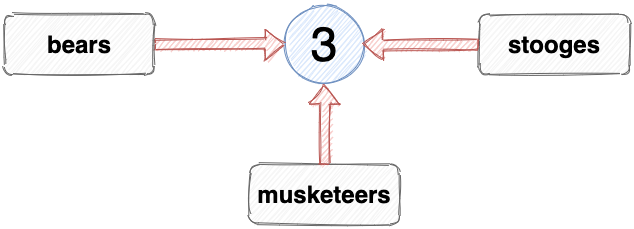
This means that if D’Artagnan joins the Musketeers and we set the value of the musketeers to 4, the value of stooges will remain as 3. In fact, because we declared the stooges variable using const , we can’t set it to any new value; it will always be 3.
In summary : if you declare a variable using const and set it to a primitive value, even via a reference to another variable, then its value can’t change. This is good for your code, as it means it will be more consistent and predictable.
A value is said to be mutable if it can be changed. That’s all there is to it: a mutation is the act of changing the properties of a value.
All primitive value in JavaScript are immutable : you can’t change their properties — ever. For example, if we assign the string "cake" to variable food , we can see that we can’t change any of its properties:
If we try to change the first letter to “f”, it looks like it has changed:
But if we take a look at the value of the variable, we see that nothing has actually changed:
The same thing happens if we try to change the length property:
Despite the return value implying that the length property has been changed, a quick check shows that it hasn’t:
Note that this has nothing to do with declaring the variable using const instead of let . If we had used let , we could set food to reference another string, but we can’t change any of its properties. It’s impossible to change any properties of primitive data types because they’re immutable .
Mutability and objects in JavaScript
Conversely, all objects in JavaScript are mutable, which means that their properties can be changed, even if they’re declared using const (remember let and const only control whether or not a variable can be reassigned and have nothing to do with mutability). For example, we can change the the first item of an array using the following code:
Note that this change still occurred, despite the fact that we declared the variable food using const . This shows that using const does not stop objects from being mutated .
We can also change the length property of an array, even if it has been declared using const :
Remember that when we assign variables to object literals, the variables will reference completely different objects, even if they look the same:
But if we assign a variable fantastic4 to another variable, they will both reference the same object:
This assigns the variable fantastic4 to reference the same object that the variable tmnt references, rather than a completely different object.
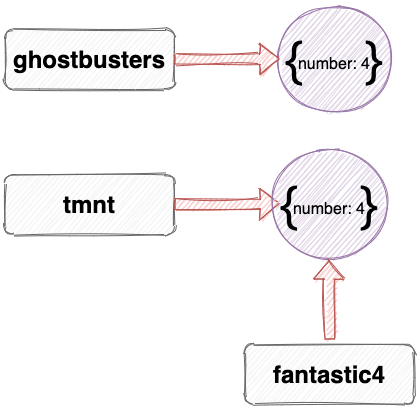
This is often referred to as copying by reference , because both variables are assigned to reference the same object.
This is important, because any mutations made to this object will be seen in both variables.
So, if Spider-Man joins The Fantastic Four, we might update the number value in the object:
This is a mutation, because we’ve changed the number property rather than setting fantastic4 to reference a new object.
This causes us a problem, because the number property of tmnt will also also change, possibly without us even realizing:
This is because both tmnt and fantastic4 are referencing the same object, so any mutations that are made to either tmnt or fantastic4 will affect both of them.
This highlights an important concept in JavaScript: when objects are copied by reference and subsequently mutated, the mutation will affect any other variables that reference that object. This can lead to unintended side effects and bugs that are difficult to track down.
So how do you make a copy of an object without creating a reference to the original object? The answer is to use the spread operator !
The spread operator was introduced for arrays and strings in ES2015 and for objects in ES2018. It allows you to easily make a shallow copy of an object without creating a reference to the original object.
The example below shows how we could set the variable fantastic4 to reference a copy of the tmnt object. This copy will be exactly the same as the tmnt object, but fantastic4 will reference a completely new object. This is done by placing the name of the variable to be copied inside an object literal with the spread operator in front of it:
What we’ve actually done here is assign the variable fantastic4 to a new object literal and then used the spread operator to copy all the enumerable properties of the object referenced by the tmnt variable. Because these properties are values, they’re copied into the fantastic4 object by value, rather than by reference.
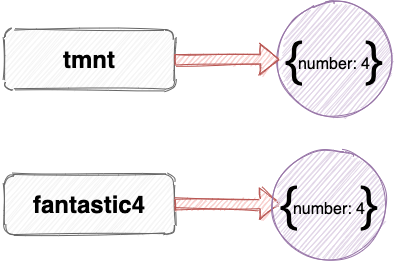
Now any changes that are made to either object won’t affect the other. For example, if we update the number property of the fantastic4 variable to 5, it won’t affect the tmnt variable:
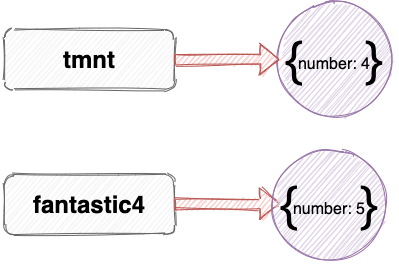
The spread operator also has a useful shortcut notation that can be used to make copies of an object and then make some changes to the new object in a single line of code.
For example, say we wanted to create an object to model the Teenage Mutant Ninja Turtles. We could create the first turtle object, and assign the variable leonardo to it:
The other turtles all have the same properties, except for the weapon and color properties, that are different for each turtle. It makes sense to make a copy of the object that leonardo references, using the spread operator, and then change the weapon and color properties, like so:
We can do this in one line by adding the properties we want to change after the reference to the spread object. Here’s the code to create new objects for the variables donatello and raphael :
Note that using the spread operator in this way only makes a shallow copy of an object. To make a deep copy, you’d have to do this recursively, or use a library. Personally, I’d advise that you try to keep your objects as shallow as possible.
In this article, we’ve covered the concepts of variable assignment and mutation and seen why — together — they can be a real pain for developers.
Mutations have a bad reputation, but they’re not necessarily bad in themselves. In fact, if you’re building a dynamic web app, it must change at some point. That’s literally the meaning of the word “dynamic”! This means that there will have to be some mutations somewhere in your code. Having said that, the fewer mutations there are, the more predictable your code will be, making it easier to maintain and less likely to develop any bugs.
A particularly toxic combination is copying by reference and mutations. This can lead to side effects and bugs that you don’t even realize have happened. If you mutate an object that’s referenced by another variable in your code, it can cause lots of problems that can be difficult to track down. The key is to try and minimize your use of mutations to the essential and keep track of which objects have been mutated.
In functional programming, a pure function is one that doesn’t cause any side effects, and mutations are one of the biggest causes of side effects.
A golden rule is to avoid copying any objects by reference. If you want to copy another object, use the spread operator and then make any mutations immediately after making the copy.
Next up, we’ll look into array mutations in JavaScript .
Don’t forget to check out my new book Learn to Code with JavaScript if you want to get up to speed with modern JavaScript. You can read the first chapter for free. And please reach out on Twitter if you have any questions or comments!
What is the difference between variable assignment and mutation in JavaScript?
In JavaScript, variable assignment refers to the process of assigning a value to a variable. For example, let x = 5; Here, we are assigning the value 5 to the variable x. On the other hand, mutation refers to the process of changing the value of an existing variable. For example, if we later write x = 10; we are mutating the variable x by changing its value from 5 to 10.
How does JavaScript handle variable assignment and mutation differently for primitive and non-primitive data types?
JavaScript treats primitive data types (like numbers, strings, and booleans) and non-primitive data types (like objects and arrays) differently when it comes to variable assignment and mutation. For primitive data types, when you assign a variable, a copy of the value is created and stored in a new memory location. However, for non-primitive data types, when you assign a variable, both variables point to the same memory location. Therefore, if you mutate one variable, the change is reflected in all variables that point to that memory location.
What is the concept of pass-by-value and pass-by-reference in JavaScript?
Pass-by-value and pass-by-reference are two ways that JavaScript can pass variables to a function. When JavaScript passes a variable by value, it creates a copy of the variable’s value and passes that copy to the function. Any changes made to the variable inside the function do not affect the original variable. However, when JavaScript passes a variable by reference, it passes a reference to the variable’s memory location. Therefore, any changes made to the variable inside the function also affect the original variable.
How can I prevent mutation in JavaScript?
There are several ways to prevent mutation in JavaScript. One way is to use the Object.freeze() method, which prevents new properties from being added to an object, existing properties from being removed, and prevents changing the enumerability, configurability, or writability of existing properties. Another way is to use the const keyword when declaring a variable. This prevents reassignment of the variable, but it does not prevent mutation of the variable’s value if the value is an object or an array.
What is the difference between shallow copy and deep copy in JavaScript?
In JavaScript, a shallow copy of an object is a copy of the object where the values of the original object and the copy point to the same memory location for non-primitive data types. Therefore, if you mutate the copy, the original object is also mutated. On the other hand, a deep copy of an object is a copy of the object where the values of the original object and the copy do not point to the same memory location. Therefore, if you mutate the copy, the original object is not mutated.
How can I create a deep copy of an object in JavaScript?
One way to create a deep copy of an object in JavaScript is to use the JSON.parse() and JSON.stringify() methods. The JSON.stringify() method converts the object into a JSON string, and the JSON.parse() method converts the JSON string back into an object. This creates a new object that is a deep copy of the original object.
What is the MutationObserver API in JavaScript?
The MutationObserver API provides developers with a way to react to changes in a DOM. It is designed to provide a general, efficient, and robust API for reacting to changes in a document.
How does JavaScript handle variable assignment and mutation in the context of closures?
In JavaScript, a closure is a function that has access to its own scope, the scope of the outer function, and the global scope. When a variable is assigned or mutated inside a closure, it can affect the value of the variable in the outer scope, depending on whether the variable was declared in the closure’s scope or the outer scope.
What is the difference between var, let, and const in JavaScript?
In JavaScript, var, let, and const are used to declare variables. var is function scoped, and if it is declared outside a function, it is globally scoped. let and const are block scoped, meaning they exist only within the block they are declared in. The difference between let and const is that let allows reassignment, while const does not.
How does JavaScript handle variable assignment and mutation in the context of asynchronous programming?
In JavaScript, asynchronous programming allows multiple things to happen at the same time. When a variable is assigned or mutated in an asynchronous function, it can lead to unexpected results if other parts of the code are relying on the value of the variable. This is because the variable assignment or mutation may not have completed before the other parts of the code run. To handle this, JavaScript provides several features, such as promises and async/await, to help manage asynchronous code.
Darren loves building web apps and coding in JavaScript, Haskell and Ruby. He is the author of Learn to Code using JavaScript , JavaScript: Novice to Ninja and Jump Start Sinatra .He is also the creator of Nanny State , a tiny alternative to React. He can be found on Twitter @daz4126.
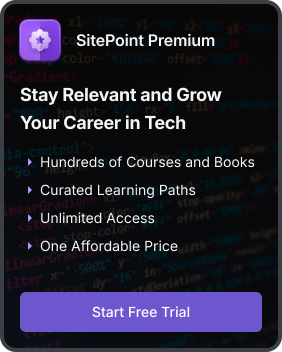
Python Variables and Assignment
Python variables, variable assignment rules, every value has a type, memory and the garbage collector, variable swap, variable names are superficial labels, assignment = is shallow, decomp by var.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Learn Programming For Free
- What is Programming? A Handbook for Beginners
- How to Learn Programming?
Basic Components of Programming
- Data Types in Programming
Variable in Programming
- Types of Operators in Programming
- Conditional Statements in Programming | Definition, Types, Best Practices
- If-Then-___ Trio in Programming
- Loops in Programming
- Functions in Programming
- Error Handling in Programming
Getting Started with Coding
- What is a Code in Programming?
- What Is Coding and What Is It Used For?
- how to learn how to code
- Most Famous Online IDE for Programming
- Getting Started with Number Programs in Programming
- Getting Started with Words and Sentences Programs in Programming
- Getting Started with Printing Patterns in Programming
- Getting Started with Geometry Problems in Programming
- Getting Started with Date and Time Problems in Programming
- Getting Started with Menu Driven Programs in Programming
Learn How to Code Popular Character Encoding Systems in Programming
- What is ASCII - A Complete Guide to Generating ASCII Code
- Morse Code Tutorial
- Program for Morse Code Translator (Conversion of Morse Code to English Text)
In programming, we often need a named storage location to store the data or values. Using variables, we can store the data in our program and access it afterward. In this article, we will learn about variables in programming, their types, declarations, initialization, naming conventions, etc.
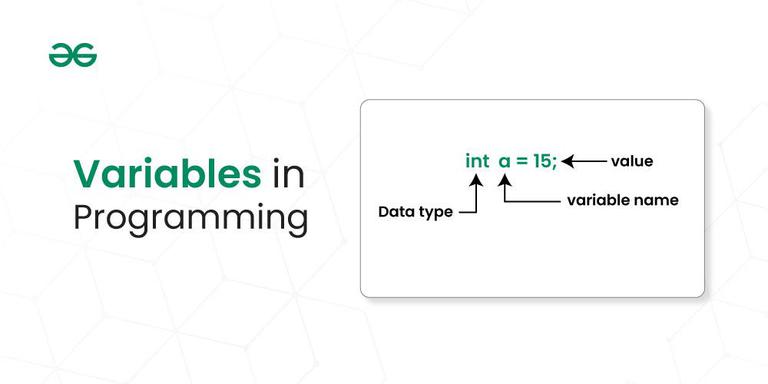
Variables in Programming
Table of Content
- What are Variables In Programming?
- Declaration of Variables In Programming
- Initialization of Variables In Programming
- Types of Variables In Programming
- Difference between Variable and Constant
- Difference between Local variables and Global Variables
- Naming Conventions
- Scope of a variable
What is a Variable in Programming?
Variable in Programming is a named storage location t hat holds a value or data. These values can change during the execution of a program, hence the term “variable.” Variables are essential for storing and manipulating data in computer programs. A variable is the basic building block of a program that can be used in expressions as a substitute in place of the value it stores.
Declaration of Variable in Programming:
In programming, the declaration of variables involves specifying the type and name of a variable before it is used in the program. The syntax can vary slightly between programming languages, but the fundamental concept remains consistent.
Initialization of Variable in Programming:
Initialization of variables In Programming involves assigning an initial value to a declared variable. The syntax for variable initialization varies across programming languages.
Types of Variable In Programming:
1. global variables:.
Global variables in programming are declared outside any function or block in a program and accessible throughout the entire codebase . In simpler words, Global variables can be accessed in any part of the program, including functions, blocks, or modules.
2. Local Variables:
Local variables in programming are declared within a specific function, block, or scope and are only accessible within that limited context . In simpler words, Local variables are confined to the block or function where they are declared and cannot be directly accessed outside that scope.
Difference between Variable and Constant :
Difference between local variables and global variables:, naming conventions:.
Naming conventions for variables In Programming help maintain code readability and consistency across programming projects. While specific conventions can vary between programming languages, some common practices are widely followed. Here are general guidelines for naming variables:
- Descriptive and Meaningful: Choose names that indicate the purpose or content of the variable. A descriptive name improves code readability and understanding.
- Camel Case or Underscore Separation: Use camel case (myVariableName) or underscores (my_variable_name) to separate words in a variable name. Choose one style and stick to it consistently.
- Avoid Single Letter Names: Except for loop counters or well-known conventions (e.g., i for an index), avoid single-letter variable names. Use names that convey the variable’s purpose.
- Follow Language Conventions: Adhere to the naming conventions recommended by the programming language you are using. For example, Java typically uses camel case (myVariableName), while Python often uses underscores (my_variable_name).
- Avoid Reserved Words: Avoid using reserved words or keywords of the programming language as variable names.
- Avoid Abbreviations : Use full words instead of abbreviations, except when widely accepted or for standard terms (e.g., max, min).
Scope of a variable:
The scope of a variable in programming refers to the region of the program where the variable can be accessed or modified. It defines the visibility and lifetime of a variable within a program. There are typically two types of variable scope:
Local Scope:
- A variable declared inside a function, block, or loop has a local scope.
- It can only be accessed within the specific function, block, or loop where it is declared.
- Its lifetime is limited to the duration of the function, block, or loop.
Global Scope:
- A variable declared outside of any function or block has a global scope.
- It can be accessed from anywhere in the program, including inside functions.
- Its lifetime extends throughout the entire program.
Understanding and managing variable scope is crucial for writing maintainable and bug-free code, as it helps avoid naming conflicts and unintended side effects.
In conclusion, variables In programming are fundamental elements in programming, serving as named storage locations to hold and manipulate data. They are crucial in writing dynamic, adaptable, and functional code. The proper use of variables, adherence to clear naming conventions, and understanding of scope contribute to code readability, maintainability, and scalability.
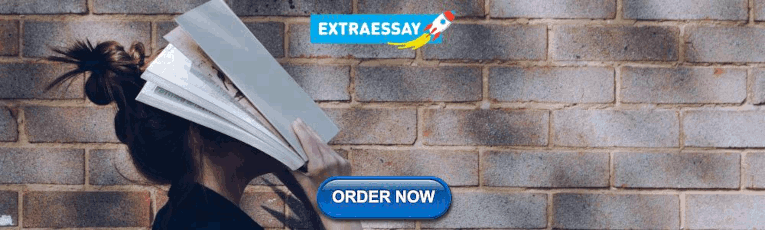
Please Login to comment...
Similar reads.
- Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
- Hands-on Python Tutorial »
- 1. Beginning With Python »
1.6. Variables and Assignment ¶
Each set-off line in this section should be tried in the Shell.
Nothing is displayed by the interpreter after this entry, so it is not clear anything happened. Something has happened. This is an assignment statement , with a variable , width , on the left. A variable is a name for a value. An assignment statement associates a variable name on the left of the equal sign with the value of an expression calculated from the right of the equal sign. Enter
Once a variable is assigned a value, the variable can be used in place of that value. The response to the expression width is the same as if its value had been entered.
The interpreter does not print a value after an assignment statement because the value of the expression on the right is not lost. It can be recovered if you like, by entering the variable name and we did above.
Try each of the following lines:
The equal sign is an unfortunate choice of symbol for assignment, since Python’s usage is not the mathematical usage of the equal sign. If the symbol ↤ had appeared on keyboards in the early 1990’s, it would probably have been used for assignment instead of =, emphasizing the asymmetry of assignment. In mathematics an equation is an assertion that both sides of the equal sign are already, in fact, equal . A Python assignment statement forces the variable on the left hand side to become associated with the value of the expression on the right side. The difference from the mathematical usage can be illustrated. Try:
so this is not equivalent in Python to width = 10 . The left hand side must be a variable, to which the assignment is made. Reversed, we get a syntax error . Try
This is, of course, nonsensical as mathematics, but it makes perfectly good sense as an assignment, with the right-hand side calculated first. Can you figure out the value that is now associated with width? Check by entering
In the assignment statement, the expression on the right is evaluated first . At that point width was associated with its original value 10, so width + 5 had the value of 10 + 5 which is 15. That value was then assigned to the variable on the left ( width again) to give it a new value. We will modify the value of variables in a similar way routinely.
Assignment and variables work equally well with strings. Try:
Try entering:
Note the different form of the error message. The earlier errors in these tutorials were syntax errors: errors in translation of the instruction. In this last case the syntax was legal, so the interpreter went on to execute the instruction. Only then did it find the error described. There are no quotes around fred , so the interpreter assumed fred was an identifier, but the name fred was not defined at the time the line was executed.
It is both easy to forget quotes where you need them for a literal string and to mistakenly put them around a variable name that should not have them!
Try in the Shell :
There fred , without the quotes, makes sense.
There are more subtleties to assignment and the idea of a variable being a “name for” a value, but we will worry about them later, in Issues with Mutable Objects . They do not come up if our variables are just numbers and strings.
Autocompletion: A handy short cut. Idle remembers all the variables you have defined at any moment. This is handy when editing. Without pressing Enter, type into the Shell just
Assuming you are following on the earlier variable entries to the Shell, you should see f autocompleted to be
This is particularly useful if you have long identifiers! You can press Alt-/ several times if more than one identifier starts with the initial sequence of characters you typed. If you press Alt-/ again you should see fred . Backspace and edit so you have fi , and then and press Alt-/ again. You should not see fred this time, since it does not start with fi .
1.6.1. Literals and Identifiers ¶
Expressions like 27 or 'hello' are called literals , coming from the fact that they literally mean exactly what they say. They are distinguished from variables, whose value is not directly determined by their name.
The sequence of characters used to form a variable name (and names for other Python entities later) is called an identifier . It identifies a Python variable or other entity.
There are some restrictions on the character sequence that make up an identifier:
The characters must all be letters, digits, or underscores _ , and must start with a letter. In particular, punctuation and blanks are not allowed.
There are some words that are reserved for special use in Python. You may not use these words as your own identifiers. They are easy to recognize in Idle, because they are automatically colored orange. For the curious, you may read the full list:
There are also identifiers that are automatically defined in Python, and that you could redefine, but you probably should not unless you really know what you are doing! When you start the editor, we will see how Idle uses color to help you know what identifies are predefined.
Python is case sensitive: The identifiers last , LAST , and LaSt are all different. Be sure to be consistent. Using the Alt-/ auto-completion shortcut in Idle helps ensure you are consistent.
What is legal is distinct from what is conventional or good practice or recommended. Meaningful names for variables are important for the humans who are looking at programs, understanding them, and revising them. That sometimes means you would like to use a name that is more than one word long, like price at opening , but blanks are illegal! One poor option is just leaving out the blanks, like priceatopening . Then it may be hard to figure out where words split. Two practical options are
- underscore separated: putting underscores (which are legal) in place of the blanks, like price_at_opening .
- using camel-case : omitting spaces and using all lowercase, except capitalizing all words after the first, like priceAtOpening
Use the choice that fits your taste (or the taste or convention of the people you are working with).
Table Of Contents
- 1.6.1. Literals and Identifiers
Previous topic
1.5. Strings, Part I
1.7. Print Function, Part I
- Show Source
Quick search
Enter search terms or a module, class or function name.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Variables (Transact-SQL)
- 14 contributors
A Transact-SQL local variable is an object that can hold a single data value of a specific type. Variables in batches and scripts are typically used:
- As a counter either to count the number of times a loop is performed, or to control how many times the loop is performed.
- To hold a data value to be tested by a control-of-flow statement.
- To save a data value to be returned by a stored procedure return code or function return value.
The names of some Transact-SQL system functions begin with two at signs ( @@ ). Although in earlier versions of SQL Server, the @@ functions are referred to as global variables, @@ functions aren't variables, and they don't have the same behaviors as variables. The @@ functions are system functions, and their syntax usage follows the rules for functions.
You can't use variables in a view.
Changes to variables aren't affected by the rollback of a transaction.
Declare a Transact-SQL variable
The DECLARE statement initializes a Transact-SQL variable by:
Assigning a name. The name must have a single @ as the first character.
Assigning a system-supplied or user-defined data type and a length. For numeric variables, a precision and scale are also assigned. For variables of type XML, an optional schema collection might be assigned.
Setting the value to NULL .
For example, the following DECLARE statement creates a local variable named @mycounter with an int data type. By default, the value for this variable is NULL .
To declare more than one local variable, use a comma after the first local variable defined, and then specify the next local variable name and data type.
For example, the following DECLARE statement creates three local variables named @LastName , @FirstName and @StateProvince , and initializes each to NULL :
The scope of a variable is the range of Transact-SQL statements that can reference the variable. The scope of a variable lasts from the point it's declared until the end of the batch or stored procedure in which it's declared. For example, the following script generates a syntax error because the variable is declared in one batch (separated by the GO keyword) and referenced in another:
Variables have local scope and are only visible within the batch or procedure where they're defined. In the following example, the nested scope created for execution of sp_executesql doesn't have access to the variable declared in the higher scope and returns and error.
Set a value in a Transact-SQL variable
When a variable is first declared, its value is set to NULL . To assign a value to a variable, use the SET statement. This is the preferred method of assigning a value to a variable. A variable can also have a value assigned by being referenced in the select list of a SELECT statement.
To assign a variable a value by using the SET statement, include the variable name and the value to assign to the variable. This is the preferred method of assigning a value to a variable. The following batch, for example, declares two variables, assigns values to them, and then uses them in the WHERE clause of a SELECT statement:
A variable can also have a value assigned by being referenced in a select list. If a variable is referenced in a select list, it should be assigned a scalar value or the SELECT statement should only return one row. For example:
If there are multiple assignment clauses in a single SELECT statement, SQL Server doesn't guarantee the order of evaluation of the expressions. Effects are only visible if there are references among the assignments.
If a SELECT statement returns more than one row and the variable references a nonscalar expression, the variable is set to the value returned for the expression in the last row of the result set. For example, in the following batch @EmpIDVariable is set to the BusinessEntityID value of the last row returned, which is 1 :
The following script creates a small test table and populates it with 26 rows. The script uses a variable to do three things:
- Control how many rows are inserted by controlling how many times the loop is executed.
- Supply the value inserted into the integer column.
- Function as part of the expression that generates letters to be inserted into the character column.
Related content
- DECLARE @local_variable (Transact-SQL)
- SET @local_variable (Transact-SQL)
- SELECT @local_variable (Transact-SQL)
- Expressions (Transact-SQL)
- Compound Operators (Transact-SQL)
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
How-To Geek
How to work with variables in bash.
Want to take your Linux command-line skills to the next level? Here's everything you need to know to start working with variables.
Hannah Stryker / How-To Geek
Quick Links
What is a variable in bash, examples of bash variables, how to use bash variables in scripts, how to use command line parameters in scripts, working with special variables, environment variables, how to export variables, how to quote variables, echo is your friend, key takeaways.
- Variables are named symbols representing strings or numeric values. They are treated as their value when used in commands and expressions.
- Variable names should be descriptive and cannot start with a number or contain spaces. They can start with an underscore and can have alphanumeric characters.
- Variables can be used to store and reference values. The value of a variable can be changed, and it can be referenced by using the dollar sign $ before the variable name.
Variables are vital if you want to write scripts and understand what that code you're about to cut and paste from the web will do to your Linux computer. We'll get you started!
Variables are named symbols that represent either a string or numeric value. When you use them in commands and expressions, they are treated as if you had typed the value they hold instead of the name of the variable.
To create a variable, you just provide a name and value for it. Your variable names should be descriptive and remind you of the value they hold. A variable name cannot start with a number, nor can it contain spaces. It can, however, start with an underscore. Apart from that, you can use any mix of upper- and lowercase alphanumeric characters.
Here, we'll create five variables. The format is to type the name, the equals sign = , and the value. Note there isn't a space before or after the equals sign. Giving a variable a value is often referred to as assigning a value to the variable.
We'll create four string variables and one numeric variable,
my_name=Dave
my_boost=Linux
his_boost=Spinach
this_year=2019
To see the value held in a variable, use the echo command. You must precede the variable name with a dollar sign $ whenever you reference the value it contains, as shown below:
echo $my_name
echo $my_boost
echo $this_year
Let's use all of our variables at once:
echo "$my_boost is to $me as $his_boost is to $him (c) $this_year"
The values of the variables replace their names. You can also change the values of variables. To assign a new value to the variable, my_boost , you just repeat what you did when you assigned its first value, like so:
my_boost=Tequila
If you re-run the previous command, you now get a different result:
So, you can use the same command that references the same variables and get different results if you change the values held in the variables.
We'll talk about quoting variables later. For now, here are some things to remember:
- A variable in single quotes ' is treated as a literal string, and not as a variable.
- Variables in quotation marks " are treated as variables.
- To get the value held in a variable, you have to provide the dollar sign $ .
- A variable without the dollar sign $ only provides the name of the variable.
You can also create a variable that takes its value from an existing variable or number of variables. The following command defines a new variable called drink_of_the_Year, and assigns it the combined values of the my_boost and this_year variables:
drink_of-the_Year="$my_boost $this_year"
echo drink_of_the-Year
Scripts would be completely hamstrung without variables. Variables provide the flexibility that makes a script a general, rather than a specific, solution. To illustrate the difference, here's a script that counts the files in the /dev directory.
Type this into a text file, and then save it as fcnt.sh (for "file count"):
#!/bin/bashfolder_to_count=/devfile_count=$(ls $folder_to_count | wc -l)echo $file_count files in $folder_to_count
Before you can run the script, you have to make it executable, as shown below:
chmod +x fcnt.sh
Type the following to run the script:
This prints the number of files in the /dev directory. Here's how it works:
- A variable called folder_to_count is defined, and it's set to hold the string "/dev."
- Another variable, called file_count , is defined. This variable takes its value from a command substitution. This is the command phrase between the parentheses $( ) . Note there's a dollar sign $ before the first parenthesis. This construct $( ) evaluates the commands within the parentheses, and then returns their final value. In this example, that value is assigned to the file_count variable. As far as the file_count variable is concerned, it's passed a value to hold; it isn't concerned with how the value was obtained.
- The command evaluated in the command substitution performs an ls file listing on the directory in the folder_to_count variable, which has been set to "/dev." So, the script executes the command "ls /dev."
- The output from this command is piped into the wc command. The -l (line count) option causes wc to count the number of lines in the output from the ls command. As each file is listed on a separate line, this is the count of files and subdirectories in the "/dev" directory. This value is assigned to the file_count variable.
- The final line uses echo to output the result.
But this only works for the "/dev" directory. How can we make the script work with any directory? All it takes is one small change.
Many commands, such as ls and wc , take command line parameters. These provide information to the command, so it knows what you want it to do. If you want ls to work on your home directory and also to show hidden files , you can use the following command, where the tilde ~ and the -a (all) option are command line parameters:
Our scripts can accept command line parameters. They're referenced as $1 for the first parameter, $2 as the second, and so on, up to $9 for the ninth parameter. (Actually, there's a $0 , as well, but that's reserved to always hold the script.)
You can reference command line parameters in a script just as you would regular variables. Let's modify our script, as shown below, and save it with the new name fcnt2.sh :
#!/bin/bashfolder_to_count=$1file_count=$(ls $folder_to_count | wc -l)echo $file_count files in $folder_to_count
This time, the folder_to_count variable is assigned the value of the first command line parameter, $1 .
The rest of the script works exactly as it did before. Rather than a specific solution, your script is now a general one. You can use it on any directory because it's not hardcoded to work only with "/dev."
Here's how you make the script executable:
chmod +x fcnt2.sh
Now, try it with a few directories. You can do "/dev" first to make sure you get the same result as before. Type the following:
./fnct2.sh /dev
./fnct2.sh /etc
./fnct2.sh /bin
You get the same result (207 files) as before for the "/dev" directory. This is encouraging, and you get directory-specific results for each of the other command line parameters.
To shorten the script, you could dispense with the variable, folder_to_count , altogether, and just reference $1 throughout, as follows:
#!/bin/bash file_count=$(ls $1 wc -l) echo $file_count files in $1
We mentioned $0 , which is always set to the filename of the script. This allows you to use the script to do things like print its name out correctly, even if it's renamed. This is useful in logging situations, in which you want to know the name of the process that added an entry.
The following are the other special preset variables:
- $# : How many command line parameters were passed to the script.
- $@ : All the command line parameters passed to the script.
- $? : The exit status of the last process to run.
- $$ : The Process ID (PID) of the current script.
- $USER : The username of the user executing the script.
- $HOSTNAME : The hostname of the computer running the script.
- $SECONDS : The number of seconds the script has been running for.
- $RANDOM : Returns a random number.
- $LINENO : Returns the current line number of the script.
You want to see all of them in one script, don't you? You can! Save the following as a text file called, special.sh :
#!/bin/bashecho "There were $# command line parameters"echo "They are: $@"echo "Parameter 1 is: $1"echo "The script is called: $0"# any old process so that we can report on the exit statuspwdecho "pwd returned $?"echo "This script has Process ID $$"echo "The script was started by $USER"echo "It is running on $HOSTNAME"sleep 3echo "It has been running for $SECONDS seconds"echo "Random number: $RANDOM"echo "This is line number $LINENO of the script"
Type the following to make it executable:
chmod +x special.sh
Now, you can run it with a bunch of different command line parameters, as shown below.
Bash uses environment variables to define and record the properties of the environment it creates when it launches. These hold information Bash can readily access, such as your username, locale, the number of commands your history file can hold, your default editor, and lots more.
To see the active environment variables in your Bash session, use this command:
If you scroll through the list, you might find some that would be useful to reference in your scripts.
When a script runs, it's in its own process, and the variables it uses cannot be seen outside of that process. If you want to share a variable with another script that your script launches, you have to export that variable. We'll show you how to this with two scripts.
First, save the following with the filename script_one.sh :
#!/bin/bashfirst_var=alphasecond_var=bravo# check their valuesecho "$0: first_var=$first_var, second_var=$second_var"export first_varexport second_var./script_two.sh# check their values againecho "$0: first_var=$first_var, second_var=$second_var"
This creates two variables, first_var and second_var , and it assigns some values. It prints these to the terminal window, exports the variables, and calls script_two.sh . When script_two.sh terminates, and process flow returns to this script, it again prints the variables to the terminal window. Then, you can see if they changed.
The second script we'll use is script_two.sh . This is the script that script_one.sh calls. Type the following:
#!/bin/bash# check their valuesecho "$0: first_var=$first_var, second_var=$second_var"# set new valuesfirst_var=charliesecond_var=delta# check their values againecho "$0: first_var=$first_var, second_var=$second_var"
This second script prints the values of the two variables, assigns new values to them, and then prints them again.
To run these scripts, you have to type the following to make them executable:
chmod +x script_one.shchmod +x script_two.sh
And now, type the following to launch script_one.sh :
./script_one.sh
This is what the output tells us:
- script_one.sh prints the values of the variables, which are alpha and bravo.
- script_two.sh prints the values of the variables (alpha and bravo) as it received them.
- script_two.sh changes them to charlie and delta.
- script_one.sh prints the values of the variables, which are still alpha and bravo.
What happens in the second script, stays in the second script. It's like copies of the variables are sent to the second script, but they're discarded when that script exits. The original variables in the first script aren't altered by anything that happens to the copies of them in the second.
You might have noticed that when scripts reference variables, they're in quotation marks " . This allows variables to be referenced correctly, so their values are used when the line is executed in the script.
If the value you assign to a variable includes spaces, they must be in quotation marks when you assign them to the variable. This is because, by default, Bash uses a space as a delimiter.
Here's an example:
site_name=How-To Geek
Bash sees the space before "Geek" as an indication that a new command is starting. It reports that there is no such command, and abandons the line. echo shows us that the site_name variable holds nothing — not even the "How-To" text.
Try that again with quotation marks around the value, as shown below:
site_name="How-To Geek"
This time, it's recognized as a single value and assigned correctly to the site_name variable.
It can take some time to get used to command substitution, quoting variables, and remembering when to include the dollar sign.
Before you hit Enter and execute a line of Bash commands, try it with echo in front of it. This way, you can make sure what's going to happen is what you want. You can also catch any mistakes you might have made in the syntax.
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript variables, variables are containers for storing data.
JavaScript Variables can be declared in 4 ways:
- Automatically
- Using const
In this first example, x , y , and z are undeclared variables.
They are automatically declared when first used:
It is considered good programming practice to always declare variables before use.
From the examples you can guess:
- x stores the value 5
- y stores the value 6
- z stores the value 11
Example using var
The var keyword was used in all JavaScript code from 1995 to 2015.
The let and const keywords were added to JavaScript in 2015.
The var keyword should only be used in code written for older browsers.
Example using let
Example using const, mixed example.
The two variables price1 and price2 are declared with the const keyword.
These are constant values and cannot be changed.
The variable total is declared with the let keyword.
The value total can be changed.
When to Use var, let, or const?
1. Always declare variables
2. Always use const if the value should not be changed
3. Always use const if the type should not be changed (Arrays and Objects)
4. Only use let if you can't use const
5. Only use var if you MUST support old browsers.
Just Like Algebra
Just like in algebra, variables hold values:
Just like in algebra, variables are used in expressions:
From the example above, you can guess that the total is calculated to be 11.
Variables are containers for storing values.
Advertisement
JavaScript Identifiers
All JavaScript variables must be identified with unique names .
These unique names are called identifiers .
Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume).
The general rules for constructing names for variables (unique identifiers) are:
- Names can contain letters, digits, underscores, and dollar signs.
- Names must begin with a letter.
- Names can also begin with $ and _ (but we will not use it in this tutorial).
- Names are case sensitive (y and Y are different variables).
- Reserved words (like JavaScript keywords) cannot be used as names.
JavaScript identifiers are case-sensitive.
The Assignment Operator
In JavaScript, the equal sign ( = ) is an "assignment" operator, not an "equal to" operator.
This is different from algebra. The following does not make sense in algebra:
In JavaScript, however, it makes perfect sense: it assigns the value of x + 5 to x.
(It calculates the value of x + 5 and puts the result into x. The value of x is incremented by 5.)
The "equal to" operator is written like == in JavaScript.
JavaScript Data Types
JavaScript variables can hold numbers like 100 and text values like "John Doe".
In programming, text values are called text strings.
JavaScript can handle many types of data, but for now, just think of numbers and strings.
Strings are written inside double or single quotes. Numbers are written without quotes.
If you put a number in quotes, it will be treated as a text string.
Declaring a JavaScript Variable
Creating a variable in JavaScript is called "declaring" a variable.
You declare a JavaScript variable with the var or the let keyword:
After the declaration, the variable has no value (technically it is undefined ).
To assign a value to the variable, use the equal sign:
You can also assign a value to the variable when you declare it:
In the example below, we create a variable called carName and assign the value "Volvo" to it.
Then we "output" the value inside an HTML paragraph with id="demo":
It's a good programming practice to declare all variables at the beginning of a script.
One Statement, Many Variables
You can declare many variables in one statement.
Start the statement with let and separate the variables by comma :
A declaration can span multiple lines:
Value = undefined
In computer programs, variables are often declared without a value. The value can be something that has to be calculated, or something that will be provided later, like user input.
A variable declared without a value will have the value undefined .
The variable carName will have the value undefined after the execution of this statement:
Re-Declaring JavaScript Variables
If you re-declare a JavaScript variable declared with var , it will not lose its value.
The variable carName will still have the value "Volvo" after the execution of these statements:
You cannot re-declare a variable declared with let or const .
This will not work:
JavaScript Arithmetic
As with algebra, you can do arithmetic with JavaScript variables, using operators like = and + :
You can also add strings, but strings will be concatenated:
Also try this:
If you put a number in quotes, the rest of the numbers will be treated as strings, and concatenated.
Now try this:
JavaScript Dollar Sign $
Since JavaScript treats a dollar sign as a letter, identifiers containing $ are valid variable names:
Using the dollar sign is not very common in JavaScript, but professional programmers often use it as an alias for the main function in a JavaScript library.
In the JavaScript library jQuery, for instance, the main function $ is used to select HTML elements. In jQuery $("p"); means "select all p elements".
JavaScript Underscore (_)
Since JavaScript treats underscore as a letter, identifiers containing _ are valid variable names:
Using the underscore is not very common in JavaScript, but a convention among professional programmers is to use it as an alias for "private (hidden)" variables.
Test Yourself With Exercises
Create a variable called carName and assign the value Volvo to it.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
A c++ code processor that reads a .txt file containing c++ code with variable initialization and assignment. The processor stores the variables in a stack data structure.
aalhabibi/variable-processing-system
Folders and files.
Liquid, JavaScript, themes, sales channels
Shopify Community
- Shopify Discussion
- Technical Q&A
change the assigned value of a liquid variable based on radio select
- Subscribe to RSS Feed
- Mark Topic as New
- Mark Topic as Read
- Float this Topic for Current User
- Printer Friendly Page
- Mark as New
- Report Inappropriate Content
- All forum topics
- Previous Topic
- Next Topic Next topic
Community Blog Articles
Hey, Shopify users! If you’ve picked a free theme from Shopify, you're in for some great ...
How Shopify Got Started Ever wondered how Shopify began? Back in 2004, a snowboard en...
Congratulations on deciding to start your own Shopify store! Whether you're aiming t...
Quick Links
- Português do Brasil
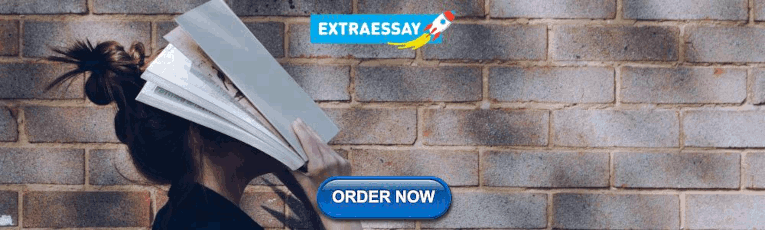
IMAGES
VIDEO
COMMENTS
1.4 — Variable assignment and initialization. Alex May 26, 2024. In the previous lesson ( 1.3 -- Introduction to objects and variables ), we covered how to define a variable that we can use to store values. In this lesson, we'll explore how to actually put values into variables and use those values. As a reminder, here's a short snippet ...
In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ): Python. >>> n = 300. This is read or interpreted as " n is assigned the value 300 .".
Assigning variables. Here's how we create a variable named score in JavaScript: var score = 0; That line of code is called a statement. All programs are made up of statements, and each statement is an instruction to the computer about something we need it to do. Let's add the lives variable: var score = 0; var lives = 3;
In Python, variables are created the moment you give or assign a value to them. How Do I Assign a Value to a Variable? Assigning a value to a variable in Python is an easy process. You simply use the equal sign = as an assignment operator, followed by the value you want to assign to the variable. Here's an example:
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
Variable Assignment. Think of a variable as a name attached to a particular object. In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ).
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
A variable is a way to store values. To use a variable, we must both declare it—to let the program know about the variable—and then assign it—to let the program know what value we are storing in the variable. Here's how we would declare a variable named "xPos": var xPos; Now, we can assign xPos to hold the value 10:
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Declare And Assign Value To Variable. Assignment sets a value to a variable. To assign variable a value, use the equals sign (=) myFirstVariable = 1 mySecondVariable = 2 myFirstVariable = "Hello You" Assigning a value is known as binding in Python. In the example above, we have assigned the value of 2 to mySecondVariable.
In JavaScript, variable assignment refers to the process of assigning a value to a variable. For example, let x = 5; Here, we are assigning the value 5 to the variable x. On the other hand ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
A Python variable is a named bit of computer memory, keeping track of a value as the code runs. A variable is created with an "assignment" equal sign =, with the variable's name on the left and the value it should store on the right: x = 42 In the computer's memory, each variable is like a box, identified by the name of the variable.
Agreed, this is my favorite answer as it specifically addresses JavaScript variable assignment concerns. Additionally, if you choose to use a ternary as one of the subsequent variables to test for assignment (after the operator) you must wrap the ternary in parentheses for assignment evaluation to work properly. -
Variable in Programming is a named storage location that holds a value or data. These values can change during the execution of a program, hence the term "variable.". Variables are essential for storing and manipulating data in computer programs. A variable is the basic building block of a program that can be used in expressions as a ...
Assignment (=) The assignment ( =) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A variable is a name for a value. An assignment statement associates a variable name on the left of the equal sign with the value of an expression calculated from the right of the equal sign. Enter. width. Once a variable is assigned a value, the variable can be used in place of that value. The response to the expression width is the same as if ...
Java Variables. Variables are containers for storing data values. In Java, there are different types of variables, for example: String - stores text, such as "Hello". String values are surrounded by double quotes. int - stores integers (whole numbers), without decimals, such as 123 or -123. float - stores floating point numbers, with decimals ...
Python Variables Variable Names Assign Multiple Values Output Variables Global Variables Variable Exercises. Python Data Types Python Numbers Python Casting Python Strings. Python Strings Slicing Strings Modify Strings Concatenate Strings Format Strings Escape Characters String Methods String Exercises.
Set a value in a Transact-SQL variable. When a variable is first declared, its value is set to NULL. To assign a value to a variable, use the SET statement. This is the preferred method of assigning a value to a variable. A variable can also have a value assigned by being referenced in the select list of a SELECT statement.
Here, we'll create five variables. The format is to type the name, the equals sign =, and the value. Note there isn't a space before or after the equals sign. Giving a variable a value is often referred to as assigning a value to the variable. We'll create four string variables and one numeric variable, my_name=Dave.
All JavaScript variables must be identified with unique names. These unique names are called identifiers. Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume). The general rules for constructing names for variables (unique identifiers) are: Names can contain letters, digits, underscores, and dollar signs.
Variable assignment. A basic concept in (statistical) programming is called a variable. A variable allows you to store a value (e.g. 4) or an object (e.g. a function description) in R. You can then later use this variable's name to easily access the value or the object that is stored within this variable. You can assign a value 4 to a variable ...
The processor stores the variables in a stack data structure. - aalhabibi/variable-processing-system A c++ code processor that reads a .txt file containing c++ code with variable initialization and assignment.
41m ago. I guess you misunderstood i want to change the value of this liquid variable {%- assign product = block.settings.featured_product | default: product | default: collections ['all'].products.first -%} based on input select as it will change the assigned product to my featured product. 0.