
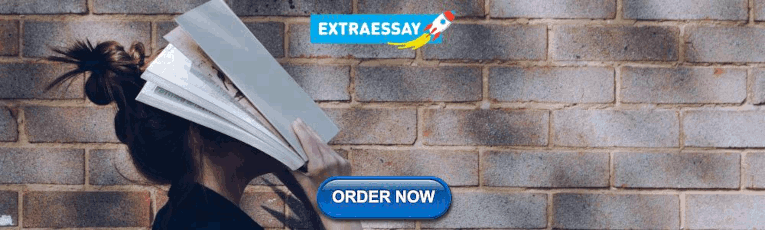
22.5 — std::unique_ptr
At the beginning of the chapter, we discussed how the use of pointers can lead to bugs and memory leaks in some situations. For example, this can happen when a function early returns, or throws an exception, and the pointer is not properly deleted.
Now that we’ve covered the fundamentals of move semantics, we can return to the topic of smart pointer classes. As a reminder, a smart pointer is a class that manages a dynamically allocated object. Although smart pointers can offer other features, the defining characteristic of a smart pointer is that it manages a dynamically allocated resource, and ensures the dynamically allocated object is properly cleaned up at the appropriate time (usually when the smart pointer goes out of scope).
Because of this, smart pointers should never be dynamically allocated themselves (otherwise, there is the risk that the smart pointer may not be properly deallocated, which means the object it owns would not be deallocated, causing a memory leak). By always allocating smart pointers on the stack (as local variables or composition members of a class), we’re guaranteed that the smart pointer will properly go out of scope when the function or object it is contained within ends, ensuring the object the smart pointer owns is properly deallocated.
C++11 standard library ships with 4 smart pointer classes: std::auto_ptr (removed in C++17), std::unique_ptr, std::shared_ptr, and std::weak_ptr. std::unique_ptr is by far the most used smart pointer class, so we’ll cover that one first. In the following lessons, we’ll cover std::shared_ptr and std::weak_ptr.
std::unique_ptr
std::unique_ptr is the C++11 replacement for std::auto_ptr. It should be used to manage any dynamically allocated object that is not shared by multiple objects. That is, std::unique_ptr should completely own the object it manages, not share that ownership with other classes. std::unique_ptr lives in the <memory> header.
Let’s take a look at a simple smart pointer example:
Because the std::unique_ptr is allocated on the stack here, it’s guaranteed to eventually go out of scope, and when it does, it will delete the Resource it is managing.
Unlike std::auto_ptr, std::unique_ptr properly implements move semantics.
This prints:
Because std::unique_ptr is designed with move semantics in mind, copy initialization and copy assignment are disabled. If you want to transfer the contents managed by std::unique_ptr, you must use move semantics. In the program above, we accomplish this via std::move (which converts res1 into an r-value, which triggers a move assignment instead of a copy assignment).
Accessing the managed object
std::unique_ptr has an overloaded operator* and operator-> that can be used to return the resource being managed. Operator* returns a reference to the managed resource, and operator-> returns a pointer.
Remember that std::unique_ptr may not always be managing an object -- either because it was created empty (using the default constructor or passing in a nullptr as the parameter), or because the resource it was managing got moved to another std::unique_ptr. So before we use either of these operators, we should check whether the std::unique_ptr actually has a resource. Fortunately, this is easy: std::unique_ptr has a cast to bool that returns true if the std::unique_ptr is managing a resource.
Here’s an example of this:
In the above program, we use the overloaded operator* to get the Resource object owned by std::unique_ptr res, which we then send to std::cout for printing.
std::unique_ptr and arrays
Unlike std::auto_ptr, std::unique_ptr is smart enough to know whether to use scalar delete or array delete, so std::unique_ptr is okay to use with both scalar objects and arrays.
However, std::array or std::vector (or std::string) are almost always better choices than using std::unique_ptr with a fixed array, dynamic array, or C-style string.
Best practice
Favor std::array, std::vector, or std::string over a smart pointer managing a fixed array, dynamic array, or C-style string.
std::make_unique
C++14 comes with an additional function named std::make_unique(). This templated function constructs an object of the template type and initializes it with the arguments passed into the function.
The code above prints:
Use of std::make_unique() is optional, but is recommended over creating std::unique_ptr yourself. This is because code using std::make_unique is simpler, and it also requires less typing (when used with automatic type deduction). Furthermore, in C++14 it resolves an exception safety issue that can result from C++ leaving the order of evaluation for function arguments unspecified.
Use std::make_unique() instead of creating std::unique_ptr and using new yourself.
The exception safety issue in more detail
For those wondering what the “exception safety issue” mentioned above is, here’s a description of the issue.
Consider an expression like this one:
The compiler is given a lot of flexibility in terms of how it handles this call. It could create a new T, then call function_that_can_throw_exception(), then create the std::unique_ptr that manages the dynamically allocated T. If function_that_can_throw_exception() throws an exception, then the T that was allocated will not be deallocated, because the smart pointer to do the deallocation hasn’t been created yet. This leads to T being leaked.
std::make_unique() doesn’t suffer from this problem because the creation of the object T and the creation of the std::unique_ptr happen inside the std::make_unique() function, where there’s no ambiguity about order of execution.
This issue was fixed in C++17, as evaluation of function arguments can no longer be interleaved.
Returning std::unique_ptr from a function
std::unique_ptr can be safely returned from a function by value:
In the above code, createResource() returns a std::unique_ptr by value. If this value is not assigned to anything, the temporary return value will go out of scope and the Resource will be cleaned up. If it is assigned (as shown in main()), in C++14 or earlier, move semantics will be employed to transfer the Resource from the return value to the object assigned to (in the above example, ptr), and in C++17 or newer, the return will be elided. This makes returning a resource by std::unique_ptr much safer than returning raw pointers!
In general, you should not return std::unique_ptr by pointer (ever) or reference (unless you have a specific compelling reason to).
Passing std::unique_ptr to a function
If you want the function to take ownership of the contents of the pointer, pass the std::unique_ptr by value. Note that because copy semantics have been disabled, you’ll need to use std::move to actually pass the variable in.
The above program prints:
Note that in this case, ownership of the Resource was transferred to takeOwnership(), so the Resource was destroyed at the end of takeOwnership() rather than the end of main().
However, most of the time, you won’t want the function to take ownership of the resource. Although you can pass a std::unique_ptr by reference (which will allow the function to use the object without assuming ownership), you should only do so when the called function might alter or change the object being managed.
Instead, it’s better to just pass the resource itself (by pointer or reference, depending on whether null is a valid argument). This allows the function to remain agnostic of how the caller is managing its resources. To get a raw resource pointer from a std::unique_ptr, you can use the get() member function:
std::unique_ptr and classes
You can, of course, use std::unique_ptr as a composition member of your class. This way, you don’t have to worry about ensuring your class destructor deletes the dynamic memory, as the std::unique_ptr will be automatically destroyed when the class object is destroyed.
However, if the class object is not destroyed properly (e.g. it is dynamically allocated and not deallocated properly), then the std::unique_ptr member will not be destroyed either, and the object being managed by the std::unique_ptr will not be deallocated.
Misusing std::unique_ptr
There are two easy ways to misuse std::unique_ptrs, both of which are easily avoided. First, don’t let multiple objects manage the same resource. For example:
While this is legal syntactically, the end result will be that both res1 and res2 will try to delete the Resource, which will lead to undefined behavior.
Second, don’t manually delete the resource out from underneath the std::unique_ptr.
If you do, the std::unique_ptr will try to delete an already deleted resource, again leading to undefined behavior.
Note that std::make_unique() prevents both of the above cases from happening inadvertently.
Question #1
Convert the following program from using a normal pointer to using std::unique_ptr where appropriate:
Show Solution
std::unique_ptr
Defined in header <memory> .
Declarations
Description .
std::unique_ptr is a smart pointer that owns and manages another object through a pointer and disposes of that object when the unique_ptr goes out of scope.
The object is disposed of, using the associated deleter when either of the following happens:
the managing unique_ptr object is destroyed
the managing unique_ptr object is assigned another pointer via operator = or reset()
The object is disposed of, using a potentially user-supplied deleter by calling get_deleter()(ptr) . The default deleter uses the delete operator, which destroys the object and deallocates the memory.
A unique_ptr may alternatively own no object, in which case it is called empty .
There are two versions of std::unique_ptr :
- Manages a single object (e.g. allocated with new );
- Manages a dynamically-allocated array of objects (e.g. allocated with new[] ).
The class satisfies the requirements of MoveConstructible and MoveAssignable, but of neither CopyConstructible nor CopyAssignable.
Type requirements
Deleter must be FunctionObject or lvalue reference to a FunctionObject or lvalue reference to function, callable with an argument of type unique_ptr<T, Deleter>::pointer .
Only non-const unique_ptr can transfer the ownership of the managed object to another unique_ptr . If an object's lifetime is managed by a const std::unique_ptr , it is limited to the scope in which the pointer was created.
std::unique_ptr is commonly used to manage the lifetime of objects, including:
- Providing exception safety to classes and functions that handle objects with dynamic lifetime, by guaranteeing deletion on both normal exit and exit through exception;
- Passing ownership of uniquely-owned objects with dynamic lifetime into functions;
- Acquiring ownership of uniquely-owned objects with dynamic lifetime from functions;
- As the element type in move-aware containers, such as std::vector , which hold pointers to dynamically-allocated objects (e.g. if polymorphic behavior is desired).
std::unique_ptr may be constructed for an incomplete type T , such as to facilitate the use as a handle in the pImpl idiom. If the default deleter is used, T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr . (Conversely, std::shared_ptr can't be constructed from a raw pointer to incomplete type, but can be destroyed where T is incomplete). Note that if T is a class template specialization, use of unique_ptr as an operand, e.g. !p requires T 's parameters to be complete due to ADL.
If T is a derived class of some base B , then std::unique_ptr<T> is implicitly convertible to std::unique_ptr<B> . The default deleter of the resulting std::unique_ptr<B> will use operator delete for B , leading to undefined behavior unless the destructor of B is virtual. Note that std::shared_ptr behaves differently: std::shared_ptr<B> will use the operator delete for the type T and the owned object will be deleted correctly even if the destructor of B is not virtual.
Unlike std::shared_ptr , std::unique_ptr may manage an object through any custom handle type that satisfies NullablePointer. This allows, for example, managing objects located in shared memory, by supplying a Deleter that defines typedef boost::offset_ptr pointer ; or another fancy pointer.
Member types
Member functions , modifiers , observers , single-object version, unique_ptr<t> , array version, unique_ptr<t[]> , non-member functions , helper classes .
- Declarations
- Description
- Member types
- Member functions
- Non-member functions
- Helper Classes

Programming Tutorials
Copying and Moving unique_ptr in C++
In C++, a unique_ptr is a smart pointer that owns and manages dynamically allocated memory. It automatically deletes the linked memory when the unique_ptr goes out of scope. One key aspect of unique_ptr is ownership uniqueness, meaning that there can only be one unique_ptr pointing to any given resource at a time. This uniqueness is enforced by the language to prevent multiple unique_ptr s from managing the same resource, which would lead to issues like double deletion.
Can a Unique_ptr be copied?
Since unique_ptr s enforce unique ownership, you cannot copy a unique_ptr . Attempting to do so will result in a compilation error. Here’s a code snippet that shows what happens when you try to copy a unique_ptr :
Moving Unique_ptr is allowed
Although copying a unique_ptr object is not allowed, but you can transfer ownership of underlying memory resource managed by a unique_ptr to another unique_ptr using the std::move() function. This process is called “moving” and effectively renders the source unique_ptr empty (i.e., it becomes nullptr ) and the destination unique_ptr now owns the resource (memory).
Here’s an example of how to move a unique_ptr :
In Modern C++, the Smart Pointer unique_ptr ensures that only one smart pointer can manage a given resource at a time. You cannot copy a unique_ptr but you can transfer its ownership to another unique_ptr using std::move() . This mechanism is important for maintaining resource safety and preventing memory leaks in C++ programs. Remember, once moved, the original unique_ptr will be reset to nullptr , and attempting to use it will be as if it points to no resource at all.
Related posts:
- Reset unique_ptr in Modern C++
- What is shared_ptr in C++?
- Shared_ptr & Custom Deleter in Modern C++
- Smart Pointer vs Raw Pointer in C++
- How not to use Smart Pointers in C++?
- What is weak_ptr in Modern C++ & why do we need it?
- What is unique_ptr in C++?
- Introduction to Smart Pointers in Modern C++
- Using std::find & std::find_if with User Defined Classes
- C++11 Multithreading – Part 4: Data Sharing and Race Conditions
- C++11 Multithreading – Part 5: Using mutex to fix Race Conditions
- C++ Set example and Tutorial – Part 1
- C++11 Multithreading – Part 7: Condition Variables Explained
- C++11 Multithreading – Part 8: std::future , std::promise and Returning values from Thread
- Lambda Functions in C++
- std::bind in C++ – Explained with Examples
- The auto Keyword in C++11
- multimap Example and Tutorial in C++
- C++11 Multithreading – Part 9: std::async Tutorial & Example
- How to Capture Member Variables in Lambda function in C++?
Share your love
Leave a comment cancel reply.
Your email address will not be published. Required fields are marked *
This site uses Akismet to reduce spam. Learn how your comment data is processed .
cppreference.com
Std:: unique_ptr.
std::unique_ptr is a smart pointer that owns and manages another object through a pointer and disposes of that object when the unique_ptr goes out of scope.
The object is disposed of using the associated deleter when either of the following happens:
- the managing unique_ptr object is destroyed
- the managing unique_ptr object is assigned another pointer via operator= or reset() .
The object is disposed of using a potentially user-supplied deleter by calling get_deleter ( ) ( ptr ) . The default deleter uses the delete operator, which destroys the object and deallocates the memory.
A unique_ptr may alternatively own no object, in which case it is called empty .
There are two versions of std::unique_ptr :
The class satisfies the requirements of MoveConstructible and MoveAssignable , but not the requirements of either CopyConstructible or CopyAssignable .
[ edit ] Notes
Only non-const unique_ptr can transfer the ownership of the managed object to another unique_ptr . If an object's lifetime is managed by a const std :: unique_ptr , it is limited to the scope in which the pointer was created.
std::unique_ptr is commonly used to manage the lifetime of objects, including:
- providing exception safety to classes and functions that handle objects with dynamic lifetime, by guaranteeing deletion on both normal exit and exit through exception
- passing ownership of uniquely-owned objects with dynamic lifetime into functions
- acquiring ownership of uniquely-owned objects with dynamic lifetime from functions
- as the element type in move-aware containers, such as std::vector , which hold pointers to dynamically-allocated objects (e.g. if polymorphic behavior is desired)
std::unique_ptr may be constructed for an incomplete type T , such as to facilitate the use as a handle in the pImpl idiom . If the default deleter is used, T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr . (Conversely, std::shared_ptr can't be constructed from a raw pointer to incomplete type, but can be destroyed where T is incomplete). Note that if T is a class template specialization, use of unique_ptr as an operand, e.g. ! p requires T 's parameters to be complete due to ADL .
If T is a derived class of some base B , then std :: unique_ptr < T > is implicitly convertible to std :: unique_ptr < B > . The default deleter of the resulting std :: unique_ptr < B > will use operator delete for B , leading to undefined behavior unless the destructor of B is virtual . Note that std::shared_ptr behaves differently: std:: shared_ptr < B > will use the operator delete for the type T and the owned object will be deleted correctly even if the destructor of B is not virtual .
Unlike std::shared_ptr , std::unique_ptr may manage an object through any custom handle type that satisfies NullablePointer . This allows, for example, managing objects located in shared memory, by supplying a Deleter that defines typedef boost::offset_ptr pointer; or another fancy pointer .
[ edit ] Member types
[ edit ] member functions, [ edit ] non-member functions, [ edit ] helper classes, [ edit ] example.
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 8 February 2019, at 16:06.
- This page has been accessed 2,282,962 times.
- Privacy policy
- About cppreference.com
- Disclaimers
cppreference.com

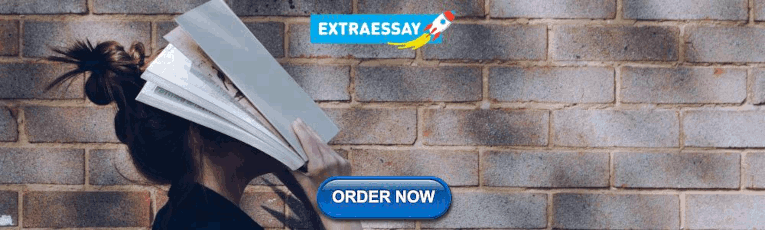
std:: unique_ptr
std::unique_ptr is a smart pointer that retains sole ownership of an object through a pointer and destroys that object when the unique_ptr goes out of scope. No two unique_ptr instances can manage the same object.
The object is destroyed and its memory deallocated when either of the following happens:
- unique_ptr managing the object is destroyed
- unique_ptr managing the object is assigned another pointer via operator= or reset() .
The object is destroyed using a potentially user-supplied deleter by calling Deleter ( ptr ) . The deleter calls the destructor of the object and dispenses the memory.
A unique_ptr may alternatively own no object, in which case it is called empty .
There are two versions of std::unique_ptr :
The class satisfies the requirements of MoveConstructible and MoveAssignable , but not the requirements of either CopyConstructible or CopyAssignable .
[ edit ] Notes
Only non-const unique_ptr can transfer the ownership of the managed object to another unique_ptr . The lifetime of an object managed by const std :: unique_ptr is limited to the scope in which the pointer was created.
Typical uses of std::unique_ptr include:
- providing exception safety to classes and functions that handle objects with dynamic lifetime, by guaranteeing deletion on both normal exit and exit through exception
- passing ownership of uniquely-owned objects with dynamic lifetime into functions
- acquiring ownership of uniquely-owned objects with dynamic lifetime from functions
- as the element type in move-aware containers, such as std::vector , which hold pointers to dynamically-allocated objects (e.g. if polymorphic behavior is desired)
std::unique_ptr may be constructed for an incomplete type T , such as to facilitate the use as a handle in the Pimpl idiom. If the default deleter is used, T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr . (Conversely, std::shared_ptr can't be constructed from a raw pointer to incomplete type, but can be destroyed where T is incomplete).
If T is a derived class of some base B , then std :: unique_ptr < T > is implicitly convertible to std :: unique_ptr < B > . The default deleter of the resulting std :: unique_ptr < B > will use operator delete for B , leading to undefined behavior unless the destructor of B is virtual . Note that std::shared_ptr behaves differently: std:: shared_ptr < B > will use the operator delete for the type T and the owned object will be deleted correctly even if the destructor of B is not virtual .
Unlike std::shared_ptr , std::unique_ptr may manage an object through any custom handle type that satisfies NullablePointer . This allows, for example, managing objects located in shared memory, by supplying a Deleter that defines typedef boost::offset_ptr pointer;
[ edit ] Member types
[ edit ] member functions, [ edit ] non-member functions, [ edit ] helper classes, [ edit ] example.
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 24 November 2015, at 05:11.
- This page has been accessed 645,787 times.
- Privacy policy
- About cppreference.com
- Disclaimers

This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
How to: Create and use unique_ptr instances
- 8 contributors
A unique_ptr does not share its pointer. It cannot be copied to another unique_ptr , passed by value to a function, or used in any C++ Standard Library algorithm that requires copies to be made. A unique_ptr can only be moved. This means that the ownership of the memory resource is transferred to another unique_ptr and the original unique_ptr no longer owns it. We recommend that you restrict an object to one owner, because multiple ownership adds complexity to the program logic. Therefore, when you need a smart pointer for a plain C++ object, use unique_ptr , and when you construct a unique_ptr , use the make_unique helper function.
The following diagram illustrates the transfer of ownership between two unique_ptr instances.

unique_ptr is defined in the <memory> header in the C++ Standard Library. It is exactly as efficient as a raw pointer and can be used in C++ Standard Library containers. The addition of unique_ptr instances to C++ Standard Library containers is efficient because the move constructor of the unique_ptr eliminates the need for a copy operation.
The following example shows how to create unique_ptr instances and pass them between functions.
These examples demonstrate this basic characteristic of unique_ptr : it can be moved, but not copied. "Moving" transfers ownership to a new unique_ptr and resets the old unique_ptr .
The following example shows how to create unique_ptr instances and use them in a vector.
In the range for loop, notice that the unique_ptr is passed by reference. If you try to pass by value here, the compiler will throw an error because the unique_ptr copy constructor is deleted.
The following example shows how to initialize a unique_ptr that is a class member.
You can use make_unique to create a unique_ptr to an array, but you cannot use make_unique to initialize the array elements.
For more examples, see make_unique .
Smart Pointers (Modern C++) make_unique
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- <cassert> (assert.h)
- <cctype> (ctype.h)
- <cerrno> (errno.h)
- C++11 <cfenv> (fenv.h)
- <cfloat> (float.h)
- C++11 <cinttypes> (inttypes.h)
- <ciso646> (iso646.h)
- <climits> (limits.h)
- <clocale> (locale.h)
- <cmath> (math.h)
- <csetjmp> (setjmp.h)
- <csignal> (signal.h)
- <cstdarg> (stdarg.h)
- C++11 <cstdbool> (stdbool.h)
- <cstddef> (stddef.h)
- C++11 <cstdint> (stdint.h)
- <cstdio> (stdio.h)
- <cstdlib> (stdlib.h)
- <cstring> (string.h)
- C++11 <ctgmath> (tgmath.h)
- <ctime> (time.h)
- C++11 <cuchar> (uchar.h)
- <cwchar> (wchar.h)
- <cwctype> (wctype.h)
Containers:
- C++11 <array>
- <deque>
- C++11 <forward_list>
- <list>
- <map>
- <queue>
- <set>
- <stack>
- C++11 <unordered_map>
- C++11 <unordered_set>
- <vector>
Input/Output:
- <fstream>
- <iomanip>
- <ios>
- <iosfwd>
- <iostream>
- <istream>
- <ostream>
- <sstream>
- <streambuf>
Multi-threading:
- C++11 <atomic>
- C++11 <condition_variable>
- C++11 <future>
- C++11 <mutex>
- C++11 <thread>
- <algorithm>
- <bitset>
- C++11 <chrono>
- C++11 <codecvt>
- <complex>
- <exception>
- <functional>
- C++11 <initializer_list>
- <iterator>
- <limits>
- <locale>
- <memory>
- <new>
- <numeric>
- C++11 <random>
- C++11 <ratio>
- C++11 <regex>
- <stdexcept>
- <string>
- C++11 <system_error>
- C++11 <tuple>
- C++11 <type_traits>
- C++11 <typeindex>
- <typeinfo>
- <utility>
- <valarray>
- C++11 allocator_arg_t
- C++11 allocator_traits
- auto_ptr_ref
- C++11 bad_weak_ptr
- C++11 default_delete
- C++11 enable_shared_from_this
- C++11 owner_less
- C++11 pointer_traits
- raw_storage_iterator
- C++11 shared_ptr
- C++11 unique_ptr
- C++11 uses_allocator
- C++11 weak_ptr
enum classes
- C++11 pointer_safety
- C++11 addressof
- C++11 align
- C++11 allocate_shared
- C++11 const_pointer_cast
- C++11 declare_no_pointers
- C++11 declare_reachable
- C++11 dynamic_pointer_cast
- C++11 get_deleter
- C++11 get_pointer_safety
- get_temporary_buffer
- C++11 make_shared
- return_temporary_buffer
- C++11 static_pointer_cast
- C++11 undeclare_no_pointers
- C++11 undeclare_reachable
- uninitialized_copy
- C++11 uninitialized_copy_n
- uninitialized_fill
- uninitialized_fill_n
- C++11 allocator_arg
- C++11 unique_ptr::~unique_ptr
- C++11 unique_ptr::unique_ptr
member functions
- C++11 unique_ptr::get
- C++11 unique_ptr::get_deleter
- C++11 unique_ptr::operator bool
- C++11 /" title="unique_ptr::operator->"> unique_ptr::operator->
- C++11 unique_ptr::operator[]
- C++11 unique_ptr::operator*
- C++11 unique_ptr::operator=
- C++11 unique_ptr::release
- C++11 unique_ptr::reset
- C++11 unique_ptr::swap
non-member overloads
- C++11 relational operators (unique_ptr)
- C++11 swap (unique_ptr)
std:: unique_ptr ::reset
Return value.
std:: unique_ptr
std::unique_ptr is a smart pointer that retains sole ownership of an object through a pointer and destroys that object when the unique_ptr goes out of scope. No two unique_ptr instances can manage the same object.
The object is destroyed and its memory deallocated when either of the following happens:
- unique_ptr managing the object is destroyed
- unique_ptr managing the object is assigned another pointer via operator= or reset() .
The object is destroyed using a potentially user-supplied deleter by calling Deleter ( ptr ) . The deleter calls the destructor of the object and dispenses the memory.
A unique_ptr may alternatively own no object, in which case it is called empty .
There are two versions of std::unique_ptr :
The class satisfies the requirements of MoveConstructible and MoveAssignable , but not the requirements of either CopyConstructible or CopyAssignable .
[ edit ] Notes
Only non-const unique_ptr can transfer the ownership of the managed object to another unique_ptr . The lifetime of an object managed by const std :: unique_ptr is limited to the scope in which the pointer was created.
Typical uses of std::unique_ptr include:
- providing exception safety to classes and functions that handle objects with dynamic lifetime, by guaranteeing deletion on both normal exit and exit through exception
- passing ownership of uniquely-owned objects with dynamic lifetime into functions
- acquiring ownership of uniquely-owned objects with dynamic lifetime from functions
- as the element type in move-aware containers, such as std::vector , which hold pointers to dynamically-allocated objects (e.g. if polymorphic behavior is desired)
std::unique_ptr may be constructed for an incomplete type T , such as to facilitate the use as a handle in the Pimpl idiom. If the default deleter is used, T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr . (Conversely, std::shared_ptr can't be constructed from a raw pointer to incomplete type, but can be destroyed where T is incomplete).
If T is a derived class of some base B , then std :: unique_ptr < T > is implicitly convertible to std :: unique_ptr < B > . The default deleter of the resulting std :: unique_ptr < B > will use operator delete for B , leading to undefined behavior unless the destructor of B is virtual . Note that std::shared_ptr behaves differently: std:: shared_ptr < B > will use the operator delete for the type T and the owned object will be deleted correctly even if the destructor of B is not virtual .
Unlike std::shared_ptr , std::unique_ptr may manage an object through any custom handle type that satisfies NullablePointer . This allows, for example, managing objects located in shared memory, by supplying a Deleter that defines typedef boost::offset_ptr pointer;
[ edit ] Member types
[ edit ] member functions, [ edit ] non-member functions, [ edit ] helper classes, [ edit ] example.
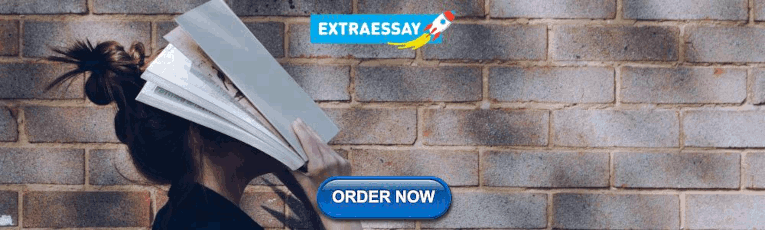
IMAGES
VIDEO
COMMENTS
std::unique_ptr is a smart pointer that owns and manages another object through a pointer and disposes of that object when the unique_ptr goes out of scope. ... T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr.
std::move creates a RValue Reference from unique_ptr. function_call_move takes a RValue Reference, but until a RValue Reference assignment operator or constructor is used to steal the unique_ptr's information it doesn't get harmed. Essentially just because you can mug it and steal it's info doesn't mean you have to.
Because std::unique_ptr is designed with move semantics in mind, copy initialization and copy assignment are disabled. If you want to transfer the contents managed by std::unique_ptr, you must use move semantics. ... In the program above, we accomplish this via std::move (which converts res1 into an r-value, which triggers a move assignment ...
unique_ptr objects replicate a limited pointer functionality by providing access to its managed object through operators * and -> (for individual objects), or operator [] (for array objects). For safety reasons, they do not support pointer arithmetics, and only support move assignment (disabling copy assignments).
std::unique_ptr is a smart pointer that owns and manages another object through a pointer and disposes of that object when the unique_ptr goes out of scope. ... T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr.
In Modern C++, the Smart Pointer unique_ptr ensures that only one smart pointer can manage a given resource at a time. You cannot copy a unique_ptr but you can transfer its ownership to another unique_ptr using std::move(). This mechanism is important for maintaining resource safety and preventing memory leaks in C++ programs.
As a move-only type, unique_ptr's assignment operator only accepts rvalues arguments (e.g. the result of std::make_unique or a std::move 'd unique_ptr variable). [ edit ] Example Run this code
From cppreference.com < cpp | memorycpp | memory C++
Constructs a unique_ptr object, depending on the signature used: default constructor (1), and (2) The object is empty (owns nothing), with value-initialized stored pointer and stored deleter. construct from pointer (3) The object takes ownership of p, initializing its stored pointer to p and value-initializing its stored deleter. construct from pointer + lvalue deleter (4)
If the default deleter is used, T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr. (Conversely, std::shared_ptr can't be constructed from a raw pointer to incomplete type, but can be destroyed where T is incomplete). If T ...
The object acquires the ownership of x's content, including both the stored pointer and the stored deleter (along with the responsibility of deleting the object at some point). Any object owned by the unique_ptr object before the call is deleted (as if unique_ptr's destructor was called). If the argument is a null pointer (2), the unique_ptr object becomes empty, with the same behavior as if ...
1. When you do x = std::move(y) And y is of type std::unique_ptr<T>, then x must also be of type std::unique_ptr<T> (or two types whose pointers are implicitly convertible) or the assignment won't work. Your code, as shown, can only work if T is int8_t because that's what's on the left. edited May 7, 2020 at 22:03. answered May 7, 2020 at 20:32.
The following example shows how to create unique_ptr instances and pass them between functions. // Implicit move operation into the variable that stores the result. return make_unique<Song>(artist, title); void MakeSongs() {. // Create a new unique_ptr with a new object. auto song = make_unique<Song>(L"Mr. Children", L"Namonaki Uta");
unique_ptr objects replicate a limited pointer functionality by providing access to its managed object through operators * and -> (for individual objects), or operator [] (for array objects). For safety reasons, they do not support pointer arithmetics, and only support move assignment (disabling copy assignments).
If you can reasonably use construction instead of assignment, do that. These criteria are good for many types, including when U is std::unique_ptr&& and S is std::shared_ptr. One caveat for the smart pointer case is that the assignment must be from an rvalue. That is, if the unique_ptr is not being returned by a function, you probably have to ...
Since you don't have one, it's copy assignment operator will be called. Then RenderManger's copy assignment will be called. Finally it's the copy assignment of unique_ptr, which is deleted. A move assignment is needed for Engine, as . Engine & operator = (Engine && rhs) { r._currentCamera = std::move(rhs.r._currentCamera); return * this; }
Destroys the object currently managed by the unique_ptr (if any) and takes ownership of p. If p is a null pointer (such as a default-initialized pointer), the unique_ptr becomes empty, managing no object after the call. To release the ownership of the stored pointer without destroying it, use member function release instead. The specialization of unique_ptr for array objects with runtime ...
If the default deleter is used, T must be complete at the point in code where the deleter is invoked, which happens in the destructor, move assignment operator, and reset member function of std::unique_ptr. (Conversely, std::shared_ptr can't be constructed from a raw pointer to incomplete type, but can be destroyed where T is incomplete). If T ...