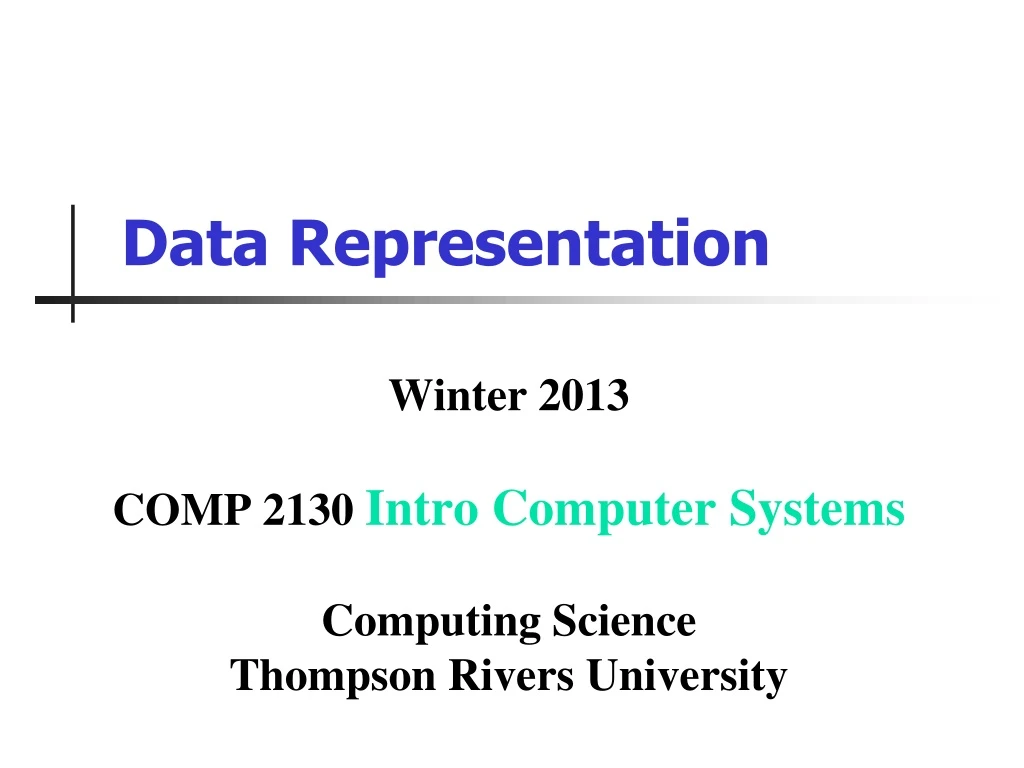
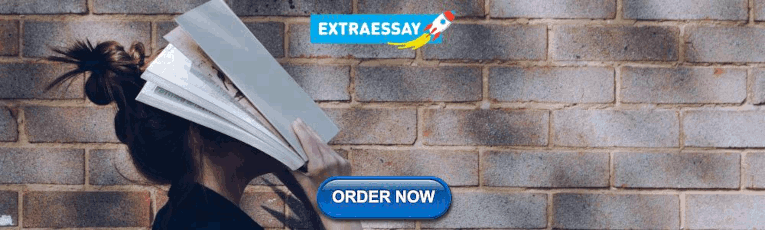
Data Representation
Dec 20, 2019
790 likes | 1.21k Views
Data Representation. Winter 2013 COMP 2130 Intro Computer Systems Computing Science Thompson Rivers University. Course Objectives. The better knowledge of computer systems, the better programing. Course Contents. Introduction to computer systems: B&O 1
Share Presentation

Presentation Transcript
Data Representation Winter 2013 COMP 2130 Intro Computer Systems Computing Science Thompson Rivers University
Course Objectives • The better knowledge of computer systems, the better programing. Data Representation
Course Contents • Introduction to computer systems: B&O 1 • Introduction to C programming: K&R 1 – 4 • Data representations: B&O 2.1 – 2.4 • C: advanced topics: K&R 5.1 – 5.10, 6 – 7 • Introduction to IA32 (Intel Architecture 32): B&O 3.1 – 3.8, 3.13 • Compiling, linking, loading, and executing: B&O 7 (except 7.12) • Dynamic memory management – Heap: B&O 9.9.1 – 9.9.2, 9.9.4 – 9.9.5, 9.11 • Code optimization: B&O 5.1 – 5.6, 5.13 • Memory hierarchy, locality, caching: B&O 5.12, 6.1 – 6.3, 6.4.1 – 6.4.2, 6.5, 6.6.2 – 6.6.3, 6.7 • Virtual memory (if time permits): B&O 9.4 – 9.5 Data Representation
Unit Learning Objectives • Convert a decimal number to binary number. • Convert a decimal number to hexadecimal number. • Convert a binary number to decimal number. • Convert a binary number to hexadecimal number. • Convert a hexadecimal number to binary number . • Distinguish little endian byte order and big endian byte order. • Compute binary addition. • Compute binary subtraction using 2’s complement. • Determine the 2’s complement representation of a signed integer. • Understand the overflow of unsigned integers and signed integers. • Trace and fix faulty code. Data Representation
Add two binary numbers. • Compute the 1’s complement of a binary number. • Compute the 2’s complement of a binary number. • Understand the 2’s complement representation for negative integers. • Subtract a binary number by using the 2’s complement addition. • Multiply two binary numbers. • Use of left shift and right shift. • Binary division Data Representation
Unit Contents • Information Storage • Integer Representations • Integer Arithmetic • Floating Point Data Representation
1. Information Storage • Virtual memory, address, and virtual address space • Virtual address space is a conceptual image presented to the machine-level program. • Partitioned into more manageable units to store the different program objects, i.e., instructions, program data, and control information. • The actual machine level program simply treats each program object as a block of bytes, and the program itself as a sequence of bytes. • Example • int number = 28; • 4 bytes, i.e., 32 bits, will be allocated to the variable number. • The decimal number 28 will be stored in the 32 bits? How? • Binary number, not the decimal, 00000000 00000000 00000000 00011100 will be stored in the 32 bits. • Do programmers have to convert 28 to it’s binary number? Data Representation
The Decimal System • Uses 10 digits 0, 1, 2, ..., 9. • Decimal expansion: • 83 = 8×10 + 3 • 4728 = 4×103 + 7×102 + 2×101 + 8×100 • 84037 = ??? • 43.087 = ??? • Do you know addition, subtraction, multiplication and division? • 1234 + 435.78 • 1234 – 435.78 • 1234 × 435.78 • 1234 / 435.78 Number Systems
The Binary System • In computer systems, the most basic memory unit is a bit that contains 0 and 1. • The data unit of 8 bits is referred as a byte that is the basic memory unit used in main memories and hard disks. • All data are represented by using binary numbers. Data types such as text, voice, image and video have no meaning in the data representation. • 8 bits are usually used to express English alphabets. • A collection of nbits has 2npossible states. Is it true? • E.g., • How many different numbers can you express using 2 bits? • How many different numbers can you express using 4 bits? • How many different numbers can you express using 8 bits? • How many different numbers can you express using 32 bits? Number Systems
How can we store integers(i.e., positive numbers only) in a computer? • E.g., • A decimal number 329? • Is it okay to store 3 characters ‘3’, ‘2’, and ‘9’ for 329? • How are characters stored? • 32910 = ???2 Number Systems
Uses two digits 0 and 1. How to expand binary numbers? • 02 = 0×20 = 010 • 12 = 1×20 = 110 • 102 = 1×21 + 0×20 = 210 • 112 = 1×21 + 1×20 = 310 • 1002 = 1×22 + 0×21 + 0×20 = 410 • 1012 = 1×22 + 0×21 + 1×20 = 510 • 1102 = 1×22 + 1×21 + 0×20 = 610 • 1112 = 1×22 + 1×21 + 1×20 = 710 • 10002 = 1×23 + 0×22 + 0×21 + 0×20 = 810 • 10012 = 1×23 + 0×22 + 0×21 + 1×20 = 910 • ... Number Systems
Powers of 2 • 12 = 1×20 = 110 • 102 = 1×21 + 0×20 = 210 • 1002 = 1×22 + 0×21 + 0×20 = 410 • 10002 = 1×23 + 0×22 + 0×21 + 0×20 = 810 • 1 00002 = 1610 • 10 00002 = 3210 • 100 00002 = 6410 Number Systems
1000 00002 = ???10 • 1 0000 00002 = ???10 • 10 0000 00002 = ???10 • 100 0000 00002 = ???10 • 1000 0000 00002 = ???10 • 1 0000 0000 00002 = ???10 • Can you memorize the above powers of 2? • Converting to decimals • 11012 = ???10 • 1011 00102 = ???10 • 1011.00102 = ???10 Number Systems
Converting Decimal to Binary Quotient Remainder • 21 / 2 10 1 10 / 2 5 0 5 / 2 2 1 2 / 2 1 0 1 / 2 0 1 => 2110 = 1 01012 • 27110 = ???2 • 607110 = ???2 Number Systems
Another similar idea • 27110 = ???2 256 < 271 < 512 -> 271 = 256 + 15 = 1 0000 00002 + 15 8< 15 < 16 -> 15 = 8 + 7 = 10002 + 7 => 271 = 1 0000 00002 + 15 = 1 0000 00002 + 10002 + 7 = 1 0000 00002 + 10002 + 1112 = 1 0000 11112 • 127110 = ???2 Number Systems
Hexadecimal Number System • 010 = 00002 = 016 = 0x0 • 110 = 00012 = 116 = 0x1 • 210 = 00102 = 216 = 0x2 • 310 = 00112 = 316 = 0x3 • 410 = 01002 = 416 = 0x4 • 510 = 01012 = 516 = 0x5 • 610 = 01102 = 616 = 0x6 • 710 = 01112 = 716 = 0x7 • 810 = 10002 = 816 = 0x8 • 910 = 10012 = 916 = 0x9 • 1010 = 10102 = A16 = 0xA • 1110 = 10112 = B16 = 0xB • 1210 = 11002 = C16 = 0xC • 1310 = 11012 = D16 = 0xD • 1410 = 11102 = E16 = 0xE • 1510 = 11112 = F16 = 0xF • 14816= ???1014816= ???2 • 23c9d6ef = ???1023c9d6ef = ???2 4 bitscan be used for a hexadecimal number, 0, ..., F. Please memorize it! Number Systems
Converting Decimal to Hexadecimal Quotient Remainder • 328 / 16 = 20 × 16 + 8 20 / 16 = 1 × 16 + 4 1 / 16 = 0 × 16 + 1 => 32810 = 14816 = ???2 • 14816 = (1 × 162 + 4 × 161 + 8 × 160)10 • 19210 = ???16 Number Systems
Converting Binary to Hexadecimal • 4DA916= ???2 • 1001101101010012 = ???16 = 100 1101 1010 1001 = 4DA9 • 10 11102 = 0x??? = ???10 • 0100 1110 1011 1001 01002 = 0x??? = ???10 Number Systems
Format specifiers in printf() for hexadecimal and decimal numbers? for (i = 0; i < num; i++) printf(“%d = 0x%x\n”, data[i], data[i]); • But there is no printf format specifier to print an integer in the binary form. • Write a function to make a string of 0’s and 1’s for a given integer. Data Representation
Words • A word size – the nominal size of integer and pointer data. A pointer variable contains an address in the virtual address space. We will discuss pointer variable in the next unit. • The word size determines the maximum size of the virtual address space. • 32bit operating systems? • 64bit operating systems? Data Representation
Data Sizes • printf(“%lu\n”, sizeof(long)); //lu: long unsigned // integer Data Representation
Addressing and Byte Ordering • A variable x of type int • Address of x: 0x100 • This means the 4 bytes of x would be stored in memory locations 0x100, 0x101, 0x102, and 0x103. • How to interpret the bytes in memory locations 0x100, 0x101, 0x102, and 0x103? • Let’s assume x has 0x1234567. There are two conventions to store the values in the 4 consecutive byte memory locations. 0x01, 0x23, 0x45, and 0x67, or 0x67, 0x45, 0x23, and 0x01, depending on CPU architecture. • Little endian byte order – Intel-compatible machines 0x103 0x102 0x101 0x100 address 0x01 0x23 0x45 0x67 value • Big endian byte order – machines from IBM and Sun Microsystems 0x103 0x102 0x101 0x100 0x67 0x45 0x23 0x01 Data Representation
The byte orderings are totally invisible for most application programmers. • Why are the byte orderings important? • Think of data exchange between two machines through a network. • Assembly programming • When type casting is used Data Representation
Representing Strings • A string is encoded by an array of characters terminated by the null (having 0) character ‘\0’; • The ASCII character set • Unicode – Some libraries are available for C. Data Representation
Representing Code • Different machine types use different and incompatible instructions and encodings. • Even identical processors running different OSes have differences in their coding conventions and hence are no binary compatible. Data Representation
Comparison and Logical Operations • Comparison operators ??? • Logical operators ??? Data Representation
Bit-Level Operations • ??? • &, |, ~, ^, >>, << • >>: Logical right shift and arithmetic right shift. • Logical right shit for unsigned integers: filled with 0 • Arithmetic right shit for signed integers: filled with the MSB • Examples char x = -128; unsigned char y = 128; x = x >> 1; y = y >> 1; printf (“%d, %d\n”, x, y); • We will discuss about this example later again. • Some examples a ^ a = ??? a ^ 0 = ??? x = 10; y = 20; y = x ^ y; x = x ^ y; y = x ^ y; Data Representation
Some more examples 11110000 & 11001100 = ??? 11110000 | 11001100 = ??? 11110000 ^ 11001100 = ??? 0x3B & 0x33 = ??? 0x3B | 0x33 = ??? 0x3B ^ 0x33 = ??? 0x3B >> 2 = ??? 0x33 << 2 = ??? • Consult with programming assignments Data Representation
2. Integer Representations C Java Size char, unsigned char byte 1B short, unsigned short short 2Bs char in Java uses 2Bs. int, unsigned int int 4Bs long, unsigned long long 8Bs // there is no unsigned in Java float float 4Bs double double 8Bs Data Representation
Unsigned Encodings • unsigned char All 8 bits are used. No sign bit. • The smallest number is 0 • The maximum number is 0xff. • unsigned short 16 bits • The smallest number is ??? • The maximum number is ??? • unsigned int 32 bits • The smallest number is ??? • The maximum number is ??? • unsigned long 64 bits • The smallest number is ??? • The maximum number is ??? Data Representations
Representation of Unsigned Integers • 8-bit representation of unsigned char 255 11111111 254 11111110 ... ... 128 10000000 127 01111111 126 01111110 ... ... 2 00000010 1 00000001 0 00000000 • The maximum number is ? • The minimum number is ? • What if we add the maximum number by 1 ??? • What if we subtract the minimum number by 1 ??? +1 +1 +1 overflow Data Representations
unsigned char x, y; x = 128; y = 128; printf(“x = %d, y = %d\n”, x, y); printf(“x + y = %d\n”, x + y); // int (not char) addition x = x + y; // 256 -> 100000000 -> truncation printf(“x = %d, y = %d\n”, x, y); X = 128; x = x >> 1; // logical right shift for unsigned printf(“x = %d\n”, x); • The output is ??? • 128, 128 256 0, 128 64 • 16-bit, 32-bit, 64-bit representations have the same overflowproblem. • How to represent signed integers? Data Representation
Binary Addition • We will discuss binary addition and binary subtraction, before we discuss the representation of signed integers. • How to add two binary numbers? Let’s consider only unsigned integers(i.e., positive numbers only) for a while. • Just like the addition of two decimal numbers. • E.g., 10010 10010 1111 + 1001 + 1011 + 1 11011 11101 ??? 10111 + 111 ??? carry Data Representations
Binary Subtraction • How to subtract a binary number? • Just like the subtraction of decimal numbers. • E.g., 0112 02 02 1000 10 10 10010 10010 10010 -1-1 -11 -11 -11 1 ?1 ?11 1111 Try: 101010 How to do? 1 -101-10 Data Representations
In the previous slide, 10010 – 11 = 1111 • What if we add 00010010 + 11111100 1 00001110 + 1 00001111 • Is there any relationship between 112 and 111002? • The 1’s complement of 112 is ??? Switching 0 1 • This type of addition is called 1’s complement addition. • Find the 8-bit one’s complements of the followings. • 11011 -> 00011011 -> • 10 -> 00000010 -> • 101 -> 00000101 -> Data Representations
In the previous slide, 10010 – 11 = 1111 • What if we add 00010010 + 11111101 1 00001111 • Is there any relationship between 11 and 11101? • The 2’s complement of 11 is ??? • 2’s complement ≡ 1’s complement + 1 -> 11100 + 1 = 11101 • This type of addition is called 2’s complement addition. • Find the 16-bit two’s complements of the followings. • 11011 -> 0000000000011011 -> • 10 • 101 Data Representations
Another example 101010 - 101 ??? • What if we use 1’s complement addition or 2’s complement addition instead as follow? Let’s use 8-bit representation. 00101010 00101010 + 11111010+ 11111011 1 00100100 1 00100101 + 1 00100101 • What does this mean? • A – B = A + (–B), where A and B are positive • Is the 1’s complement or the 2’s complement of B sort of equal to –B? Data Representations
Can we use 8-bit 1’s complement addition for 12 – 102 = –12? 1 00000001 - 10+ 11111101<- 8-bit 1’s complement of 10 11111110 <- Is this correct? (1’s complement of 1?) • Let’s use 8-bit 2’s complement addition for 12 – 102. 00000001 +11111110<- 2’s complement of 10 11111111 <- Correct? (2’s complement of 1?) • 12 – 102 = 12 + (–102) = –12 • How to represent negative binary numbers, i.e., signed integers? Data Representations
Representation of Negative Binaries • Representation of signed integers • 8 or 16 or 32 bits are usually used for integers. • Let’s use 8 bits for examples. • The left most bit (called most significant bit) is used as sign. • When the MSB is 0, non-negative integers. • When the MSB is 1, negative integers. • The other 7 bits are used for integers. • How to represent positive integer 9? • 00001001 • How about -9? • 10001001 is really okay? • 00001001 (9) + 10001001 (-9) = 10010010 (-18) It is wrong! • We need a different representation for negative integers. Data Representations
How about -9? • 10001001 is really okay? • 00001001 (9) + 10001001 (-9) = 10010010 (-18) It is wrong! • We need a different representation for negative integers. • What is the 8-bit 1’s complement of 9? • 11110110 <- 8-bit 1’s complement of 9 • 00001001 + 11110110 <- 9 + 8-bit 1’s complement of 9 = 11111111 <- Is it zero? (1’s complement of 0?) • What is the 2’s complement of 9? • 11110111 <- 8-bit 2’s complement of 9 • 00001001 + 11110111 <- 9 + 8-bit 2’s complement of 9 = 1 00000000 <- It looks like zero. • 2’s complement representation is used for negative integers. Data Representations
12 – 102 = 12 + (–102) ??? What is the result in decimal? 00000001 + 11111110<- 2’s complement of 10, i.e., -102 11111111 <- 2’s complement of 1, i.e., -1 (= 1 – 2) • 1010102 – 1012 = 1010102 + (–1012) ??? • 100102 – 112 ??? • 102 – 12 ??? • -102 – 12 ??? • Is the two’s complement of the two’s complement of an integer the same integer? • What is x when the 8-bit 2’s complement of x is • 11111111 11110011 10000001 Data Representations
8-bit representation of signed char with 2’s complement 127 01111111 126 01111110 ... ... 2 00000010 1 00000001 0 00000000 -1 11111111 -2 11111110 -3 11111101 ... ... -127 10000001 -128 10000000 • The maximum number is ? • The minimum number is ? • What if we add the maximum number by 1 ??? • What if we subtract the minimum number by 1 ??? overflow +1 +1 -1 overflow +1 -1 -1 Data Representations
16-bit representation signed short with 2’s complement ... 01111111 11111111 ... 01111111 11111110 ... ... 3 00000000 00000011 2 00000000 00000010 1 00000000 00000001 0 00000000 00000000 -1 11111111 11111111 -2 11111111 11111110 -3 11111111 11111101 ... ... ... 10000000 00000001 ... 10000000 00000000 • The maximum number is ? What if we add the maximum number by 1 ??? • The minimum number is ? What if we subtract the minimum number by 1 ??? overflow +1 +1 +1 -1 -1 -1 overflow Data Representations
Note that computers use the 8-bit representation, the 16-bit representation, the 32-bit representation and the 64-bit representation with 2’complement for negative integers. • In programming languages • char, unsigned char 8-bits • short, unsigned short 16-bits • int, unsigned int 32-bits • long, unsigned long 64-bits • When we use the 32-bit representation with 2’s complement, • The maximum number is ? • What if we add the maximum number by 1 ??? • The minimum number is ? • What if we subtract the minimum number by 1 ??? Data Representations
Now we know how to represent negative integers. • 2’ complement addtion A + (–B) is computed for subtraction A – B. • Let’s suppose B is negative. Then –B is really a positive integer? For example, let’s consider 1 byte signed integer. 127 01111111 126 01111110 ... ... 2 00000010 1 00000001 0 00000000 -1 11111111 -2 11111110 -3 11111101 2’s complement of -3 is 00000011, i.e., 3. ... ... -127 10000001 -128 10000000 2’s complement of -128 is 10000000 again. • For any -127 < x < 127, x – x = 0. But (-128) – (-128) = ??? Data Representation
char x, y; x = 128; // 128 -> 10000000 This is -128. y = 128; printf(“x = %d, y = %d\n”, x, y); printf(“x – y = %d\n”, x - y); x = x – y; printf(“x = %d, y = %d\n”, x, y); x = 128; x = x >> 1; // arithmetic shift for signed printf(“x = %d\n”, x); • The output is ??? • -128, -128 0 0, -128 -64 • -128 – (-128) = -128 + (-(-128)) = -128 + (-128) = 10000000 + 10000000 = 00000000 = 0 Data Representation
char a = 127, b = 127, c; unsigned char d; c = a + b; d = a + b; printf(“a=%d, b=%d, c=%d, d=%d\n”, a, b, c, d); • The output is ??? • a=127, b=127, c=-2, d=254 01111111 + 01111111 = 11111110 ??? • We have to be very careful with overflow for integer values. Data Representation
Advice on Signed vs. Unsigned • Practice Problem 2.25 float sum_elements (float a[], unsigned int length) { int i; float result = 0; for (i = 0; i <= length-1; i++) result += a[i]; return result; } • When run with length equal to 0, this code should return 0.0. Instead it encounters a memory error. Why??? • unsigned int length length-1 • How to fix this code? Data Representations
short x = -5; unsigned short y = 128; printf(“%x, %x\n”, x, y); printf(“%x, %x, %x, %x\n”, x<<3, x>>3, y<<3, y>>3); The output is ??? fffffffb, 80 ffffffd8, ffffffff, 400, 10 • Singed integers: • Arithmetic right shift: Left part is filled with the most significant bit of the original value. • Unsigned integers: • Logical right shift: Left part is filled with 0. Data Representations
Practice Problem 2.26 size_t strlen(const char* s); // defined in string.h int strlonger(char s[], char t[]) { return strlen(s) – strlen(t) > 0; } Note that size_t is defined in stdio.h to be unsigned int. • For what cases will this function produce an incorrect result? • 0 – 1 > 0 -> 1 (true value) • Explain how this incorrect result comes about. • Unsigned int of 0 – 1 is 0xffffffff that is greater than 0. • Show how to fix the code so that it will work reliably. Data Representations
- More by User
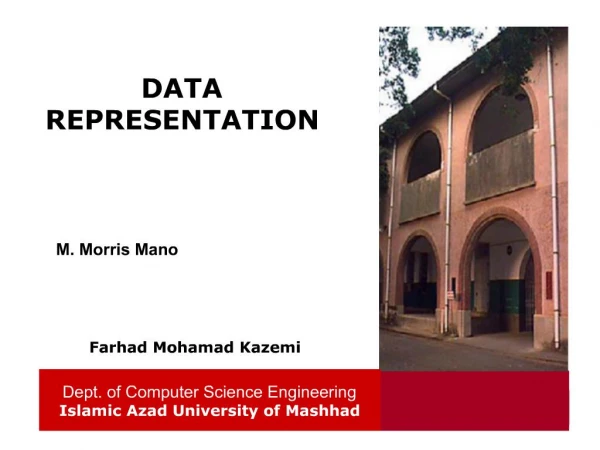
DATA REPRESENTATION
2. OUTLINES. Data TypesComplementsFixed Point RepresentationsOther Binary CodesError Detection Codes. 3. Data Types. Information that a Computer is dealing withDataNumeric DataNumbers( Integer, real)Non-numeric DataLetters, SymbolsRelationship between data elementsData StructuresLinear L
462 views • 24 slides
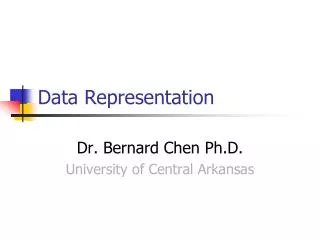
Data Representation. Dr. Bernard Chen Ph.D. University of Central Arkansas. Outline. Data Representation Compliments Subtraction of Unsigned Numbers using r’s complement How To Represent Signed Numbers Floating-Point Representation. Data Types.
1.18k views • 73 slides
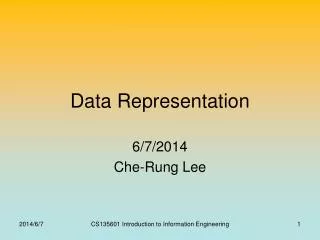
Data Representation. 6/7/2014 Che-Rung Lee. Two different worlds. 01100001,01100010,01100011. 00000001,00000010,00000011. 01001100010101000110100…. 10001001010100000100111. 00110000001001101011001…. Binary system. Computers uses 0 and 1 to represent and store all kinds of data.
1.04k views • 85 slides
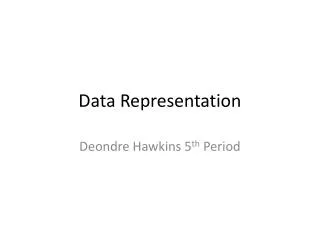
Data Representation. Deondre Hawkins 5 th Period.
148 views • 6 slides
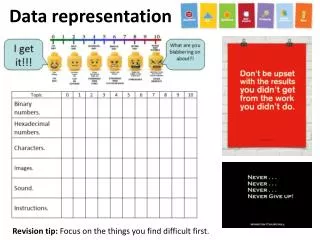
Data representation
Data representation. Revision tip: Focus on the things you find difficult first. “Every lesson counts – don’t waste a minute.”. Exam tip: When converting binary numbers in the exam, draw a table to show your workings. Binary numbers (base 2). Convert 10110 to denary (base 10) (1 mark).
438 views • 17 slides
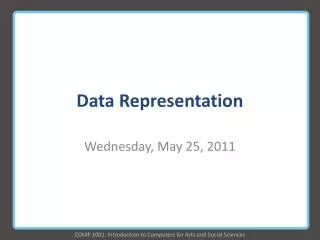
Data Representation. Wednesday, May 25, 2011. Bits and Bytes. 4 bytes = 32 bits → 1 integer = a number up to 4,294,967,296. ASCII Code. ASCII Code. Text Compression. The Rain Pitter patter Pitter patter Listen to the rain Pitter patter Pitter patter On the window pane.
479 views • 27 slides
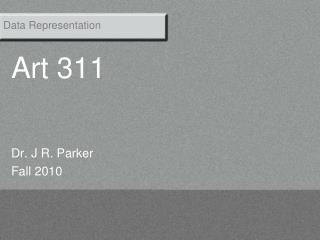
Data Representation. Art 311 Dr. J R. Parker Fall 2010. Data Representation. The basic question today is: “Given that a computer only manipulates numbers, how can we represent interesting things like images, sounds, graphics, text, video, and so on”?
688 views • 55 slides
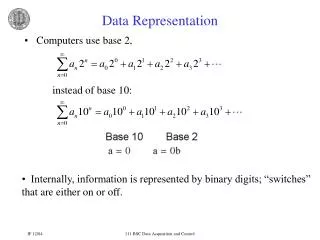
Data Representation. Computers use base 2,. instead of base 10:. Internally, information is represented by binary digits; “switches” that are either on or off. Digital information must be converted to analog signals: DAC: Digital to Analog Converter. Computer. Computer. Data Converters.
500 views • 38 slides
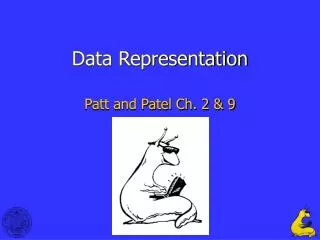
Data Representation. Patt and Patel Ch. 2 & 9. Data Representation. Goal: Store numbers, characters, sets, database records in the computer. What we got: Circuit that stores 2 voltages, one for logic 0 (0 volts) and one for logic 1 (ex: 3.3 volts).
585 views • 43 slides
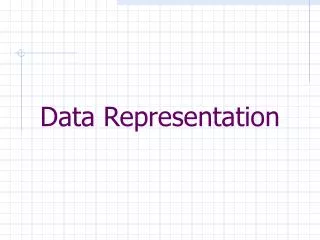
Data Representation. Data Representation. Goal: Store numbers, characters, sets, database records in the computer. What we got: Circuit that stores 2 voltages, one for logic 0 (0 volts) and one for logic 1 (3.3 volts). DRAM – uses a single capacitor to store and a transistor to select.
438 views • 28 slides
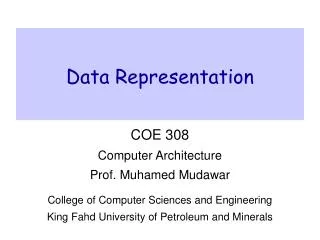
Data Representation. COE 308 Computer Architecture Prof. Muhamed Mudawar College of Computer Sciences and Engineering King Fahd University of Petroleum and Minerals. Presentation Outline. Positional Number Systems Binary and Hexadecimal Numbers Base Conversions Integer Storage Sizes
627 views • 28 slides
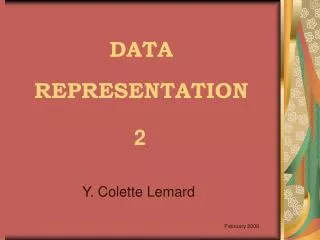
DATA REPRESENTATION. 2. Y. Colette Lemard. February 2009. TYPES OF NUMBER REPRESENTATION. There are two major classes of number representation :- FIXED POINT FLOATING POINT. FIXED POINT SYSTEMS. Most of us are familiar with fixed representation to some extent
985 views • 67 slides
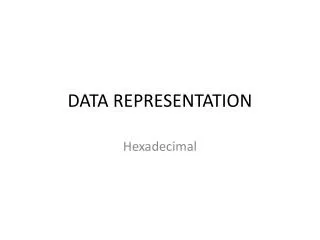
DATA REPRESENTATION. Hexadecimal. Review. So far we have covered: Binary Number Representation Why Binary is used in computers Binary to Denary & Denary to Binary conversion Integers and numbers with a fractional part. Representing SIGNED Integers by Two’s Complement.
157 views • 4 slides
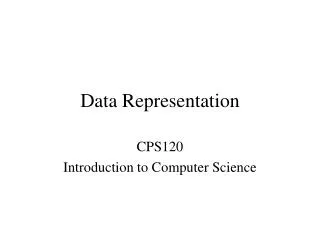
Data Representation. CPS120 Introduction to Computer Science. Data and Computers. Computers are multimedia devices, dealing with a vast array of information categories. Computers store, present, and help us modify: Numbers Text Audio Images and graphics Video. Data Representation.
667 views • 49 slides
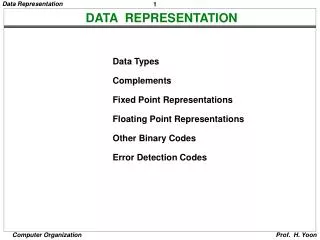
DATA REPRESENTATION. Data Types Complements Fixed Point Representations Floating Point Representations Other Binary Codes Error Detection Codes. Data Types. DATA REPRESENTATION. Information that a Computer is dealing with * Data - Numeric Data
522 views • 30 slides
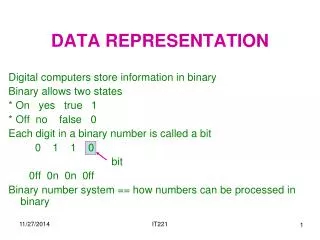
DATA REPRESENTATION. Digital computers store information in binary Binary allows two states * On yes true 1 * Off no false 0 Each digit in a binary number is called a bit 0 1 1 0 bit 0ff 0n 0n 0ff
1.33k views • 74 slides
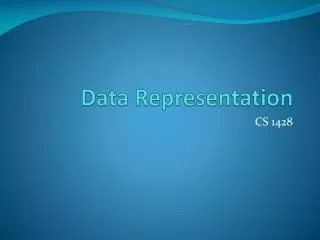
Data Representation. CS 1428. Binary Representation of Information. Detecting Voltage Levels Why not 10 levels? Would be unreliable Not enough difference between states On/Off Fully Charged - Fully Discharged Magnetized - Demagnetized. Bits, Bytes, and so on. A bit is one 0 or 1
265 views • 17 slides
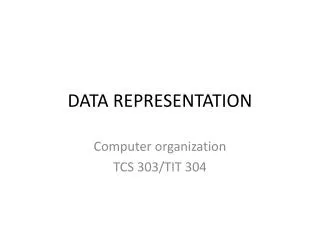
DATA REPRESENTATION. Computer organization TCS 303/TIT 304. I ntroduction A bit is the most basic unit of information in a computer. It is a state of “on” or “off” in a digital circuit. Or “high” or “low” voltage instead of “on” or “off.” A byte is a group of eight bits.
396 views • 28 slides
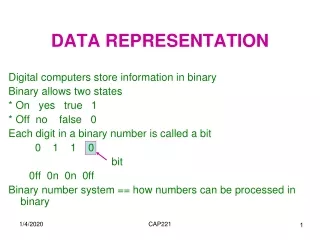
750 views • 74 slides
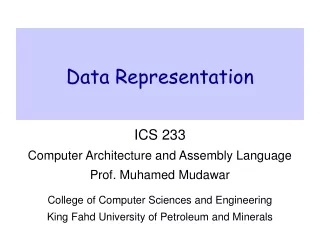
Data Representation. ICS 233 Computer Architecture and Assembly Language Prof. Muhamed Mudawar College of Computer Sciences and Engineering King Fahd University of Petroleum and Minerals. Presentation Outline. Positional Number Systems Binary and Hexadecimal Numbers Base Conversions
288 views • 28 slides
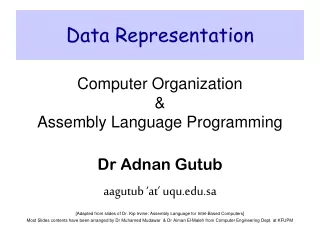
Data Representation. Computer Organization & Assembly Language Programming Dr Adnan Gutub aagutub ‘at’ uqu.edu.sa [Adapted from slides of Dr. Kip Irvine: Assembly Language for Intel-Based Computers]
335 views • 33 slides
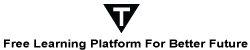
- Computer Fundamentals
Computer Network
Control System
- Interview Q
COA Tutorial
Basic co and design, computer instructions, digital logic circuits, map simplification, combinational circuits, flip - flops, digital components, register transfer, micro-operations, memory organization.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Data Mining

Data Warehouse

If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
Computers and the Internet
Course: computers and the internet > unit 1, how do computers represent data.
- Binary & data
- Bits (binary digits)

Want to join the conversation?
- Upvote Button navigates to signup page
- Downvote Button navigates to signup page
- Flag Button navigates to signup page

Computer Science & Engineering Department (Semester – 4)
Computer organization & architecture (3140707) – lecture ppt.
1. Unit – 1 Computer Data Representation
2. Unit – 2 Basic Computer Organization and Design
3. Unit – 3 Assembly Language Programming
4. Unit – 4 Micro programmed Control Organization
5. Unit – 5 Central Processing Unit
6. Unit – 6 Pipeline And Vector Processing
7. Unit – 7 Computer Arithmetic
8. Unit – 8 Input-Output Organization
9. Unit – 9 Memory Organization
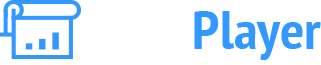
- My presentations
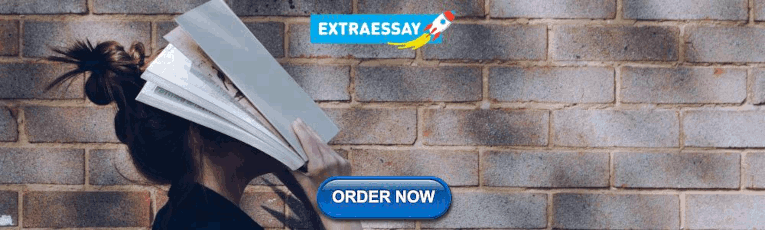
Auth with social network:
Download presentation
We think you have liked this presentation. If you wish to download it, please recommend it to your friends in any social system. Share buttons are a little bit lower. Thank you!
Presentation is loading. Please wait.
Data Representation.
Published by Adam Lucas Modified over 5 years ago
Similar presentations
Presentation on theme: "Data Representation."— Presentation transcript:
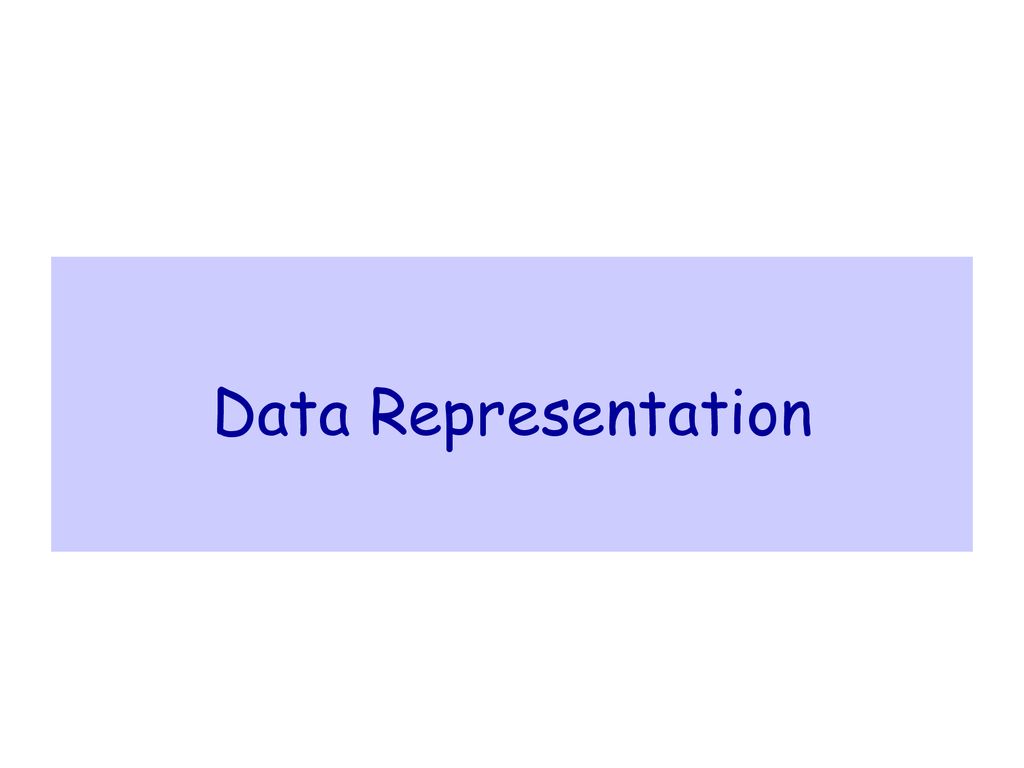
Assembly Language for Intel-Based Computers, 4 th Edition Chapter 1: Basic Concepts (c) Pearson Education, All rights reserved. You may modify and.
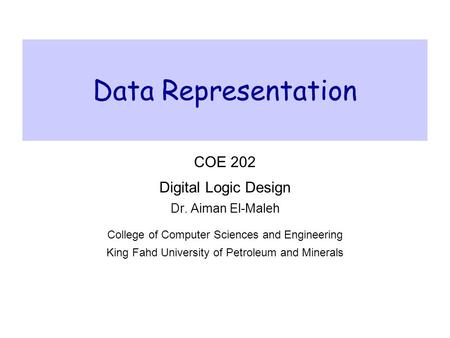
Data Representation COE 202 Digital Logic Design Dr. Aiman El-Maleh
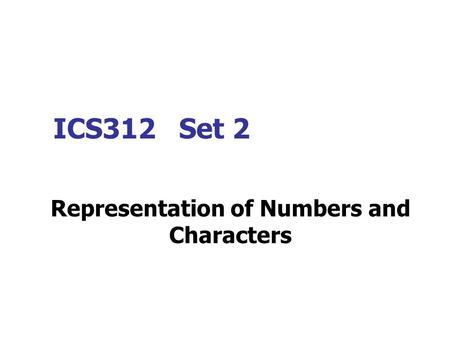
ICS312 Set 2 Representation of Numbers and Characters.
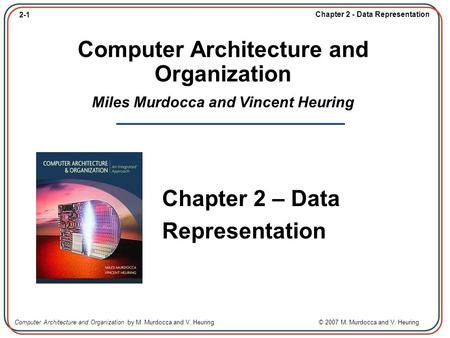
2-1 Chapter 2 - Data Representation Computer Architecture and Organization by M. Murdocca and V. Heuring © 2007 M. Murdocca and V. Heuring Computer Architecture.
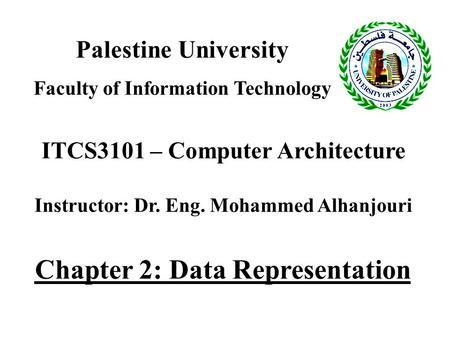
Chapter 2: Data Representation
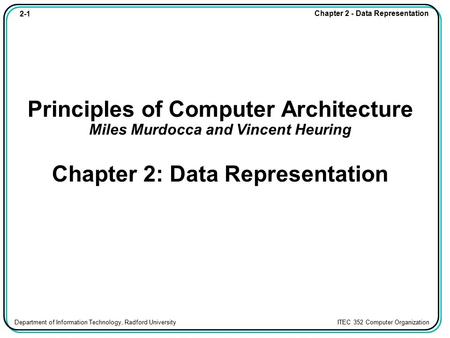
Principles of Computer Architecture Miles Murdocca and Vincent Heuring Chapter 2: Data Representation.
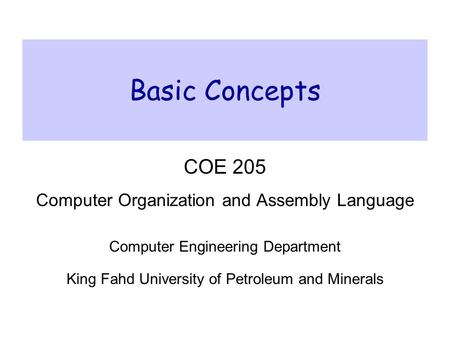
Basic Concepts COE 205 Computer Organization and Assembly Language
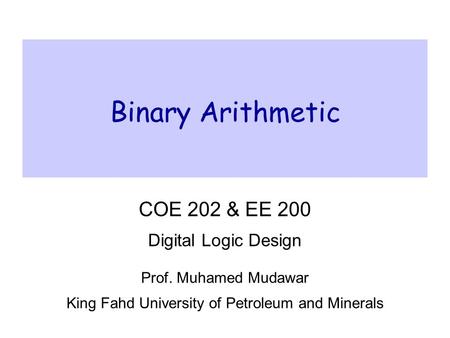
King Fahd University of Petroleum and Minerals
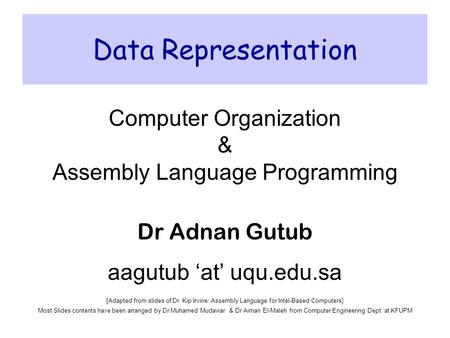
Data Representation Computer Organization &
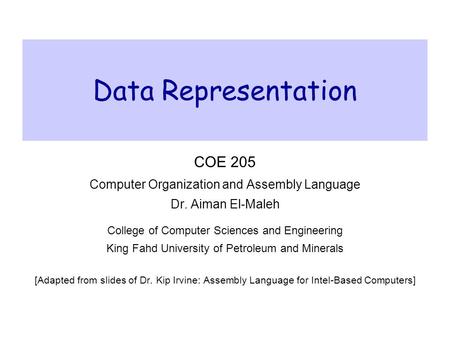
Data Representation COE 205
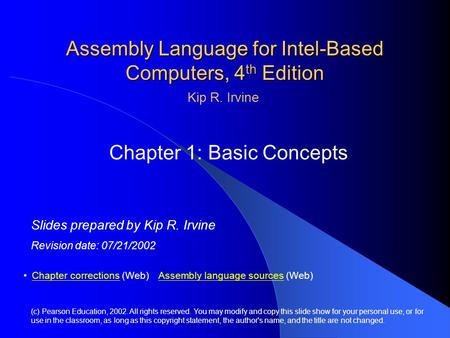
Assembly Language for Intel-Based Computers, 4th Edition
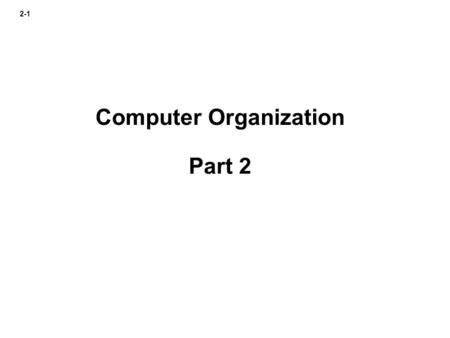
2-1 Computer Organization Part Fixed Point Numbers Using only two digits of precision for signed base 10 numbers, the range (interval between lowest.
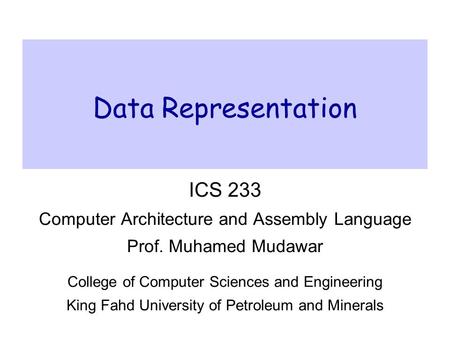
Data Representation ICS 233
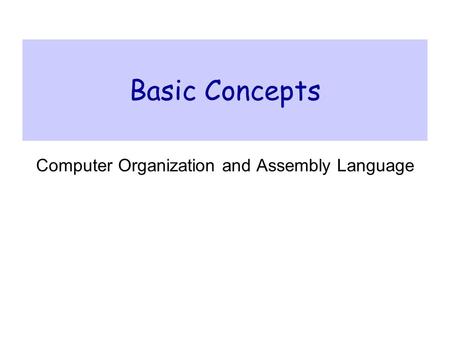
Basic Concepts Computer Organization and Assembly Language.
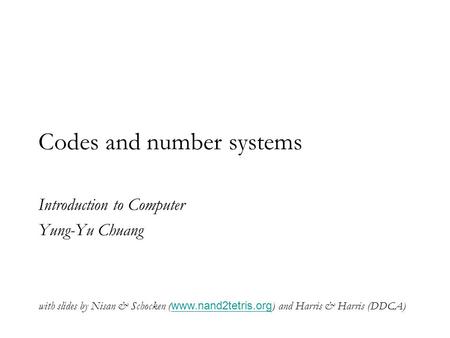
Codes and number systems Introduction to Computer Yung-Yu Chuang with slides by Nisan & Schocken ( ) and Harris & Harris (DDCA)
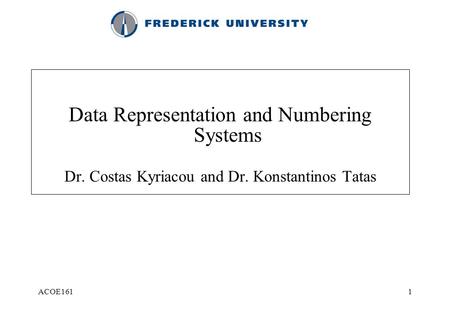
ACOE1611 Data Representation and Numbering Systems Dr. Costas Kyriacou and Dr. Konstantinos Tatas.
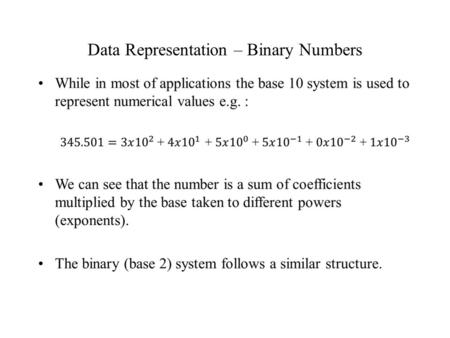
Data Representation – Binary Numbers
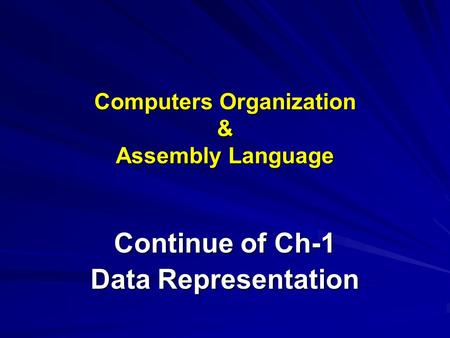
Computers Organization & Assembly Language
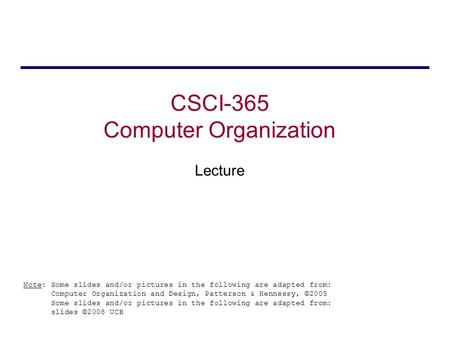
CSCI-365 Computer Organization Lecture Note: Some slides and/or pictures in the following are adapted from: Computer Organization and Design, Patterson.
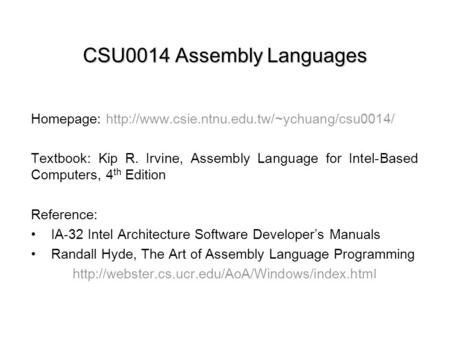
CSU0014 Assembly Languages Homepage: Textbook: Kip R. Irvine, Assembly Language for Intel-Based Computers,
About project
© 2024 SlidePlayer.com Inc. All rights reserved.
- Engineering Mathematics
- Discrete Mathematics
- Operating System
- Computer Networks
- Digital Logic and Design
- C Programming
- Data Structures
- Theory of Computation
- Compiler Design
- Computer Org and Architecture
Computer Organization and Architecture Tutorial
- Basic Computer Instructions
- What is Computer
- Issues in Computer Design
- Difference between assembly language and high level language
- Addressing Modes
- Difference between Memory based and Register based Addressing Modes
- Computer Organization | Von Neumann architecture
- Harvard Architecture
- Interaction of a Program with Hardware
- Simplified Instructional Computer (SIC)
- Instruction Set used in simplified instructional Computer (SIC)
- Instruction Set used in SIC/XE
- RISC and CISC in Computer Organization
- Vector processor classification
- Essential Registers for Instruction Execution
- Introduction of Single Accumulator based CPU organization
- Introduction of Stack based CPU Organization
- Machine Control Instructions in Microprocessor
- Very Long Instruction Word (VLIW) Architecture
- Input and Output Systems
- Computer Organization | Different Instruction Cycles
- Machine Instructions
- Computer Organization | Instruction Formats (Zero, One, Two and Three Address Instruction)
- Difference between 2-address instruction and 1-address instructions
- Difference between 3-address instruction and 0-address instruction
- Register content and Flag status after Instructions
- Debugging a machine level program
- Vector Instruction Format in Vector Processors
- Vector instruction types
- Instruction Design and Format
- Introduction of ALU and Data Path
- Computer Arithmetic | Set - 1
- Computer Arithmetic | Set - 2
- Difference between 1's Complement representation and 2's Complement representation Technique
- Restoring Division Algorithm For Unsigned Integer
- Non-Restoring Division For Unsigned Integer
- Computer Organization | Booth's Algorithm
- How the negative numbers are stored in memory?
- Microprogrammed Control
- Computer Organization | Micro-Operation
- Microarchitecture and Instruction Set Architecture
- Types of Program Control Instructions
- Difference between CALL and JUMP instructions
- Computer Organization | Hardwired v/s Micro-programmed Control Unit
- Implementation of Micro Instructions Sequencer
- Performance of Computer in Computer Organization
- Introduction of Control Unit and its Design
- Computer Organization | Amdahl's law and its proof
- Subroutine, Subroutine nesting and Stack memory
- Different Types of RAM (Random Access Memory )
- Random Access Memory (RAM) and Read Only Memory (ROM)
- 2D and 2.5D Memory organization
Input and Output Organization
- Priority Interrupts | (S/W Polling and Daisy Chaining)
- I/O Interface (Interrupt and DMA Mode)
- Direct memory access with DMA controller 8257/8237
- Computer Organization | Asynchronous input output synchronization
- Programmable peripheral interface 8255
- Synchronous Data Transfer in Computer Organization
- Introduction of Input-Output Processor
- MPU Communication in Computer Organization
- Memory mapped I/O and Isolated I/O
- Memory Organization
- Introduction to memory and memory units
- Memory Hierarchy Design and its Characteristics
- Register Allocations in Code Generation
- Cache Memory
- Cache Organization | Set 1 (Introduction)
- Multilevel Cache Organisation
- Difference between RAM and ROM
- What's difference between CPU Cache and TLB?
- Introduction to Solid-State Drive (SSD)
- Read and Write operations in Memory
- Instruction Level Parallelism
- Computer Organization and Architecture | Pipelining | Set 1 (Execution, Stages and Throughput)
- Computer Organization and Architecture | Pipelining | Set 3 (Types and Stalling)
- Computer Organization and Architecture | Pipelining | Set 2 (Dependencies and Data Hazard)
- Last Minute Notes Computer Organization
COA GATE PYQ's AND COA Quiz
- Computer Organization and Architecture
- Digital Logic & Number representation
- Number Representation
- Microprocessor
- GATE CS Preparation
Computer Organization and Architecture is used to design computer systems. Computer Architecture is considered to be those attributes of a system that are visible to the user like addressing techniques, instruction sets, and bits used for data, and have a direct impact on the logic execution of a program, It defines the system in an abstract manner, It deals with What does the system do.
Whereas, Computer Organization is the way in which a system has to structure and It is operational units and the interconnections between them that achieve the architectural specifications, It is the realization of the abstract model, and It deals with How to implement the system.
In this Computer Organization and Architecture Tutorial, you’ll learn all the basic to advanced concepts like pipelining, microprogrammed control, computer architecture, instruction design, and format.
Recent Articles on Computer Organisation
- Computer Arithmetic
- Miscellaneous
- Quick Links
Basic Computer Instructions :
- A simple understanding of Computer
- Computer System Level Hierarchy
- Computer Architecture and Computer Organization
- Timing diagram of MOV Instruction in Microprocessor
- Assembly language and High level language
- Memory based Vs Register based addressing modes
- Von Neumann architecture
- Data Transfer instructions in AVR microcontroller
- Arithmetic instructions in AVR microcontroller
- Conditional Branch Instructions in AVR Microcontroller
- CALL Instructions and Stack in AVR Microcontroller
- Branch Instructions in AVR Microcontroller
- Logical Instructions in AVR Microcontroller
- Data Manipulation Instructions
Instruction Design and Format :
- Different Instruction Cycles
- Instruction Formats (Zero, One, Two and Three Address Instruction)
- 2-address instruction and 1-address instructions
- 3-address instruction and 0-address instruction
- 3-address instruction and 2-address instructions
Computer Arithmetic :
- Computer Arithmetic | ALU and Data Path
- Computer Arithmetic | Set 1
- Computer Arithmetic | Set 2
- Difference between 1’s complement and 2’s complement
- Booth’s Algorithm
Microprogrammed Control :
- Micro-Operation
- Hardwired v/s Micro-programmed Control Unit
Memory Organization :
- What’s difference between CPU Cache and TLB?
- Different Types of RAM
- Types of computer memory (RAM and ROM)
- Introduction to solid-state drive (SSD)
Input and Output Systems :
- Asynchronous input output synchronization
Pipelining :
- Execution, Stages and Throughput
- Types and Stalling
- Dependencies and Data Hazard
IEEE Number Statndards
Miscellaneous :
- Generations of computer
- Introduction to quantum computing
- Conventional Computing vs Quantum Computing
- Flynn’s taxonomy
- Clusters In Computer Organisation
- Program for Binary To Decimal Conversion
- Program for Decimal to Binary Conversion
- Program for decimal to octal conversion
- Program for octal to decimal conversion
- Program for hexadecimal to decimal
Quick Links :
- ‘Quizzes’ on Computer Organization and Architecture !
- ‘Practice Problems’ on Computer Organization and Architecture !
Please Login to comment...
Related articles, improve your coding skills with practice.
What kind of Experience do you want to share?
Page Statistics
Table of contents.
- Introduction to Functional Computer
- Fundamentals of Architectural Design
Data Representation
- Instruction Set Architecture : Instructions and Formats
- Instruction Set Architecture : Design Models
- Instruction Set Architecture : Addressing Modes
- Performance Measurements and Issues
- Computer Architecture Assessment 1
- Fixed Point Arithmetic : Addition and Subtraction
- Fixed Point Arithmetic : Multiplication
- Fixed Point Arithmetic : Division
- Floating Point Arithmetic
- Arithmetic Logic Unit Design
- CPU's Data Path
- CPU's Control Unit
- Control Unit Design
- Concepts of Pipelining
- Computer Architecture Assessment 2
- Pipeline Hazards
- Memory Characteristics and Organization
- Cache Memory
- Virtual Memory
- I/O Communication and I/O Controller
- Input/Output Data Transfer
- Direct Memory Access controller and I/O Processor
- CPU Interrupts and Interrupt Handling
- Computer Architecture Assessment 3
Course Computer Architecture
Digital computers store and process information in binary form as digital logic has only two values "1" and "0" or in other words "True or False" or also said as "ON or OFF". This system is called radix 2. We human generally deal with radix 10 i.e. decimal. As a matter of convenience there are many other representations like Octal (Radix 8), Hexadecimal (Radix 16), Binary coded decimal (BCD), Decimal etc.
Every computer's CPU has a width measured in terms of bits such as 8 bit CPU, 16 bit CPU, 32 bit CPU etc. Similarly, each memory location can store a fixed number of bits and is called memory width. Given the size of the CPU and Memory, it is for the programmer to handle his data representation. Most of the readers may be knowing that 4 bits form a Nibble, 8 bits form a byte. The word length is defined by the Instruction Set Architecture of the CPU. The word length may be equal to the width of the CPU.
The memory simply stores information as a binary pattern of 1's and 0's. It is to be interpreted as what the content of a memory location means. If the CPU is in the Fetch cycle, it interprets the fetched memory content to be instruction and decodes based on Instruction format. In the Execute cycle, the information from memory is considered as data. As a common man using a computer, we think computers handle English or other alphabets, special characters or numbers. A programmer considers memory content to be data types of the programming language he uses. Now recall figure 1.2 and 1.3 of chapter 1 to reinforce your thought that conversion happens from computer user interface to internal representation and storage.
- Data Representation in Computers
Information handled by a computer is classified as instruction and data. A broad overview of the internal representation of the information is illustrated in figure 3.1. No matter whether it is data in a numeric or non-numeric form or integer, everything is internally represented in Binary. It is up to the programmer to handle the interpretation of the binary pattern and this interpretation is called Data Representation . These data representation schemes are all standardized by international organizations.
Choice of Data representation to be used in a computer is decided by
- The number types to be represented (integer, real, signed, unsigned, etc.)
- Range of values likely to be represented (maximum and minimum to be represented)
- The Precision of the numbers i.e. maximum accuracy of representation (floating point single precision, double precision etc)
- If non-numeric i.e. character, character representation standard to be chosen. ASCII, EBCDIC, UTF are examples of character representation standards.
- The hardware support in terms of word width, instruction.
Before we go into the details, let us take an example of interpretation. Say a byte in Memory has value "0011 0001". Although there exists a possibility of so many interpretations as in figure 3.2, the program has only one interpretation as decided by the programmer and declared in the program.
- Fixed point Number Representation
Fixed point numbers are also known as whole numbers or Integers. The number of bits used in representing the integer also implies the maximum number that can be represented in the system hardware. However for the efficiency of storage and operations, one may choose to represent the integer with one Byte, two Bytes, Four bytes or more. This space allocation is translated from the definition used by the programmer while defining a variable as integer short or long and the Instruction Set Architecture.
In addition to the bit length definition for integers, we also have a choice to represent them as below:
- Unsigned Integer : A positive number including zero can be represented in this format. All the allotted bits are utilised in defining the number. So if one is using 8 bits to represent the unsigned integer, the range of values that can be represented is 28 i.e. "0" to "255". If 16 bits are used for representing then the range is 216 i.e. "0 to 65535".
- Signed Integer : In this format negative numbers, zero, and positive numbers can be represented. A sign bit indicates the magnitude direction as positive or negative. There are three possible representations for signed integer and these are Sign Magnitude format, 1's Compliment format and 2's Complement format .
Signed Integer – Sign Magnitude format: Most Significant Bit (MSB) is reserved for indicating the direction of the magnitude (value). A "0" on MSB means a positive number and a "1" on MSB means a negative number. If n bits are used for representation, n-1 bits indicate the absolute value of the number. Examples for n=8:
Examples for n=8:
0010 1111 = + 47 Decimal (Positive number)
1010 1111 = - 47 Decimal (Negative Number)
0111 1110 = +126 (Positive number)
1111 1110 = -126 (Negative Number)
0000 0000 = + 0 (Postive Number)
1000 0000 = - 0 (Negative Number)
Although this method is easy to understand, Sign Magnitude representation has several shortcomings like
- Zero can be represented in two ways causing redundancy and confusion.
- The total range for magnitude representation is limited to 2n-1, although n bits were accounted.
- The separate sign bit makes the addition and subtraction more complicated. Also, comparing two numbers is not straightforward.
Signed Integer – 1’s Complement format: In this format too, MSB is reserved as the sign bit. But the difference is in representing the Magnitude part of the value for negative numbers (magnitude) is inversed and hence called 1’s Complement form. The positive numbers are represented as it is in binary. Let us see some examples to better our understanding.
1101 0000 = - 47 Decimal (Negative Number)
1000 0001 = -126 (Negative Number)
1111 1111 = - 0 (Negative Number)
- Converting a given binary number to its 2's complement form
Step 1 . -x = x' + 1 where x' is the one's complement of x.
Step 2 Extend the data width of the number, fill up with sign extension i.e. MSB bit is used to fill the bits.
Example: -47 decimal over 8bit representation
As you can see zero is not getting represented with redundancy. There is only one way of representing zero. The other problem of the complexity of the arithmetic operation is also eliminated in 2’s complement representation. Subtraction is done as Addition.
More exercises on number conversion are left to the self-interest of readers.
- Floating Point Number system
The maximum number at best represented as a whole number is 2 n . In the Scientific world, we do come across numbers like Mass of an Electron is 9.10939 x 10-31 Kg. Velocity of light is 2.99792458 x 108 m/s. Imagine to write the number in a piece of paper without exponent and converting into binary for computer representation. Sure you are tired!!. It makes no sense to write a number in non- readable form or non- processible form. Hence we write such large or small numbers using exponent and mantissa. This is said to be Floating Point representation or real number representation. he real number system could have infinite values between 0 and 1.
Representation in computer
Unlike the two's complement representation for integer numbers, Floating Point number uses Sign and Magnitude representation for both mantissa and exponent . In the number 9.10939 x 1031, in decimal form, +31 is Exponent, 9.10939 is known as Fraction . Mantissa, Significand and fraction are synonymously used terms. In the computer, the representation is binary and the binary point is not fixed. For example, a number, say, 23.345 can be written as 2.3345 x 101 or 0.23345 x 102 or 2334.5 x 10-2. The representation 2.3345 x 101 is said to be in normalised form.
Floating-point numbers usually use multiple words in memory as we need to allot a sign bit, few bits for exponent and many bits for mantissa. There are standards for such allocation which we will see sooner.
- IEEE 754 Floating Point Representation
We have two standards known as Single Precision and Double Precision from IEEE. These standards enable portability among different computers. Figure 3.3 picturizes Single precision while figure 3.4 picturizes double precision. Single Precision uses 32bit format while double precision is 64 bits word length. As the name suggests double precision can represent fractions with larger accuracy. In both the cases, MSB is sign bit for the mantissa part, followed by Exponent and Mantissa. The exponent part has its sign bit.
It is to be noted that in Single Precision, we can represent an exponent in the range -127 to +127. It is possible as a result of arithmetic operations the resulting exponent may not fit in. This situation is called overflow in the case of positive exponent and underflow in the case of negative exponent. The Double Precision format has 11 bits for exponent meaning a number as large as -1023 to 1023 can be represented. The programmer has to make a choice between Single Precision and Double Precision declaration using his knowledge about the data being handled.
The Floating Point operations on the regular CPU is very very slow. Generally, a special purpose CPU known as Co-processor is used. This Co-processor works in tandem with the main CPU. The programmer should be using the float declaration only if his data is in real number form. Float declaration is not to be used generously.
- Decimal Numbers Representation
Decimal numbers (radix 10) are represented and processed in the system with the support of additional hardware. We deal with numbers in decimal format in everyday life. Some machines implement decimal arithmetic too, like floating-point arithmetic hardware. In such a case, the CPU uses decimal numbers in BCD (binary coded decimal) form and does BCD arithmetic operation. BCD operates on radix 10. This hardware operates without conversion to pure binary. It uses a nibble to represent a number in packed BCD form. BCD operations require not only special hardware but also decimal instruction set.
- Exceptions and Error Detection
All of us know that when we do arithmetic operations, we get answers which have more digits than the operands (Ex: 8 x 2= 16). This happens in computer arithmetic operations too. When the result size exceeds the allotted size of the variable or the register, it becomes an error and exception. The exception conditions associated with numbers and number operations are Overflow, Underflow, Truncation, Rounding and Multiple Precision . These are detected by the associated hardware in arithmetic Unit. These exceptions apply to both Fixed Point and Floating Point operations. Each of these exceptional conditions has a flag bit assigned in the Processor Status Word (PSW). We may discuss more in detail in the later chapters.
- Character Representation
Another data type is non-numeric and is largely character sets. We use a human-understandable character set to communicate with computer i.e. for both input and output. Standard character sets like EBCDIC and ASCII are chosen to represent alphabets, numbers and special characters. Nowadays Unicode standard is also in use for non-English language like Chinese, Hindi, Spanish, etc. These codes are accessible and available on the internet. Interested readers may access and learn more.
1. Track your progress [Earn 200 points]
Mark as complete
2. Provide your ratings to this chapter [Earn 100 points]
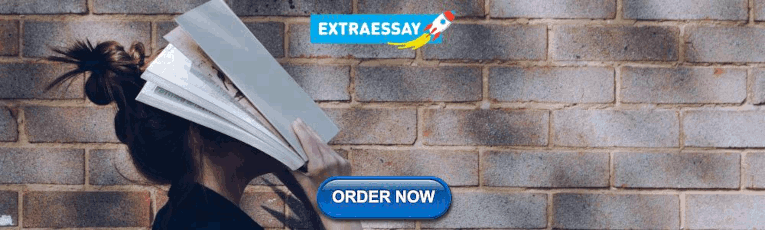
IMAGES
VIDEO
COMMENTS
4. DIGITAL REPRESENTATION • Within a computer, information is represented and stored in a digital binary format. • The term bit is an abbreviation of binary digit and represents the smallest piece of data. • Humans interpret words and pictures; computers interpret only patterns of bits. 5.
Data representation. Mar 31, 2017 • Download as PPTX, PDF •. 6 likes • 7,300 views. Prashant Saurabh. data representation this is related to computers. Education. Download now. Data representation - Download as a PDF or view online for free.
CS 3401 - Computer organization & assembly language. Data Representation. in. Computer Systems. Outline Data Organization Bits, Nibbles, Bytes, Words, Double Words Numbering Systems Unsigned Binary System Signed and Magnitude System 1's Complement System 2's Complement System Hexadecimal System Floating Point Representation BCD ...
Chapter 2 Data Representation CS140 Computer Organization These slides are derived from those of Null & Lobur + the work of others. Chapter 2: Data Representation. Chapter 2 Objectives • Understand the fundamentals of numerical data representation and manipulation in digital computers - both integer and floating point. • Master the skill of converting between various radix systems.
2 Data Representation and Processing To "process" or manipulate data, it must be converted into a form that the "processor" can understand Any data processing system (for example, a human or a computer system) must have the ability to: Recognize external data/stimuli and convert them into a suitable internal format Store and retrieve data internally Transport data among internal and ...
Objectives Understand the fundamentals of numerical data representation and manipulation in digital computers. Master the skill of converting between various radix systems. ... Download ppt "Data Representation in Computer Systems" ... 2-1 Chapter 2 - Data Representation Computer Architecture and Organization by M. Murdocca and V. Heuring ...
A bit is a 0 or 1 used in the digital representation of data. In digital computers, the user input is first converted and transmitted as electrical pulses that can be represented by two unique states ON and OFF. The ON state may be represented by a "1" and the off state by a "0".The sequence of ON'S and OFF'S forms the electrical ...
Presentation transcript: 1 Data Representation. 2 Topics Bit patterns Binary numbers Data type formats. Character representation Integer representation Floating point number representation. 3 Data Representation Data representation refers to the manner in which data is stored in the computer There are several different formats for data storage ...
DATA REPRESENTATION. DATA REPRESENTATION. Computer organization TCS 303/TIT 304. I ntroduction A bit is the most basic unit of information in a computer. It is a state of "on" or "off" in a digital circuit. Or "high" or "low" voltage instead of "on" or "off." A byte is a group of eight bits. 396 views • 28 slides
Data Representation Formats and Number Systems Explained. This document discusses different methods of representing data in a computer, including numeric data types, number systems, and encoding schemes. It covers binary, decimal, octal, and hexadecimal number systems. Methods for representing signed and unsigned integers are described, such as ...
Representing Data: 2. Image Representation Image representation methods 1. Bitmap Graphic Image is divided into matrix of pixels. A pixel represents a dot which is the smallest unit of the image. Image resolution depends on the number of pixels in the image. Higher resolution images require larger memory. Once image is divided into pixels, each ...
Data can be anything like a number, a name, notes in a musical composition, or the color in a photograph. Data representation can be referred to as the form in which we stored the data, processed it and transmitted it. In order to store the data in digital format, we can use any device like computers, smartphones, and iPads.
How do computers represent data? Google Classroom. When we look at a computer, we see text and images and shapes. To a computer, all of that is just binary data, 1s and 0s. The following 1s and 0s represents a tiny GIF: This next string of 1s and 0s represents a command to add a number: You might be scratching your head at this point.
1 Data Representation Computer Organization & Assembly Language Programming Dr Adnan Gutub aagutub 'at' uqu.edu.sa [Adapted from slides of Dr. Kip Irvine: Assembly Language for Intel-Based Computers] Most Slides contents have been arranged by Dr Muhamed Mudawar & Dr Aiman El-Maleh from Computer Engineering Dept. at KFUPM
Data Representation - Download as a PDF or view online for free. Data Representation - Download as a PDF or view online for free ... Basic Computer Organization and Design Aksum Institute of Technology(AIT, @Letsgo) ... Lecture 1 PPT Number systems & conversions part.pptx.
unpacked BCD number has only a single decimal digit stored in each data byte. In this case, the decimal digit will be in the low four bits and the upper 4 bits of the byte will be 0. In the packed BCD representation, two decimal digits are placed in each byte. Generally, the high order bits of the data byte contain the more significant decimal ...
COMPUTER ORGANIZATION & ARCHITECTURE (3140707) - Lecture PPT. TOPIC: 1. Unit - 1 Computer Data Representation. 2. Unit - 2 Basic Computer Organization and Design. 3. Unit - 3 Assembly Language Programming. 4.
2-1 Computer Organization Part Fixed Point Numbers Using only two digits of precision for signed base 10 numbers, the range (interval between lowest. Data Representation ICS 233 Basic Concepts Computer Organization and Assembly Language.
Computer Organization and Architecture is used to design computer systems. Computer Architecture is considered to be those attributes of a system that are visible to the user like addressing techniques, instruction sets, and bits used for data, and have a direct impact on the logic execution of a program, It defines the system in an abstract manner, It deals with What does the system do.
Mantissa, Significand and fraction are synonymously used terms. In the computer, the representation is binary and the binary point is not fixed. For example, a number, say, 23.345 can be written as 2.3345 x 101 or 0.23345 x 102 or 2334.5 x 10-2. The representation 2.3345 x 101 is said to be in normalised form.
Technology. 1 of 46. Download Now. Download to read offline. Computer Systems Data Representation - Download as a PDF or view online for free.