How to create a copy of an array in Go or Golang?
September 2, 2022 - 3 min read
To create a copy of an array in Go or golang, we can simply assign the array to another variable using the = operator (assignment) and the contents will be copied over to the new array variable.
For example, let's say we have an array of 5 numbers like this,
Now to copy the elements of the nums array we can define another variable called numsCopy and then use the = operator and then simply assign the values of the nums array to it.
It can be done like this,
Now to check if the numsCopy has the elements like in the nums variable, we can first print the numsCopy variable to the console using the Println() method from the fmt standard package like this,
Now let's mutate the contents on the numsCopy array and see if the contents of the nums array change. It can be done like this,
As you can see that the numsCopy array contents are changed but not the nums array content. This proves to us that the nums array is successfully copied into the numsCopy array variable. Yay š„³!
See the above code live in The Go Playground .
That's all š.
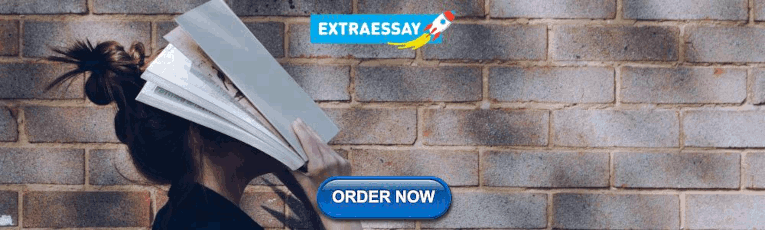
Feel free to share if you found this useful š.

How to Deep Copy or Duplicate a Slice in Go
This article provides a comprehensive guide on duplicating slices in Go, highlighting the importance of using copy() and append() for deep copying.
In Go, simply assigning one slice to another doesn’t create a new, independent copy. Instead, both variables refer to the same underlying array, meaning changes in one slice will reflect in the other.
This shared reference becomes apparent in function calls, as slices are passed by reference:
1. Deep copying with copy()
The built-in copy() function creates an independent copy of a slice. It requires initializing a new slice of the appropriate length first:
The copy() function in Go requires two arguments: the destination slice first and the source slice second, with both being of the same type. It returns the count of elements copied. You can disregard this return value if it’s not needed.
A key aspect of copy() is that the lengths of the destination and source slices can differ; it copies elements only up to the length of the shorter slice. Therefore, copying to a slice that is empty or nil results in no elements being transferred.
An important edge case to note with copy() is that the destination slice remains non-nil, even if the source slice is nil :
To ensure that the destination slice also becomes nil if the source is nil , you can modify your code as follows:
2. Deep copying with append()
The built-in append() offers another way to duplicate a slice, ensuring the new slice is independent of the original:
In this scenario, elements from s1 are appended to a nil slice, and the resultant slice becomes s2 . This approach ensures a complete duplication of the slice, irrespective of the destination’s initial length, unlike the copy() function. It’s important to note that the final length of s2 adjusts to match the length of s1 , either by truncating or expanding as necessary.
When using append() , if the source slice is non-nil but empty, the resulting destination slice becomes nil :
To address this, create a three-index subslice from the original slice and append it instead. This method ensures the new slice does not share any elements with the source by setting both the length and capacity of the subslice to zero:
Final thoughts
In this article, we explored two methods for cloning a slice in Go, while addressing some important edge cases related to either technique. If you have additional insights or methods to share, please feel free to contribute in the comments section.
Thank you for reading, and happy coding!
How to use the copy function
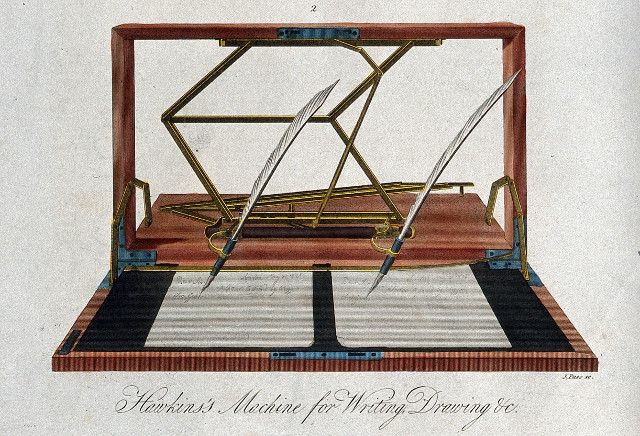
The built-in copy function copies elements into a destination slice dst from a source sliceĀ src .
It returns the number of elements copied, which will be the minimum of len(dst) and len(src) . The result does not depend on whether the arguments overlap.
As a special case , it’s legal to copy bytes from a string to a slice ofĀ bytes.
Copy from one slice to another
Copy from a slice to itself, copy from a string to a byte slice (specialĀ case), further reading.
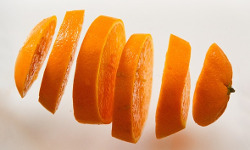
Slices and arrays in 6 easy steps
This package is not in the latest version of its module.
Documentation ¶
Package builtin provides documentation for Go's predeclared identifiers. The items documented here are not actually in package builtin but their descriptions here allow godoc to present documentation for the language's special identifiers.
- func append(slice []Type, elems ...Type) []Type
- func cap(v Type) int
- func clear[T ~[]Type | ~map[Type]Type1](t T)
- func close(c chan<- Type)
- func complex(r, i FloatType) ComplexType
- func copy(dst, src []Type) int
- func delete(m map[Type]Type1, key Type)
- func imag(c ComplexType) FloatType
- func len(v Type) int
- func make(t Type, size ...IntegerType) Type
- func max[T cmp.Ordered](x T, y ...T) T
- func min[T cmp.Ordered](x T, y ...T) T
- func new(Type) *Type
- func panic(v any)
- func print(args ...Type)
- func println(args ...Type)
- func real(c ComplexType) FloatType
- func recover() any
- type ComplexType
- type FloatType
- type IntegerType
- type comparable
- type complex128
- type complex64
- type float32
- type float64
- type string
- type uint16
- type uint32
- type uint64
- type uintptr
Constants ¶
true and false are the two untyped boolean values.
iota is a predeclared identifier representing the untyped integer ordinal number of the current const specification in a (usually parenthesized) const declaration. It is zero-indexed.
Variables ¶
nil is a predeclared identifier representing the zero value for a pointer, channel, func, interface, map, or slice type.
Functions ¶
Func append ¶.
The append built-in function appends elements to the end of a slice. If it has sufficient capacity, the destination is resliced to accommodate the new elements. If it does not, a new underlying array will be allocated. Append returns the updated slice. It is therefore necessary to store the result of append, often in the variable holding the slice itself:
As a special case, it is legal to append a string to a byte slice, like this:
The cap built-in function returns the capacity of v, according to its type:
For some arguments, such as a simple array expression, the result can be a constant. See the Go language specification's "Length and capacity" section for details.
func clear ¶ added in go1.21.0
The clear built-in function clears maps and slices. For maps, clear deletes all entries, resulting in an empty map. For slices, clear sets all elements up to the length of the slice to the zero value of the respective element type. If the argument type is a type parameter, the type parameter's type set must contain only map or slice types, and clear performs the operation implied by the type argument.
func close ¶
The close built-in function closes a channel, which must be either bidirectional or send-only. It should be executed only by the sender, never the receiver, and has the effect of shutting down the channel after the last sent value is received. After the last value has been received from a closed channel c, any receive from c will succeed without blocking, returning the zero value for the channel element. The form
will also set ok to false for a closed and empty channel.
func complex ¶
The complex built-in function constructs a complex value from two floating-point values. The real and imaginary parts must be of the same size, either float32 or float64 (or assignable to them), and the return value will be the corresponding complex type (complex64 for float32, complex128 for float64).
func copy ¶
The copy built-in function copies elements from a source slice into a destination slice. (As a special case, it also will copy bytes from a string to a slice of bytes.) The source and destination may overlap. Copy returns the number of elements copied, which will be the minimum of len(src) and len(dst).
func delete ¶
The delete built-in function deletes the element with the specified key (m[key]) from the map. If m is nil or there is no such element, delete is a no-op.
func imag ¶
The imag built-in function returns the imaginary part of the complex number c. The return value will be floating point type corresponding to the type of c.
The len built-in function returns the length of v, according to its type:
For some arguments, such as a string literal or a simple array expression, the result can be a constant. See the Go language specification's "Length and capacity" section for details.
func make ¶
The make built-in function allocates and initializes an object of type slice, map, or chan (only). Like new, the first argument is a type, not a value. Unlike new, make's return type is the same as the type of its argument, not a pointer to it. The specification of the result depends on the type:
func max ¶ added in go1.21.0
The max built-in function returns the largest value of a fixed number of arguments of cmp.Ordered types. There must be at least one argument. If T is a floating-point type and any of the arguments are NaNs, max will return NaN.
func min ¶ added in go1.21.0
The min built-in function returns the smallest value of a fixed number of arguments of cmp.Ordered types. There must be at least one argument. If T is a floating-point type and any of the arguments are NaNs, min will return NaN.
The new built-in function allocates memory. The first argument is a type, not a value, and the value returned is a pointer to a newly allocated zero value of that type.
func panic ¶
The panic built-in function stops normal execution of the current goroutine. When a function F calls panic, normal execution of F stops immediately. Any functions whose execution was deferred by F are run in the usual way, and then F returns to its caller. To the caller G, the invocation of F then behaves like a call to panic, terminating G's execution and running any deferred functions. This continues until all functions in the executing goroutine have stopped, in reverse order. At that point, the program is terminated with a non-zero exit code. This termination sequence is called panicking and can be controlled by the built-in function recover.
Starting in Go 1.21, calling panic with a nil interface value or an untyped nil causes a run-time error (a different panic). The GODEBUG setting panicnil=1 disables the run-time error.
func print ¶ added in go1.2
The print built-in function formats its arguments in an implementation-specific way and writes the result to standard error. Print is useful for bootstrapping and debugging; it is not guaranteed to stay in the language.
func println ¶ added in go1.2
The println built-in function formats its arguments in an implementation-specific way and writes the result to standard error. Spaces are always added between arguments and a newline is appended. Println is useful for bootstrapping and debugging; it is not guaranteed to stay in the language.
func real ¶
The real built-in function returns the real part of the complex number c. The return value will be floating point type corresponding to the type of c.
func recover ¶
The recover built-in function allows a program to manage behavior of a panicking goroutine. Executing a call to recover inside a deferred function (but not any function called by it) stops the panicking sequence by restoring normal execution and retrieves the error value passed to the call of panic. If recover is called outside the deferred function it will not stop a panicking sequence. In this case, or when the goroutine is not panicking, recover returns nil.
Prior to Go 1.21, recover would also return nil if panic is called with a nil argument. See [panic] for details.
type ComplexType ¶
ComplexType is here for the purposes of documentation only. It is a stand-in for either complex type: complex64 or complex128.
type FloatType ¶
FloatType is here for the purposes of documentation only. It is a stand-in for either float type: float32 or float64.
type IntegerType ¶
IntegerType is here for the purposes of documentation only. It is a stand-in for any integer type: int, uint, int8 etc.
type Type ¶
Type is here for the purposes of documentation only. It is a stand-in for any Go type, but represents the same type for any given function invocation.
type Type1 ¶
Type1 is here for the purposes of documentation only. It is a stand-in for any Go type, but represents the same type for any given function invocation.
type any ¶ added in go1.18
any is an alias for interface{} and is equivalent to interface{} in all ways.
type bool ¶
bool is the set of boolean values, true and false.
type byte ¶
byte is an alias for uint8 and is equivalent to uint8 in all ways. It is used, by convention, to distinguish byte values from 8-bit unsigned integer values.
type comparable ¶ added in go1.18
comparable is an interface that is implemented by all comparable types (booleans, numbers, strings, pointers, channels, arrays of comparable types, structs whose fields are all comparable types). The comparable interface may only be used as a type parameter constraint, not as the type of a variable.
type complex128 ¶
complex128 is the set of all complex numbers with float64 real and imaginary parts.
type complex64 ¶
complex64 is the set of all complex numbers with float32 real and imaginary parts.
type error ¶
The error built-in interface type is the conventional interface for representing an error condition, with the nil value representing no error.
type float32 ¶
float32 is the set of all IEEE-754 32-bit floating-point numbers.
type float64 ¶
float64 is the set of all IEEE-754 64-bit floating-point numbers.
int is a signed integer type that is at least 32 bits in size. It is a distinct type, however, and not an alias for, say, int32.
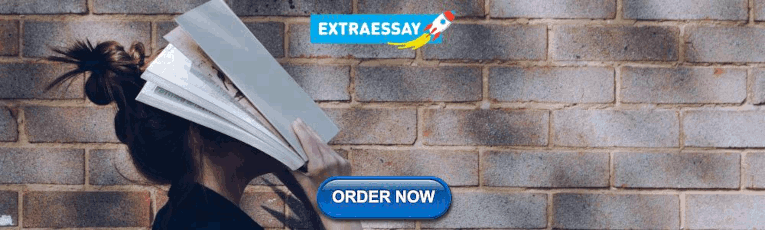
type int16 ¶
int16 is the set of all signed 16-bit integers. Range: -32768 through 32767.
type int32 ¶
int32 is the set of all signed 32-bit integers. Range: -2147483648 through 2147483647.
type int64 ¶
int64 is the set of all signed 64-bit integers. Range: -9223372036854775808 through 9223372036854775807.
type int8 ¶
int8 is the set of all signed 8-bit integers. Range: -128 through 127.
type rune ¶
rune is an alias for int32 and is equivalent to int32 in all ways. It is used, by convention, to distinguish character values from integer values.
type string ¶
string is the set of all strings of 8-bit bytes, conventionally but not necessarily representing UTF-8-encoded text. A string may be empty, but not nil. Values of string type are immutable.
type uint ¶
uint is an unsigned integer type that is at least 32 bits in size. It is a distinct type, however, and not an alias for, say, uint32.
type uint16 ¶
uint16 is the set of all unsigned 16-bit integers. Range: 0 through 65535.
type uint32 ¶
uint32 is the set of all unsigned 32-bit integers. Range: 0 through 4294967295.
type uint64 ¶
uint64 is the set of all unsigned 64-bit integers. Range: 0 through 18446744073709551615.
type uint8 ¶
uint8 is the set of all unsigned 8-bit integers. Range: 0 through 255.
type uintptr ¶
uintptr is an integer type that is large enough to hold the bit pattern of any pointer.
Source Files ¶
Keyboard shortcuts.
Learn to Clone or Copy Map in GO Like a PRO [5 Methods]
November 1, 2023
In the vast and versatile world of Go (Golang) programming, one often encounters the necessity to replicate data structures, ensuring that modifications in the replica do not affect the original. This necessity brings us to the concepts of copy map and clone map. When we talk about copying or cloning a map in Go, we refer to the creation of a duplicate map, possessing the same keys and values as the original. This duplication process is essential in various scenarios, such as preserving the original mapās state, preventing unauthorized modifications, or performing operations on the map without altering the actual data.
In this tutorial we will explore different methods which can be used to copy map or what we can also term as clone map in Golang.
Different Methods to Copy Map in GO
Here are various methods you can use to copy or clone a map in Go (Golang):
- Using Range Loop with Make : A simple method where a new map is initialized, and elements from the original map are iterated over and assigned to the new map, ensuring a separate memory allocation.
- Using JSON Marshalling and Unmarshalling : This technique involves converting the original map into a JSON object and then converting it back into a new map, ensuring that the new map is a separate entity.
- Using Deep Copy with Third-Party Packages : Utilizing available third-party packages that offer deep copy functionalities, ensuring that nested maps or maps with reference types are effectively cloned.
- Using Reflection : Employing the reflect package to dynamically create a new map and populate it with the elements of the original map, catering to various data types.
- Custom Implementation Based on Type : Crafting specific functions or methods tailored to the types stored in the map, ensuring an effective copying process tuned to the map's content.
Deep Copy vs Shallow Copy
When you copy map elements in Go, itās essential to understand the difference between a deep copy and a shallow copy. The distinction lies in how the map's contents, particularly reference types like slices, maps, and pointers, are duplicated.
A deep copy creates a new map with not only the immediate elements of the original map being duplicated but also the underlying elements of any reference types recursively copied. This means that modifications to the nested elements in the copied map wonāt affect the original map.
Situations where Deep Copy is applicable:
- When the map contains reference types like slices , maps, or pointers, and you want to manipulate them without affecting the original map.
- When you need complete isolation between the original and copied map.
Example of Deep Copy:
In this example, a deep copy of the map is made, and modifying the copied map does not affect the original map.
Shallow Copy
A shallow copy duplicates the immediate elements of the original map into a new map, but the reference types within the map still point to the same memory locations as those in the original map.
Situations where Shallow Copy is applicable:
- When you only need to modify the top-level elements of the map and want to maintain links to the original nested structures.
- When full isolation between the original and copied map is not necessary.
Example of Shallow Copy:
In this example, a shallow copy of the map is made. Modifying the copied map's slice also affects the original map because the slice's underlying array is still shared between both maps.
1. Using Range Loop with Make
When you want to copy map elements in Go, one straightforward approach is utilizing a range loop in conjunction with the make() function. Hereās how it works:
Performing Shallow Copy
In a shallow copy, the outermost objects are duplicated, while the inner elements retain references to the same memory locations as the original mapās inner elements.
Here, both the original and copied maps refer to the same memory locations of the inner elements. Modifying the copied map also modifies the original map.
Performing Deep Copy
A deep copy means cloning every item recursively, ensuring that no memory locations of the inner elements are shared between the original and copied map.
Modifying the copied map doesnāt affect the original map because every element, including the inner slices, has been recursively cloned.
We can also declare the copyMap logic inside a function to make it re-usable:
2. Using JSON Marshalling and Unmarshalling
JSON Marshalling and Unmarshalling can also be used to copy map contents in Go. This method involves converting the map into a JSON string (marshalling) and then creating a new map by parsing the JSON string back (unmarshalling). This is more commonly used for deep copying and it is not so straight forward to perform shallow copy using this method.
By default, using JSON marshalling and unmarshalling performs a deep copy because the data is serialized and deserialized into a new memory allocation .
3. Using Third-Party Package (copier)
The copier package primarily focuses on struct copying but can also be utilized for maps, primarily resulting in deep copying behavior. Here's how you can use copier for copying maps:
In this example, even though pointers are used, copier creates a new instance of the ValueStruct , making the copiedMap independent of the originalMap . This is essentially a deep copy, as modifications to the copiedMap do not affect the originalMap .
The copier package might not directly support a shallow copy in terms of retaining the original pointers. For a shallow copy, you might need to use a different approach or package.
4. Using Reflection
Reflection in Go is a powerful tool that allows for inspection and modification of variable types and values at runtime. Itās more complex and might not be the most performance-efficient way of copying maps, but it offers flexibility. Hereās how you could employ reflection to accomplish both shallow and deep copy tasks:
A shallow copy creates a new map, but the elements inside the map might still refer to the same memory locations as the original map.
A deep copy using reflection involves recursively copying each element, ensuring that the original and copied maps donāt share references.
In these examples, functions shallowCopyMap() and deepCopyMap() utilize reflection to perform the copy operations.
Note that using reflection might not be the most performant choice due to the dynamic type checking and recursive calls involved, especially in a deep copy. However, reflection provides a generic way to copy or clone maps, even when the exact types are not known at compile-time, offering a degree of flexibility and generality in handling various data structures.
5. Custom Implementation Based on Type
Creating a custom implementation based on types allows for a more targeted approach in copying maps. It gives you the flexibility to define the copying process, specifically according to the types and structures you are dealing with.
Creating a shallow copy of a map based on specific types involves duplicating the outer map while keeping references to the same inner elements.
Creating a deep copy of a map based on specific types involves duplicating not only the outer map but also the inner elements, ensuring no shared references.
In these examples, shallowCopyIntMap and deepCopyStructMap functions are designed specifically based on the data types in the maps.
Frequently Asked Questions (FAQs)
What does it mean to "copy a map" in Go?
Copying a map in Go refers to creating a new map that contains all the keys and values of the original map. Depending on how you choose to copy the map, the new map might share references to the same underlying data (shallow copy) or have completely separate data (deep copy).
What is the difference between a "shallow copy" and a "deep copy" of a map in Go?
A shallow copy of a map involves creating a new map with new keys, but the values still hold references to the same objects as the original map. In a deep copy, a new map is created with new keys, and new objects are also created for the values, ensuring no shared references with the original map.
How do you perform a shallow copy of a map in Go?
A shallow copy can be performed using a simple loop to copy keys and values from the original map to a new one, for instance using the range loop. The new map gets filled with the keys and values of the original map, but the values (if they are reference types like slices or maps) still point to the same memory locations.
How do you perform a deep copy of a map in Go?
A deep copy can be achieved using various methods such as manually creating new objects for each value, utilizing the encoding/json package to marshal and unmarshal the map, or using third-party libraries that offer deep copying functionalities like copier .
When should I use a shallow copy, and when should I use a deep copy?
Use a shallow copy when itās acceptable to have the copied map's values point to the same references as the original mapās values. Choose a deep copy when you want the copied map to be entirely independent of the original map, with no shared references, ensuring changes in the copied map do not affect the original map.
Is it possible to copy maps of custom structs in Go?
Yes, you can copy maps with custom structs as values. When copying, you can choose whether to perform a shallow copy, where the struct references are shared, or a deep copy, where new struct instances are created for the copied map.
Are there any third-party libraries that help in copying maps in Go?
Yes, there are third-party libraries, like copier or go-cmp , which provide functionalities to easily perform shallow and deep copies of maps and other data structures in Go. Before using a third-party library, ensure it is well-maintained and widely accepted by the community for reliability and performance.
Does Go have built-in support for deep copying maps?
Go doesnāt have a built-in function specifically for deep copying maps. However, you can achieve deep copying by using various techniques such as the encoding/json package for marshaling and unmarshaling maps, creating custom functions, or using third-party libraries.
In conclusion, copying or cloning maps in Go can be accomplished through various methods, each with its unique applications and considerations. The fundamental distinction lies between performing a shallow copy and a deep copy. A shallow copy is simpler and entails replicating the map's structure and entries, but not deeply duplicating nested reference types, leading to shared references. In contrast, a deep copy creates a completely independent copy of the map, ensuring that all nested elements, even those of reference types, are independently replicated without sharing memory references.
Different techniques, ranging from using simple range loops, JSON marshaling and unmarshaling, reflection, custom type-based implementations, to employing third-party packages, offer a spectrum of tools to achieve the desired level of copying. The selection of a suitable method is guided by various factors such as the necessity to maintain or sever reference linkages between the original and copied maps, performance considerations, and the specific data types and structures involved.
For more information and official documentation on maps in Go, refer to the following links:
- Go Maps in Action - A blog post that goes deep into maps, providing a fundamental understanding.
- Go by Example: Maps - An illustrative guide that walks through the basics of using maps in Go.
- Go Documentation: Map Type - The official Go documentation describing the map type.
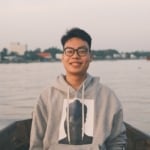
Tuan Nguyen
He is proficient in Golang, Python, Java, MongoDB, Selenium, Spring Boot, Kubernetes, Scrapy, API development, Docker, Data Scraping, PrimeFaces, Linux, Data Structures, and Data Mining. With expertise spanning these technologies, he develops robust solutions and implements efficient data processing and management strategies across various projects and platforms. You can connect with him on his LinkedIn profile.
Can't find what you're searching for? Let us assist you.
Enter your query below, and we'll provide instant results tailored to your needs.
If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation.
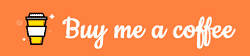
For any other feedbacks or questions you can send mail to [email protected]
Thank You for your support!!
Leave a Comment Cancel reply
Save my name and email in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
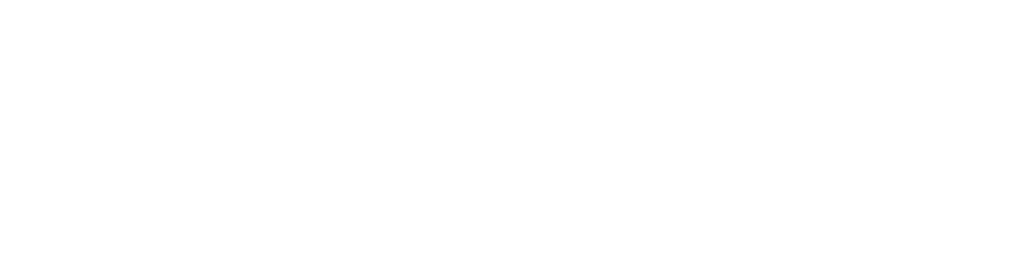
We try to offer easy-to-follow guides and tips on various topics such as Linux, Cloud Computing, Programming Languages, Ethical Hacking and much more.
Recent Comments
Popular posts, 7 tools to detect memory leaks with examples, 100+ linux commands cheat sheet & examples, tutorial: beginners guide on linux memory management, top 15 tools to monitor disk io performance with examples, overview on different disk types and disk interface types, 6 ssh authentication methods to secure connection (sshd_config), how to check security updates list & perform linux patch management rhel 6/7/8, 8 ways to prevent brute force ssh attacks in linux (centos/rhel 7).
Privacy Policy
HTML Sitemap
- How to Copy Slice in Go
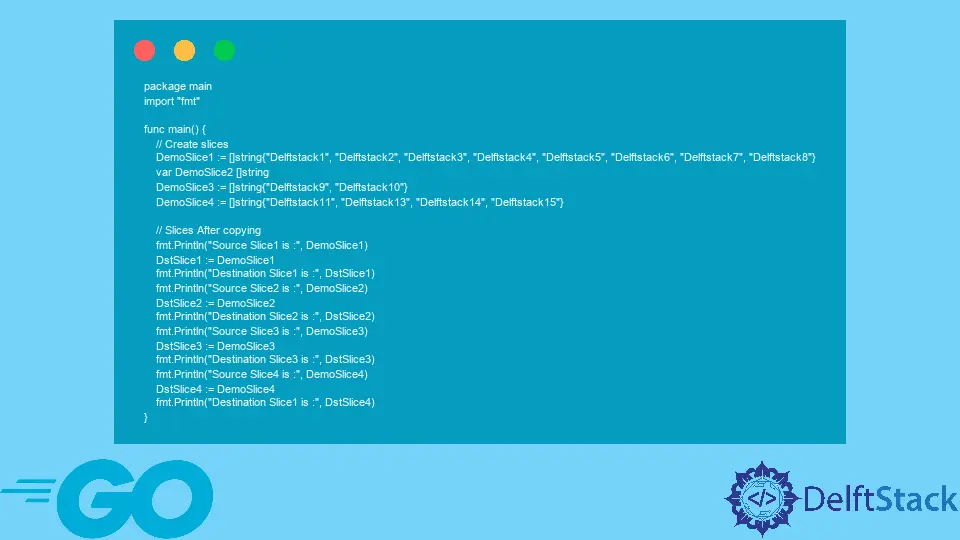
This tutorial demonstrates how to copy a slice in GoLang.
Copy Slice in GoLang
Copying a slice in GoLang can be achieved through different methods. The copy() and append() methods are usually used for this purpose, where the copy() gets the deep copy of a given slice, and the append() method will copy the content of a slice into an empty slice.
Use the Copy() Method to Copy a Slice in Go
The copy() method is considered the best method to copy a slice in the Golang because it creates a deep copy of the slice.
The destination is the copied slice, and the source is the slice from which we copy. The function returns the number of elements of the copied slice.
Code Example:
The code above creates four source slices and four destination slices with different members or empty with the same length.
Use the Append() Method to Copy a Slice in Go
The append method will append the content of a given slice to the destination slice.
The append method will copy the content of the source slice to an empty destination slice or a slice with members. It will return the slice with previous and copied members, which is different from the copy method.
The code snippet above will copy a slice’s content to another.
Use the Assignment Method to Copy a Slice in Go
The assignment method copy is a shallow copy of a slice where we assign a source slice to the destination slice.
As we can see, we assign the slices to other slices. This is a shallow method and not widely used because when we modify the content of copy, the original slice will also change.
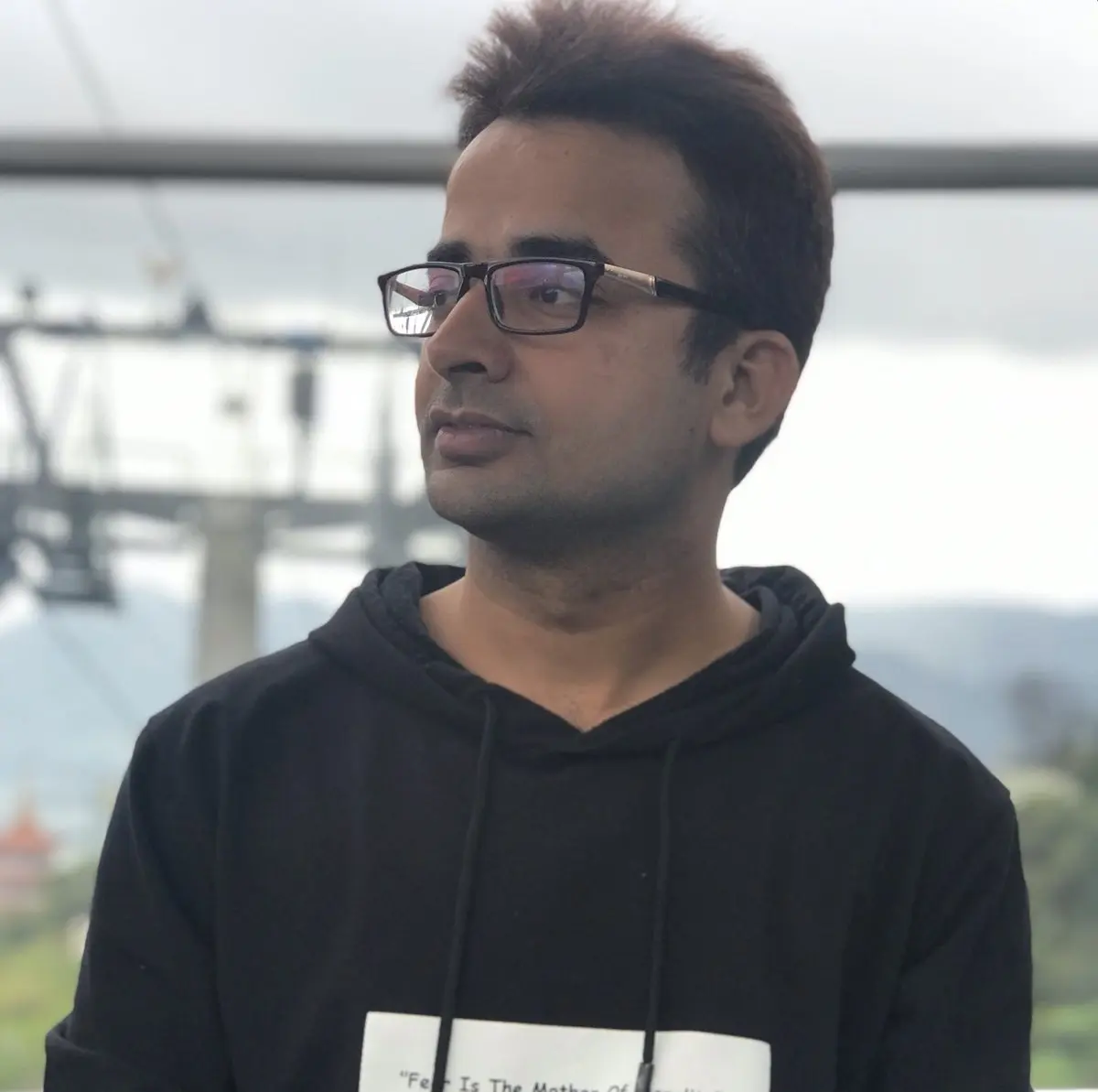
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
Related Article - Go Slice
- How to Delete an Element From a Slice in Golang
- How to Sort Slice of Structs in Go
- Difference Between []String and ...String in Go
- How to Check if a Slice Contains an Element in Golang
- How to Create an Empty Slice in Go
š Copy a map in Go
Maps in Go are reference types , so to deep copy the contents of a map, you cannot assign one instance to another. You can do this by creating a new, empty map and then iterating over the old map in a for range loop to assign the appropriate key-value pairs to the new map. It is the simplest and most efficient solution to this problem in Go.
As you see in the output, the copied map is a deep clone, and adding new elements does not affect the old map.
Be careful when making a shallow copy by assigning one map to another. In this case, a modification in either map will cause a change in the data of both maps.
Thank you for being on our site š. If you like our tutorials and examples, please consider supporting us with a cup of coffee and we'll turn it into more great Go examples.
Have a great day!
šļø Check if a map contains a key in Go
Learn how to check if a key exists in a map, š« count the occurrences of an element in a slice in go, learn how to count elements in a slice that meet certain conditions, ā¤ļøā𩹠recover function in go, learn what it is for and how to use the built-in recover() function.
- Data Types in Go
- Go Keywords
- Go Control Flow
- Go Functions
- GoLang Structures
- GoLang Arrays
- GoLang Strings
- GoLang Pointers
- GoLang Interface
- GoLang Concurrency
- Go Programming Language (Introduction)
- How to Install Go on Windows?
- How to Install Golang on MacOS?
- Hello World in Golang
Fundamentals
- Identifiers in Go Language
- Go Variables
- Constants- Go Language
- Go Operators
Control Statements
- Go Decision Making (if, if-else, Nested-if, if-else-if)
- Loops in Go Language
- Switch Statement in Go
Functions & Methods
- Functions in Go Language
- Variadic Functions in Go
- Anonymous function in Go Language
- main and init function in Golang
- What is Blank Identifier(underscore) in Golang?
- Defer Keyword in Golang
- Methods in Golang
- Structures in Golang
- Nested Structure in Golang
- Anonymous Structure and Field in Golang
- Arrays in Go
How to Copy an Array into Another Array in Golang?
- How to pass an Array to a Function in Golang?
- Slices in Golang
- Slice Composite Literal in Go
- How to sort a slice of ints in Golang?
- How to trim a slice of bytes in Golang?
- How to split a slice of bytes in Golang?
- Strings in Golang
- How to Trim a String in Golang?
- How to Split a String in Golang?
- Different ways to compare Strings in Golang
- Pointers in Golang
- Passing Pointers to a Function in Go
- Pointer to a Struct in Golang
- Go Pointer to Pointer (Double Pointer)
- Comparing Pointers in Golang
Concurrency
- Goroutines - Concurrency in Golang
- Select Statement in Go Language
- Multiple Goroutines
- Channel in Golang
- Unidirectional Channel in Golang
Arrays in Golang or Go programming language is much similar to other programming languages. In the program, sometimes we need to store a collection of data of the same type, like a list of student marks. Such type of collection is stored in a program using an Array. An array is a fixed-length sequence that is used to store homogeneous elements in the memory. Golang does not provide a specific built-in function to copy one array into another array. But we can create a copy of an array by simply assigning an array to a new variable by value or by reference. If we create a copy of an array by value and made some changes in the values of the original array, then it will not reflect in the copy of that array. And if we create a copy of an array by reference and made some changes in the values of the original array, then it will reflect in the copy of that array. As shown in the below examples:
Let us discuss this concept with the help of the examples:
Example 1:
Example 2:
There are several ways to copy an array into another array in Go. Here are three common methods:
1.using a loop:, 2.using the copy function:, 3. using the append function:.
These are the three most common methods for copying an array into another array in Go. The copy function is the most efficient way to copy arrays, but the other methods can be useful in different scenarios.
Please Login to comment...
Similar reads.
- Golang-Arrays
- Go Language
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
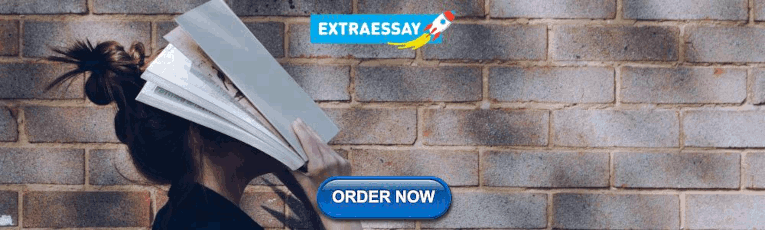
COMMENTS
I know that Go is a "pass by value" language and even managed to reason about this behavior and understand all the implications. All assignments in Go also create copies. In some cases, it's just a value, in some -- a pointer. For some data structures, it's a bit trickier in that the whole structure is copied and might include an implicit ...
While learning nuances of array data structure in Go I came across an interesting confusion. I have learnt from blog.golang.org that - when you assign or pass around an array value you will make a copy of its contents. To check this myself I wrote the following piece of code:
Copying map, slice,.. does a copy by reference. So modifying the destination is equal to modify the source and versa. Copy struct in Golang Perform deep copy of a struct. Here is an example of deep copying a struct to another a variable. All the fields in the struct are primitive data types:
Arrays. The slice type is an abstraction built on top of Go's array type, and so to understand slices we must first understand arrays. An array type definition specifies a length and an element type. For example, the type [4]int represents an array of four integers. An array's size is fixed; its length is part of its type ( [4]int and [5 ...
September 2, 2022 - 3 min read. To create a copy of an array in Go or golang, we can simply assign the array to another variable using the = operator (assignment) and the contents will be copied over to the new array variable.
Copy. When we doubled the capacity of our slice in the previous section, we wrote a loop to copy the old data to the new slice. Go has a built-in function, copy, to make this easier. Its arguments are two slices, and it copies the data from the right-hand argument to the left-hand argument. Here's our example rewritten to use copy:
The copy() function in Go requires two arguments: the destination slice first and the source slice second, with both being of the same type. It returns the count of elements copied. You can disregard this return value if it's not needed. A key aspect of copy() is that the lengths of the destination and source slices can differ; it copies elements only up to the length of the shorter slice.
Copy a slice using the append() function. Output: source slice: [a b c], address: 0xc0000981b0. Copying a slice using the append() function is really simple. You just need to define a new empty slice, and use the append() to add all elements of the src to the dst slice. In that way, you get a new slice with all the elements duplicated.
assignment copies lock value to wg2: sync.WaitGroup contains sync.noCopy How does the IDE achieve this? Can we use this mechanism to tell others not to copy a particular structure?
copy built-in function. copy function copies elements from a source (src) slice into a destination (dst) slice. func copy(dst, src []Type) int. Create a new empty slice with the same size of the src and then copy all the elements of the src to the empty slice. Using the copy function, src and dst slices have different backing arrays.
func copy(dst, src []Type) int It returns the number of elements copied, which will be the minimum of len(dst) and len(src). The result does not depend on whether the arguments overlap. As a special case, it's legal to copy bytes from a string to a slice of bytes. copy(dst []byte, src string) int Examples Copy from one slice to another
The copy built-in function copies elements from a source slice into a destination slice. (As a special case, it also will copy bytes from a string to a slice of bytes.) The source and destination may overlap. Copy returns the number of elements copied, which will be the minimum of len (src) and len (dst).
Different Methods to Copy Map in GO. Here are various methods you can use to copy or clone a map in Go (Golang): Using Range Loop with Make: A simple method where a new map is initialized, and elements from the original map are iterated over and assigned to the new map, ensuring a separate memory allocation.; Using JSON Marshalling and Unmarshalling: This technique involves converting the ...
The copy() and append() methods are usually used for this purpose, where the copy() gets the deep copy of a given slice, and the append() method will copy the content of a slice into an empty slice. Use the Copy() Method to Copy a Slice in Go. The copy() method is considered the best method to copy a slice in the Golang because it creates a ...
It is the simplest and most efficient solution to this problem in Go. Output: map[blueberry:2 raspberry:3 strawberry:1] copied map. map[apple:4 blueberry:2 raspberry:3 strawberry:1] As you see in the output, the copied map is a deep clone, and adding new elements does not affect the old map. Be careful when making a shallow copy by assigning ...
A structure or struct in Golang is a user-defined data type that allows to combine data types of different kinds and act as a record. A struct variable in Golang can be copied to another variable easily using the assignment statement (=). Any changes made to the second struct will not be reflected back to the first struct. Example 1: Output: In ...
The pre-Go1.18 version, without generics, can be found here . For more information and other documents, see go.dev . Go is a general-purpose language designed with systems programming in mind. It is strongly typed and garbage-collected and has explicit support for concurrent programming.
Golang does not provide a specific built-in function to copy one array into another array. But we can create a copy of an array by simply assigning an array to a new variable by value or by reference. If we create a copy of an array by value and made some changes in the values of the original array, then it will not reflect in the copy of that ...
The primary "function" for copying an array in Go is the assignment operator =, as it is the case for any other value of any other type. package main. import "fmt". func main() {. var a, b [4]int. a[2] = 42. b = a. fmt.Println(a, b) // 2D array.