Type Assertion in TypeScript
Here, you will learn about how TypeScript infers and checks the type of a variable using some internal logic mechanism called Type Assertion.
Type assertion allows you to set the type of a value and tell the compiler not to infer it. This is when you, as a programmer, might have a better understanding of the type of a variable than what TypeScript can infer on its own. Such a situation can occur when you might be porting over code from JavaScript and you may know a more accurate type of the variable than what is currently assigned. It is similar to type casting in other languages like C# and Java. However, unlike C# and Java, there is no runtime effect of type assertion in TypeScript. It is merely a way to let the TypeScript compiler know the type of a variable.
In the above example, we have a variable code of type any . We assign the value of this variable to another variable called employeeCode . However, we know that code is of type number, even though it has been declared as 'any'. So, while assigning code to employeeCode , we have asserted that code is of type number in this case, and we are certain about it. Now, the type of employeeCode is number.
Similarly, we might have a situation where we have an object that has been declared without any properties yet.
The above example will give a compiler error, because the compiler assumes that the type of employee is {} with no properties. But, we can avoid this situation by using type assertion, as shown below.
In the above example, we created an interface Employee with the properties name and code. We then used this type assertion on employee. Interfaces are used to define the structure of variables. Learn more about this in interface chapter.
Be careful while using type assertion. The TypeScript compiler will autocomplete Employee properties, but it won't show any compile time error if you forgot to add the properties. For example:
You can also use the JavaScript library in TypeScript for some existing functions.
In the above example, we assume that myJSLib is a separate JavaScript library and we call its GetEmployeeCode() function. So, we set the type of return value as number because we know that it returns a number.
There are two ways to do type assertion in TypeScript:
1. Using the angular bracket <> syntax. So far in this section, we have used angular brackets to show type assertion.
However, there is another way to do type assertion, using the 'as' syntax.
2. Using as keyword
Both the syntaxes are equivalent and we can use any of these type assertions syntaxes. However, while dealing with JSX in TypeScript, only the as syntax is allowed, because JSX is embeddable in XML like a syntax. And since XML uses angular brackets, it creates a conflict while using type assertions with angular brackets in JSX.
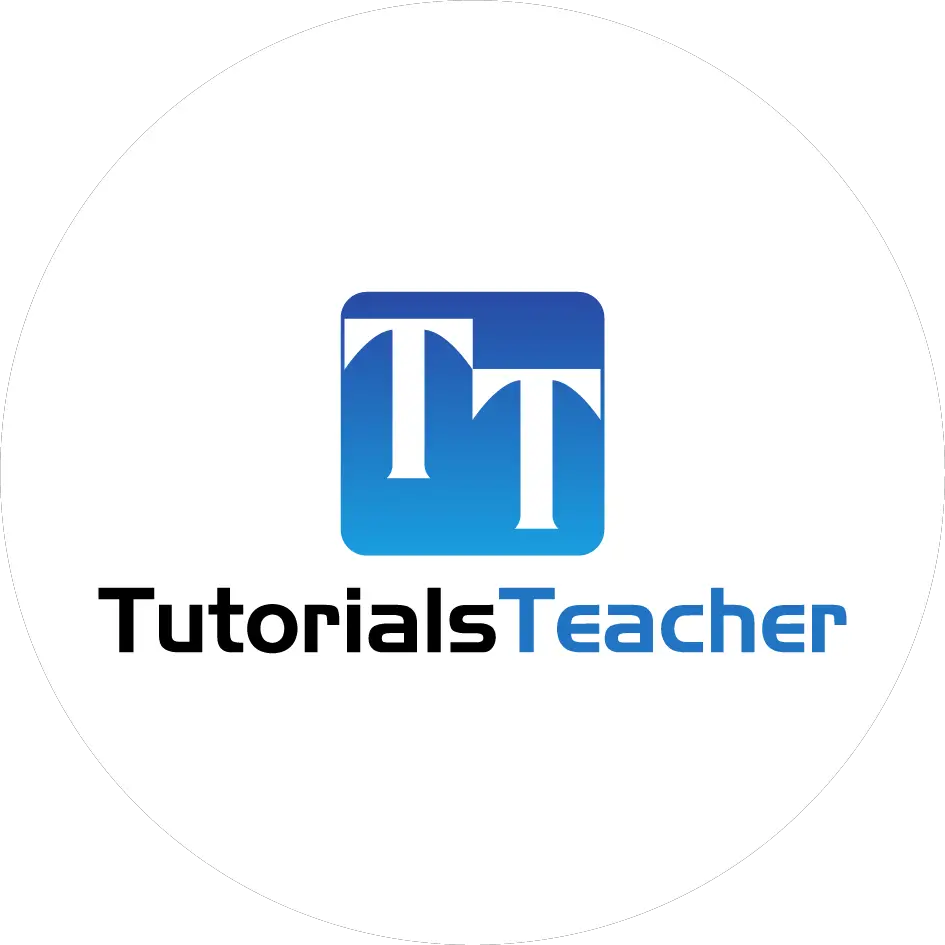
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
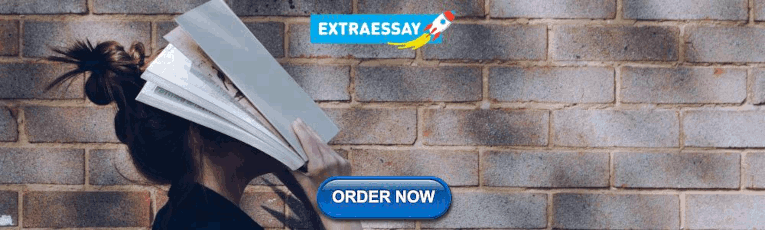
Golang Type Assertion Explained with Examples
October 4, 2022
Getting started with golang Type Assertion
A type assertion is an operation applied to an interface value. Syntactically, it looks like x.(T) , where x  is an expression of an interface type and T  is a type, called the âassertedâ type. A type assertion checks that the dynamic type of its operand matches the asserted type.
There are two possibilities. First, if the asserted type T  is a concrete type, then the type assertion checks whether xâs dynamic type is identical to T . If this check succeeds, the result of the type assertion is xâs dynamic value, whose type is of course T . In other words, a type assertion to a concrete type extracts the concrete value from its operand. If the check fails, then the operation panics. For example:
Second, if instead the asserted type T  is an interface type, then the type assertion checks whether xâs dynamic type satisfies T . If this check succeeds, the dynamic value is not extracted; the result is still an interface value with the same type and value components, but the result has the interface type T .
In other words, a type assertion to an interface type changes the type of the expression, making a different (and usually larger) set of methods accessible, but it preserves the dynamic type and value components inside the interface value.
After the first type assertion below, both w  and rw  hold os.Stdout  so each has a dynamic type of *os.File , but w , an io.Writer , exposes only the fileâs Write method, whereas rw  exposes its Read method too.
No matter what type was asserted, if the operand is a nil interface value, the type assertion fails. A type assertion to a less restrictive interface type (one with fewer methods) is rarely needed, as it behaves just like an assignment, except in the nil case.
Example 1: Simple example of using type assertion in Golang
The code shown below using type assertion to check the concrete type of an interface:
Explanation:
- The variable checkInterface is interface{} type which means it can hold any type.
- The concrete type that is assigned to the variable is string
- If the type assertion is against the int type, running this program will produce a run-time error because we have wrong type assertion.
Example 2: Check Type Assertion status using ok comma idiom
The below example use ok comma idiom to check assertion is successful or not:
Example 3: Logging type assertion errors
We can modify the code in example 3 a little bit to log more information when type assertion has errors. One way is we use the printf format string %T that produces the type.
Example 4: Using Type Switch to determine type of interface
What happens when you do not know the data type before attempting a type assertion? How can you differentiate between the supported data types and the unsupported ones? How can you choose a different action for each supported data type?
The answer is by using type switches . Type switches use switch blocks for data types and allow you to differentiate between type assertion values, which are data types, and process each data type the way you want. On the other hand, in order to use the empty interface in type switches, you need to use type assertions.
In this example, we will use Switch key word to determine interface's type.
- Create 2 interface: one contains a string and one contains a map
- Use the .(type) function and Switch key word to compare the type of underlying interface's data
A type assertion  is a mechanism for working with the underlying concrete value of an interface. This mainly happens because interfaces are virtual data types without their own valuesâinterfaces just define behavior and do not hold data of their own.
Type assertions use the x.(T)  notation, where x  is an interface type and T  is a type, and help you extract the value that is hidden behind the empty interface. For a type assertion to work, x  should not be nil  and the dynamic type of x  should be identical to the T  type.
https://pkg.go.dev/fmt
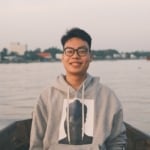
Tuan Nguyen
He is proficient in Golang, Python, Java, MongoDB, Selenium, Spring Boot, Kubernetes, Scrapy, API development, Docker, Data Scraping, PrimeFaces, Linux, Data Structures, and Data Mining. With expertise spanning these technologies, he develops robust solutions and implements efficient data processing and management strategies across various projects and platforms. You can connect with him on his LinkedIn profile.
Can't find what you're searching for? Let us assist you.
Enter your query below, and we'll provide instant results tailored to your needs.
If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation.
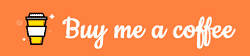
For any other feedbacks or questions you can send mail to [email protected]
Thank You for your support!!
Leave a Comment Cancel reply
Save my name and email in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
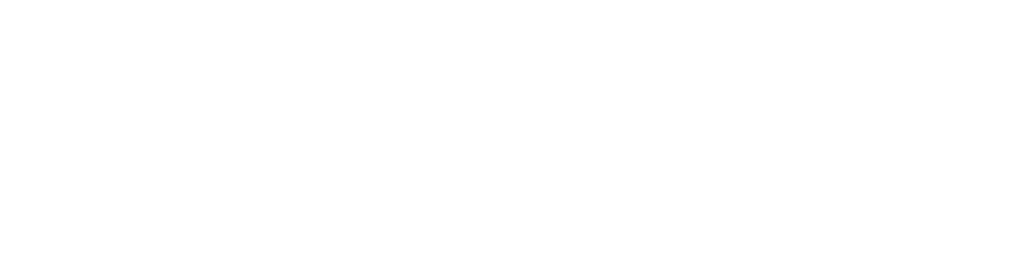
We try to offer easy-to-follow guides and tips on various topics such as Linux, Cloud Computing, Programming Languages, Ethical Hacking and much more.
Recent Comments
Popular posts, 7 tools to detect memory leaks with examples, 100+ linux commands cheat sheet & examples, tutorial: beginners guide on linux memory management, top 15 tools to monitor disk io performance with examples, overview on different disk types and disk interface types, 6 ssh authentication methods to secure connection (sshd_config), how to check security updates list & perform linux patch management rhel 6/7/8, 8 ways to prevent brute force ssh attacks in linux (centos/rhel 7).
Privacy Policy
HTML Sitemap
Mastering Type Assertion in TypeScript
Introduction.
TypeScriptâs type system is both powerful and complex, allowing developers to write safer and more predictable code. One particular feature of this type system is type assertion, which tells the compiler to consider an entity as a different type than the one it has inferred.
Getting Started with Type Assertions
To begin using type assertion, itâs important to recognize its syntax and basic usage. In TypeScript, you can assert a type using the angle-bracket syntax or the as keyword.
Note that type assertion does not perform any special checking or restructuring of data. It has no runtime impact; itâs purely a compile-time construct.
Type Assertions with Objects
Assertions are significantly more useful when dealing with objects or complex types.
This indicates to the TypeScript compiler what the shape of person is supposed to be, enforcing the correct property types.
Advanced Type Assertions
Moving to more complex scenarios, type assertions can be used to work with union types and type guards.
Type assertions can also be chained in complex control flow situations to narrow down the type as you refine your logic.
Assertion vs Casting
Itâs crucial to note that type assertion is not the same thing as casting in languages like Java or C#. Casting often implies some level of data transformation, while assertion is purely a compile-time signal to the TypeScriptâs type checker. Thereâs no runtime check or conversion that happens when you use a type assertion in TypeScript.
Code Example:
Type Assertion Best Practices
Type assertions should be used judiciously. Overusing them can lead to a false sense of security and potentially undermine the benefits of TypeScriptâs type system.
Itâs also a good practice to perform appropriate checks before asserting types to avoid runtime errors.
TypeScriptâs type assertion can be a powerful tool for developers, allowing you to provide more information to the compiler for effective type checking. Remember to use it wisely, in conjunction with type guards and proper programming patterns to exploit the full capabilities of TypeScriptâs type system without losing the safety it provides.
Next Article: TypeScript: Object with Optional Properties
Previous Article: How to Properly Use '!' (Exclamation Mark) in TypeScript
Series: The First Steps to TypeScript
Related Articles
- TypeScript Function to Convert Date Time to Time Ago (2 examples)
- TypeScript: setInterval() and clearInterval() methods (3 examples)
- TypeScript sessionStorage: CRUD example
- Using setTimeout() method with TypeScript (practical examples)
- Working with window.navigator object in TypeScript
- TypeScript: Scrolling to a specific location
- How to resize the current window in TypeScript
- TypeScript: Checking if an element is a descendant of another element
- TypeScript: Get the first/last child node of an element
- TypeScript window.getComputerStyle() method (with examples)
- Using element.classList.toggle() method in TypeScript (with examples)
- TypeScript element.classList.remove() method (with examples)
Search tutorials, examples, and resources
- PHP programming
- Symfony & Doctrine
- Laravel & Eloquent
- Tailwind CSS
- Sequelize.js
- Mongoose.js
Emerson Souza's Blog
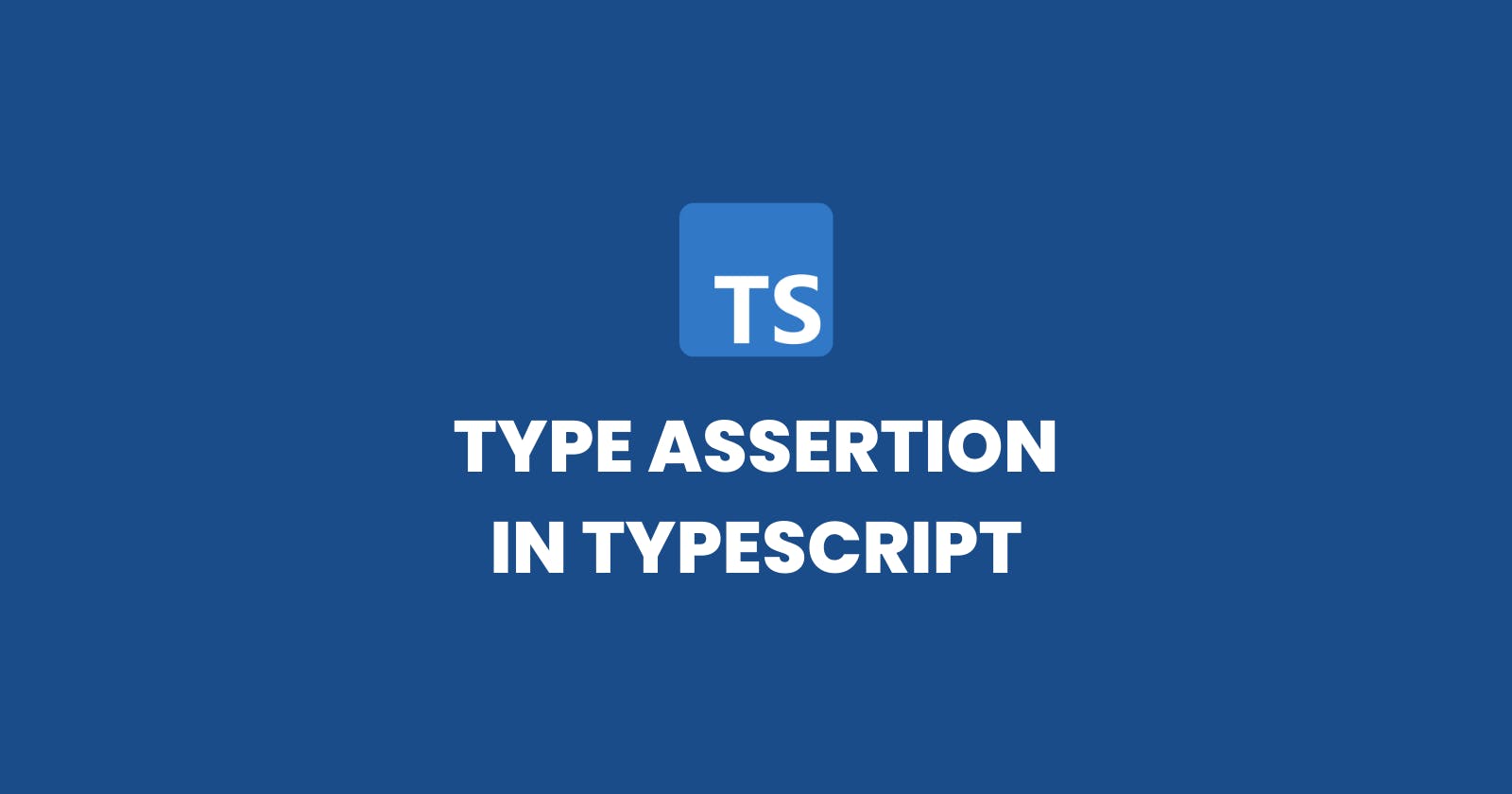
Mastering Type Assertion in TypeScript
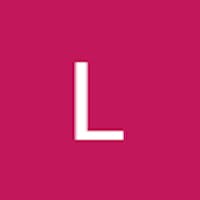
Type Assertion, also known as Type Casting, is a feature in TypeScript that allows you to explicitly define the type of a value when the type system cannot infer it automatically. It provides a way to override the default type inference and treat a value as a specific type.
Imagine you're working with an external library that doesn't have TypeScript support or has incomplete type definitions. In such cases, the type inference may not accurately capture the types of the library's API responses or objects. This lack of type safety can lead to potential bugs or limitations in your code.
Type Assertion enables you to assert the correct type for such scenarios. Let's say you have an API response that returns data in a generic response variable. By using type assertion, you can explicitly define the type of the response variable, ensuring proper type checking and enabling IDE auto-completion:
In this example, the as keyword is used for type assertion, where ApiResponse is the desired type. This allows you to work with parsedData confidently, leveraging TypeScript's type checking and auto-completion.
Type Assertion empowers developers to handle scenarios where type inference falls short, providing flexibility and control over types in TypeScript. It enables seamless integration with external libraries and allows for safer and more reliable coding practices.
Learn TypeScript
Using type assertions.
In a TypeScript program, a variable can move from a less precise type to a more precise type. This process is called type narrowing , and we'll learn all about this in this module. We'll start in this lesson by learning how to use type assertions to narrow the type of a variable.
Understanding the need for type assertions
Type assertions are helpful when we know more about a variable value than TypeScript does. We are going to explore type assertions in the code editor below:

The code contains a variable assignment from a DOM selector method.
What is the type of button ?
- Let's add code to disable the button:
A type error occurs. Why is this so?
It would be nice to narrow the type of button to HTMLButtonElement so that TypeScript recognizes the disabled property. Type assertions allow us to do this.
Angle-bracket syntax
There are two types of syntax for a type assertion. The first is to define the type in angle-brackets just before the value or expression we are asserting:
- Add the following type assertion to button on line 1:
What is the type of button now?
ESLint may warn about the use of the angle-bracket syntax because the "As" syntax is generally preferred. This is the case when using TypeScript in React apps because the React transpilation process may think the type assertion is a React component.
âAsâ syntax
The alternative and preferred syntax is to put the type after an as keyword after the expression:
- Update the code to use this syntax.
The inferred type of the button variable is still HTMLButtonElement .
We can use type assertions in our code to tell the TypeScript what a type should be when it infers it incorrectly. We should prefer the as syntax when using a type assertion.
Introduction
Using the non-null assertion operator, on this page.
Type assertions and type switches
A type assertion provides access to an interfaceâs concrete value.
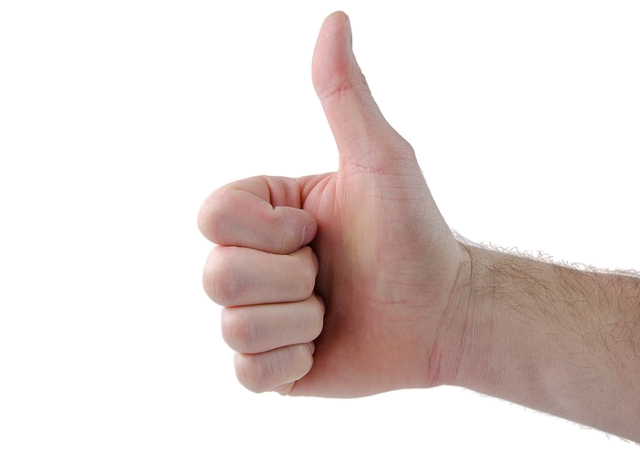
Type assertions
Type switches.
A type assertion doesnât really convert an interface to another data type, but it provides access to an interfaceâs concrete value, which is typically what you want.
The type assertion x.(T) asserts that the concrete value stored in x is of type T , and that x is not nil.
- If T is not an interface, it asserts that the dynamic type of x is identical to T .
- If T is an interface, it asserts that the dynamic type of x implements T .
A type switch performs several type assertions in series and runs the first case with a matching type.
Further reading
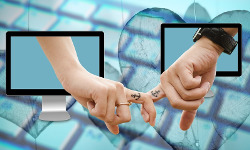
Type assertions in TypeScript
This blog post is about type assertions in TypeScript, which are related to type casts in other languages and performed via the as operator.
Type assertions #
A type assertion lets us override a static type that TypeScript has computed for a storage location. That is useful for working around limitations of the type system.
Type assertions are related to type casts in other languages, but they donât throw exceptions and donât do anything at runtime (they do perform a few minimal checks statically).
In line A, we widen the type of the Array to object .
In line B, we see that this type doesnât let us access any properties ( details ).
In line C, we use a type assertion (the operator as ) to tell TypeScript that data is an Array. Now we can access property .length .
Type assertions are a last resort and should be avoided as much as possible. They (temporarily) remove the safety net that the static type system normally gives us.
Note that, in line A, we also overrode TypeScriptâs static type. But we did it via a type annotation. This way of overriding is much safer than type assertions because there is much less you can do. TypeScriptâs type must be assignable to the type of the annotation.
Alternative syntax for type assertions #
TypeScript has an alternative âangle-bracketâ syntax for type assertions:
That syntax has grown out of style and is not compatible with React JSX code (in .tsx files).
Example: asserting an interface #
In order to access property .name of an arbitrary object obj , we temporarily change the static type of obj to Named (line A and line B).
Example: asserting an index signature #
In the following code (line A), we use the type assertion as Dict , so that we can access the properties of a value whose inferred type is object . That is, we are overriding the inferred static type object with the static type Dict .
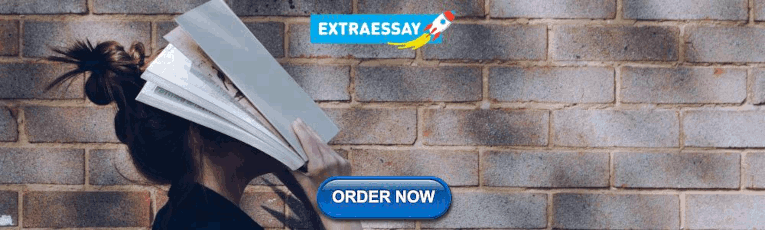
Constructs related to type assertions #
Non-nullish assertion operator (postfix ) #.
If a valueâs type is a union that includes the types undefined or null , the non-nullish assertion operator (or non-null assertion operator ) removes these types from the union. We are telling TypeScript: âThis value canât be undefined or null .â As a consequence, we can perform operations that are prevented by the types of these two values â for example:
Example â Maps: .get() after .has() #
After we use the Map method .has() , we know that a Map has a given key. Alas, the result of .get() does not reflect that knowledge, which is why we have to use the nullish assertion operator:
Since the values of strMap are never undefined , we can detect missing Map entries by checking if the result of .get() is undefined (line A):
Definite assignment assertions #
If strict property initialization is switched on, we occasionally need to tell TypeScript that we do initialize certain properties â even though it thinks we donât.
This is an example where TypeScript complains even though it shouldnât:
The errors go away if we use definite assignment assertions (exclamation marks) in line A and line B:
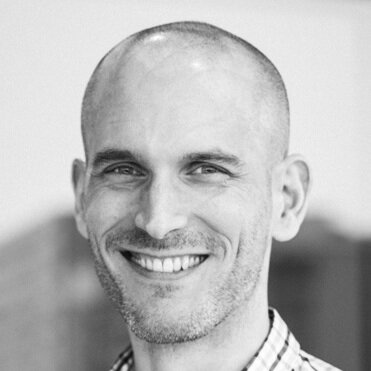
21 Type assertions (related to casting)
- 21.1.1 Alternative syntax for type assertions
- 21.1.2 Example: asserting an interface
- 21.1.3 Example: asserting an index signature
- 21.2.1 Non-nullish assertion operator (postfix ! )
- 21.2.2 Definite assignment assertions
This chapter is about type assertions in TypeScript, which are related to type casts in other languages and performed via the as operator.
21.1 Type assertions
A type assertion lets us override a static type that TypeScript has computed for a value. That is useful for working around limitations of the type system.
Type assertions are related to type casts in other languages, but they donât throw exceptions and donât do anything at runtime (they do perform a few minimal checks statically).
In line A, we widen the type of the Array to object .
In line B, we see that this type doesnât let us access any properties ( details ).
In line C, we use a type assertion (the operator as ) to tell TypeScript that data is an Array. Now we can access property .length .
Type assertions are a last resort and should be avoided as much as possible. They (temporarily) remove the safety net that the static type system normally gives us.
Note that, in line A, we also overrode TypeScriptâs static type. But we did it via a type annotation. This way of overriding is much safer than type assertions because we are much more constrained: TypeScriptâs type must be assignable to the type of the annotation.
21.1.1 Alternative syntax for type assertions
TypeScript has an alternative âangle-bracketâ syntax for type assertions:
I recommend avoiding this syntax. It has grown out of style and is not compatible with React JSX code (in .tsx files).
21.1.2 Example: asserting an interface
In order to access property .name of an arbitrary object obj , we temporarily change the static type of obj to Named (line A and line B).
21.1.3 Example: asserting an index signature
In the following code (line A), we use the type assertion as Dict , so that we can access the properties of a value whose inferred type is object . That is, we are overriding the static type object with the static type Dict .
21.2 Constructs related to type assertions
21.2.1 non-nullish assertion operator (postfix ).
If a valueâs type is a union that includes the types undefined or null , the non-nullish assertion operator (or non-null assertion operator ) removes these types from the union. We are telling TypeScript: âThis value canât be undefined or null .â As a consequence, we can perform operations that are prevented by the types of these two values â for example:
21.2.1.1 Example â Maps: .get() after .has()
After we use the Map method .has() , we know that a Map has a given key. Alas, the result of .get() does not reflect that knowledge, which is why we have to use the nullish assertion operator:
We can avoid the nullish assertion operator whenever the values of a Map canât be undefined . Then missing entries can be detected by checking if the result of .get() is undefined :
21.2.2 Definite assignment assertions
If strict property initialization is switched on, we occasionally need to tell TypeScript that we do initialize certain properties â even though it thinks we donât.
This is an example where TypeScript complains even though it shouldnât:
The errors go away if we use definite assignment assertions (exclamation marks) in line A and line B:
- Data Types in Go
- Go Keywords
- Go Control Flow
- Go Functions
- GoLang Structures
- GoLang Arrays
- GoLang Strings
- GoLang Pointers
- GoLang Interface
- GoLang Concurrency
Type Assertions in Golang
- Time Durations in Golang
- Type Casting or Type Conversion in Golang
- Comparing Maps in Golang
- Generics in Golang
- Composition in Golang
- Comparing Pointers in Golang
- bits Package in Golang
- Function as a Field in Golang Structure
- fmt Package in GoLang
- reflect.Type() Function in Golang with Examples
- Command Line Arguments in Golang
- Function Arguments in Golang
- Templates in GoLang
- Basics of JSON with GoLang
- How to use Ellipsis (...) in Golang?
- Type Switches in GoLang
- reflect.TypeOf() Function in Golang with Examples
- bits.Add() Function in Golang with Examples
- Defer Keyword in Golang
- Embedding Interfaces in Golang
- Structure Equality in Golang
- Recursive Anonymous Function in Golang
- filepath.Abs() Function in Golang With Examples
- complx.Exp() Function in Golang With Examples
- Explain Type assertions in TypeScript
- TypeScript Assertion functions
- TypeScript Assertions Type
- What is Type Assertion in TypeScript ?
Type assertions in Golang provide access to the exact type of variable of an interface. If already the data type is present in the interface, then it will retrieve the actual data type value held by the interface. A type assertion takes an interface value and extracts from it a value of the specified explicit type. Basically, it is used to remove the ambiguity from the interface variables.
where value is a variable whose type must be an interface, typeName is the concrete type we want to check and underlying typeName value is assigned to variable t .
In the above code, since the value interface does not hold an int type, the statement triggered panic and the type assertion fails. To check whether an interface value holds a specific type, it is possible for type assertion to return two values, the variable with typeName value and a boolean value that reports whether the assertion was successful or not. This is shown in the following example:
Please Login to comment...
Similar reads.
- Golang-Misc
- Go Language

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Definite Assignment Assertions (!)
The Definite Assignment Assertions or also called non-null assertion operator tells the TypeScript compiler that a value typed cannot be null or undefined which is a way to override the compiler's analysis and inform it that a variable will be assigned a value before it is used.
Advisory boards arenât only for executives. Join the LogRocket Content Advisory Board today →
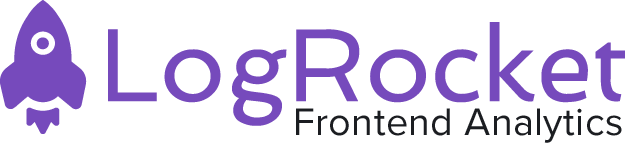
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Assertion functions in TypeScript

Assertion functions in TypeScript are a very expressive type of function whose signature states that a given condition is verified if the function itself returns.
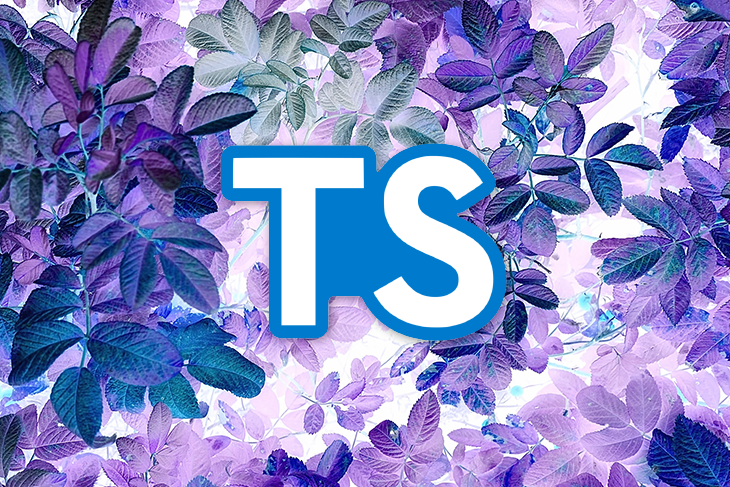
In its basic form, a typical assert function just checks a given predicate and throws an error if such a predicate is false. For example, Node.jsâs assert throws an AssertionError if the predicate is false.
TypeScript, since its version 3.7, has gone a little beyond that by implementing the support of assertions at the type system level.
In this article, weâre going to explore assertion functions in TypeScript and see how they can be used to express invariants on our variables.
Table of Contents
Javascript-like assertions, function declarations and expressions, assertion functions and type guards, assertion functions without a type predicate.
Node.js comes with a predefined assert function. As we mentioned in the introduction, it throws an AssertionError if a given predicate is false:
In JavaScript, this was useful to guard against improper types in a function:
Unfortunately, the code flow analysis does not take into account those assertions. In fact, they are simply evaluated at runtime and then forgotten.
With its assertion functions, TypeScriptâs code flow analysis will be able to use the type of a function (in brief, its signature) to infer some properties of our code. We can use this new feature to make guarantees of our types throughout our code.
TypeScript-like assertion
An assertion function specifies, in its signature, the type predicate to evaluate. For instance, the following function ensures a given value be a string :
If we invoke the function above with a given parameter, and it returns correctly, TypeScript knows that value has type string . Hence, it will narrow down its type to string :
Of course, nothing prevents us from messing up the assertion. For example, we could have written a (wrong) function as follows:
Note that weâre now checking whether value's type is not number , instead of string . In this case, TypeScriptâs code flow analysis will see a Value of type never , instead of string as above.
Assertion functions can be very useful with enums :
In the example above, we first defined a type whose value can only be either "r" , "w" , or "rw" . Letâs assume such a type simply defines the three types of access to a given resource. We then declare an assertion function throwing if its actual parameter does not allow a read operation.
As you can see, weâre narrowing down the type explicitly, stating that, if the function returns, the value must be either "r" or "rw" . If we call allowsReadAccess with writeOnly as the actual parameter, weâll get an error as expected, stating that "Read access is not allowed" .
Another common use of assertion functions is expressing non-nullability. The following snippet of code shows a way to make sure a value is defined, that is itâs not either null or undefined :
Where NonNullable<T> is a TypeScript type that excludes null and undefined from the legit values of the type T.
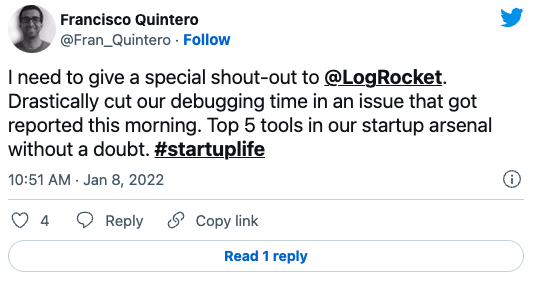
Over 200k developers use LogRocket to create better digital experiences

At the time of writing, assertion functions may not be defined as plain function expressions. Generally speaking, function expressions can be seen as anonymous functions; that is, functions without a name:
The main advantage of function declarations is hoisting, which is the possibility of using the function anywhere in the file where itâs defined. On the other hand, function expressions can only be used after they are created.
There is actually a workaround to write assertion functions as function expressions. Instead of defining the function along with its implementation, weâll have to define its signature as an isolated type:
Assertion functions in TypeScript are somewhat similar to type guards. Type guards were originally introduced to perform runtime checks to guarantee the type of a value in a given scope.
In particular, a type guard is a function that simply evaluates a type predicate, returning either true or false . This is slightly different from assertion functions, which, as we saw above, are supposed to throw an error instead of returning false if the predicate is not verified.
There is another big difference though. Assertion functions can also be used without a type predicate, as weâll see in the following section.
The assertion functions weâve seen so far were all checking whether a given value had a given type. Hence, they were all fairly tailored for the target type. Nonetheless, assertion functions give us much more power. In particular, we can write a completely general function asserting a condition that gets input as a parameter:
The assert function now inputs a condition , whose type is unknown , and, possibly, a message . Its body simply evaluates such a condition. If it is false , then assert throws an error, as expected.
Note, however, that the signature makes use of the condition parameter after asserts . This way, weâre telling TypeScript code flow analysis that, if the function returns correctly, it can assume that whatever predicate we passed in was, in fact, verified.
TypeScriptâs Playground gives us a pretty good visual representation of what the code flow analysis does. Letâs consider the following snippet of code, where we generate a random number and then call assert to make sure the generated number is 10 :
If we inspect the inferred properties of randomValue before the call to assert , TypeScript just tells us the type (Figure 1).
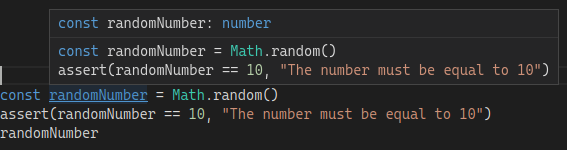
Then, as soon as we call assert , with the condition randomNumber == 10 , TypeScript knows that the value will be 10 for the rest of the execution (Figure 2).

Lastly, if we attempt to check the equality of randomNumber and another number, TypeScript will be able to evaluate the property without even running the program. For example, the code flow analysis will complain about the following assignment, saying, âThis condition will always return âfalseâ since the types â10â and â20â have no overlap.â:
In this article, we dove into what TypeScript assertion functions are and how we can use them to have the code flow analysis infer a set of properties about our values. They are a very nice feature that makes sense considering that TypeScript is transpiled to JavaScript, which gives programmers a lot more flexibility.
In particular, we took a look at a handful of usages, including narrowing types down and expressing conditions on the actual value of our variables. Lastly, we briefly mentioned the differences and similarities with type guards and grasped the syntactic limitations of assertions functions.
LogRocket : Full visibility into your web and mobile apps
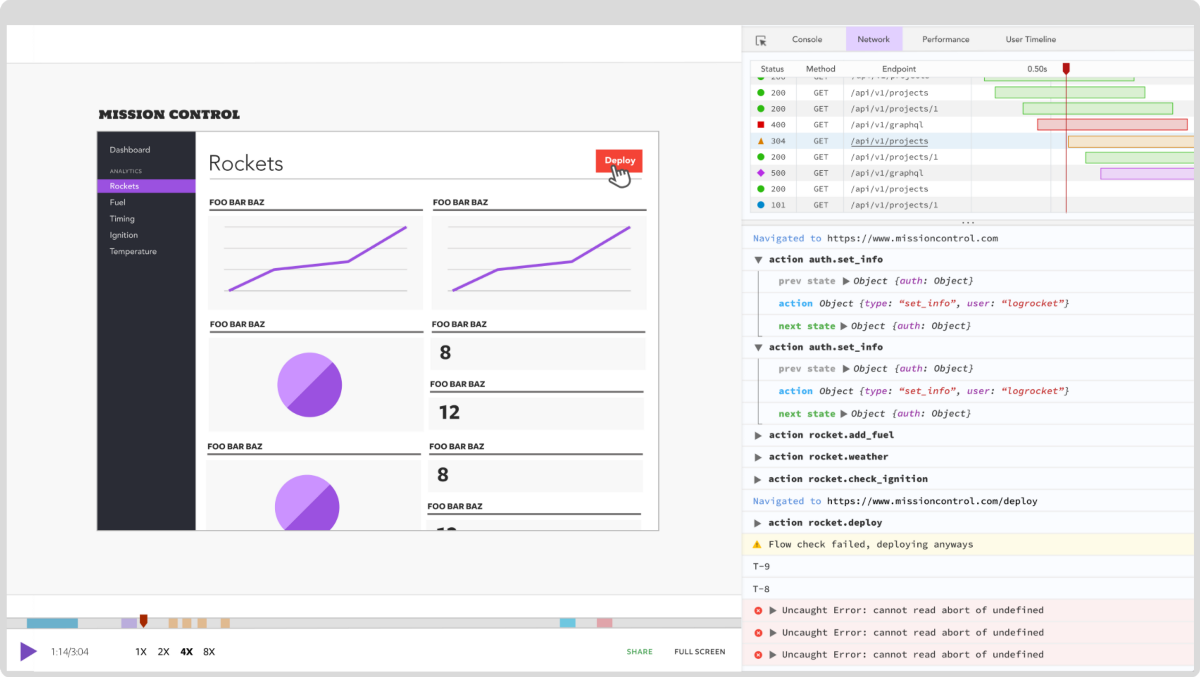
LogRocket is a frontend application monitoring solution that lets you replay problems as if they happened in your own browser. Instead of guessing why errors happen or asking users for screenshots and log dumps, LogRocket lets you replay the session to quickly understand what went wrong. It works perfectly with any app, regardless of framework, and has plugins to log additional context from Redux, Vuex, and @ngrx/store.
In addition to logging Redux actions and state, LogRocket records console logs, JavaScript errors, stacktraces, network requests/responses with headers + bodies, browser metadata, and custom logs. It also instruments the DOM to record the HTML and CSS on the page, recreating pixel-perfect videos of even the most complex single-page and mobile apps.
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #typescript
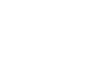
Stop guessing about your digital experience with LogRocket
Recent posts:.
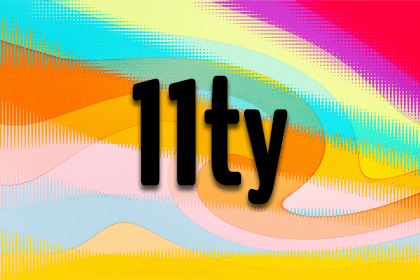
Eleventy adoption guide: Overview, examples, and alternatives
Eleventy (11ty) is a compelling solution for developers seeking a straightforward, performance-oriented approach to static site generation.
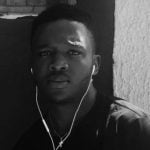
6 CSS tools for more efficient and flexible CSS handling
Explore some CSS tools that offer the perfect blend of efficiency and flexibility when handling CSS, such as styled-components and Emotion.
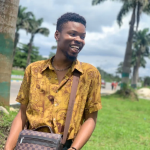
Leveraging React Server Components in RedwoodJS
RedwoodJS announced support for server-side rendering and RSCs in its Bighorn release. Explore this feature for when it’s production-ready.
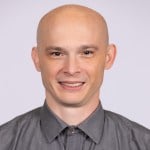
Exploring the AHA stack: Tutorial, demo, and comparison
The AHA stack â Astro, htmx, and Alpine â is a solid web development stack for smaller apps that emphasize frontend speed and SEO.
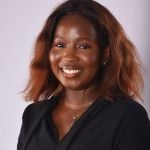
Leave a Reply Cancel reply
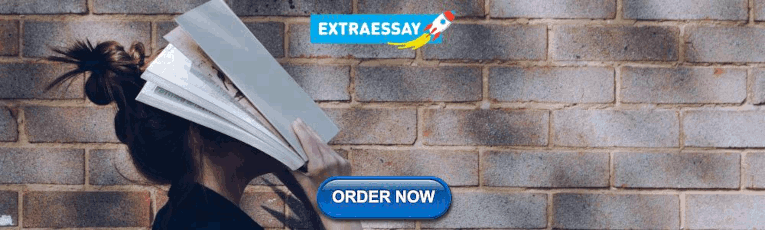
IMAGES
VIDEO
COMMENTS
Here is the explanation from a tour of go: A type assertion provides access to an interface value's underlying concrete value. This statement asserts that the interface value i holds the concrete type T and assigns the underlying T value to the variable t. If i does not hold a T, the statement will trigger a panic.
A type assertion does not impact the object you are asserting on. Instead, it returns the value stored in the interface alongside a success bool. This means that you need to save this returned value and use it. In your case, change your type assertion to the following: if res, ok := input[0].(int); ok {. tree.Val = res.
So, we set the type of return value as number because we know that it returns a number. There are two ways to do type assertion in TypeScript: 1. Using the angular bracket <> syntax. So far in this section, we have used angular brackets to show type assertion. let code: any = 123;
A type assertion is an operation applied to an interface value. Syntactically, it looks like x.(T), where x is an expression of an interface type and T is a type, called the "asserted" type. A type assertion checks that the dynamic type of its operand matches the asserted type. There are two possibilities. First, if the asserted type T is a ...
Type assertions can also be chained in complex control flow situations to narrow down the type as you refine your logic. Assertion vs Casting. It's crucial to note that type assertion is not the same thing as casting in languages like Java or C#. Casting often implies some level of data transformation, while assertion is purely a compile-time ...
Type Assertion, also known as Type Casting, is a feature in TypeScript that allows you to explicitly define the type of a value when the type system cannot infer it automatically. It provides a way to override the default type inference and treat a value as a specific type. Example. Imagine you're working with an external library that doesn't ...
A type can be assigned in different ways in TypeScript: In this example, the type J<Type\> uses a mapped type with a template literal to remap the keys of Type. It creates new properties with a prefix. _ added to each key, and their corresponding values are functions returning the original property values.. It is worth noting that when using a type assertion, TypeScript will not execute excess ...
Angle-bracket syntax. There are two types of syntax for a type assertion. The first is to define the type in angle-brackets just before the value or expression we are asserting: <TypeName>expression; Add the following type assertion to button on line 1: const button = <HTMLButtonElement>document.querySelector(".go"); đ€.
Type assertions; Type switches; Type assertions. A type assertion doesn't really convert an interface to another data type, but it provides access to an interface's concrete value, which is typically what you want.. The type assertion x.(T) asserts that the concrete value stored in x is of type T, and that x is not nil.. If T is not an interface, it asserts that the dynamic type of x is ...
To fix this, move the definition of person to the top level (that is, move it out of the body of main), and change FromJson` to this: var obj person{} json.Unmarshal([]byte(jsonSrc), &obj) return obj. Now, when you return obj, the interface{} that's returned has person as its underlying type.
Type assertion in Go. In Go, the syntax for type assertions is t := i.(type). Here is a snippet of a full type assertion operation: t := i.(string) // "a string" } The type assertion operation consists of three main elements: i, which is the variable whose type we are asserting. This variable must be defined as an interface.
Type assertions. A type assertion provides access to an interface value's underlying concrete value.. t := i.(T) This statement asserts that the interface value i holds the concrete type T and assigns the underlying T value to the variable t.. If i does not hold a T, the statement will trigger a panic.. To test whether an interface value holds a specific type, a type assertion can return two ...
In the following code (line A), we use the type assertion as Dict, so that we can access the properties of a value whose inferred type is object. That is, we are overriding the inferred static type object with the static type Dict. type Dict = {[k:string]: any}; function getPropertyValue(dict: unknown, key: string): any {.
The syntax is similar to type assertion but type keyword is used. Output is nil ( source code) since the value of interface type value is nil but if we'll set value of v instead: var v I1 = T2 ...
21.2 Constructs related to type assertions 21.2.1 Non-nullish assertion operator (postfix !. If a value's type is a union that includes the types undefined or null, the non-nullish assertion operator (or non-null assertion operator) removes these types from the union.We are telling TypeScript: "This value can't be undefined or null."As a consequence, we can perform operations that are ...
The non-null assertion operator is useful when the developer knows that a value will not be null or undefined, but the TypeScript compiler is unable to infer this based on the type of the value ...
A type assertion takes an interface value and extracts from it a value of the specified explicit type. Basically, it is used to remove the ambiguity from the interface variables. Syntax: where value is a variable whose type must be an interface, typeName is the concrete type we want to check and underlying typeName value is assigned to variable t.
Definite Assignment Assertions (!) The Definite Assignment Assertions or also called non-null assertion operator tells the TypeScript compiler that a value typed cannot be null or undefined which is a way to override the compiler's analysis and inform it that a variable will be assigned a value before it is used. type Person = {. name: string; };
A solution to your problem, simply initiate the map as an empty interface of empty interfaces: m := map[interface{}]interface{} then you can assign any type key or value you want in the 'ReturnTuples' function. playground example. NOTE: remember that if you want to use the values later as the original types, you will need to use type assertion ...
TypeScript, since its version 3.7, has gone a little beyond that by implementing the support of assertions at the type system level. In this article, we're going to explore assertion functions in TypeScript and see how they can be used to express invariants on our variables. ... value is string { return typeof value === "string" } // Type ...