- Language Reference
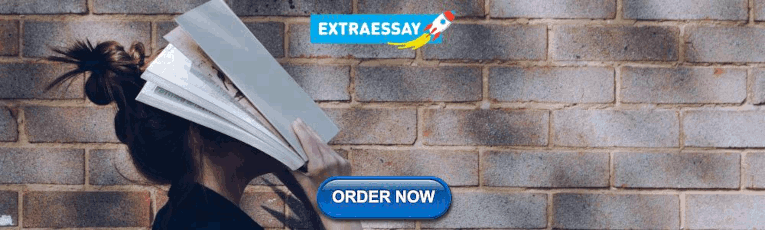
String Operators
There are two string operators. The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (' .= '), which appends the argument on the right side to the argument on the left side. Please read Assignment Operators for more information.
<?php $a = "Hello " ; $b = $a . "World!" ; // now $b contains "Hello World!" $a = "Hello " ; $a .= "World!" ; // now $a contains "Hello World!" ?>
- String type
- String functions
Improve This Page
User contributed notes 6 notes.

PHP Concatenation Operators
Php tutorial index.
PHP Compensation Operator is used to combine character strings.
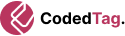
- PHP String Operators
In PHP, string operators, such as the concatenation operator (.) and its assignment variant (.=), are employed for manipulating and concatenating strings in PHP. This entails combining two or more strings. The concatenation assignment operator (.=) is particularly useful for appending the right operand to the left operand.
Let’s explore these operators in more detail:
Concatenation Operator (.)
The concatenation operator (.) is utilized to combine two strings. Here’s an example:
You can concatenate more than two strings by chaining multiple concatenation operations.
Let’s take a look at another pattern of the concatenation operator, specifically the concatenation assignment operator.
Concatenation Assignment Operator (.=)
The .= operator is a shorthand assignment operator that concatenates the right operand to the left operand and assigns the result to the left operand. This is particularly useful for building strings incrementally:
This is equivalent to $greeting = $greeting . " World!"; .
Let’s see some examples
Examples of Concatenating Strings in PHP
Here are some more advanced examples demonstrating the use of both the concatenation operator (.) and the concatenation assignment operator (.=) in PHP:
Concatenation Operator ( . ):
In this example, the . operator is used to concatenate multiple strings and variables into a single string.
Concatenation Assignment Operator ( .=) :
Here, the .= operator is used to append additional text to the existing string in the $paragraph variable. It is a convenient way to build up a string gradually.
Concatenation Within Iterations:
You can also use concatenation within iterations to build strings dynamically. Here’s an example using a loop to concatenate numbers from 1 to 5:
In this example, the .= operator is used within the for loop to concatenate the current number and a string to the existing $result string. The loop iterates from 1 to 5, building the final string. The rtrim function is then used to remove the trailing comma and space.
You can adapt this concept to various scenarios where you need to dynamically build strings within loops, such as constructing lists, sentences, or any other formatted output.
These examples showcase how you can use string concatenation operators in PHP to create more complex strings by combining variables, literals, iterations and other strings.
Let’s summarize it.
Wrapping Up
PHP provides powerful string operators that are essential for manipulating and concatenating strings. The primary concatenation operator (.) allows for the seamless combination of strings, while the concatenation assignment operator (.=) provides a convenient means of appending content to existing strings.
This versatility is demonstrated through various examples, including simple concatenation operations, the use of concatenation assignment for gradual string construction, and dynamic string building within iterations.
For more PHP tutorials, visit here or visit PHP Manual .
Did you find this article helpful?
Sorry about that. How can we improve it ?
- Facebook -->
- Twitter -->
- Linked In -->
- Install PHP
- Hello World
- PHP Constant
- PHP Comments
PHP Functions
- Parameters and Arguments
- Anonymous Functions
- Variable Function
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
Control Structures
- If-else Block
- Break Statement
PHP Operators
- Operator Precedence
- PHP Arithmetic Operators
- Assignment Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- Array Operators
- Conditional Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
- Null Coalescing Operator
Data Format and Types
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
String and Patterns
- Remove the Last Char
Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Addition assignment (+=)
The addition assignment ( += ) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand.
Description
x += y is equivalent to x = x + y , except that the expression x is only evaluated once.
Using addition assignment
Specifications, browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Addition ( + )
- PHP Tutorial
- PHP Exercises
- PHP Calendar
- PHP Filesystem
- PHP Programs
- PHP Array Programs
- PHP String Programs
- PHP Interview Questions
- PHP IntlChar
- PHP Image Processing
- PHP Formatter
- Web Technology
- How to Concatenate Strings in PHP ?
- How to Concatenate Two Arrays in PHP ?
- How to append a string in PHP ?
- How to convert array to string in PHP ?
- How to write Multi-Line Strings in PHP ?
- How to get a substring between two strings in PHP?
- How to add a Character to String in PHP ?
- How to prepend a string in PHP ?
- How to replace multiple characters in a string in PHP ?
- PHP Program to Check if Two Strings are Same or Not
- PHP Program to Split & Join a String
- How to put string in array, split by new line in PHP ?
- How to create a string by joining the array elements using PHP ?
- Concatenating Two Strings in C
- Shell Script to Concatenate Two Strings
- Batch Script - String Concatenation
- CONCAT_WS() Function in SQL Server
- Bash Concatenate String
- CONCAT_WS() Function in MySQL
Concatenation of two strings in PHP
There are two string operators. The first is the concatenation operator (‘ . ‘), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (‘ .= ‘), which appends the argument on the right side to the argument on the left side.
Code #1:
Time complexity : O(n) Auxiliary Space : O(n)
Code #2 :
Code #3 :
Code #4 :
PHP is a server-side scripting language designed specifically for web development. You can learn PHP from the ground up by following this PHP Tutorial and PHP Examples .
Please Login to comment...
Similar reads.
- Web Technologies
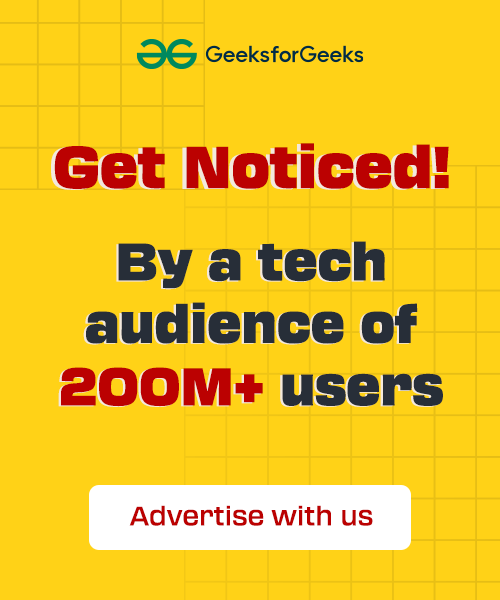
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
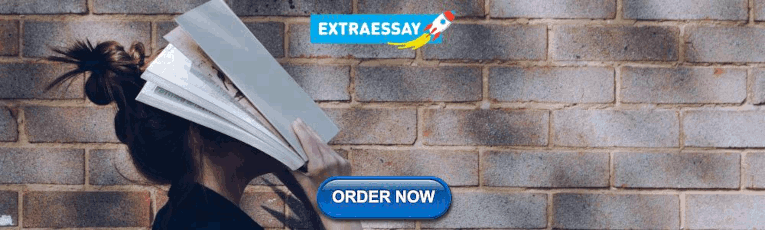
Concatenate strings in PHP
This article demonstrates how to concatenate strings in PHP.
1. Using Concatenation Operator
Unlike other programming languages, which use the + operator for concatenating two strings, PHP uses the . concatenation operator for string concatenation. It returns the concatenation of its right and left arguments.
Download Run Code
Consider using the concatenating assignment operator ( .= ) to append one string to another. It appends the argument on the right side to the argument on the left side.
2. Using Variable Interpolation
The string concatenation using the . operator in PHP might result in performance overhead from constructing lots of intermediate strings along the way. For concatenating more than two strings, variable interpolation is a better choice. Using the curly braces syntax, you can interpolate a variable in a string literal. When a double-quoted string is processed by PHP, any interpolated variables will be parsed and replaced with their corresponding values.
It should be noted that the variable interpolation will only work in double-quoted strings. Interpolating a variable into a single-quoted string will result in the literal name of the supplied variable. For example, the following code tries to interpolate variables into a string literal enclosed in single quotes.
The above syntax encloses the variable name in curly braces for better visibility. However, it is also possible to interpolate variables within a string literal with double quotes without curly braces:
3. Using Heredoc syntax
Another option to concatenate strings in PHP is the Heredoc text. It behaves like a double-quoted string, without the double quotes. It has the syntax: <<< which is followed by an identifier, a newline, and the string itself. Finally, the same identifier is provided to close the quotation.
4. Using sprintf() function
If you want to apply some formatting options to the strings, you can use the sprintf() function for string concatenation. This is likely to run slower than all of the above proposed solutions. The printf() function can also be used if you want to output the result.
5. Using echo with commas
Finally, you can directly pass your strings to the echo statement, separated by commas ( , ). This will result in a minor speed improvement over the string concatenation operator ( . ), but will output the results and not assign them to a variable.
That’s all about concatenating strings in PHP.
Convert an integer or a float to a string in PHP
Rate this post
Average rating 5 /5. Vote count: 1
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Software Engineer | Content Writer | 12+ years experience
🌟 Dreaming of a bright future? 🎓 Ask about the Treehouse Scholarship program! 🚀
🤖 Level up your chatbot knowledge with our latest AI course.
Join our free community Discord server here !
Learn React with us !
Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Start a free Courses trial to watch this video
Combining Strings
Combining data in a string is called concatenation. Besides using double quotes to add another variable into a string, PHP string concatenation also provides two string operators.
- Teacher's Notes
- Video Transcript
Concatenation
There are two string operators . The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator ('.='), which appends the argument on the right side to the argument on the left side. Please read Assignment Operators for more information.
To summarize concatenation. If you want to add a single variable to a string you can include that variable within your double quoted assignment. If you are concatenating a single line you would use a single dot between each string. If you are concatenating multiple lines, you should use the .= assignment operator.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Combining data in a string is called concatenation. 0:00 We perform concatenation in the last video 0:04 by adding one string variable to another string value. 0:07 By using double quotes, we included the first variable 0:10 into the string being assigned to the second variable. 0:14 PHP also provides two string operators for concatenation. 0:16 The dot and the dot equals. 0:22 Let's take a look at how to use these operators for more concatenation options. 0:25 The first string operator for concatenation is a single dot, or period. 0:30 The dot allows us to add as many strings together as we want in one statement. 0:35 For example, let me duplicate this line, then I can show you how to do 0:40 this same thing using single quotes, but still show the value of the variable. 0:44 We end this string here and concatenate with a single dot, 0:49 concatenate again, and start our final string. 0:54 Our string works, and our variable value is displayed. 1:03 But our escape sequences aren't working properly. 1:06 We can remove the escape character from before the double quotes, and 1:10 then we can concatenate this escape sequence, the /n, to the end. 1:15 Now when we run our script, just like we want. 1:28 Remember, PHP doesn't care about extra white spaces. 1:32 So as long as you haven't finished your statement with a semicolon, 1:35 you can split this line up however you want. 1:38 Be aware that if you add a line break within the quotes, 1:50 that line break will be displayed. 1:53 This could be a good or bad thing, depending on the desired results. 1:56 Early on I said, with great power comes great responsibility. 2:01 So just because you can concatenate your string over multiple lines 2:05 doesn't mean that you should, splitting up a statement 2:09 into multiple lines can make it extremely hard to follow. 2:12 If you are going to split the line, try to make it as easy to read as possible. 2:15 Indent the next lines and start each line with a concatenation character. 2:20 Notice that extra space right before Hello Alena? 2:39 That's because our string is split between two lines, and it's within single quotes. 2:43 So it's trying to display the characters exactly as they're shown. 2:49 I could end my string here, and then start my string again down here. 2:53 Now we have a single line. 3:03 Now this is easier to read, but 3:05 it's probably not the best choice if you're going to use multiple lines. 3:07 You're probably better off using the second operator, the dot equals. 3:11 This concatenation assignment operator appends or 3:15 adds the argument on the right side to the variable on the left side. 3:19 This can make multiple lines easier to read because 3:24 each line is a complete statement. 3:27 You finish each line with a semicolon, and 3:30 then you add to the variable, string one, 3:35 dot equals, Hello, end with a semicolon, 3:40 and again, string one, 3:45 dot equals name, end with a semicolon. 3:48 String one, dot equals, 3:56 end with a semicolon, and our last line. 3:59 Now let's run this script again. 4:10 Same thing. 4:13 This is the same as saying string one equals string 4:15 one concatenated to this next string, 4:19 because it appends or adds the new value to the end of the string. 4:24 If I wanted to add a string to the beginning instead of the end, 4:30 I could do just like this line, but instead, 4:35 I add to the beginning, I am, in the concatenation. 4:39 Now let's run this script. 4:47 I am learning to display Hello Alena to the screen. 4:50 Besides making multiple lines easier to read, 4:54 making each line a separate statement will allow you to add other 4:57 code between the lines, such as adding a comment. 5:01 Prepend to a string. 5:07 To summarize concatenation, if you want to add a single variable to a string, 5:12 you can include that variable within your double quoted assignment. 5:17 If you are concatenating a single line, 5:21 you would use a single dot between each string. 5:24 If you are concatenating multiple lines, 5:28 you should use the dot equals assignment operator. 5:30
You need to sign up for Treehouse in order to download course files.
You need to sign up for Treehouse in order to set up Workspace
Primary links
- HTML Color Chart
- Knowledge Base
- Devguru Resume
- Testimonials
- Privacy Policy
Font-size: A A A
PHP » Operators » .=
Concatenation assignment operator.
Concatenates the left operand and the right operand.
Explanation:
A variable is changed with an assignment operator.

© Copyright 1999-2018 by Infinite Software Solutions, Inc. All rights reserved.
Trademark Information | Privacy Policy
Event Management Software and Association Management Software
Know the Code
Developing & Empowering WordPress Developers
Unlock your potential with a Pro Membership
Here is where you propel your career forward. Come join us today. Learn more.
Login to your account
PHP 101: Concatenating Assignment Operator
Lab: wordpress custom taxonomy basics.
Video Runtime: 02:26
The term “concatenating” means that we are smooshing two strings together by appending the one on the right to the one on the left. The result is a new string value.
For example, let’s say that a variable $post_meta has a value of '[post_categories] [post_tags]' . You want to append another shortcode to the end of it. How do you do that?
There are two different ways to achieve this and make an assignment.
Long Hand Approach
To concatenate an existing variable’s string value to some value you are processing, you have a couple of choices in PHP. You can do it with the long hand approach, like this:
$post_meta = $post_meta . ' [post_terms taxonomy="department"]';
where the shortcode’s string literal is smooshed together to the end of the string value in the variable $post_meta . Then the new string is assigned back to the variable. That’s the long hand version.
Short Hand Approach
PHP provides you with a concatenating assignment operator as a shorthand approach:
$post_meta .= ‘ [post_terms taxonomy=”department”]’;
This code works the same as the full version; however, it’s more condensed. It eliminates the repetitious repeating of the variable. Therefore, this approach is more readable and maintainable.
This approach is very popular and prevalent! Make sure you understand it completely!
What’s the Sequence and Result?
Let’s walk through the processing and look at the result.
Step 1: Concatenate
First the variable’s value and string literal are concatenated to form a new string value of:
'[post_categories] [post_tags] [post_terms taxonomy="department"]';
Step 2: Assignment
The next step is to assign the new string to the variable $post_meta , which means the value it represents is changed to:
$post_meta = '[post_categories] [post_tags] [post_terms taxonomy="department"]';
This Episode
In this episode, let’s talk about the process of concatenating. Then we’ll walk through how each of these approaches works. Finally, we’ll see the results.
Code Challenge
Let’s challenge you. Ready? When this function is called, a string literal of '[post_categories]' is passed to it and assigned to the parameter $html . What is the value returned when the function is done running?
Answer : '[post_categories] [post_terms taxonomy="department"]'
Why? PHP concatenates the incoming value with the string literal and then assigns it back to the variable. Then that variable’s value is returned.
Did you get it right? If yes, way to go!! If no, watch the video and if it still doesn’t make sense, come ask me in the Pro Forums.
Code. Eat. Code. Sleep. Dream about Code. Code.
Total Lab Runtime: 01:30:53
- 1 Lab Introduction free 08:15
- 2 Custom Taxonomy - The What, Why, and When free 08:32
- 3 Registering a Custom Taxonomy pro 09:54
- 4 Configure the Labels pro 14:51
- 5 Bind to Post Types pro 11:55
- 6 Configuring Arguments pro 07:52
- 7 Render Entry Footer Terms pro 08:40
- 8 PHP 101: Concatenating Assignment Operator pro 02:26
- 9 PHP 101: Building Strings pro 08:19
- 10 Test Entry Footer Terms pro 03:03
- 11 Flush Rewrite Rules pro 03:56
- 12 Wrap it Up pro 03:10
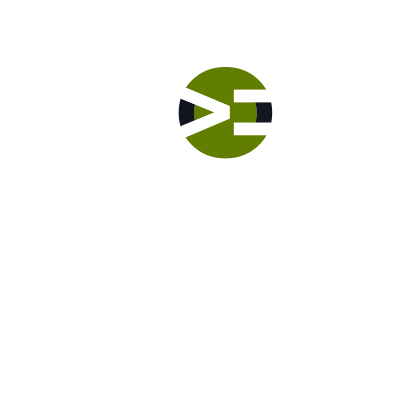
Know the Code develops and empowers professional WordPress developers, like you. We help you to grow, innovate, and prosper.
- Mastery Libraries
- What’s New
- Help Center
- Developer Stories
- My Dashboard
- WP Developers’ Club
Know the Code flies on WP Engine . Check out the managed hosting solutions from WP Engine.
WordPress® and its related trademarks are registered trademarks of the WordPress Foundation. The Genesis framework and its related trademarks are registered trademarks of StudioPress. This website is not affiliated with or sponsored by Automattic, Inc., the WordPress Foundation, or the WordPress® Open Source Project.

PHP Tutorial
Control statement, php programs, php functions, php strings, php include, state management, upload download, php oops concepts, related tutorials.
Interview Questions
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

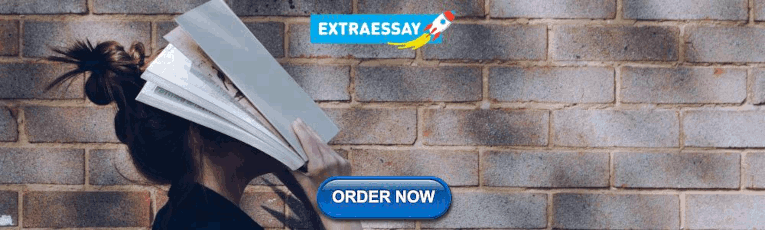
IMAGES
VIDEO
COMMENTS
There are two string operators. The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (' .= '), which appends the argument on the right side to the argument on the left side. Please read Assignment Operators for more information.
PHP Compensation Operator is used to combine character strings. Operator. Description. . The PHP concatenation operator (.) is used to combine two string values to create one string. .=. Concatenation assignment.
In this PHP programming guide, we take a look at The Concatenating Assignment Operator, Codecademy's Learn PHP, Concat Assignment Operator in PHP. In this l...
The primary concatenation operator (.) allows for the seamless combination of strings, while the concatenation assignment operator (.=) provides a convenient means of appending content to existing strings. This versatility is demonstrated through various examples, including simple concatenation operations, the use of concatenation assignment ...
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
The addition assignment (+=) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand. Try it. Syntax. js. x += y Description. x += y is equivalent to x = x + y, except that the expression x is only evaluated once.
Use the Concatenation Assignment Operator to Concatenate Strings in PHP. In PHP, we can also use the concatenation assignment operator to concatenate strings. The concatenation assignment operator is .=. The difference between .= and . is that the concatenation assignment operator .= appends the string on the right side. The correct syntax to ...
There are two string operators. The first is the concatenation operator ('. '), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (' .= '), which appends the argument on the right side to the argument on the left side. Examples : string2 : World! Output : HelloWorld!
This article demonstrates how to concatenate strings in PHP. 1. Using Concatenation Operator. Unlike other programming languages, which use the + operator for concatenating two strings, PHP uses the . concatenation operator for string concatenation. It returns the concatenation of its right and left arguments. 1.
With the "Concatenating Assignment Operator" I assigned in the loop two variables. I need to get each loop result separately. The problem is that I don't know why each next loop result is copied to each next loop result.
The second is the concatenating assignment operator ('.='), which appends the argument on the right side to the argument on the left side. Please read Assignment Operators for more information. To summarize concatenation. If you want to add a single variable to a string you can include that variable within your double quoted assignment.
PHP » Operators » .= Syntax: $var .= expressionvarA variable.expressionA value to concatenate the variable with.Concatenation assignment operator.
PHP 101: Concatenating Assignment Operator Lab: WordPress Custom Taxonomy Basics. Video Runtime: 02:26. Skip to episode playlist. The term "concatenating" means that we are smooshing two strings together by appending the one on the right to the one on the left. The result is a new string value.
Operations to the right of the assignment operator will be evaluated before assignment takes place. The concatenating assignment operator (.=) is a shorthand notation for reassigning a string variable to its current value appended with another string value. If that was a lot to take in, don't worry about memorizing everything right away.
Concatenating Assignment operator (".="): This operation appends the argument on the right side to the argument on the left side. Let's take the various examples of how to use the Concatenation Assignment Operator (".=") to concatenate the strings in PHP. Example 1:
Codecademy is the easiest way to learn how to code. It's interactive, fun, and you can do it with your friends.
String Operators. There are two string operators : concatenation operator ('.') and concatenating assignment operator ('.='). Example : PHP string concatenation operator
Please see below code. str += '<br>Multiply & assign: ' + initB; I get that the += operator, when dealing with strings concatenates the two operands and when dealing with numbers they add the values of the operands and reassign to the computed value to first operand. I also get that str is being initialised as 'Add & assign string ' + msg; and ...
3. Because numbers with leading zeroes are treated as octals. if the concatenation would've included the leading zeroes, then 006 would've been interpretted as a string, which makes no sense, because it hasn't got quotes around it. If you want 006 to be treated as a string, write it as one: '006'. Leave it as is, and it'll be interpreted as an ...
Assignment operator concatenating instead of copying. Ask Question Asked 10 years, 11 months ago. Modified 10 years, 11 months ago. Viewed 184 times 1 I'm having a hard time figuring out my assignment operator in a specific case. I have two arrays of objects, one in the other.